在Python中避免使用多个if语句的方法包括使用字典映射、面向对象编程、策略模式、函数装饰器。
其中,使用字典映射是一种非常实用且高效的方式。通过将关键字与对应的处理函数绑定在一起,可以用更简洁的代码实现相同的功能。举个简单的例子:
def handle_a():
return "Handle A"
def handle_b():
return "Handle B"
def handle_c():
return "Handle C"
handlers = {
'a': handle_a,
'b': handle_b,
'c': handle_c
}
def handle_key(key):
if key in handlers:
return handlers[key]()
else:
return "Invalid key"
print(handle_key('a')) # Output: Handle A
print(handle_key('b')) # Output: Handle B
print(handle_key('d')) # Output: Invalid key
这种方法不仅使代码更加简洁明了,还能方便地扩展处理逻辑。接下来,我们将详细讨论Python避免多个if语句的其他方法。
一、字典映射
字典映射是一种常见的避免多个if语句的方法,它通过将键值对映射到相应的处理函数或值来实现。
1. 使用函数映射
如上文所示,通过将函数与关键字绑定在一起,可以用字典来替代多个if语句。进一步举例:
def process_apple():
return "Processing Apple"
def process_banana():
return "Processing Banana"
def process_cherry():
return "Processing Cherry"
fruit_handlers = {
'apple': process_apple,
'banana': process_banana,
'cherry': process_cherry
}
def handle_fruit(fruit):
return fruit_handlers.get(fruit, lambda: "Unknown fruit")()
print(handle_fruit('apple')) # Output: Processing Apple
print(handle_fruit('banana')) # Output: Processing Banana
print(handle_fruit('durian')) # Output: Unknown fruit
2. 使用值映射
有时候,我们只需要根据不同的键返回不同的值,此时也可以使用字典映射:
fruit_colors = {
'apple': 'red',
'banana': 'yellow',
'cherry': 'red'
}
def get_fruit_color(fruit):
return fruit_colors.get(fruit, 'unknown')
print(get_fruit_color('apple')) # Output: red
print(get_fruit_color('banana')) # Output: yellow
print(get_fruit_color('durian')) # Output: unknown
这种方法简单直接,适用于各种情况。
二、面向对象编程
面向对象编程(OOP)是另一种避免多个if语句的有效方法。通过将不同的行为封装在不同的类中,可以更好地组织代码,增强可维护性和可扩展性。
1. 基本用法
假设我们有一个动物园程序,不同的动物有不同的叫声:
class Animal:
def make_sound(self):
raise NotImplementedError("Subclass must implement abstract method")
class Dog(Animal):
def make_sound(self):
return "Woof"
class Cat(Animal):
def make_sound(self):
return "Meow"
class Cow(Animal):
def make_sound(self):
return "Moo"
def get_animal_sound(animal):
return animal.make_sound()
dog = Dog()
cat = Cat()
cow = Cow()
print(get_animal_sound(dog)) # Output: Woof
print(get_animal_sound(cat)) # Output: Meow
print(get_animal_sound(cow)) # Output: Moo
2. 工厂模式
为了更好地管理对象的创建,可以使用工厂模式:
class AnimalFactory:
@staticmethod
def create_animal(animal_type):
if animal_type == 'dog':
return Dog()
elif animal_type == 'cat':
return Cat()
elif animal_type == 'cow':
return Cow()
else:
raise ValueError(f"Unknown animal type: {animal_type}")
factory = AnimalFactory()
animal = factory.create_animal('dog')
print(get_animal_sound(animal)) # Output: Woof
这种方法将对象创建逻辑集中在一个地方,便于管理和扩展。
三、策略模式
策略模式是一种行为设计模式,它定义了一系列算法,并将每种算法封装起来,使得它们可以互换使用。这种模式适用于需要在运行时选择算法的情况。
1. 基本用法
假设我们有一个支付系统,不同的支付方式有不同的处理逻辑:
class PaymentStrategy:
def pay(self, amount):
raise NotImplementedError("Subclass must implement abstract method")
class CreditCardPayment(PaymentStrategy):
def pay(self, amount):
return f"Paying {amount} using Credit Card"
class PayPalPayment(PaymentStrategy):
def pay(self, amount):
return f"Paying {amount} using PayPal"
class BitcoinPayment(PaymentStrategy):
def pay(self, amount):
return f"Paying {amount} using Bitcoin"
def process_payment(strategy, amount):
return strategy.pay(amount)
credit_card = CreditCardPayment()
paypal = PayPalPayment()
bitcoin = BitcoinPayment()
print(process_payment(credit_card, 100)) # Output: Paying 100 using Credit Card
