如何用Python做一个自动答题软件
Python是一种灵活且强大的编程语言,用它做一个自动答题软件涉及到题库的获取、答案的匹配、界面的设计、自动化操作等多个方面。核心步骤包括题库的获取与解析、答案匹配算法、界面设计与用户交互、自动化操作等。 在这篇博客文章中,我们将详细探讨如何用Python实现一个自动答题软件,从基础知识到实际操作,确保你能理解并实现这个项目。
一、题库的获取与解析
题库是自动答题软件的核心数据源,有几个常见的方式可以获取题库:
1、手动录入
手动录入题库是一种最直接但也最耗时的方法。你可以将题目和答案手动输入到一个文件中,如CSV、JSON、或数据库中。虽然这种方法效率较低,但它有利于确保数据的准确性。
2、网络爬虫
网络爬虫是一种自动化获取题库数据的方法。你可以编写一个爬虫程序,从各种在线题库网站上抓取题目和答案。这种方法效率高,但需要处理反爬虫机制,并且可能涉及版权问题。
例如,使用BeautifulSoup和requests库来抓取题库数据:
import requests
from bs4 import BeautifulSoup
url = 'https://example.com/quiz'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
questions = []
for item in soup.find_all('div', class_='question'):
question_text = item.find('p', class_='question-text').text
answer = item.find('p', class_='answer').text
questions.append({'question': question_text, 'answer': answer})
3、API接口
有些题库网站提供API接口,允许开发者直接获取题目和答案。使用API接口获取数据的优点在于它通常会提供结构化的数据格式,便于解析和使用。
例如,通过调用一个假设的题库API接口:
import requests
api_url = 'https://exampleapi.com/get_questions'
response = requests.get(api_url)
questions = response.json()
for question in questions:
print(f"Question: {question['question']}, Answer: {question['answer']}")
二、答案匹配算法
有了题库之后,接下来就是实现答案匹配算法。常见的方法有直接匹配和模糊匹配。
1、直接匹配
直接匹配是一种简单而有效的方法,尤其适用于题目和答案都是标准格式的情况。你可以将用户输入的题目与题库中的题目逐一比对,找到匹配的题目,然后返回对应的答案。
def find_answer(question, questions):
for item in questions:
if item['question'] == question:
return item['answer']
return "No matching answer found."
2、模糊匹配
模糊匹配适用于题目不完全一致的情况。你可以使用一些字符串匹配算法,如Levenshtein距离、Jaccard相似度等,来计算题目之间的相似度,从而找到最接近的匹配。
from difflib import get_close_matches
def find_fuzzy_answer(question, questions):
question_texts = [item['question'] for item in questions]
matches = get_close_matches(question, question_texts)
if matches:
for item in questions:
if item['question'] == matches[0]:
return item['answer']
return "No matching answer found."
三、界面设计与用户交互
一个好的自动答题软件需要一个友好的用户界面。你可以使用一些GUI库来设计界面,如Tkinter、PyQt、或Kivy。
1、使用Tkinter设计界面
Tkinter是Python的标准GUI库,适合快速开发简单的桌面应用程序。
import tkinter as tk
class QuizApp:
def __init__(self, master, questions):
self.master = master
self.questions = questions
self.master.title("Quiz App")
self.question_label = tk.Label(master, text="Enter your question:")
self.question_label.pack()
self.question_entry = tk.Entry(master)
self.question_entry.pack()
self.answer_button = tk.Button(master, text="Get Answer", command=self.get_answer)
self.answer_button.pack()
self.answer_label = tk.Label(master, text="")
self.answer_label.pack()
def get_answer(self):
question = self.question_entry.get()
answer = find_answer(question, self.questions)
self.answer_label.config(text=answer)
questions = [
{'question': 'What is the capital of France?', 'answer': 'Paris'},
{'question': 'What is 2 + 2?', 'answer': '4'}
]
root = tk.Tk()
app = QuizApp(root, questions)
root.mainloop()
2、使用PyQt设计界面
PyQt是一个更强大的GUI库,适合开发复杂的桌面应用程序。
import sys
from PyQt5.QtWidgets import QApplication, QWidget, QLabel, QLineEdit, QPushButton, QVBoxLayout
class QuizApp(QWidget):
def __init__(self, questions):
super().__init__()
self.questions = questions
self.initUI()
def initUI(self):
self.setWindowTitle('Quiz App')
self.layout = QVBoxLayout()
self.question_label = QLabel('Enter your question:')
self.layout.addWidget(self.question_label)
self.question_entry = QLineEdit(self)
self.layout.addWidget(self.question_entry)
self.answer_button = QPushButton('Get Answer', self)
self.answer_button.clicked.connect(self.get_answer)
self.layout.addWidget(self.answer_button)
