Python多线程通过使用threading
模块来实现并发执行多个线程。Python多线程、并发处理、GIL影响。其中,Python多线程可以通过创建和启动线程对象实现。并发处理意味着多个线程可以在同一时间段内执行,而GIL(全局解释器锁)影响了Python多线程的效率,需要特别注意。接下来,我们详细介绍Python多线程的相关内容。
一、Python多线程概述
Python中的多线程是一种在程序中创建多个线程来执行并发任务的方法。线程是轻量级的子进程,与进程相比,线程之间的上下文切换开销较小。Python多线程主要用于I/O密集型任务,例如网络请求、文件读写等。
1. 线程与进程的区别
进程是操作系统分配资源和调度的基本单位,每个进程都有独立的内存空间和资源。线程是进程中的一个执行单元,同一进程中的多个线程共享进程的资源。
- 进程:独立的内存空间,资源开销大,适合CPU密集型任务。
- 线程:共享进程资源,资源开销小,适合I/O密集型任务。
2. Python线程的实现
Python通过threading
模块来创建和管理线程。以下是一个简单的多线程示例:
import threading
import time
def worker():
print("Worker thread is running")
time.sleep(2)
print("Worker thread has finished")
创建线程对象
thread = threading.Thread(target=worker)
启动线程
thread.start()
等待线程结束
thread.join()
print("Main thread has finished")
二、Python多线程的优势与劣势
1. 优势
- 并发执行:多线程可以同时执行多个任务,提高程序的并发性和响应性。
- 资源共享:同一进程中的线程共享进程的资源,资源开销小。
- 适合I/O密集型任务:多线程可以有效地处理I/O密集型任务,例如网络请求、文件读写等。
2. 劣势
- 全局解释器锁(GIL):Python的GIL限制了同一时刻只有一个线程执行Python字节码,影响了多线程的性能。
- 线程安全问题:多线程访问共享资源时可能会出现竞争条件,需要使用锁机制来保证线程安全。
- 调试难度大:多线程程序的调试和维护难度较大,容易出现死锁和竞态条件等问题。
三、全局解释器锁(GIL)
全局解释器锁(GIL)是CPython解释器中的一个全局锁,用于保护Python对象的内存管理。由于GIL的存在,同一时刻只有一个线程可以执行Python字节码,这限制了多线程的并发性能。
1. GIL的影响
GIL的存在使得Python多线程在CPU密集型任务中无法充分利用多核CPU的优势,因为多个线程不能真正并行执行。虽然GIL在I/O密集型任务中影响较小,但在高并发场景下仍然可能成为性能瓶颈。
2. 解决GIL问题的方法
- 使用多进程:通过
multiprocessing
模块创建多个进程,每个进程都有独立的GIL,可以充分利用多核CPU。 - 使用C扩展:将性能关键部分用C语言实现,释放GIL,提高执行效率。
- 选择其他解释器:如Jython、IronPython等,它们没有GIL限制,但需要考虑兼容性问题。
四、线程同步与线程安全
多线程访问共享资源时需要注意线程同步和线程安全问题。Python提供了多种同步机制来解决这些问题。
1. 锁(Lock)
锁是最基本的同步机制,用于保护共享资源,确保同一时刻只有一个线程可以访问共享资源。
import threading
lock = threading.Lock()
def worker():
with lock:
# 访问共享资源
pass
2. 递归锁(RLock)
递归锁允许同一线程多次获取锁,而不会发生死锁。
import threading
lock = threading.RLock()
def worker():
with lock:
with lock:
# 访问共享资源
pass
3. 条件变量(Condition)
条件变量用于线程间通信和协作,线程可以等待某个条件满足后再继续执行。
import threading
condition = threading.Condition()
def worker():
with condition:
condition.wait()
# 条件满足后继续执行
4. 信号量(Semaphore)
信号量用于控制对共享资源的访问,允许一定数量的线程同时访问共享资源。
import threading
semaphore = threading.Semaphore(2)
def worker():
with semaphore:
# 访问共享资源
pass
五、Python多线程的实际应用
1. 网络请求
多线程可以加速网络请求,提高网络爬虫和API调用的效率。
import threading
import requests
def fetch_url(url):
response = requests.get(url)
print(f"Fetched {url}: {response.status_code}")
