Python如何画一个分形几何图形
Python画分形几何图形的方法有很多,使用标准库Turtle、使用Pygame库、使用Matplotlib库。本文将详细介绍使用这些方法来绘制分形几何图形,并提供代码示例和详细解释。
一、使用Turtle库绘制分形几何图形
Turtle库是Python的标准库之一,非常适合用来绘制简单的图形和分形几何图形。
1. 安装和导入Turtle库
Turtle库是Python的标准库,不需要额外安装。只需要在代码中导入即可:
import turtle
2. 绘制分形树
分形树是一个典型的分形图形,可以通过递归函数实现。下面是一个简单的分形树绘制示例:
import turtle
def draw_branch(branch_length, t):
if branch_length > 5:
t.forward(branch_length)
t.right(20)
draw_branch(branch_length - 15, t)
t.left(40)
draw_branch(branch_length - 15, t)
t.right(20)
t.backward(branch_length)
def main():
t = turtle.Turtle()
my_win = turtle.Screen()
t.left(90)
t.up()
t.backward(100)
t.down()
t.color("green")
draw_branch(75, t)
my_win.exitonclick()
if __name__ == "__main__":
main()
在这个示例中,函数draw_branch
是一个递归函数,每次调用自己来绘制分支,直到分支长度小于等于5。通过调整参数,可以绘制出不同形状的分形树。
3. 绘制Sierpinski三角形
Sierpinski三角形是另一种常见的分形图形。可以使用递归方法来绘制:
import turtle
def draw_triangle(points, color, t):
t.fillcolor(color)
t.up()
t.goto(points[0][0], points[0][1])
t.down()
t.begin_fill()
t.goto(points[1][0], points[1][1])
t.goto(points[2][0], points[2][1])
t.goto(points[0][0], points[0][1])
t.end_fill()
def get_mid(p1, p2):
return ((p1[0] + p2[0]) / 2, (p1[1] + p2[1]) / 2)
def sierpinski(points, degree, t):
colormap = ['blue', 'red', 'green', 'white', 'yellow', 'violet', 'orange']
draw_triangle(points, colormap[degree], t)
if degree > 0:
sierpinski([points[0],
get_mid(points[0], points[1]),
get_mid(points[0], points[2])],
degree-1, t)
sierpinski([points[1],
get_mid(points[0], points[1]),
get_mid(points[1], points[2])],
degree-1, t)
sierpinski([points[2],
get_mid(points[2], points[1]),
get_mid(points[0], points[2])],
degree-1, t)
def main():
t = turtle.Turtle()
my_win = turtle.Screen()
my_points = [[-200, -100], [0, 200], [200, -100]]
sierpinski(my_points, 3, t)
my_win.exitonclick()
if __name__ == "__main__":
main()
这里,draw_triangle
函数绘制一个三角形,sierpinski
函数递归地调用自己来绘制Sierpinski三角形。通过调整递归深度,可以绘制出不同层次的Sierpinski三角形。
二、使用Pygame库绘制分形几何图形
Pygame是一个跨平台的Python模块,用于开发视频游戏。它包括计算机图形和声音库,适合绘制复杂的分形几何图形。
1. 安装和导入Pygame库
首先需要安装Pygame库,可以使用pip进行安装:
pip install pygame
然后在代码中导入Pygame库:
import pygame
import math
2. 绘制Mandelbrot集合
Mandelbrot集合是著名的分形图形,可以使用Pygame来绘制:
import pygame
import numpy as np
Initialize Pygame
pygame.init()
Set up display
width, height = 800, 800
window = pygame.display.set_mode((width, height))
pygame.display.set_caption("Mandelbrot Set")
