如何在Python中制作聊天机器人
在Python中制作聊天机器人可以通过以下几种方式来实现:使用自然语言处理库(如NLTK、spaCy)、利用机器学习模型(如TensorFlow、PyTorch)、使用现成的聊天机器人框架(如ChatterBot)、集成第三方API(如Dialogflow)。选择合适的工具、设计对话逻辑、训练模型、持续优化是制作高效聊天机器人的关键。下面将详细介绍其中的“设计对话逻辑”,因为这是构建用户体验的核心部分。
设计对话逻辑是制作聊天机器人的重要步骤之一,它决定了机器人如何与用户互动。一个好的对话逻辑需要考虑用户的各种可能输入,并设计相应的响应策略。可以通过状态机、规则引擎或深度学习模型来实现复杂的对话逻辑。
一、选择合适的工具
1、自然语言处理库
自然语言处理(NLP)是聊天机器人理解用户输入的关键。Python有许多强大的NLP库,如NLTK和spaCy。NLTK是一个强大的库,适合学习和研究,而spaCy则更适合在生产环境中使用,因为它速度更快且更易于使用。
NLTK
NLTK提供了很多工具和资源,如词汇资源、分类器、解析器等。它适合用来处理文本数据、标注词性、提取命名实体等。
import nltk
from nltk.tokenize import word_tokenize
nltk.download('punkt')
sentence = "Hello, how can I help you today?"
tokens = word_tokenize(sentence)
print(tokens)
spaCy
spaCy是一个工业级的NLP库,拥有高性能和易用的API。它包含预训练的模型,可以用于命名实体识别、词性标注、依存解析等任务。
import spacy
nlp = spacy.load("en_core_web_sm")
doc = nlp("Hello, how can I help you today?")
for token in doc:
print(token.text, token.pos_, token.dep_)
2、机器学习模型
机器学习模型可以用来训练聊天机器人,使其能够理解和生成自然语言。TensorFlow和PyTorch是两个流行的深度学习框架,可以用来训练复杂的对话模型。
TensorFlow
TensorFlow是一个由Google开发的开源深度学习框架,适用于大规模机器学习模型的训练和部署。
import tensorflow as tf
Define a simple neural network model
model = tf.keras.Sequential([
tf.keras.layers.Dense(128, activation='relu', input_shape=(input_dim,)),
tf.keras.layers.Dense(128, activation='relu'),
tf.keras.layers.Dense(output_dim, activation='softmax')
])
model.compile(optimizer='adam', loss='sparse_categorical_crossentropy', metrics=['accuracy'])
model.summary()
PyTorch
PyTorch是一个由Facebook AI Research团队开发的开源深度学习框架,具有灵活性和动态计算图的特点。
import torch
import torch.nn as nn
import torch.optim as optim
Define a simple neural network model
class SimpleNN(nn.Module):
def __init__(self, input_dim, output_dim):
super(SimpleNN, self).__init__()
self.fc1 = nn.Linear(input_dim, 128)
self.fc2 = nn.Linear(128, 128)
self.fc3 = nn.Linear(128, output_dim)
def forward(self, x):
x = torch.relu(self.fc1(x))
x = torch.relu(self.fc2(x))
x = torch.softmax(self.fc3(x), dim=1)
return x
model = SimpleNN(input_dim, output_dim)
criterion = nn.CrossEntropyLoss()
optimizer = optim.Adam(model.parameters(), lr=0.001)
3、聊天机器人框架
使用现成的聊天机器人框架可以大大简化开发过程。ChatterBot是一个基于机器学习的Python库,适合快速构建和训练聊天机器人。
ChatterBot
ChatterBot提供了简单易用的API,可以快速创建和训练聊天机器人。它支持多种语言和输入格式。
from chatterbot import ChatBot
from chatterbot.trainers import ListTrainer
Create a new instance of a ChatBot
chatbot = ChatBot('Example Bot')
Train the chatbot with a list of conversations
trainer = ListTrainer(chatbot)
trainer.train([
"Hi, how can I help you?",
"I need some assistance.",
"Sure, what do you need help with?"
])
Get a response for a given input
response = chatbot.get_response("I need some assistance.")
print(response)
4、第三方API
集成第三方API如Dialogflow,可以利用其强大的自然语言理解能力,快速构建高质量的聊天机器人。
Dialogflow
Dialogflow是一个由Google提供的对话平台,支持多种语言和渠道。它可以识别用户意图、管理对话上下文,并生成自然语言响应。
import dialogflow_v2 as dialogflow
Initialize the Dialogflow session
session_client = dialogflow.SessionsClient()
session = session_client.session_path('your-project-id', 'your-session-id')
Send a text query to Dialogflow
text_input = dialogflow.types.TextInput(text="Hello", language_code="en")
query_input = dialogflow.types.QueryInput(text=text_input)
response = session_client.detect_intent(session=session, query_input=query_input)
print(response.query_result.fulfillment_text)
二、设计对话逻辑
1、状态机
状态机是一种简单但功能强大的工具,用于管理聊天机器人的对话状态。通过定义状态和状态之间的转移,可以设计出复杂的对话逻辑。
定义状态和转移
class StateMachine:
def __init__(self):
self.state = 'INIT'
def transition(self, user_input):
if self.state == 'INIT':
if user_input == 'Hi':
self.state = 'GREET'
return "Hello! How can I help you?"