urls = ["https://example.com", "https://example.org", "https://example.net"]
threads = []
for url in urls:
thread = threading.Thread(target=fetch_url, args=(url,))
thread.start()
threads.append(thread)
for thread in threads:
thread.join()
2. 文件读写
多线程可以加速文件读写操作,提高数据处理的效率。
import threading
def read_file(file_path):
with open(file_path, 'r') as file:
data = file.read()
print(f"Read {file_path}: {len(data)} bytes")
file_paths = ["file1.txt", "file2.txt", "file3.txt"]
threads = []
for file_path in file_paths:
thread = threading.Thread(target=read_file, args=(file_path,))
thread.start()
threads.append(thread)
for thread in threads:
thread.join()
3. 数据处理
多线程可以加速数据处理任务,例如数据清洗、数据转换等。
import threading
def process_data(data):
# 数据处理逻辑
pass
data_chunks = [data1, data2, data3]
threads = []
for data in data_chunks:
thread = threading.Thread(target=process_data, args=(data,))
thread.start()
threads.append(thread)
for thread in threads:
thread.join()
六、Python多线程的最佳实践
1. 避免使用全局变量
全局变量在多线程程序中可能会导致数据竞争和线程安全问题,尽量使用局部变量或线程本地存储。
2. 使用线程池
线程池可以高效管理线程资源,避免频繁创建和销毁线程带来的开销。Python的concurrent.futures
模块提供了线程池的实现。
from concurrent.futures import ThreadPoolExecutor
def worker(data):
# 任务处理逻辑
pass
data_list = [data1, data2, data3]
with ThreadPoolExecutor(max_workers=3) as executor:
executor.map(worker, data_list)
3. 使用上下文管理器
使用上下文管理器可以简化线程同步代码,提高代码的可读性和可靠性。
import threading
lock = threading.Lock()
def worker():
with lock:
# 访问共享资源
pass
4. 处理异常
多线程程序中,线程内部的异常不会传播到主线程,需要在每个线程中处理异常,避免程序崩溃。
import threading
def worker():
try:
# 任务处理逻辑
pass
except Exception as e:
print(f"Error in worker thread: {e}")
thread = threading.Thread(target=worker)
thread.start()
thread.join()
七、Python多线程的调试与测试
1. 日志记录
使用日志记录线程的执行情况,有助于调试和定位问题。Python的logging
模块提供了强大的日志记录功能。
import logging
import threading
logging.basicConfig(level=logging.INFO, format='%(asctime)s - %(threadName)s - %(message)s')
def worker():
logging.info("Worker thread is running")
# 任务处理逻辑
logging.info("Worker thread has finished")
thread = threading.Thread(target=worker, name="WorkerThread")
thread.start()
thread.join()
2. 单元测试
为多线程程序编写单元测试,确保每个线程的任务逻辑正确。Python的unittest
模块支持多线程测试。
import unittest
import threading
class TestWorker(unittest.TestCase):
def test_worker(self):
def worker():
# 任务处理逻辑
pass
thread = threading.Thread(target=worker)