print(process_payment(paypal, 200)) # Output: Paying 200 using PayPal
print(process_payment(bitcoin, 300)) # Output: Paying 300 using Bitcoin
2. 动态选择策略
可以根据用户的选择动态选择策略:
payment_strategies = {
'credit_card': CreditCardPayment,
'paypal': PayPalPayment,
'bitcoin': BitcoinPayment
}
def get_payment_strategy(strategy_type):
strategy_class = payment_strategies.get(strategy_type)
if strategy_class:
return strategy_class()
else:
raise ValueError(f"Unknown strategy type: {strategy_type}")
strategy = get_payment_strategy('paypal')
print(process_payment(strategy, 250)) # Output: Paying 250 using PayPal
这种方式不仅简化了代码,还能根据需求轻松扩展新的支付策略。
四、函数装饰器
函数装饰器是一种高级特性,它允许在不改变函数定义的情况下扩展函数的行为。装饰器可以有效地避免多个if语句。
1. 基本用法
假设我们有一组函数,需要在调用前后记录日志:
def log_decorator(func):
def wrapper(*args, kwargs):
print(f"Calling {func.__name__}")
result = func(*args, kwargs)
print(f"{func.__name__} returned {result}")
return result
return wrapper
@log_decorator
def add(a, b):
return a + b
@log_decorator
def multiply(a, b):
return a * b
print(add(2, 3)) # Output: Calling add / add returned 5 / 5
print(multiply(2, 3)) # Output: Calling multiply / multiply returned 6 / 6
2. 参数化装饰器
装饰器还可以接受参数,从而实现更灵活的功能:
def log_decorator(level):
def decorator(func):
def wrapper(*args, kwargs):
print(f"{level}: Calling {func.__name__}")
result = func(*args, kwargs)
print(f"{level}: {func.__name__} returned {result}")
return result
return wrapper
return decorator
@log_decorator(level='INFO')
def subtract(a, b):
return a - b
@log_decorator(level='DEBUG')
def divide(a, b):
return a / b
print(subtract(10, 5)) # Output: INFO: Calling subtract / INFO: subtract returned 5 / 5
print(divide(10, 2)) # Output: DEBUG: Calling divide / DEBUG: divide returned 5.0 / 5.0
通过使用装饰器,可以有效地管理函数的额外行为,避免重复代码和多个if语句。
五、其他方法
除了上述方法,还有一些其他技术可以帮助我们避免多个if语句。
1. 使用列表和元组
在某些情况下,可以使用列表或元组来简化代码:
operations = [
("apple", lambda: "Processing Apple"),
("banana", lambda: "Processing Banana"),
("cherry", lambda: "Processing Cherry")
]
def process_fruit(fruit):
for name, handler in operations:
if name == fruit:
return handler()
return "Unknown fruit"
print(process_fruit("apple")) # Output: Processing Apple
print(process_fruit("banana")) # Output: Processing Banana
print(process_fruit("durian")) # Output: Unknown fruit
2. 使用枚举
枚举类型可以帮助我们更清晰地表达不同的选项:
from enum import Enum, auto
class Fruit(Enum):
APPLE = auto()
BANANA = auto()
CHERRY = auto()
def process_fruit(fruit):
if fruit == Fruit.APPLE:
return "Processing Apple"
elif fruit == Fruit.BANANA:
return "Processing Banana"
elif fruit == Fruit.CHERRY:
return "Processing Cherry"
else:
return "Unknown fruit"
print(process_fruit(Fruit.APPLE)) # Output: Processing Apple
print(process_fruit(Fruit.BANANA)) # Output: Processing Banana
print(process_fruit(Fruit.CHERRY)) # Output: Processing Cherry
使用枚举类型可以让代码更具可读性和可维护性。
综上所述,避免多个if语句的方法有很多,包括字典映射、面向对象编程、策略模式、函数装饰器、以及其他技术。通过选择适合的方法,可以使代码更加简洁、易于维护和扩展。在实际开发中,根据具体需求选择合适的技术方案,将大大提高代码的质量和效率。
相关问答FAQs:
如何在Python中使用其他控制结构来替代多个if语句?
在Python中,可以使用多种控制结构来简化代码并避免多个if语句。例如,可以使用elif
来处理多个条件,或者使用字典映射来替代多个if-else判断。此外,列表推导式和生成器表达式也可以在某些情况下提供更简洁的解决方案。
有没有推荐的设计模式可以减少if语句的使用?
有些设计模式,比如策略模式和状态模式,可以有效地减少if语句的数量。通过将不同的行为封装在类中,可以根据条件动态选择合适的策略,从而使代码更加清晰和可维护。
在Python中,如何使用逻辑运算符来简化if条件?
逻辑运算符(如and
、or
和not
)可以帮助将多个条件组合在一起,从而减少if语句的数量。例如,可以通过将多个条件组合成一个复合条件,使用一个if语句来判断多个情况,从而简化代码的结构。
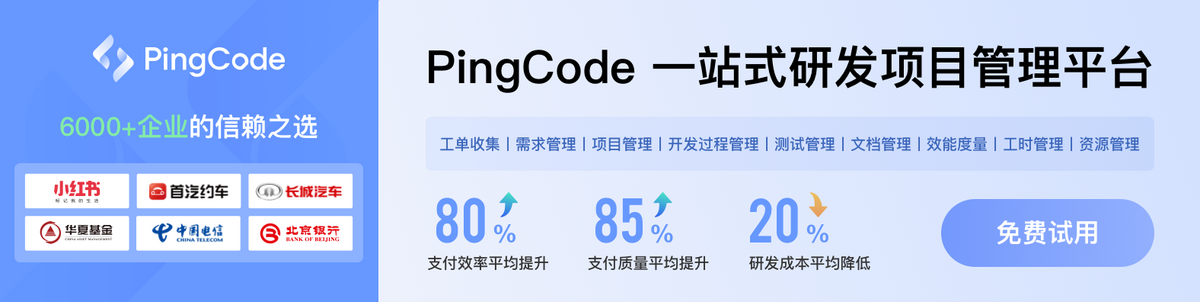