使用Python爬取天气预报需要:选择合适的数据源、安装必要的库、编写爬虫代码、处理和解析数据、存储和展示数据。我们将详细介绍如何使用Python实现这些步骤。
一、选择合适的数据源
在爬取天气预报数据之前,我们需要选择一个可靠的数据源。常见的天气预报数据源包括:OpenWeatherMap、Weather.com、AccuWeather、国家气象局等。大多数天气网站提供API接口,方便我们获取天气数据,但可能需要注册和获取API密钥。
二、安装必要的库
在编写爬虫代码之前,我们需要安装一些必要的Python库。常用的库包括requests、BeautifulSoup、pandas等。这些库可以帮助我们发送HTTP请求、解析HTML页面和处理数据。可以使用以下命令安装这些库:
pip install requests beautifulsoup4 pandas
三、编写爬虫代码
- 发送HTTP请求获取天气数据
可以使用requests库发送HTTP请求,获取天气网站的HTML页面。以OpenWeatherMap为例,获取天气数据的代码如下:
import requests
api_key = 'YOUR_API_KEY'
city = 'London'
url = f'http://api.openweathermap.org/data/2.5/weather?q={city}&appid={api_key}'
response = requests.get(url)
data = response.json()
print(data)
- 解析天气数据
获取到的数据通常是JSON格式的,我们可以使用Python内置的json库解析这些数据。以下是解析天气数据的示例代码:
import json
weather_data = json.loads(response.text)
temperature = weather_data['main']['temp']
weather_description = weather_data['weather'][0]['description']
print(f"Temperature: {temperature}")
print(f"Weather Description: {weather_description}")
四、处理和解析数据
- 使用BeautifulSoup解析HTML页面
如果你选择的天气网站没有提供API接口,你可以使用BeautifulSoup解析HTML页面,提取需要的天气数据。以下是一个示例代码,展示如何从Weather.com获取天气预报数据:
from bs4 import BeautifulSoup
url = 'https://weather.com/weather/today/l/USNY0996:1:US'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
temperature = soup.find('span', class_='CurrentConditions--tempValue--3KcTQ').text
weather_description = soup.find('div', class_='CurrentConditions--phraseValue--2xXSr').text
print(f"Temperature: {temperature}")
print(f"Weather Description: {weather_description}")
- 处理数据
获取到天气数据后,我们可以使用pandas库进行处理和分析。以下是一个示例代码,展示如何将天气数据存储到DataFrame中,并进行简单的分析:
import pandas as pd
data = {
'City': ['London', 'New York', 'Tokyo'],
'Temperature': [15, 20, 25],
'Weather Description': ['Clear', 'Cloudy', 'Rainy']
}
df = pd.DataFrame(data)
print(df)
分析天气数据
average_temperature = df['Temperature'].mean()
print(f"Average Temperature: {average_temperature}")
五、存储和展示数据
- 存储数据
我们可以将天气数据存储到CSV文件或数据库中,方便后续的分析和展示。以下是一个示例代码,展示如何将天气数据存储到CSV文件中:
df.to_csv('weather_data.csv', index=False)
- 展示数据
我们可以使用Matplotlib或其他数据可视化库展示天气数据。以下是一个示例代码,展示如何使用Matplotlib绘制温度变化图:
import matplotlib.pyplot as plt
cities = df['City']
temperatures = df['Temperature']
plt.plot(cities, temperatures, marker='o')
plt.xlabel('City')
plt.ylabel('Temperature')
plt.title('Temperature in Different Cities')
plt.show()
通过以上步骤,我们可以使用Python爬取天气预报数据,并进行处理、存储和展示。
六、定时爬取天气数据
如果我们希望定时爬取天气数据,可以使用Python的schedule库或操作系统的定时任务功能。以下是一个示例代码,展示如何使用schedule库定时爬取天气数据:
import schedule
import time
def job():
response = requests.get(url)
