Python2中的if语句如何使用:
Python2中的if语句用于条件判断,语法简单、可嵌套使用、支持多条件判断。例如,基本的if语句结构如下:
if condition:
# code to execute if condition is true
elif another_condition:
# code to execute if another_condition is true
else:
# code to execute if no condition is true
语法简单:Python的if语句使用一个冒号(:)和缩进来表示代码块,语法非常直观明了。接下来,我们将详细介绍Python2中的if语句的使用方法和注意事项。
一、基本语法和结构
Python的if语句主要由三个部分组成:if、elif(可选)和else(可选)。每个部分后面都跟着一个条件表达式和一个代码块。
1、if语句的基本结构
if condition:
# 当条件为真时执行的代码块
2、if-else语句的结构
if condition:
# 当条件为真时执行的代码块
else:
# 当条件为假时执行的代码块
3、if-elif-else语句的结构
if condition1:
# 当condition1为真时执行的代码块
elif condition2:
# 当condition2为真时执行的代码块
else:
# 当所有条件都为假时执行的代码块
二、条件表达式
在if语句中,条件表达式通常是一个布尔表达式,它可以是任何返回布尔值的表达式,包括比较运算、逻辑运算等。
1、比较运算符
Python中的比较运算符包括:
- 等于:
==
- 不等于:
!=
- 大于:
>
- 小于:
<
- 大于等于:
>=
- 小于等于:
<=
例如:
a = 10
b = 20
if a < b:
print("a 小于 b")
2、逻辑运算符
Python中的逻辑运算符包括:
- 与:
and
- 或:
or
- 非:
not
例如:
a = 10
b = 20
if a < b and a > 5:
print("a 小于 b 并且 a 大于 5")
三、嵌套if语句
在实际编程中,我们经常需要在一个if语句内部再嵌套另一个if语句,以处理更复杂的条件判断。
例如:
a = 10
b = 20
c = 30
if a < b:
if b < c:
print("a 小于 b 并且 b 小于 c")
else:
print("a 小于 b 但是 b 不小于 c")
else:
print("a 不小于 b")
四、使用逻辑运算符组合条件
有时,我们需要同时检查多个条件,在这种情况下,可以使用逻辑运算符and
和or
来组合条件。
例如:
a = 10
b = 20
c = 30
if a < b and b < c:
print("a 小于 b 并且 b 小于 c")
五、if语句中的常见错误和注意事项
1、缩进问题
Python使用缩进来表示代码块的层级关系,因此缩进不正确会导致语法错误或逻辑错误。
a = 10
b = 20
if a < b:
print("a 小于 b") # 缩进错误
2、条件表达式的类型
条件表达式可以是任何返回布尔值的表达式,但要注意类型的匹配和转换。
a = "10"
b = 20
if int(a) < b:
print("a 小于 b")
3、避免过多的嵌套
虽然嵌套if语句可以处理复杂的条件判断,但过多的嵌套会降低代码的可读性和可维护性。应尽量简化条件判断,或将复杂的条件判断提取为函数。
六、if语句的高级用法
1、使用三元运算符
Python支持三元运算符,可以简化简单的条件判断。
a = 10
b = 20
result = "a 小于 b" if a < b else "a 不小于 b"
print(result)
2、使用列表或字典映射
在某些情况下,可以使用列表或字典来简化条件判断。
例如:
status_code = 200
messages = {
200: "OK",
404: "Not Found",
500: "Internal Server Error"
}
print(messages.get(status_code, "Unknown Status Code"))
3、使用函数和类
对于复杂的条件判断,可以将其提取为函数或类,以提高代码的可读性和可维护性。
例如:
def check_conditions(a, b, c):
if a < b and b < c:
return "a 小于 b 并且 b 小于 c"
else:
return "条件不满足"
a = 10
b = 20
c = 30
print(check_conditions(a, b, c))
七、实际应用示例
1、用户登录系统
def authenticate(username, password):
# 假设我们有一个用户数据库
user_db = {
"user1": "password1",
"user2": "password2"
}
if username in user_db:
if user_db[username] == password:
return "登录成功"
else:
return "密码错误"
else:
return "用户不存在"
username = "user1"
password = "password1"
print(authenticate(username, password))
2、计算成绩等级
def get_grade(score):
if score >= 90:
return "A"
elif score >= 80:
return "B"
elif score >= 70:
return "C"
elif score >= 60:
return "D"
else:
return "F"
score = 85
print("成绩等级:", get_grade(score))
八、总结
Python2中的if语句是条件判断的基础工具,语法简单、可嵌套使用、支持多条件判断。通过合理使用if语句,我们可以实现各种复杂的逻辑控制。在实际编程中,应注意缩进、条件表达式的类型匹配,避免过多嵌套,以提高代码的可读性和可维护性。
希望本文能帮助你更好地理解和使用Python2中的if语句。如果你有任何问题或建议,欢迎在下方留言。
相关问答FAQs:
如何在Python 2中编写条件语句?
在Python 2中,if语句的基本结构与Python 3类似。你可以使用if
、elif
和else
来控制代码的执行流。例如:
x = 10
if x > 5:
print "x is greater than 5"
elif x == 5:
print "x is equal to 5"
else:
print "x is less than 5"
注意,Python 2使用的是传统的print语句,不需要括号。
在Python 2中,如何使用逻辑运算符与if语句结合?
在Python 2中,可以使用and
、or
和not
等逻辑运算符来结合多个条件。例如:
x = 10
y = 5
if x > 5 and y < 10:
print "Both conditions are true"
这种方式使得你可以对多个条件进行组合判断,从而实现更复杂的逻辑。
如何在Python 2中处理用户输入并使用if语句?
可以使用raw_input()
函数从用户获取输入,并结合if语句进行判断。示例代码如下:
user_input = raw_input("Please enter a number: ")
if user_input.isdigit():
number = int(user_input)
if number > 0:
print "You entered a positive number."
else:
print "You entered a non-positive number."
else:
print "That's not a valid number."
这种方式允许你根据用户输入的值执行不同的逻辑。
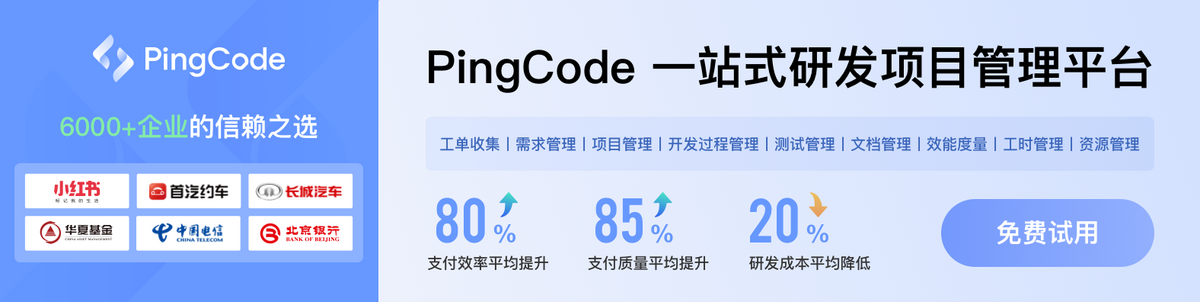