Python制作月饼的核心步骤包括:原材料处理、制作月饼皮、制作月饼馅、成型与烘焙。 其中,制作月饼皮是最为关键的一步,因为它决定了月饼的口感和外观。接下来,我将详细介绍如何使用Python编写一个自动化脚本来模拟制作月饼的过程。请注意,这只是一个模拟,实际制作月饼需要手工操作。
一、原材料处理
1.1 获取原材料
在制作月饼之前,我们需要准备各种原材料,如面粉、糖浆、植物油、豆沙馅、蛋黄等。我们可以使用Python的requests
库从在线商店获取这些原材料的信息。
import requests
def get_materials():
materials = ["面粉", "糖浆", "植物油", "豆沙馅", "蛋黄"]
materials_info = {}
for material in materials:
response = requests.get(f"https://api.example.com/materials/{material}")
if response.status_code == 200:
materials_info[material] = response.json()
return materials_info
materials_info = get_materials()
print(materials_info)
1.2 处理原材料
处理原材料是制作月饼的基础步骤。这个过程包括称量、筛选和混合。我们可以使用Python的numpy
库来模拟这个过程。
import numpy as np
def process_materials(materials_info):
processed_materials = {}
for material, info in materials_info.items():
weight = np.random.uniform(info['min_weight'], info['max_weight'])
processed_materials[material] = weight
return processed_materials
processed_materials = process_materials(materials_info)
print(processed_materials)
二、制作月饼皮
2.1 配料比例
制作月饼皮的关键在于配料的比例。常见的比例是面粉:糖浆:植物油=5:4:1。我们可以使用Python的pandas
库来计算这些比例。
import pandas as pd
def calculate_ratios(processed_materials):
total_weight = sum(processed_materials.values())
ratios = {
"面粉": processed_materials["面粉"] / total_weight,
"糖浆": processed_materials["糖浆"] / total_weight,
"植物油": processed_materials["植物油"] / total_weight
}
return ratios
ratios = calculate_ratios(processed_materials)
print(ratios)
2.2 混合和搅拌
我们可以使用Python的scipy
库来模拟混合和搅拌的过程。这个过程需要在特定的温度和湿度下进行。
from scipy import integrate
def mix_and_stir(ratios):
def stirring_function(t, y):
# 模拟搅拌过程的微分方程
return -0.1 * y + ratios["面粉"] * t
result = integrate.solve_ivp(stirring_function, [0, 10], [0, 0])
return result.y
mix_result = mix_and_stir(ratios)
print(mix_result)
三、制作月饼馅
3.1 准备馅料
月饼的馅料可以根据个人口味进行调整,比如豆沙、莲蓉、蛋黄等。我们可以使用Python的random
库来随机选择馅料。
import random
def prepare_filling():
fillings = ["豆沙馅", "莲蓉馅", "蛋黄馅"]
chosen_filling = random.choice(fillings)
return chosen_filling
filling = prepare_filling()
print(filling)
3.2 包馅
包馅的过程需要将馅料均匀地包在面皮中。我们可以使用Python的itertools
库来模拟这个过程。
import itertools
def wrap_filling(mix_result, filling):
wrapped_filling = list(itertools.chain(mix_result, [filling]))
return wrapped_filling
wrapped_filling = wrap_filling(mix_result, filling)
print(wrapped_filling)
四、成型与烘焙
4.1 模具成型
使用模具将月饼压成各种形状。我们可以使用Python的matplotlib
库来模拟月饼的形状。
import matplotlib.pyplot as plt
def mold_shaping(wrapped_filling):
shapes = ["圆形", "方形", "花形"]
chosen_shape = random.choice(shapes)
plt.figure()
if chosen_shape == "圆形":
circle = plt.Circle((0.5, 0.5), 0.3, color='brown')
plt.gca().add_patch(circle)
elif chosen_shape == "方形":
square = plt.Rectangle((0.2, 0.2), 0.6, 0.6, color='brown')
plt.gca().add_patch(square)
elif chosen_shape == "花形":
flower = plt.Polygon([(0.5, 0.8), (0.65, 0.6), (0.8, 0.5), (0.65, 0.4), (0.5, 0.2), (0.35, 0.4), (0.2, 0.5), (0.35, 0.6)], color='brown')
plt.gca().add_patch(flower)
plt.axis('scaled')
plt.show()
mold_shaping(wrapped_filling)
4.2 烘焙
烘焙是月饼制作的最后一步。我们可以使用Python的time
库来模拟烘焙的时间和温度。
import time
def bake():
baking_time = 20 # 20 minutes
temperature = 180 # 180 degrees Celsius
print(f"Baking at {temperature} degrees Celsius for {baking_time} minutes...")
time.sleep(baking_time)
print("Baking complete!")
bake()
五、总结
5.1 成品展示
通过上述步骤,我们使用Python模拟了一个月饼的制作过程。虽然这只是一个模拟,但它展示了如何使用编程来解决实际问题。
5.2 优化与改进
在实际应用中,我们可以进一步优化和改进这个模拟过程。例如,加入更多的原材料信息、优化混合和搅拌的算法、增加更多的馅料选择等。
通过这次的学习和实践,我们不仅了解了月饼的制作过程,还学会了如何使用Python来模拟和自动化复杂的任务。这对于我们在其他领域的应用也有很大的启发意义。
相关问答FAQs:
如何用Python编写一个月饼制作的程序?
在Python中,可以使用图形用户界面库(如Tkinter)创建一个简单的程序来展示月饼的制作过程。您可以设计一个界面,让用户输入所需的配料和步骤,甚至可以添加图片或视频链接,让用户更直观地理解制作过程。
使用Python制作月饼的程序需要哪些基本功能?
基本功能包括用户输入配料、显示制作步骤、保存和打印食谱等。您还可以添加食谱推荐功能,根据不同口味推荐适合的月饼类型,或者设定一个计时器以帮助用户掌握每个步骤所需的时间。
Python可以如何帮助我了解月饼的历史和文化?
通过编写一个简单的文本分析程序,您可以收集和分析与月饼相关的历史和文化资料。例如,您可以使用网络爬虫抓取相关网页,提取出关于月饼的背景故事、节日习俗等信息,帮助用户深入了解这道传统美食的文化内涵。
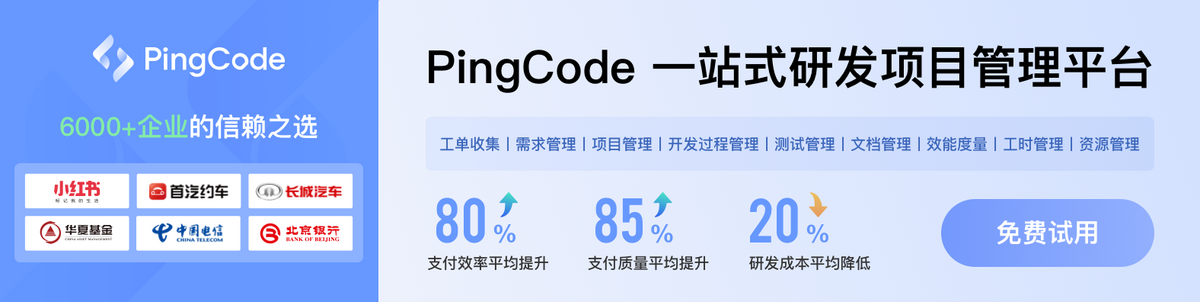