weather_data = json.loads(response.text)
temperature = weather_data['main']['temp']
weather_description = weather_data['weather'][0]['description']
print(f"Temperature: {temperature}")
print(f"Weather Description: {weather_description}")
schedule.every().hour.do(job)
while True:
schedule.run_pending()
time.sleep(1)
七、处理异常和错误
在编写爬虫代码时,我们需要处理可能出现的异常和错误。例如,网络连接失败、API请求次数超限、解析错误等。以下是一个示例代码,展示如何处理异常和错误:
try:
response = requests.get(url)
response.raise_for_status()
weather_data = json.loads(response.text)
temperature = weather_data['main']['temp']
weather_description = weather_data['weather'][0]['description']
print(f"Temperature: {temperature}")
print(f"Weather Description: {weather_description}")
except requests.exceptions.RequestException as e:
print(f"Error: {e}")
except json.JSONDecodeError as e:
print(f"Error decoding JSON: {e}")
通过以上步骤,我们可以使用Python爬取天气预报数据,并进行处理、存储和展示。希望这篇文章对你有所帮助。如果你有任何问题或建议,欢迎在评论区留言。
八、扩展功能
- 获取未来几天的天气预报
除了获取当前的天气数据,我们还可以获取未来几天的天气预报。以OpenWeatherMap为例,我们可以使用以下代码获取未来几天的天气预报:
forecast_url = f'http://api.openweathermap.org/data/2.5/forecast?q={city}&appid={api_key}'
forecast_response = requests.get(forecast_url)
forecast_data = json.loads(forecast_response.text)
for forecast in forecast_data['list']:
date = forecast['dt_txt']
temperature = forecast['main']['temp']
weather_description = forecast['weather'][0]['description']
print(f"Date: {date}, Temperature: {temperature}, Weather Description: {weather_description}")
- 将天气数据发送到邮箱
如果我们希望将爬取到的天气数据发送到邮箱,可以使用smtplib库。以下是一个示例代码,展示如何将天气数据发送到邮箱:
import smtplib
from email.mime.text import MIMEText
from email.mime.multipart import MIMEMultipart
def send_email(subject, body, to_email):
from_email = 'your_email@example.com'
from_password = 'your_password'
msg = MIMEMultipart()
msg['From'] = from_email
msg['To'] = to_email
msg['Subject'] = subject
msg.attach(MIMEText(body, 'plain'))
try:
server = smtplib.SMTP('smtp.example.com', 587)
server.starttls()
server.login(from_email, from_password)
text = msg.as_string()
server.sendmail(from_email, to_email, text)
server.quit()
print("Email sent successfully")
except Exception as e:
print(f"Error: {e}")
subject = "Weather Report"
body = f"Temperature: {temperature}\nWeather Description: {weather_description}"
send_email(subject, body, 'recipient@example.com')
- 创建一个Web应用展示天气数据
我们可以使用Flask或Django创建一个Web应用,展示爬取到的天气数据。以下是一个使用Flask创建Web应用的示例代码:
from flask import Flask, render_template
import requests
import json
app = Flask(__name__)
@app.route('/')
def index():
api_key = 'YOUR_API_KEY'
city = 'London'
