在Python中实现红绿灯显示的关键技术点包括:图形界面库的选择、颜色的设置、定时器的使用、循环逻辑的控制。本文将详细讲解如何使用Python在电子屏上实现红绿灯的显示。
一、引言
在现代交通系统中,红绿灯的作用不可忽视。使用Python编写模拟红绿灯的程序,可以帮助初学者理解基本的编程概念,如图形界面编程、定时器和事件处理等。本文将逐步介绍如何使用Python实现这一功能。
二、选择图形界面库
Python有许多图形界面库可供选择,其中最常用的是Tkinter。Tkinter是Python的标准GUI库,易于使用,适合初学者。其他选择还包括Pygame、PyQt等,但为了简单起见,我们将使用Tkinter。
1、安装Tkinter
Tkinter通常随Python安装包一同安装。如果没有安装,可以使用以下命令进行安装:
pip install tk
2、创建基本窗口
首先,我们需要创建一个基本窗口来显示红绿灯。
import tkinter as tk
def create_window():
window = tk.Tk()
window.title("Traffic Light Simulation")
window.geometry("200x500")
return window
window = create_window()
window.mainloop()
三、绘制红绿灯
在Tkinter中,可以使用Canvas组件来绘制图形。我们将使用Canvas组件来绘制红绿灯。
1、创建Canvas组件
canvas = tk.Canvas(window, width=200, height=500, bg='white')
canvas.pack()
2、绘制圆形表示红绿灯
我们可以使用Canvas的create_oval
方法来绘制红绿灯的红、黄、绿三种颜色。
# Red light
red_light = canvas.create_oval(50, 50, 150, 150, fill='grey')
Yellow light
yellow_light = canvas.create_oval(50, 200, 150, 300, fill='grey')
Green light
green_light = canvas.create_oval(50, 350, 150, 450, fill='grey')
四、实现红绿灯的切换逻辑
红绿灯的切换逻辑是整个程序的核心。我们需要使用定时器来实现红、黄、绿灯的循环切换。
1、设置初始状态
首先,我们设置红绿灯的初始状态,红灯亮起。
canvas.itemconfig(red_light, fill='red')
current_light = 'red'
2、定义切换函数
我们需要定义一个函数来切换红绿灯的状态。
def switch_lights():
global current_light
if current_light == 'red':
canvas.itemconfig(red_light, fill='grey')
canvas.itemconfig(green_light, fill='green')
current_light = 'green'
window.after(5000, switch_lights) # Green light for 5 seconds
elif current_light == 'green':
canvas.itemconfig(green_light, fill='grey')
canvas.itemconfig(yellow_light, fill='yellow')
current_light = 'yellow'
window.after(2000, switch_lights) # Yellow light for 2 seconds
elif current_light == 'yellow':
canvas.itemconfig(yellow_light, fill='grey')
canvas.itemconfig(red_light, fill='red')
current_light = 'red'
window.after(5000, switch_lights) # Red light for 5 seconds
3、启动定时器
在程序启动时,我们需要启动定时器,以便红绿灯开始切换。
window.after(5000, switch_lights) # Start with red light for 5 seconds
五、完整代码
将以上所有部分结合起来,得到完整的红绿灯模拟程序。
import tkinter as tk
def create_window():
window = tk.Tk()
window.title("Traffic Light Simulation")
window.geometry("200x500")
return window
window = create_window()
canvas = tk.Canvas(window, width=200, height=500, bg='white')
canvas.pack()
Red light
red_light = canvas.create_oval(50, 50, 150, 150, fill='grey')
Yellow light
yellow_light = canvas.create_oval(50, 200, 150, 300, fill='grey')
Green light
green_light = canvas.create_oval(50, 350, 150, 450, fill='grey')
canvas.itemconfig(red_light, fill='red')
current_light = 'red'
def switch_lights():
global current_light
if current_light == 'red':
canvas.itemconfig(red_light, fill='grey')
canvas.itemconfig(green_light, fill='green')
current_light = 'green'
window.after(5000, switch_lights) # Green light for 5 seconds
elif current_light == 'green':
canvas.itemconfig(green_light, fill='grey')
canvas.itemconfig(yellow_light, fill='yellow')
current_light = 'yellow'
window.after(2000, switch_lights) # Yellow light for 2 seconds