else:
return "I don't understand. Please say 'Hi'."
elif self.state == 'GREET':
if user_input == 'I need help':
self.state = 'ASSIST'
return "Sure, what do you need help with?"
else:
return "I don't understand. Please say 'I need help'."
state_machine = StateMachine()
print(state_machine.transition('Hi'))
print(state_machine.transition('I need help'))
2、规则引擎
规则引擎是一种基于规则的系统,可以根据用户输入和预定义的规则生成响应。它适用于对话逻辑较为固定的场景。
定义规则和响应
class RuleEngine:
def __init__(self):
self.rules = {
'Hi': 'Hello! How can I help you?',
'I need help': 'Sure, what do you need help with?'
}
def get_response(self, user_input):
return self.rules.get(user_input, "I don't understand.")
rule_engine = RuleEngine()
print(rule_engine.get_response('Hi'))
print(rule_engine.get_response('I need help'))
3、深度学习模型
深度学习模型可以自动学习对话逻辑,从而生成更加自然的对话。常用的方法有序列到序列(Seq2Seq)模型、变分自编码器(VAE)等。
Seq2Seq模型
Seq2Seq模型是一种常用于机器翻译和对话生成的模型,由编码器和解码器组成。编码器将输入序列编码成固定长度的向量,解码器将其解码成输出序列。
import tensorflow as tf
from tensorflow.keras.layers import Embedding, LSTM, Dense
from tensorflow.keras.models import Model
Define the Seq2Seq model
class Seq2Seq(Model):
def __init__(self, input_vocab_size, output_vocab_size, embedding_dim, units):
super(Seq2Seq, self).__init__()
self.encoder = LSTM(units, return_sequences=True, return_state=True)
self.decoder = LSTM(units, return_sequences=True, return_state=True)
self.dense = Dense(output_vocab_size)
def call(self, encoder_input, decoder_input):
encoder_output, state_h, state_c = self.encoder(encoder_input)
decoder_output, _, _ = self.decoder(decoder_input, initial_state=[state_h, state_c])
output = self.dense(decoder_output)
return output
Create and compile the model
input_vocab_size = 10000
output_vocab_size = 10000
embedding_dim = 256
units = 512
model = Seq2Seq(input_vocab_size, output_vocab_size, embedding_dim, units)
model.compile(optimizer='adam', loss='sparse_categorical_crossentropy', metrics=['accuracy'])
model.summary()
三、训练模型
1、数据准备
训练聊天机器人需要大量的对话数据。可以使用公开的对话数据集,如Cornell Movie-Dialogs Corpus、Twitter数据集等。
加载和预处理数据
import pandas as pd
Load the dataset
data = pd.read_csv('path/to/dataset.csv')
questions = data['question'].tolist()
answers = data['answer'].tolist()
Tokenize and pad sequences
from tensorflow.keras.preprocessing.text import Tokenizer
from tensorflow.keras.preprocessing.sequence import pad_sequences
tokenizer = Tokenizer(num_words=10000)
tokenizer.fit_on_texts(questions + answers)
question_sequences = tokenizer.texts_to_sequences(questions)
answer_sequences = tokenizer.texts_to_sequences(answers)
max_length = max(len(seq) for seq in question_sequences + answer_sequences)
question_sequences = pad_sequences(question_sequences, maxlen=max_length, padding='post')
answer_sequences = pad_sequences(answer_sequences, maxlen=max_length, padding='post')
2、训练过程
训练过程包括前向传播、计算损失、反向传播和更新权重。可以使用TensorFlow或PyTorch来实现训练过程。
TensorFlow训练过程
# Define the training loop
epochs = 10
batch_size = 64
for epoch in range(epochs):
for i in range(0, len(question_sequences), batch_size):
batch_questions = question_sequences[i:i+batch_size]
batch_answers = answer_sequences[i:i+batch_size]
