Python如何查看网络连接数据库
在Python中查看网络连接数据库,可以通过使用数据库驱动库、执行网络请求、解析返回的数据。其中,最常用的方法是使用数据库驱动库,如psycopg2
、pymysql
、cx_Oracle
等。这些库不仅可以帮助你连接到数据库,还能执行SQL查询,获取你所需的数据。使用数据库驱动库是最常用和高效的方法,因为它们专门设计用于处理数据库连接和查询。
一、使用数据库驱动库
1. psycopg2用于PostgreSQL
psycopg2
是Python中最流行的PostgreSQL数据库适配器。使用psycopg2
,你可以轻松地连接到PostgreSQL数据库,并执行SQL查询。以下是一个简单的例子:
import psycopg2
def connect_to_postgresql():
try:
connection = psycopg2.connect(
user="your_username",
password="your_password",
host="your_host",
port="your_port",
database="your_database"
)
cursor = connection.cursor()
cursor.execute("SELECT version();")
record = cursor.fetchone()
print("You are connected to - ", record,"\n")
except (Exception, psycopg2.Error) as error:
print("Error while connecting to PostgreSQL", error)
finally:
if connection:
cursor.close()
connection.close()
print("PostgreSQL connection is closed")
connect_to_postgresql()
2. pymysql用于MySQL
pymysql
是一个纯Python写的MySQL客户端库。以下是一个使用pymysql
连接到MySQL数据库并执行查询的例子:
import pymysql
def connect_to_mysql():
try:
connection = pymysql.connect(
host='your_host',
user='your_username',
password='your_password',
database='your_database'
)
cursor = connection.cursor()
cursor.execute("SELECT VERSION()")
data = cursor.fetchone()
print("Database version : %s " % data)
except pymysql.MySQLError as e:
print(f"Error while connecting to MySQL: {e}")
finally:
if connection:
connection.close()
print("MySQL connection is closed")
connect_to_mysql()
二、执行网络请求
1. 使用requests库
在某些情况下,你可能需要通过HTTP请求来访问数据库。例如,某些数据库提供了RESTful API,你可以使用requests
库来发送HTTP请求,并解析返回的数据。以下是一个简单的例子:
import requests
def fetch_data_from_api():
try:
response = requests.get('http://your_api_endpoint')
if response.status_code == 200:
data = response.json()
print(data)
else:
print(f"Failed to retrieve data: {response.status_code}")
except requests.RequestException as e:
print(f"Error occurred: {e}")
fetch_data_from_api()
三、解析返回的数据
1. 解析JSON数据
当你从API或数据库获取数据后,通常需要解析这些数据。对于JSON数据,可以使用Python的内置库json
。以下是一个简单的例子:
import json
def parse_json_data(json_string):
try:
data = json.loads(json_string)
print(data)
except json.JSONDecodeError as e:
print(f"Error decoding JSON: {e}")
json_string = '{"name": "John", "age": 30}'
parse_json_data(json_string)
四、错误处理和日志记录
1. 使用try-except进行错误处理
在处理数据库连接和网络请求时,错误处理非常重要。使用try-except
块可以捕获并处理异常,确保程序不会因为未处理的异常而崩溃。以下是一个例子:
import psycopg2
def connect_to_postgresql():
try:
connection = psycopg2.connect(
user="your_username",
password="your_password",
host="your_host",
port="your_port",
database="your_database"
)
cursor = connection.cursor()
cursor.execute("SELECT version();")
record = cursor.fetchone()
print("You are connected to - ", record,"\n")
except (Exception, psycopg2.Error) as error:
print("Error while connecting to PostgreSQL", error)
finally:
if connection:
cursor.close()
connection.close()
print("PostgreSQL connection is closed")
connect_to_postgresql()
2. 使用logging库进行日志记录
记录日志是了解程序运行状态和调试错误的重要手段。Python的logging
