在Python中画多彩五角星的方法有很多种,通常使用的库有Turtle、Matplotlib和Pygame。其中,Turtle库是一种非常直观和简单的方式,适合初学者;Matplotlib则适合那些对绘图有更高要求的人;而Pygame则更适合需要进行游戏开发和复杂动画的人。本文将详细介绍如何使用Turtle库来画一个多彩的五角星,并进一步探讨如何使用Matplotlib和Pygame实现相同的效果。
一、使用Turtle库画多彩五角星
1、安装与导入Turtle库
Turtle库是Python的标准库之一,不需要额外安装。你只需在你的Python环境中导入它即可。
import turtle
2、设置窗口和画笔
首先,设置窗口的背景颜色和画笔的属性,例如颜色、速度和粗细。
screen = turtle.Screen()
screen.bgcolor("black")
star = turtle.Turtle()
star.speed(10)
star.pensize(2)
3、画五角星的函数
定义一个函数来画五角星。这个函数将接收颜色作为参数,以便可以画出多彩的五角星。
def draw_star(color, size):
star.color(color)
star.begin_fill()
for _ in range(5):
star.forward(size)
star.right(144)
star.end_fill()
4、画多彩五角星
使用不同的颜色调用draw_star
函数来画多彩五角星。
colors = ["red", "blue", "green", "yellow", "purple"]
for color in colors:
draw_star(color, 100)
star.penup()
star.forward(150)
star.pendown()
5、完成绘图
最后,隐藏画笔并保持窗口打开以显示结果。
star.hideturtle()
turtle.done()
二、使用Matplotlib画多彩五角星
1、安装与导入Matplotlib库
如果你还没有安装Matplotlib,可以使用pip进行安装。
pip install matplotlib
然后在你的Python脚本中导入所需的模块。
import matplotlib.pyplot as plt
import numpy as np
2、定义五角星的顶点
使用NumPy来计算五角星的顶点位置。
def star_points(center, size):
points = []
for i in range(5):
angle = i * 144 * np.pi / 180
x = center[0] + size * np.cos(angle)
y = center[1] + size * np.sin(angle)
points.append((x, y))
return points
3、画多彩五角星
使用Matplotlib的fill
函数来画多彩五角星。
colors = ["red", "blue", "green", "yellow", "purple"]
fig, ax = plt.subplots()
for i, color in enumerate(colors):
points = star_points((i*2, 0), 1)
polygon = plt.Polygon(points, closed=True, fill=True, color=color)
ax.add_patch(polygon)
ax.set_aspect('equal')
plt.xlim(-2, 10)
plt.ylim(-2, 2)
plt.axis('off')
plt.show()
三、使用Pygame画多彩五角星
1、安装与导入Pygame库
如果你还没有安装Pygame,可以使用pip进行安装。
pip install pygame
然后在你的Python脚本中导入所需的模块。
import pygame
import math
2、初始化Pygame
初始化Pygame并设置窗口大小。
pygame.init()
screen = pygame.display.set_mode((800, 600))
pygame.display.set_caption("Colorful Stars")
3、定义五角星的顶点
使用math模块计算五角星的顶点位置。
def star_points(center, size):
points = []
for i in range(5):
angle = i * 144 * math.pi / 180
x = center[0] + size * math.cos(angle)
y = center[1] + size * math.sin(angle)
points.append((x, y))
return points
4、画多彩五角星
使用Pygame的draw.polygon
函数来画多彩五角星。
colors = [(255, 0, 0), (0, 0, 255), (0, 255, 0), (255, 255, 0), (128, 0, 128)]
for i, color in enumerate(colors):
points = star_points((i*150 + 100, 300), 50)
pygame.draw.polygon(screen, color, points)
5、完成绘图
保持窗口打开以显示结果。
running = True
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
pygame.display.flip()
pygame.quit()
通过以上三种方法,你可以在Python中画出多彩的五角星。每种方法都有其优点和适用场景,你可以根据自己的需求选择适合的方法。Turtle库简单易用,非常适合初学者;Matplotlib功能强大,适合需要高质量绘图的人;Pygame则提供了更多的灵活性,适合需要进行游戏开发和复杂动画的人。
相关问答FAQs:
如何在Python中绘制五角星的基本步骤是什么?
绘制五角星的基本步骤包括选择一个绘图库,例如matplotlib或turtle。使用这些库可以创建五角星的顶点坐标,并利用绘图函数将这些顶点连接起来。通过设置不同的颜色和线条样式,可以使五角星更加多彩。
在Python中使用哪个库绘制多彩五角星最为简单?
turtle库是一个非常适合初学者的选择,因其简单易用。只需几行代码,就可以轻松绘制出五角星,并为其填充多种颜色。matplotlib也是一个不错的选择,适合需要更多自定义和复杂图形的用户。
如何为五角星添加渐变色效果?
可以通过使用matplotlib中的颜色映射功能来实现渐变色效果。在绘制五角星时,可以为每个顶点分配不同的颜色值,利用颜色映射函数生成渐变效果,使五角星看起来更加生动。
在绘制五角星时,如何调整其大小和位置?
五角星的大小和位置可以通过修改顶点坐标的比例和偏移量来实现。在使用turtle库时,可以通过调用相应的移动和缩放命令来调整五角星的显示效果,而在matplotlib中,可以通过设置坐标轴的范围来改变五角星的大小和位置。
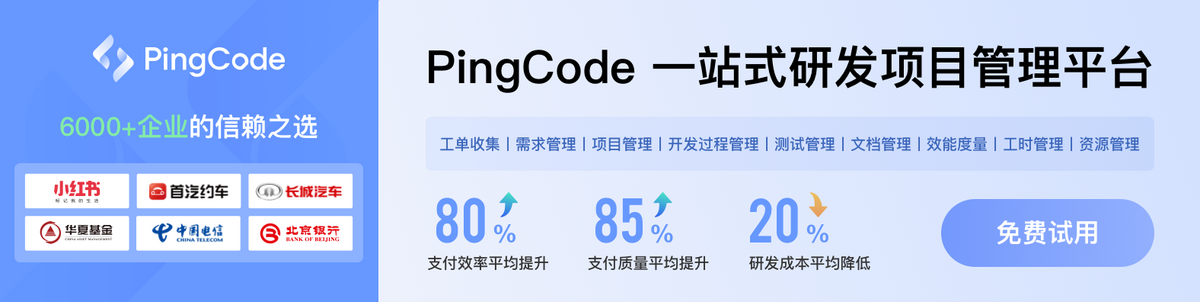