Define colors
black = (0, 0, 0)
white = (255, 255, 255)
Mandelbrot parameters
max_iter = 256
zoom = 1
move_x = 0
move_y = 0
def mandelbrot(c, max_iter):
z = c
for n in range(max_iter):
if abs(z) > 2:
return n
z = z * z + c
return max_iter
def draw_mandelbrot():
for x in range(width):
for y in range(height):
c = complex((x - width/2) * 4/width * zoom + move_x,
(y - height/2) * 4/height * zoom + move_y)
m = mandelbrot(c, max_iter)
color = 255 - int(m * 255 / max_iter)
window.set_at((x, y), (color, color, color))
Main loop
running = True
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
draw_mandelbrot()
pygame.display.update()
pygame.quit()
在这个示例中,函数mandelbrot
计算Mandelbrot集合中的点,draw_mandelbrot
函数绘制图形。通过调整参数,可以生成不同的Mandelbrot图形。
三、使用Matplotlib库绘制分形几何图形
Matplotlib是一个绘图库,它提供了一种简单的方法来绘制分形几何图形。
1. 安装和导入Matplotlib库
首先需要安装Matplotlib库,可以使用pip进行安装:
pip install matplotlib
然后在代码中导入Matplotlib库:
import matplotlib.pyplot as plt
import numpy as np
2. 绘制Julia集合
Julia集合是另一种著名的分形图形,可以使用Matplotlib来绘制:
import matplotlib.pyplot as plt
import numpy as np
Define the function to generate Julia set
def julia_set(width, height, zoom, move_x, move_y, c, max_iter):
x = np.linspace(-1.5, 1.5, width)
y = np.linspace(-1.5, 1.5, height)
X, Y = np.meshgrid(x, y)
Z = X + 1j * Y
img = np.zeros(Z.shape, dtype=int)
for i in range(max_iter):
Z = Z * Z + c
mask = np.abs(Z) < 10
img += mask
return img
Parameters
width, height = 800, 800
zoom = 1
move_x = 0
move_y = 0
c = complex(-0.7, 0.27015)
max_iter = 256
Generate Julia set and plot
img = julia_set(width, height, zoom, move_x, move_y, c, max_iter)
plt.imshow(img, extent=[-1.5, 1.5, -1.5, 1.5], cmap='inferno')
plt.colorbar()
plt.title("Julia Set")
plt.show()
在这个示例中,函数julia_set
生成Julia集合的点,并返回一个图像数组。通过调整参数,可以生成不同的Julia图形。
四、总结
本文介绍了如何使用Python绘制分形几何图形,分别使用了Turtle、Pygame和Matplotlib库。每种方法都有其独特的优势和适用场景,读者可以根据自己的需求选择合适的方法来绘制分形几何图形。希望本文对你有所帮助,祝你在分形几何图形的绘制中取得成功!
相关问答FAQs:
Python可以用什么库来绘制分形几何图形?
在Python中,常用的库有Matplotlib和Turtle。Matplotlib非常适合生成静态的分形图形,比如曼德尔布罗特集,而Turtle则适合动态绘制分形,如科赫雪花或谢尔宾斯基三角形。选择合适的库可以帮助你更好地实现你的绘图需求。
如何设置分形图形的递归深度?
分形图形的复杂度通常由递归深度决定。在绘制分形时,可以通过一个参数来控制递归的层数。例如,在生成谢尔宾斯基三角形时,可以设置一个变量来控制递归调用的次数,越高的层数会导致图形越复杂,细节也越丰富。
分形几何图形的应用场景有哪些?
分形几何不仅在艺术创作中广受欢迎,还在自然界、计算机图形学、数据压缩、生态模型等领域有广泛应用。例如,模拟自然景观、生成复杂的图像纹理,甚至用于描述一些生物生长的模型。了解这些应用场景可以激发你对分形几何的更深层次探索。
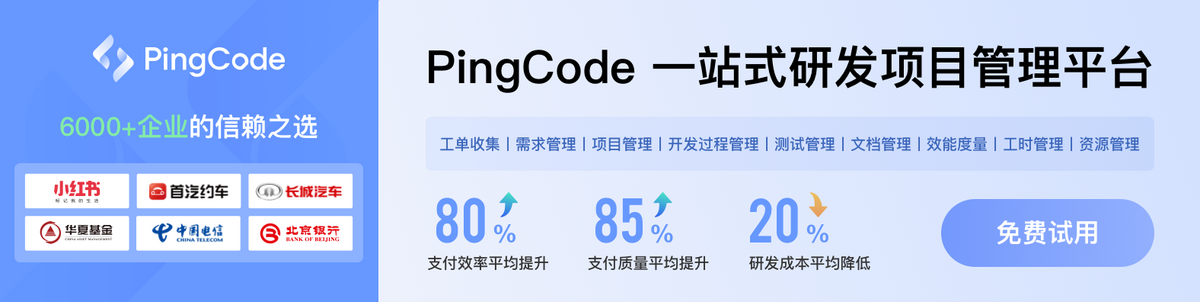