用Python优化SEO,可以通过关键词研究、内容优化、技术SEO分析、网站性能优化、自动化报告生成等手段。其中,关键词研究是非常重要的一步,因为它直接影响到内容的定位和用户搜索需求的满足。通过Python,可以使用诸如Google Trends API、Ahrefs API等工具来抓取、分析关键词数据,帮助确定高价值关键词,并指导内容创作。
关键词研究可以通过以下步骤详细展开:
- 确定目标关键词:使用Python脚本抓取相关行业的热门关键词,例如使用Google Trends API获取关键词的搜索量趋势。
- 竞争对手分析:分析竞争对手的网站,抓取他们使用的关键词,了解他们的SEO策略。
- 长尾关键词挖掘:利用Python的网络爬虫工具,如BeautifulSoup、Scrapy等,挖掘长尾关键词。
- 关键词效果分析:通过API或者爬虫抓取关键词的搜索量、点击率等数据,分析关键词的实际效果。
接下来,我们将逐步探讨如何用Python优化SEO的各个方面。
一、关键词研究
1. 确定目标关键词
目标关键词是SEO优化的核心。通过确定目标关键词,可以指导内容创作和优化策略。使用Python可以方便地从各种数据源获取关键词信息。
使用Google Trends API
Google Trends API可以提供关键词的搜索量趋势数据。以下是一个简单的Python示例:
from pytrends.request import TrendReq
初始化Google Trends API
pytrends = TrendReq(hl='en-US', tz=360)
搜索关键词
kw_list = ["Python SEO"]
pytrends.build_payload(kw_list, cat=0, timeframe='today 12-m')
获取趋势数据
trend_data = pytrends.interest_over_time()
print(trend_data)
通过上述代码,可以获取关键词在过去12个月内的搜索趋势数据。这些数据可以帮助我们确定哪些关键词是热门的,从而指导内容创作。
使用Ahrefs API
Ahrefs是一个强大的SEO工具,提供了丰富的关键词数据。通过其API,可以获取关键词的搜索量、难度、点击率等数据。
import requests
Ahrefs API凭证
api_key = "your_ahrefs_api_key"
target = "your_target_keyword"
发送请求
response = requests.get(f"https://apiv2.ahrefs.com?token={api_key}&from=keywords_explorer&target={target}&mode=exact")
data = response.json()
分析数据
print(data)
通过Ahrefs API,可以获取目标关键词的详细数据,帮助我们更好地进行关键词分析和优化。
2. 竞争对手分析
了解竞争对手的SEO策略是优化自身网站的重要手段。通过Python,可以自动化地抓取竞争对手网站的信息,分析他们使用的关键词。
使用BeautifulSoup抓取竞争对手网站
BeautifulSoup是一个Python库,用于从网页抓取数据。以下是一个简单的示例,展示如何抓取竞争对手网站的标题和关键词。
from bs4 import BeautifulSoup
import requests
获取网页内容
url = "https://www.competitor.com"
response = requests.get(url)
soup = BeautifulSoup(response.content, 'html.parser')
提取标题和关键词
title = soup.title.string
keywords = soup.find("meta", attrs={"name": "keywords"})["content"]
print(f"Title: {title}")
print(f"Keywords: {keywords}")
通过这种方式,可以批量抓取竞争对手网站的关键词,了解他们的SEO策略。
3. 长尾关键词挖掘
长尾关键词通常具有较低的搜索量和竞争度,但可以带来高质量的流量。使用Python的网络爬虫工具,可以自动化地挖掘长尾关键词。
使用Scrapy爬取长尾关键词
Scrapy是一个强大的Python爬虫框架,适用于大规模的数据抓取。以下是一个简单的Scrapy示例,展示如何爬取长尾关键词。
import scrapy
class KeywordSpider(scrapy.Spider):
name = "keyword_spider"
start_urls = ["https://www.example.com"]
def parse(self, response):
for keyword in response.css('div.keyword'):
yield {
'keyword': keyword.css('a::text').get(),
'search_volume': keyword.css('span.search_volume::text').get(),
}
运行爬虫
from scrapy.crawler import CrawlerProcess