库提供了强大的日志记录功能。以下是一个简单的例子:
import logging
logging.basicConfig(level=logging.INFO, format='%(asctime)s - %(levelname)s - %(message)s')
def log_message():
logging.info('This is an info message')
logging.error('This is an error message')
log_message()
五、数据库连接池的使用
1. 使用SQLAlchemy连接池
在高并发的应用场景中,频繁的数据库连接和关闭会带来性能问题。使用数据库连接池可以有效地提高性能。SQLAlchemy
是一个功能强大的ORM库,提供了连接池的支持。以下是一个简单的例子:
from sqlalchemy import create_engine
def use_connection_pool():
engine = create_engine('postgresql+psycopg2://your_username:your_password@your_host/your_database', pool_size=10, max_overflow=20)
with engine.connect() as connection:
result = connection.execute("SELECT version();")
for row in result:
print(row)
use_connection_pool()
2. 使用DBUtils连接池
DBUtils
是另一个流行的数据库连接池库,支持多种数据库。以下是一个使用DBUtils
的例子:
from DBUtils.PooledDB import PooledDB
import pymysql
def use_dbutils_pool():
pool = PooledDB(pymysql, 5, host='your_host', user='your_username', passwd='your_password', db='your_database')
connection = pool.connection()
cursor = connection.cursor()
cursor.execute("SELECT VERSION()")
data = cursor.fetchone()
print("Database version : %s " % data)
connection.close()
use_dbutils_pool()
六、性能优化和安全性
1. 使用索引提高查询性能
在数据库中创建索引可以显著提高查询性能。以下是一个在PostgreSQL中创建索引的SQL语句:
CREATE INDEX idx_name ON your_table (your_column);
2. 防止SQL注入
防止SQL注入是确保数据库安全的重要措施。使用参数化查询可以有效防止SQL注入。以下是一个例子:
import pymysql
def safe_query():
connection = pymysql.connect(
host='your_host',
user='your_username',
password='your_password',
database='your_database'
)
cursor = connection.cursor()
user_input = 'some_input'
query = "SELECT * FROM your_table WHERE your_column = %s"
cursor.execute(query, (user_input,))
result = cursor.fetchall()
print(result)
connection.close()
safe_query()
七、综合实例
1. 综合使用数据库驱动库和连接池
以下是一个综合使用psycopg2
和DBUtils
连接池的例子:
from DBUtils.PooledDB import PooledDB
import psycopg2
def comprehensive_example():
pool = PooledDB(psycopg2, 5, user="your_username", password="your_password", host="your_host", port="your_port", database="your_database")
connection = pool.connection()
cursor = connection.cursor()
cursor.execute("SELECT version();")
record = cursor.fetchone()
print("You are connected to - ", record,"\n")
connection.close()
comprehensive_example()
通过以上内容,我们可以系统地了解如何在Python中查看网络连接数据库,使用数据库驱动库、执行网络请求、解析返回的数据,同时处理错误和记录日志,使用数据库连接池,以及优化性能和确保安全性。希望这些内容对你有所帮助。
相关问答FAQs:
如何使用Python查看当前网络连接的数据库?
要查看当前网络连接的数据库,可以使用Python的psycopg2
库(用于PostgreSQL)或pymysql
库(用于MySQL)。通过这些库,可以连接到数据库并执行查询,获取连接信息。首先,确保已经安装所需的库,并用正确的连接参数(如主机、端口、用户名、密码和数据库名称)进行连接。连接成功后,可以使用SQL查询获取连接的状态和相关信息。
在Python中如何处理数据库连接错误?
在连接数据库时可能会遇到各种错误,例如网络不可达、身份验证失败等。通过使用try-except
结构,可以捕获并处理这些异常。可以打印出错误信息,帮助定位问题。建议使用库的连接池功能来优化连接效率,并做好错误重试机制,以保证程序的稳定性和可靠性。
如何在Python中查看数据库连接的性能指标?
要查看数据库连接的性能指标,可以使用Python的sqlalchemy
库结合数据库的性能监控工具。通过编写合适的SQL查询,可以获取连接的运行时间、查询次数等信息。此外,许多数据库提供了内置的性能监控视图,利用这些视图可以更深入地分析数据库的运行状态和性能瓶颈。使用sqlalchemy
的ORM特性也可以简化性能数据的获取与分析。
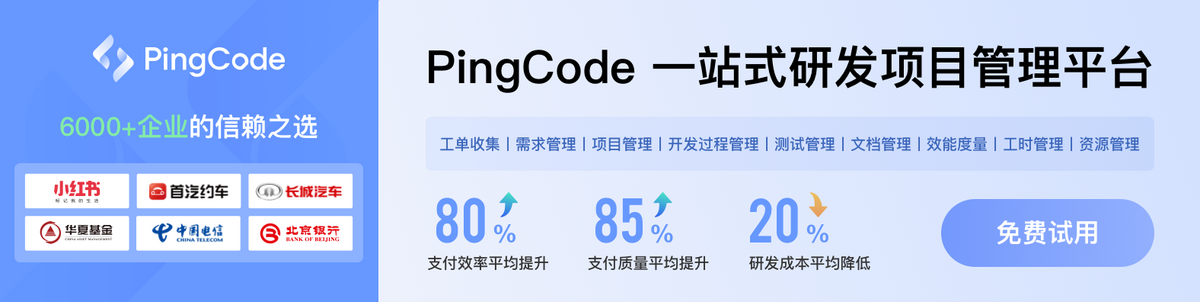