thread.start()
thread.join()
if __name__ == "__main__":
unittest.main()
3. 使用调试工具
使用调试工具如pdb
、pycharm
等,可以单步调试多线程程序,帮助发现问题。
八、Python多线程的性能优化
1. 减少锁竞争
锁竞争会影响多线程程序的性能,尽量减少锁的使用范围和时间,避免长时间持有锁。
2. 使用线程池
线程池可以高效管理线程资源,避免频繁创建和销毁线程带来的开销,提高程序性能。
3. 优化I/O操作
I/O操作是多线程程序的性能瓶颈,优化I/O操作可以显著提高程序性能。例如,使用异步I/O或批量处理数据。
九、Python多线程的安全性
1. 使用线程安全的数据结构
Python提供了一些线程安全的数据结构,例如queue.Queue
、collections.deque
等,使用这些数据结构可以避免数据竞争和线程安全问题。
import queue
import threading
q = queue.Queue()
def producer():
for i in range(10):
q.put(i)
print(f"Produced: {i}")
def consumer():
while not q.empty():
item = q.get()
print(f"Consumed: {item}")
producer_thread = threading.Thread(target=producer)
consumer_thread = threading.Thread(target=consumer)
producer_thread.start()
producer_thread.join()
consumer_thread.start()
consumer_thread.join()
2. 使用锁机制
在访问共享资源时使用锁机制,确保线程安全。Python提供了多种锁机制,例如threading.Lock
、threading.RLock
等。
import threading
lock = threading.Lock()
shared_resource = 0
def increment():
global shared_resource
with lock:
shared_resource += 1
print(f"Incremented: {shared_resource}")
threads = [threading.Thread(target=increment) for _ in range(10)]
for thread in threads:
thread.start()
for thread in threads:
thread.join()
十、Python多线程的常见问题与解决方案
1. 死锁
死锁是指两个或多个线程互相等待对方释放资源,导致程序无法继续执行。避免死锁的方法包括:
- 尽量减少锁的使用,使用高效的锁机制。
- 保证所有线程以相同的顺序获取多个锁。
- 使用超时机制,避免长时间等待锁。
2. 竞态条件
竞态条件是指多个线程竞争访问共享资源,导致数据不一致的问题。解决竞态条件的方法包括:
- 使用锁机制保护共享资源。
- 使用线程安全的数据结构。
- 使用原子操作,例如
queue.Queue
的put
和get
方法。
3. 线程泄漏
线程泄漏是指线程未能正确终止,导致资源泄漏的问题。避免线程泄漏的方法包括:
- 使用线程池管理线程,避免频繁创建和销毁线程。
- 确保每个线程在完成任务后正确终止,使用
thread.join()
等待线程结束。
总结
Python多线程通过threading
模块实现,适用于I/O密集型任务。多线程可以提高程序的并发性和响应性,但需要注意GIL的影响和线程安全问题。通过合理使用锁机制、线程池和线程安全的数据结构,可以编写高效、可靠的多线程程序。同时,使用日志记录、单元测试和调试工具,可以帮助调试和测试多线程程序。
相关问答FAQs:
Python多线程的优势是什么?
Python多线程可以提升程序的并发性,尤其在处理IO密集型任务时表现优异。通过在不同线程中同时执行多个任务,可以有效利用系统资源,提高程序的响应速度和用户体验。虽然Python的全局解释器锁(GIL)限制了CPU密集型任务的并行执行,但对于网络请求、文件读写等IO操作,多线程依然能够显著提高效率。
Python多线程适合哪些应用场景?
多线程在需要同时处理多个任务的场景中表现优异,尤其是当任务涉及网络请求、文件下载和实时数据处理等IO操作时。例如,在Web爬虫中,利用多线程可以同时请求多个网页,从而加速数据获取过程。此外,图像处理、实时数据监控等应用也适合使用多线程来提高性能。
如何有效管理Python中的多线程?
在Python中,可以使用threading
模块来创建和管理线程。为了有效管理线程,可以使用线程池来限制同时运行的线程数量,避免资源的过度消耗。此外,使用锁机制(如Lock
或RLock
)可以确保线程在访问共享资源时的安全性,防止数据冲突。在设计多线程程序时,合理划分任务和管理线程的生命周期也是提升性能和稳定性的关键。
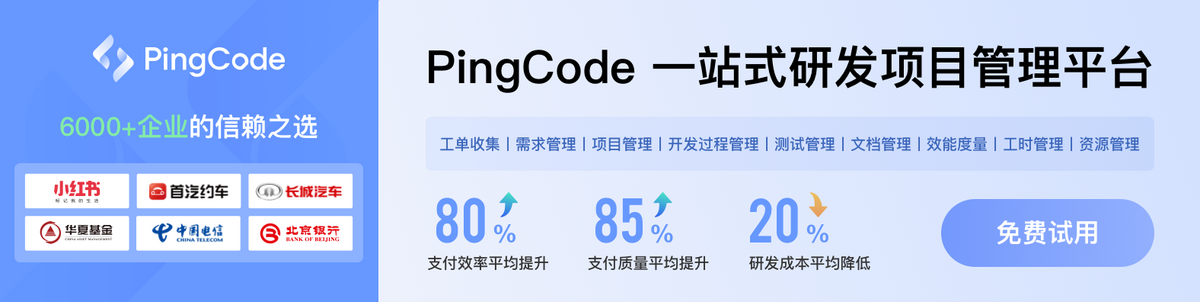