process = CrawlerProcess()
process.crawl(KeywordSpider)
process.start()
通过Scrapy,可以自动化地从目标网站爬取长尾关键词,帮助我们发现更多的关键词机会。
4. 关键词效果分析
关键词效果分析是评估SEO策略效果的重要步骤。通过抓取关键词的搜索量、点击率等数据,可以分析关键词的实际效果。
使用Python抓取关键词效果数据
以下是一个使用requests库抓取关键词效果数据的示例:
import requests
发送请求
response = requests.get("https://api.example.com/keywords/effect?keyword=python+seo")
data = response.json()
分析数据
search_volume = data["search_volume"]
click_rate = data["click_rate"]
print(f"Search Volume: {search_volume}")
print(f"Click Rate: {click_rate}")
通过这种方式,可以获取关键词的搜索量和点击率等数据,评估关键词的实际效果。
二、内容优化
内容优化是SEO优化的核心,通过优化内容,可以提高网站的搜索引擎排名。使用Python,可以自动化地进行内容优化,提高效率。
1. 内容结构优化
内容结构优化是指通过调整内容的结构,使其更符合搜索引擎的要求。使用Python,可以自动化地分析和优化内容结构。
使用Python分析内容结构
以下是一个使用Python分析内容结构的示例:
from bs4 import BeautifulSoup
import requests
获取网页内容
url = "https://www.example.com/article"
response = requests.get(url)
soup = BeautifulSoup(response.content, 'html.parser')
分析标题和段落
titles = [h1.get_text() for h1 in soup.find_all('h1')]
paragraphs = [p.get_text() for p in soup.find_all('p')]
print(f"Titles: {titles}")
print(f"Paragraphs: {paragraphs}")
通过这种方式,可以自动化地分析网页内容的结构,找出优化的方向。
2. 关键词密度优化
关键词密度是指关键词在内容中出现的频率。合理的关键词密度可以提高内容的相关性,从而提高搜索引擎排名。使用Python,可以自动化地计算和优化关键词密度。
使用Python计算关键词密度
以下是一个使用Python计算关键词密度的示例:
from collections import Counter
def calculate_keyword_density(content, keyword):
words = content.split()
word_count = len(words)
keyword_count = Counter(words)[keyword]
return keyword_count / word_count
示例内容
content = "Python SEO is important for optimizing your website. Learn how to use Python for SEO."
计算关键词密度
keyword_density = calculate_keyword_density(content, "Python")
print(f"Keyword Density: {keyword_density:.2%}")
通过这种方式,可以自动化地计算关键词密度,并根据结果进行优化。
3. 内部链接优化
内部链接优化是通过合理设置内部链接,提高网站的爬取效率和用户体验。使用Python,可以自动化地分析和优化内部链接。
使用Python分析内部链接
以下是一个使用Python分析内部链接的示例:
from bs4 import BeautifulSoup
import requests
获取网页内容
url = "https://www.example.com"
response = requests.get(url)
soup = BeautifulSoup(response.content, 'html.parser')
提取内部链接
internal_links = [a['href'] for a in soup.find_all('a', href=True) if a['href'].startswith('/')]
print(f"Internal Links: {internal_links}")
通过这种方式,可以自动化地提取和分析内部链接,找出优化的方向。
三、技术SEO分析
技术SEO分析是通过检测和优化网站的技术细节,提高搜索引擎的爬取效率和用户体验。使用Python,可以自动化地进行技术SEO分析,提高效率。
1. 网站抓取和索引分析
网站抓取和索引分析是评估搜索引擎对网站抓取和索引情况的重要手段。使用Python,可以自动化地进行抓取和索引分析。
使用Scrapy进行网站抓取
