Python监控一个爬虫的运行状态可以通过以下几种方法:日志记录、进度条、异常处理、性能监测、外部监控工具。 其中,日志记录是最常用和有效的方法之一,通过日志记录可以详细了解爬虫的运行状态、抓取的数据量、遇到的错误等信息。
日志记录是一种非常有效的方法,它可以将爬虫的运行状态实时记录下来,方便后期分析和调试。Python内置的logging
模块可以方便地实现这一点。通过设置不同的日志级别(如DEBUG、INFO、WARNING、ERROR等),可以记录从调试信息到严重错误的各种信息。
以下是详细内容:
一、日志记录
1、设置日志记录
日志记录是监控爬虫运行状态的基础,Python内置的logging
模块可以非常方便地实现这一点。首先,需要设置日志记录的格式和级别。
import logging
创建logger
logger = logging.getLogger('my_spider')
logger.setLevel(logging.DEBUG)
创建一个handler,用于写入日志文件
fh = logging.FileHandler('spider.log')
fh.setLevel(logging.DEBUG)
再创建一个handler,用于输出到控制台
ch = logging.StreamHandler()
ch.setLevel(logging.DEBUG)
定义handler的输出格式
formatter = logging.Formatter('%(asctime)s - %(name)s - %(levelname)s - %(message)s')
fh.setFormatter(formatter)
ch.setFormatter(formatter)
给logger添加handler
logger.addHandler(fh)
logger.addHandler(ch)
2、记录爬虫运行状态
在爬虫的各个关键节点,添加日志记录代码,以便详细了解爬虫的运行状态。
import requests
from bs4 import BeautifulSoup
def crawl(url):
logger.info(f'Start crawling: {url}')
try:
response = requests.get(url)
response.raise_for_status()
logger.info(f'Successfully fetched the URL: {url}')
return response.text
except requests.RequestException as e:
logger.error(f'Error fetching the URL: {url} - {str(e)}')
return None
def parse(html):
logger.info('Start parsing HTML')
try:
soup = BeautifulSoup(html, 'html.parser')
# 假设我们要抓取标题
title = soup.title.string
logger.info(f'Extracted title: {title}')
return title
except Exception as e:
logger.error(f'Error parsing HTML: {str(e)}')
return None
if __name__ == '__main__':
url = 'http://example.com'
html = crawl(url)
if html:
parse(html)
二、进度条
1、使用tqdm库
在长时间运行的爬虫任务中,使用进度条可以直观地看到爬虫的进度。tqdm
库是一个非常方便的工具,可以在终端和Jupyter Notebook中显示进度条。
from tqdm import tqdm
urls = ['http://example.com/page1', 'http://example.com/page2', 'http://example.com/page3']
for url in tqdm(urls):
html = crawl(url)
if html:
parse(html)
2、自定义进度条
有时,标准的进度条不能完全满足需求,可以自定义进度条来更精确地监控爬虫状态。
import time
total_pages = 100
for i in range(total_pages):
time.sleep(0.1) # 模拟爬取过程
print(f'\rProgress: {i+1}/{total_pages} ({(i+1)/total_pages*100:.2f}%)', end='')
三、异常处理
1、捕获和记录异常
在爬虫运行过程中,难免会遇到各种异常情况,及时捕获和记录这些异常可以帮助我们快速定位和解决问题。
try:
response = requests.get(url)
response.raise_for_status()
except requests.RequestException as e:
logger.error(f'Error fetching the URL: {url} - {str(e)}')
# 可以选择继续或终止爬虫
2、重试机制
针对一些临时性错误,可以设置重试机制,提高爬虫的稳定性。
from tenacity import retry, wait_fixed, stop_after_attempt
@retry(wait=wait_fixed(2), stop=stop_after_attempt(3))
def fetch(url):
response = requests.get(url)
response.raise_for_status()
return response.text
四、性能监测
1、CPU和内存使用情况
监控爬虫的性能也非常重要,尤其是在爬取大量数据时。可以使用psutil
库来获取CPU和内存的使用情况。
import psutil
print(f'CPU usage: {psutil.cpu_percent()}%')
print(f'Memory usage: {psutil.virtual_memory().percent}%')
2、监控网络流量
网络流量是爬虫的关键指标,监控流量可以帮助我们判断爬虫的效率和是否被目标网站限制。
import psutil
net_io = psutil.net_io_counters()
print(f'Bytes sent: {net_io.bytes_sent}')
print(f'Bytes received: {net_io.bytes_recv}')
五、外部监控工具
1、使用Prometheus和Grafana
Prometheus和Grafana是常用的监控和可视化工具,可以帮助我们实时监控爬虫的各种指标。
2、集成Prometheus
首先,安装prometheus_client
库。
pip install prometheus_client
然后,在爬虫代码中集成Prometheus。
from prometheus_client import start_http_server, Summary
REQUEST_TIME = Summary('request_processing_seconds', 'Time spent processing request')
@REQUEST_TIME.time()
def crawl(url):
logger.info(f'Start crawling: {url}')
try:
response = requests.get(url)
response.raise_for_status()
logger.info(f'Successfully fetched the URL: {url}')
return response.text
except requests.RequestException as e:
logger.error(f'Error fetching the URL: {url} - {str(e)}')
return None
if __name__ == '__main__':
start_http_server(8000) # 启动Prometheus HTTP服务器
url = 'http://example.com'
html = crawl(url)
if html:
parse(html)
3、使用Grafana进行可视化
配置Prometheus数据源,然后在Grafana中创建仪表板,添加相关的图表来监控爬虫的运行状态。
结论
监控爬虫的运行状态是确保其稳定性和高效性的关键。通过日志记录、进度条、异常处理、性能监测和外部监控工具,可以全面了解和掌握爬虫的运行状态,从而及时发现和解决问题,确保数据抓取任务顺利进行。
相关问答FAQs:
如何设置日志记录以监控爬虫的运行状态?
在 Python 中,可以使用内置的 logging 模块来记录爬虫的运行状态。通过设置不同的日志级别(如 DEBUG、INFO、WARNING、ERROR 和 CRITICAL),可以监控爬虫的各个阶段。建议在爬虫的关键位置添加日志记录,例如请求发送、数据解析和错误处理,确保可以追踪到每一步的执行情况。
有什么工具可以帮助我监控爬虫的性能?
可以考虑使用一些监控工具,如 Prometheus 和 Grafana。这些工具可以收集爬虫的性能指标,如请求速度、响应时间和成功率,并将数据可视化,帮助您实时监控爬虫的运行状态。此外,使用 APM(应用性能管理)工具如 New Relic 或 Sentry 也可以有效地监测爬虫的性能和异常。
如何处理爬虫中的异常以确保其稳定运行?
在编写爬虫时,加入异常处理机制是非常重要的。使用 try-except 语句来捕获可能出现的异常,并在捕获到异常时记录相关信息,这样可以避免爬虫因单个错误而崩溃。此外,可以实现重试机制,当请求失败时,自动重试一到两次,确保爬虫在面对临时问题时依然能够稳定运行。
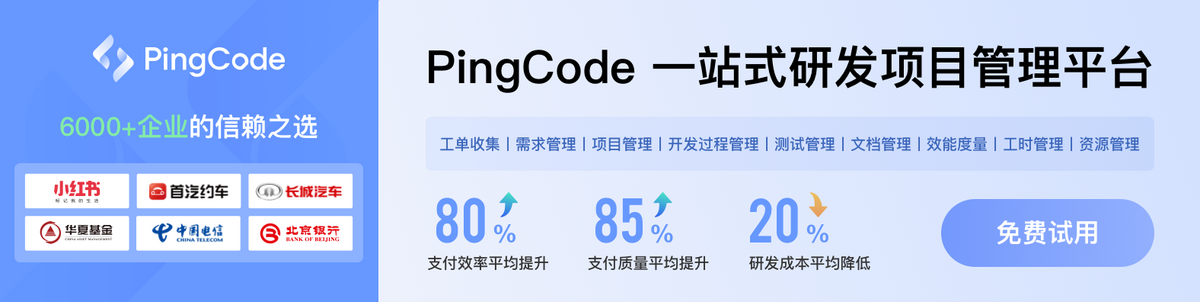