如何用Python做一个圆柱体
用Python制作一个圆柱体可以通过计算其体积和表面积来实现、使用图形库绘制三维图形、创建虚拟环境来进行模拟。其中,使用图形库如Matplotlib或PyOpenGL绘制三维图形是最常见的方法。本文将详细介绍如何使用Python进行圆柱体的计算和绘制,以及在虚拟环境中的应用。
一、计算圆柱体的基本属性
在开始绘制圆柱体之前,首先需要了解如何计算其基本属性,如体积和表面积。
1、圆柱体体积计算
体积公式为:
[ V = \pi r^2 h ]
其中,( r ) 是底面半径,( h ) 是高。
import math
def cylinder_volume(radius, height):
return math.pi * radius 2 * height
radius = 5
height = 10
volume = cylinder_volume(radius, height)
print(f"圆柱体的体积: {volume}")
2、圆柱体表面积计算
表面积公式为:
[ A = 2\pi r (r + h) ]
def cylinder_surface_area(radius, height):
return 2 * math.pi * radius * (radius + height)
surface_area = cylinder_surface_area(radius, height)
print(f"圆柱体的表面积: {surface_area}")
二、使用Matplotlib绘制三维圆柱体
1、安装Matplotlib
在绘制圆柱体之前,需要确保已安装Matplotlib:
pip install matplotlib
2、绘制圆柱体
使用Matplotlib中的Axes3D
模块可以轻松绘制三维图形。
import numpy as np
import matplotlib.pyplot as plt
from mpl_toolkits.mplot3d import Axes3D
def plot_cylinder(radius, height):
fig = plt.figure()
ax = fig.add_subplot(111, projection='3d')
z = np.linspace(0, height, 50)
theta = np.linspace(0, 2 * np.pi, 50)
theta_grid, z_grid = np.meshgrid(theta, z)
x_grid = radius * np.cos(theta_grid)
y_grid = radius * np.sin(theta_grid)
ax.plot_surface(x_grid, y_grid, z_grid, alpha=0.5, rstride=100, cstride=100)
plt.show()
plot_cylinder(radius, height)
三、使用PyOpenGL绘制三维圆柱体
1、安装PyOpenGL
首先需要安装PyOpenGL:
pip install PyOpenGL PyOpenGL_accelerate
2、绘制圆柱体
使用PyOpenGL可以创建更为复杂和交互性的三维图形。
from OpenGL.GL import *
from OpenGL.GLUT import *
from OpenGL.GLU import *
import math
def draw_cylinder(radius, height, slices=50):
glBegin(GL_QUAD_STRIP)
for i in range(slices + 1):
angle = 2 * math.pi * i / slices
x = radius * math.cos(angle)
y = radius * math.sin(angle)
glVertex3f(x, y, 0)
glVertex3f(x, y, height)
glEnd()
glBegin(GL_POLYGON)
for i in range(slices + 1):
angle = 2 * math.pi * i / slices
x = radius * math.cos(angle)
y = radius * math.sin(angle)
glVertex3f(x, y, 0)
glEnd()
glBegin(GL_POLYGON)
for i in range(slices + 1):
angle = 2 * math.pi * i / slices
x = radius * math.cos(angle)
y = radius * math.sin(angle)
glVertex3f(x, y, height)
glEnd()
def display():
glClear(GL_COLOR_BUFFER_BIT | GL_DEPTH_BUFFER_BIT)
draw_cylinder(1, 2)
glutSwapBuffers()
glutInit()
glutInitDisplayMode(GLUT_DOUBLE | GLUT_RGB | GLUT_DEPTH)
glutCreateWindow("3D Cylinder")
glutDisplayFunc(display)
glutMainLoop()
四、在虚拟环境中应用
1、创建虚拟环境
在使用Python进行复杂项目时,建议创建虚拟环境以管理依赖:
python -m venv myenv
source myenv/bin/activate # On Windows use `myenv\Scripts\activate`
2、安装必要的库
在虚拟环境中安装所需的库:
pip install matplotlib numpy PyOpenGL PyOpenGL_accelerate
3、运行项目
在虚拟环境中编写和运行项目,确保所有依赖都在环境中安装。
五、进一步应用
1、交互式应用
可以结合GUI库如Tkinter或PyQt创建交互式应用,允许用户输入圆柱体的参数并实时显示结果。
2、数据分析和可视化
通过结合Pandas和Matplotlib,可以进行数据分析,并将计算结果以图形形式展示。
import pandas as pd
data = {
'Radius': [1, 2, 3, 4, 5],
'Height': [10, 20, 30, 40, 50]
}
df = pd.DataFrame(data)
df['Volume'] = df.apply(lambda row: cylinder_volume(row['Radius'], row['Height']), axis=1)
df['Surface Area'] = df.apply(lambda row: cylinder_surface_area(row['Radius'], row['Height']), axis=1)
print(df)
六、总结
用Python制作一个圆柱体涉及到多个方面,从基本的数学计算到三维图形的绘制,再到虚拟环境中的应用。通过使用Matplotlib和PyOpenGL,可以轻松实现圆柱体的可视化,并结合其他库进行进一步的分析和应用。希望本文能为你提供全面的指导,帮助你掌握如何用Python制作和应用圆柱体。
相关问答FAQs:
如何在Python中绘制圆柱体?
在Python中,您可以使用多个库来绘制圆柱体,例如Matplotlib和Mayavi。使用Matplotlib,您可以通过绘制多个圆形轮廓并连接它们来实现圆柱体的效果。而Mayavi则提供了更高级的三维图形功能,允许您直接创建和渲染三维物体。您可以查看相关的文档和示例代码,以便更好地理解绘制的过程。
在Python中创建圆柱体需要哪些库?
创建圆柱体的常用库包括Matplotlib、NumPy和Mayavi。Matplotlib用于简单的2D和3D绘图,NumPy帮助处理数学计算,Mayavi则适合复杂的3D可视化。根据您的需求选择合适的库,可以提高您的工作效率。
是否可以在Python中对圆柱体进行动画处理?
当然可以!在Python中,您可以使用Matplotlib的FuncAnimation模块或Mayavi的动画功能来为圆柱体添加动画效果。这可以通过更新圆柱体的参数或位置来实现,从而使其在图形窗口中动态显示。可以参考相关的教程,以获取具体的实现方法和示例代码。
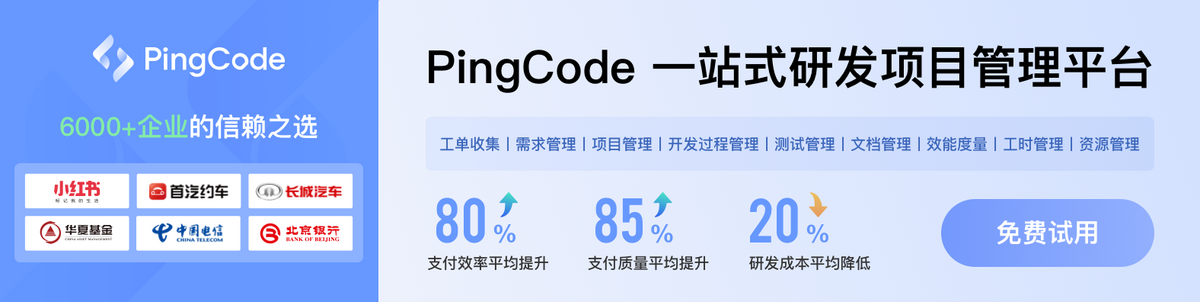