以下是一个使用Scrapy进行网站抓取的示例:
import scrapy
class SiteSpider(scrapy.Spider):
name = "site_spider"
start_urls = ["https://www.example.com"]
def parse(self, response):
for link in response.css('a::attr(href)').getall():
yield scrapy.Request(response.urljoin(link), self.parse)
运行爬虫
from scrapy.crawler import CrawlerProcess
process = CrawlerProcess()
process.crawl(SiteSpider)
process.start()
通过这种方式,可以自动化地抓取网站的所有页面,分析抓取和索引情况。
使用Python分析索引情况
以下是一个使用Python分析索引情况的示例:
import requests
def check_index_status(url):
response = requests.get(f"https://www.google.com/search?q=site:{url}")
return "No results found" not in response.text
检查索引情况
url = "https://www.example.com"
is_indexed = check_index_status(url)
print(f"Is Indexed: {is_indexed}")
通过这种方式,可以自动化地检查网站的索引情况,找出未被索引的页面。
2. 网站速度和性能优化
网站速度和性能是影响用户体验和搜索引擎排名的重要因素。使用Python,可以自动化地进行网站速度和性能优化。
使用Python测量网站速度
以下是一个使用Python测量网站速度的示例:
import requests
import time
def measure_speed(url):
start_time = time.time()
response = requests.get(url)
end_time = time.time()
return end_time - start_time
测量网站速度
url = "https://www.example.com"
speed = measure_speed(url)
print(f"Website Speed: {speed:.2f} seconds")
通过这种方式,可以自动化地测量网站速度,并根据结果进行优化。
使用Python分析页面性能
以下是一个使用Python分析页面性能的示例:
from selenium import webdriver
def analyze_performance(url):
driver = webdriver.Chrome()
driver.get(url)
performance = driver.execute_script("return window.performance.timing")
driver.quit()
return performance
分析页面性能
url = "https://www.example.com"
performance = analyze_performance(url)
print(f"Performance: {performance}")
通过这种方式,可以自动化地分析页面性能,找出影响性能的问题。
四、网站性能优化
网站性能优化是通过优化网站的各种性能指标,提高用户体验和搜索引擎排名。使用Python,可以自动化地进行网站性能优化,提高效率。
1. 图片优化
图片优化是通过压缩和调整图片,提高网站加载速度和用户体验。使用Python,可以自动化地进行图片优化。
使用Pillow进行图片优化
以下是一个使用Pillow进行图片优化的示例:
from PIL import Image
def optimize_image(input_path, output_path):
with Image.open(input_path) as img:
img.save(output_path, optimize=True, quality=85)
优化图片
input_path = "path/to/input/image.jpg"
output_path = "path/to/output/image.jpg"
optimize_image(input_path, output_path)
print(f"Image optimized and saved to {output_path}")
通过这种方式,可以自动化地压缩和优化图片,提高网站加载速度。
2. 缓存优化
缓存优化是通过设置合理的缓存策略,提高网站的加载速度和用户体验。使用Python,可以自动化地进行缓存优化。
使用Flask设置缓存
以下是一个使用Flask设置缓存的示例:
from flask import Flask, make_response
app = Flask(__name__)
@app.route('/')
def index():
response = make_response("Hello, World!")