self.answer_label = QLabel('')
self.layout.addWidget(self.answer_label)
self.setLayout(self.layout)
self.show()
def get_answer(self):
question = self.question_entry.text()
answer = find_answer(question, self.questions)
self.answer_label.setText(answer)
questions = [
{'question': 'What is the capital of France?', 'answer': 'Paris'},
{'question': 'What is 2 + 2?', 'answer': '4'}
]
app = QApplication(sys.argv)
quiz_app = QuizApp(questions)
sys.exit(app.exec_())
四、自动化操作
自动化操作是指通过编程实现一些自动化的任务,如自动提交答案、自动点击按钮等。你可以使用Selenium或PyAutoGUI等库来实现这些功能。
1、使用Selenium实现自动化
Selenium是一个强大的浏览器自动化工具,适合用于自动化Web应用程序的操作。
from selenium import webdriver
from selenium.webdriver.common.keys import Keys
def automate_quiz(questions):
driver = webdriver.Chrome()
driver.get('https://example.com/quiz')
for item in questions:
question_field = driver.find_element_by_name('question')
question_field.send_keys(item['question'])
question_field.send_keys(Keys.RETURN)
answer_field = driver.find_element_by_name('answer')
answer = find_answer(item['question'], questions)
answer_field.send_keys(answer)
answer_field.send_keys(Keys.RETURN)
driver.quit()
questions = [
{'question': 'What is the capital of France?', 'answer': 'Paris'},
{'question': 'What is 2 + 2?', 'answer': '4'}
]
automate_quiz(questions)
2、使用PyAutoGUI实现自动化
PyAutoGUI是一个跨平台的GUI自动化工具,适合用于桌面应用程序的自动化操作。
import pyautogui
def automate_quiz(questions):
for item in questions:
pyautogui.write(item['question'])
pyautogui.press('enter')
answer = find_answer(item['question'], questions)
pyautogui.write(answer)
pyautogui.press('enter')
questions = [
{'question': 'What is the capital of France?', 'answer': 'Paris'},
{'question': 'What is 2 + 2?', 'answer': '4'}
]
automate_quiz(questions)
五、总结与优化
在实现了基本功能之后,你可以考虑如何优化和扩展你的自动答题软件。
1、优化性能
如果题库非常大,直接匹配可能会导致性能问题。你可以使用一些数据结构和算法来优化性能,如哈希表、二分查找等。
def find_answer_optimized(question, question_dict):
return question_dict.get(question, "No matching answer found.")
questions = [
{'question': 'What is the capital of France?', 'answer': 'Paris'},
{'question': 'What is 2 + 2?', 'answer': '4'}
]
question_dict = {item['question']: item['answer'] for item in questions}
2、扩展功能
你可以为你的自动答题软件添加更多的功能,如支持多种题型(选择题、填空题等)、支持多种语言、提供题目解析和解释等。
def get_answer_with_explanation(question, questions):
for item in questions:
if item['question'] == question:
return item['answer'], item.get('explanation', 'No explanation available.')
return "No matching answer found.", ""
questions = [
{'question': 'What is the capital of France?', 'answer': 'Paris', 'explanation': 'Paris is the capital city of France.'},
{'question': 'What is 2 + 2?', 'answer': '4', 'explanation': '2 + 2 equals 4.'}
]
question = 'What is the capital of France?'
answer, explanation = get_answer_with_explanation(question, questions)
print(f"Answer: {answer}, Explanation: {explanation}")
通过以上步骤,你可以用Python实现一个功能强大的自动答题软件。希望这篇文章能为你提供一些有用的思路和参考,帮助你顺利完成这个项目。
相关问答FAQs:
如何用Python实现自动答题软件的基本步骤是什么?
要实现一个自动答题软件,首先需要明确软件的功能需求,包括题库的来源、答案的获取方式以及用户界面的设计。接着,可以使用Python的爬虫库(如BeautifulSoup或Scrapy)抓取题库,利用自然语言处理库(如NLTK或spaCy)进行题目解析,并使用机器学习算法训练模型来预测答案。最后,使用Tkinter或Flask等库来创建用户界面,使用户能够方便地进行答题。
自动答题软件需要处理哪些类型的题目?
自动答题软件可以处理多种类型的题目,包括选择题、填空题、问答题等。针对选择题,软件需要识别题目及选项,并通过预先建立的答案库进行匹配;填空题则需要识别缺失的部分并生成合适的答案;问答题则可以通过搜索引擎或知识库进行信息获取和答案生成。
如何提高自动答题软件的准确性?
提高自动答题软件的准确性需要多个方面的努力。首先,确保题库的质量和数量,以便软件能够获取更全面的信息。其次,选用合适的算法和模型进行训练,以提高对题目的理解和解析能力。此外,定期更新软件,优化算法,增加用户反馈机制,也有助于不断提升准确性和用户体验。
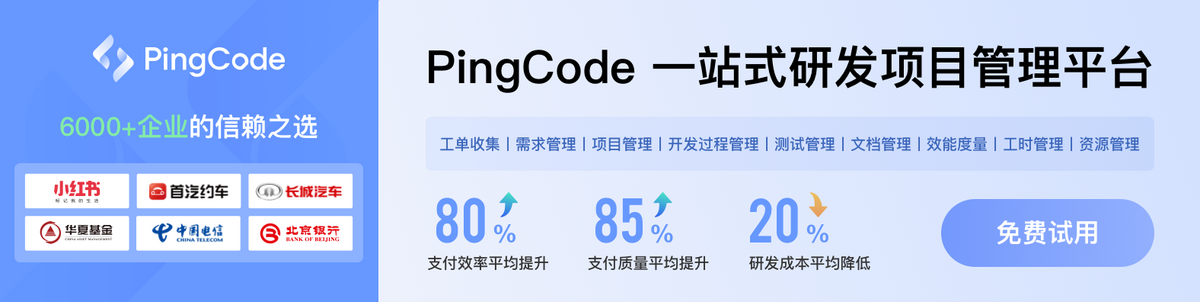