elif current_light == 'yellow':
canvas.itemconfig(yellow_light, fill='grey')
canvas.itemconfig(red_light, fill='red')
current_light = 'red'
window.after(5000, switch_lights) # Red light for 5 seconds
window.after(5000, switch_lights) # Start with red light for 5 seconds
window.mainloop()
六、扩展与优化
1、可配置的时间间隔
我们可以将红、黄、绿灯的时间间隔设为可配置的参数,以便更灵活地控制红绿灯的切换时间。
red_time = 5000
yellow_time = 2000
green_time = 5000
def switch_lights():
global current_light
if current_light == 'red':
canvas.itemconfig(red_light, fill='grey')
canvas.itemconfig(green_light, fill='green')
current_light = 'green'
window.after(green_time, switch_lights)
elif current_light == 'green':
canvas.itemconfig(green_light, fill='grey')
canvas.itemconfig(yellow_light, fill='yellow')
current_light = 'yellow'
window.after(yellow_time, switch_lights)
elif current_light == 'yellow':
canvas.itemconfig(yellow_light, fill='grey')
canvas.itemconfig(red_light, fill='red')
current_light = 'red'
window.after(red_time, switch_lights)
2、增加按钮控制
我们可以增加按钮控制,使用户可以手动切换红绿灯。
def manual_switch():
if current_light == 'red':
canvas.itemconfig(red_light, fill='grey')
canvas.itemconfig(green_light, fill='green')
current_light = 'green'
elif current_light == 'green':
canvas.itemconfig(green_light, fill='grey')
canvas.itemconfig(yellow_light, fill='yellow')
current_light = 'yellow'
elif current_light == 'yellow':
canvas.itemconfig(yellow_light, fill='grey')
canvas.itemconfig(red_light, fill='red')
current_light = 'red'
button = tk.Button(window, text="Switch", command=manual_switch)
button.pack()
3、增加状态显示
我们可以增加一个标签来显示当前红绿灯的状态。
status_label = tk.Label(window, text="Red light")
status_label.pack()
def switch_lights():
global current_light
if current_light == 'red':
canvas.itemconfig(red_light, fill='grey')
canvas.itemconfig(green_light, fill='green')
current_light = 'green'
status_label.config(text="Green light")
window.after(green_time, switch_lights)
elif current_light == 'green':
canvas.itemconfig(green_light, fill='grey')
canvas.itemconfig(yellow_light, fill='yellow')
current_light = 'yellow'
status_label.config(text="Yellow light")
window.after(yellow_time, switch_lights)
elif current_light == 'yellow':
canvas.itemconfig(yellow_light, fill='grey')
canvas.itemconfig(red_light, fill='red')
current_light = 'red'
status_label.config(text="Red light")
window.after(red_time, switch_lights)
七、总结
通过本文的讲解,我们学习了如何使用Python的Tkinter库在电子屏上显示红绿灯。关键技术点包括:图形界面库的选择、颜色的设置、定时器的使用、循环逻辑的控制。希望通过本文的介绍,读者能够掌握基本的图形界面编程技巧,并能够灵活应用于其他项目中。
相关问答FAQs:
如何使用Python控制电子屏幕上的红绿灯显示?
要在电子屏上显示红绿灯,您可以使用Python的图形库,如Pygame或Tkinter。这些库允许您创建窗口和绘制形状。您需要定义红灯、黄灯和绿灯的状态,并使用定时器在它们之间切换。通过设置适当的颜色和形状,您可以模拟红绿灯的工作原理。
我需要哪些硬件来实现红绿灯显示?
通常情况下,您需要一个电子屏幕(如LED显示屏或LCD显示器),以及与Python代码通信的微控制器(如树莓派或Arduino)。确保您的硬件能够与Python进行交互,并且安装了相应的驱动程序和库,以便能够显示图形界面。
是否有现成的Python库可以帮助我快速实现红绿灯显示?
是的,有几个Python库可以帮助您快速实现红绿灯的显示,例如Pygame、Tkinter和Matplotlib等。Pygame适用于游戏开发和图形展示,而Tkinter则提供了一个简单的GUI框架。通过使用这些库,您可以更轻松地创建动画效果,并在电子屏上显示红绿灯的状态变化。
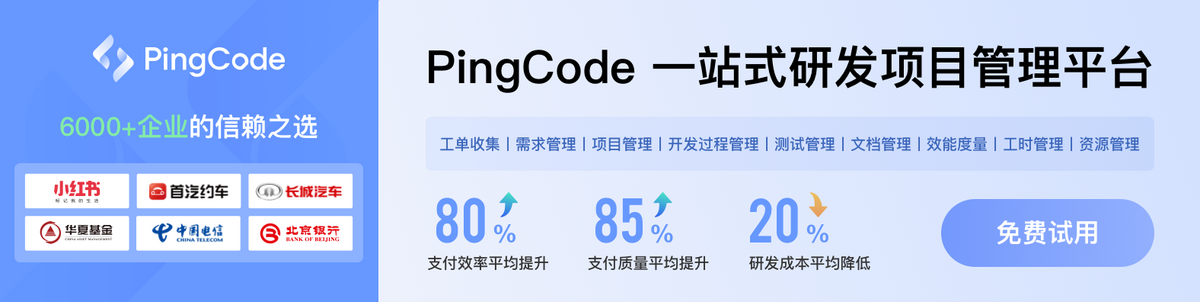