response.cache_control.max_age = 3600
return response
if __name__ == '__main__':
app.run()
通过这种方式,可以自动化地设置缓存策略,提高网站加载速度。
五、自动化报告生成
自动化报告生成是通过生成SEO报告,评估和优化SEO策略的重要手段。使用Python,可以自动化地生成SEO报告,提高效率。
1. 数据抓取和整理
数据抓取和整理是生成SEO报告的基础。使用Python,可以自动化地抓取和整理SEO数据。
使用BeautifulSoup抓取数据
以下是一个使用BeautifulSoup抓取数据的示例:
from bs4 import BeautifulSoup
import requests
def fetch_data(url):
response = requests.get(url)
soup = BeautifulSoup(response.content, 'html.parser')
titles = [h1.get_text() for h1 in soup.find_all('h1')]
return titles
抓取数据
url = "https://www.example.com"
data = fetch_data(url)
print(f"Fetched Data: {data}")
通过这种方式,可以自动化地抓取和整理SEO数据,为生成报告做准备。
2. 生成SEO报告
生成SEO报告是评估和优化SEO策略的重要手段。使用Python,可以自动化地生成SEO报告。
使用Jinja2生成HTML报告
以下是一个使用Jinja2生成HTML报告的示例:
from jinja2 import Template
def generate_report(data, output_path):
template = Template("""
<html>
<body>
<h1>SEO Report</h1>
<ul>
{% for item in data %}
<li>{{ item }}</li>
{% endfor %}
</ul>
</body>
</html>
""")
report = template.render(data=data)
with open(output_path, 'w') as f:
f.write(report)
生成报告
data = ["Title 1", "Title 2", "Title 3"]
output_path = "path/to/output/report.html"
generate_report(data, output_path)
print(f"Report generated and saved to {output_path}")
通过这种方式,可以自动化地生成SEO报告,评估和优化SEO策略。
六、总结
通过使用Python优化SEO,可以大大提高工作效率和优化效果。本文介绍了如何用Python进行关键词研究、内容优化、技术SEO分析、网站性能优化和自动化报告生成。希望这些方法和示例能够帮助你更好地优化SEO,提高网站的搜索引擎排名和用户体验。
相关问答FAQs:
如何使用Python分析网站的SEO表现?
Python可以通过多种库来分析网站的SEO表现。例如,使用Beautiful Soup和Requests库可以抓取网页内容,分析标题、描述和关键词的使用情况。利用Pandas库整理数据,并使用Matplotlib或Seaborn可视化SEO数据,帮助识别潜在问题和改进机会。
有哪些Python库可以帮助进行关键词研究?
在关键词研究方面,使用Python的Scrapy库可以高效地抓取搜索引擎结果页面,分析不同关键词的排名情况。结合Google Trends API或Ahrefs API,使用Python进行数据获取和分析,可以帮助用户识别流行的关键词和搜索趋势,从而优化内容策略。
如何利用Python进行竞争对手分析以提升SEO?
通过Python,用户可以编写脚本抓取竞争对手的网站信息,分析其页面结构、关键词布局和外链情况。使用Requests和Beautiful Soup进行数据采集后,结合数据分析工具,如Pandas,可以深入了解竞争对手的SEO策略,从而制定相应的优化措施,提高自身网站的排名。
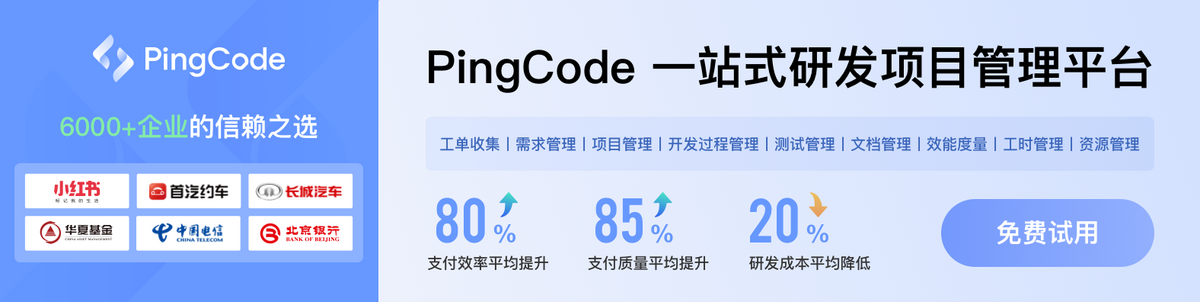