url = f'http://api.openweathermap.org/data/2.5/weather?q={city}&appid={api_key}'
response = requests.get(url)
weather_data = json.loads(response.text)
temperature = weather_data['main']['temp']
weather_description = weather_data['weather'][0]['description']
return render_template('index.html', temperature=temperature, weather_description=weather_description)
if __name__ == '__main__':
app.run(debug=True)
在index.html文件中,我们可以展示天气数据:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Weather Report</title>
</head>
<body>
<h1>Weather Report</h1>
<p>Temperature: {{ temperature }}</p>
<p>Weather Description: {{ weather_description }}</p>
</body>
</html>
通过以上扩展功能,我们可以进一步增强天气爬虫的功能,使其更加实用和便捷。
九、优化爬虫性能
- 使用多线程
我们可以使用多线程来提高爬虫的性能。以下是一个示例代码,展示如何使用多线程爬取多个城市的天气数据:
import threading
def fetch_weather(city):
url = f'http://api.openweathermap.org/data/2.5/weather?q={city}&appid={api_key}'
response = requests.get(url)
weather_data = json.loads(response.text)
temperature = weather_data['main']['temp']
weather_description = weather_data['weather'][0]['description']
print(f"City: {city}, Temperature: {temperature}, Weather Description: {weather_description}")
cities = ['London', 'New York', 'Tokyo']
threads = []
for city in cities:
thread = threading.Thread(target=fetch_weather, args=(city,))
threads.append(thread)
thread.start()
for thread in threads:
thread.join()
- 使用代理
在爬取大量数据时,我们可以使用代理来避免IP被封。以下是一个示例代码,展示如何使用代理爬取天气数据:
proxies = {
'http': 'http://your_proxy:port',
'https': 'https://your_proxy:port'
}
response = requests.get(url, proxies=proxies)
weather_data = json.loads(response.text)
temperature = weather_data['main']['temp']
weather_description = weather_data['weather'][0]['description']
print(f"Temperature: {temperature}")
print(f"Weather Description: {weather_description}")
通过以上优化措施,我们可以提高爬虫的性能,使其更加高效和稳定。
总结
使用Python爬取天气预报数据是一个非常实用的技能,可以帮助我们获取最新的天气信息,并进行处理、存储和展示。本文详细介绍了如何选择合适的数据源、安装必要的库、编写爬虫代码、处理和解析数据、存储和展示数据,并提供了一些扩展功能和优化措施。希望这篇文章对你有所帮助。如果你有任何问题或建议,欢迎在评论区留言。
相关问答FAQs:
如何选择合适的天气数据源进行爬取?
选择合适的天气数据源是成功爬取天气预报的关键。常用的数据源包括天气网站、API接口以及政府气象局网站。应优先考虑提供开放API的服务,这样可以减少对网页结构变化的依赖。确保所选数据源的更新频率和数据准确性符合你的需求,查看相关的使用条款也是很重要的。
使用Python爬取天气预报需要哪些库?
在Python中,常用的库有requests
用于发送HTTP请求,BeautifulSoup
或lxml
用于解析HTML文档,pandas
则可以用来处理和分析数据。对于需要频繁访问API的情况,可以使用json
库来处理返回的JSON数据。此外,time
库可以帮助设置请求间隔,避免过于频繁的访问导致IP被封禁。
如何处理爬取到的数据以便于后续使用?
处理爬取到的天气数据可以使用pandas
库将数据存储为DataFrame格式,方便进行数据分析和可视化。可以将数据导出为CSV或Excel文件,便于后续使用。同时,可以利用Matplotlib或Seaborn等库进行可视化,帮助更直观地理解天气趋势和变化。此外,考虑将数据存入数据库(如SQLite或MySQL)以便长期存储和查询。
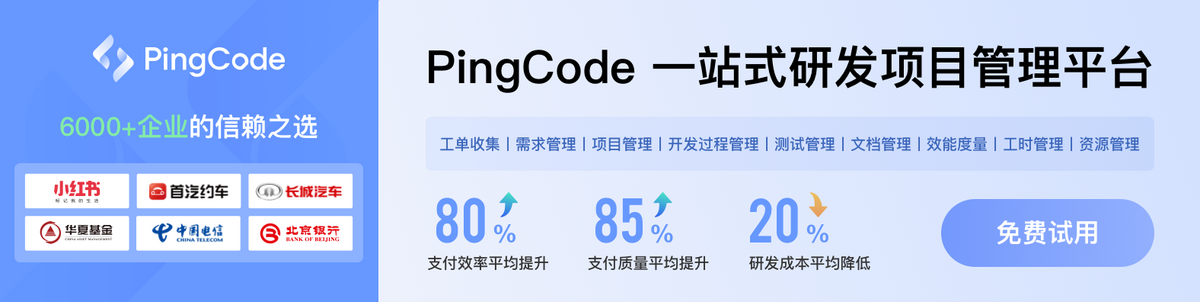