要在Python中改变字符串中的某一个值,可以使用字符串切片、字符串替换方法、或者利用列表转换的方法。 由于字符串是不可变的类型,所以你不能直接修改字符串中的某个字符,而是需要创建一个新的字符串。以下是详细说明如何实现这一点。
字符串切片方法是一个常见的手段。通过这种方法,你可以将字符串分为两部分,替换中间的字符,然后将三部分重新组合成一个新的字符串。这种方法的优点是简单直观,特别适合处理单个字符的替换。
接下来,我们将深入探讨不同的方法及其实现方式。
一、字符串切片方法
字符串切片是一种简单且直观的方法。你可以将字符串分为三部分:替换字符之前的部分、替换字符本身、替换字符之后的部分。然后将它们组合成一个新的字符串。
def replace_char_in_string(s, index, new_char):
if not (0 <= index < len(s)):
raise ValueError("Index out of range")
return s[:index] + new_char + s[index+1:]
示例
original_string = "hello"
new_string = replace_char_in_string(original_string, 1, 'a')
print(new_string) # hallo
二、字符串替换方法
虽然字符串替换方法主要用于替换子字符串,但也可以用于替换单个字符。通过这种方法,你可以使用str.replace(old, new, count)
方法将字符串中的某个字符替换为另一个字符。不过需要注意的是,这种方法通常会替换字符串中所有匹配的字符。
def replace_char(s, old_char, new_char):
return s.replace(old_char, new_char)
示例
original_string = "hello"
new_string = replace_char(original_string, 'l', 'x')
print(new_string) # hexxo
三、利用列表转换的方法
由于字符串是不可变的,而列表是可变的,你可以将字符串转换为列表,修改列表中的元素,然后再将列表转换回字符串。这种方法适合需要进行多次修改的场景。
def replace_char_using_list(s, index, new_char):
if not (0 <= index < len(s)):
raise ValueError("Index out of range")
s_list = list(s)
s_list[index] = new_char
return ''.join(s_list)
示例
original_string = "hello"
new_string = replace_char_using_list(original_string, 1, 'a')
print(new_string) # hallo
四、字符串格式化方法
字符串格式化方法也是一种灵活的方式,尤其是在需要动态生成字符串的场景中。你可以使用格式化方法来构建新的字符串。
def replace_char_with_format(s, index, new_char):
if not (0 <= index < len(s)):
raise ValueError("Index out of range")
return f"{s[:index]}{new_char}{s[index+1:]}"
示例
original_string = "hello"
new_string = replace_char_with_format(original_string, 1, 'a')
print(new_string) # hallo
五、正则表达式替换
在某些情况下,正则表达式可以提供更强大的字符串操作功能。通过re
模块,你可以使用正则表达式进行复杂的字符串替换。
import re
def replace_char_with_regex(s, index, new_char):
if not (0 <= index < len(s)):
raise ValueError("Index out of range")
pattern = re.compile(f"(.{{{index}}}).(.{{{len(s) - index - 1}}})")
return pattern.sub(rf"\1{new_char}\2", s)
示例
original_string = "hello"
new_string = replace_char_with_regex(original_string, 1, 'a')
print(new_string) # hallo
六、性能比较
在处理字符串替换时,性能可能是一个需要考虑的因素。特别是在处理大数据或在性能敏感的应用场景中,不同方法的效率可能会有所不同。通过以下示例,我们可以比较不同方法的执行效率。
import timeit
original_string = "hello"
def test_replace_char_in_string():
replace_char_in_string(original_string, 1, 'a')
def test_replace_char():
replace_char(original_string, 'l', 'x')
def test_replace_char_using_list():
replace_char_using_list(original_string, 1, 'a')
def test_replace_char_with_format():
replace_char_with_format(original_string, 1, 'a')
def test_replace_char_with_regex():
replace_char_with_regex(original_string, 1, 'a')
print(timeit.timeit(test_replace_char_in_string, number=100000))
print(timeit.timeit(test_replace_char, number=100000))
print(timeit.timeit(test_replace_char_using_list, number=100000))
print(timeit.timeit(test_replace_char_with_format, number=100000))
print(timeit.timeit(test_replace_char_with_regex, number=100000))
七、实际应用场景
不同的方法适用于不同的实际应用场景。例如,如果你只需要替换字符串中的一个字符,字符串切片和列表转换方法可能是最合适的。如果你需要进行更复杂的字符串操作,正则表达式可能会更有用。
-
简单替换:对于简单的字符替换,字符串切片和列表转换方法是最直观和高效的选择。
-
批量替换:当需要一次性替换多个字符时,可以使用字符串替换方法或正则表达式。
-
动态生成字符串:在动态生成字符串的场景中,字符串格式化方法可以提供更大的灵活性。
总之,了解不同的方法及其适用场景,可以帮助你在实际开发中选择最合适的解决方案。这不仅能够提高代码的可读性和可维护性,还能在一定程度上提升性能。
相关问答FAQs:
如何在Python中替换字符串中的特定字符?
在Python中,可以使用字符串的replace()
方法来替换特定字符或子字符串。语法为 string.replace(old, new, count)
,其中old
是要被替换的字符,new
是替换后的字符,count
是可选参数,表示替换的次数。例如:
original_string = "Hello World"
new_string = original_string.replace("o", "0")
print(new_string) # 输出:Hell0 W0rld
这种方式简单有效,适用于大多数基本的替换需求。
在Python中是否可以使用正则表达式来替换字符串中的值?
是的,Python的re
模块提供了强大的正则表达式功能,可以用来替换字符串中的字符。使用re.sub(pattern, replacement, string)
方法可以根据指定的模式进行替换。例如:
import re
original_string = "Hello World"
new_string = re.sub(r"[oO]", "0", original_string)
print(new_string) # 输出:Hell0 W0rld
这种方法适合于需要根据复杂规则进行替换的场景。
如果要替换的字符在字符串中不存在,会发生什么情况?
如果使用replace()
方法替换的字符在原字符串中不存在,返回的结果将与原字符串相同。例如:
original_string = "Hello World"
new_string = original_string.replace("x", "y")
print(new_string) # 输出:Hello World
这种行为确保了代码的健壮性,不会引发错误。
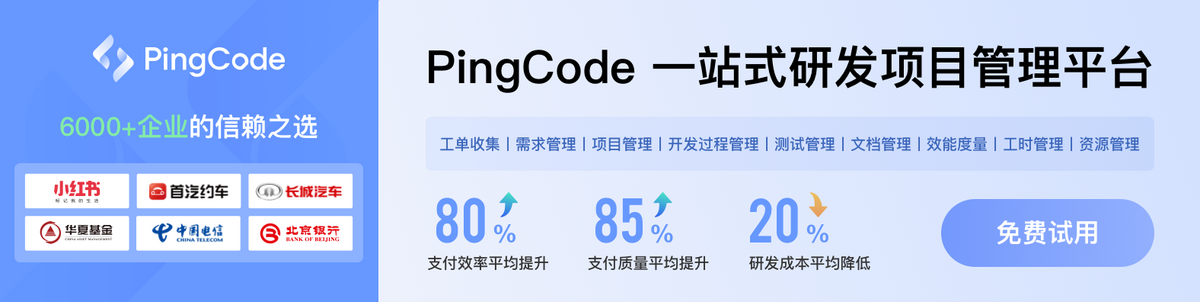