# Train the model on the batch
model.train_on_batch(batch_questions, batch_answers)
# Evaluate the model on the validation set
val_loss, val_accuracy = model.evaluate(val_questions, val_answers)
print(f'Epoch {epoch+1}, Loss: {val_loss}, Accuracy: {val_accuracy}')
PyTorch训练过程
# Define the training loop
epochs = 10
batch_size = 64
for epoch in range(epochs):
for i in range(0, len(question_sequences), batch_size):
batch_questions = torch.tensor(question_sequences[i:i+batch_size], dtype=torch.long)
batch_answers = torch.tensor(answer_sequences[i:i+batch_size], dtype=torch.long)
# Zero the gradients
optimizer.zero_grad()
# Forward pass
outputs = model(batch_questions, batch_answers)
# Compute the loss
loss = criterion(outputs.view(-1, output_vocab_size), batch_answers.view(-1))
# Backward pass and optimize
loss.backward()
optimizer.step()
# Evaluate the model on the validation set
with torch.no_grad():
val_questions = torch.tensor(val_questions, dtype=torch.long)
val_answers = torch.tensor(val_answers, dtype=torch.long)
val_outputs = model(val_questions, val_answers)
val_loss = criterion(val_outputs.view(-1, output_vocab_size), val_answers.view(-1))
print(f'Epoch {epoch+1}, Loss: {val_loss.item()}')
四、持续优化
1、性能评估
评估聊天机器人的性能是一个持续的过程。可以使用准确率、精确率、召回率、F1分数等指标来衡量模型的性能。
定义评估指标
from sklearn.metrics import accuracy_score, precision_score, recall_score, f1_score
Compute the predicted answers
predicted_answers = model.predict(test_questions)
Compute the evaluation metrics
accuracy = accuracy_score(test_answers, predicted_answers)
precision = precision_score(test_answers, predicted_answers, average='weighted')
recall = recall_score(test_answers, predicted_answers, average='weighted')
f1 = f1_score(test_answers, predicted_answers, average='weighted')
print(f'Accuracy: {accuracy}')
print(f'Precision: {precision}')
print(f'Recall: {recall}')
print(f'F1 Score: {f1}')
2、用户反馈
用户反馈是优化聊天机器人的重要依据。可以通过用户评价、会话日志分析等方式收集反馈,并根据反馈调整对话逻辑和模型参数。
收集和分析用户反馈
# Simulated user feedback data
user_feedback = [
{'input': 'Hi', 'expected_response': 'Hello! How can I help you?', 'actual_response': 'Hello!', 'satisfaction': 4},
{'input': 'I need help', 'expected_response': 'Sure, what do you need help with?', 'actual_response': 'What do you need help with?', 'satisfaction': 5}
]
Analyze feedback
for feedback in user_feedback:
if feedback['expected_response'] != feedback['actual_response']:
print(f"Mismatch for input '{feedback['input']}': expected '{feedback['expected_response']}', got '{feedback['actual_response']}'")
结论
制作一个高效的聊天机器人需要选择合适的工具、设计合理的对话逻辑、训练和优化模型,并持续收集和分析用户反馈。通过不断迭代和优化,可以构建出一个能够理解和生成自然语言的智能聊天机器人。
相关问答FAQs:
如何开始学习Python以制作聊天机器人?
要制作聊天机器人,首先需要掌握Python的基础知识。可以通过在线课程、书籍或教程来学习Python编程语言。建议从基本语法、数据结构和函数开始,逐步深入到更复杂的主题,如面向对象编程和API交互。此外,了解一些常用的Python库,如NLTK或spaCy,可以帮助你处理自然语言。
制作聊天机器人需要哪些工具和库?
在Python中,有多个库可以帮助你创建聊天机器人。常用的库包括ChatterBot、NLTK、TensorFlow和Flask。ChatterBot是一个简单易用的库,可以快速构建对话系统;NLTK提供了强大的自然语言处理功能;TensorFlow适合需要更复杂的机器学习模型的聊天机器人;Flask则可以帮助你将聊天机器人部署为Web应用程序。
聊天机器人可以实现哪些功能?
聊天机器人的功能可以非常多样化。它们可以用于客户服务,提供24/7的支持,回答常见问题;也可以用于娱乐,进行有趣的对话或游戏。此外,聊天机器人还可以集成到社交媒体平台,通过自动化消息回复来提高用户互动。根据需求的不同,聊天机器人可以根据预设规则或机器学习算法生成响应,提供个性化体验。
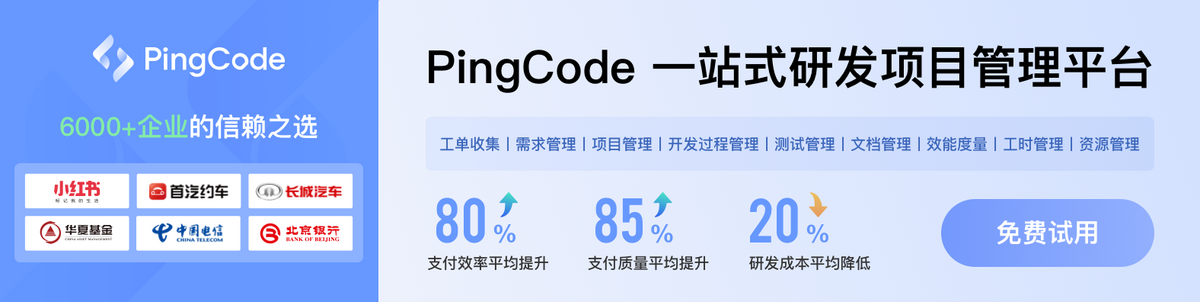