Python可以通过使用第三方库、调用API、使用自动化工具等方式来实现自动发送消息。 其中,常用的方法包括使用smtplib
库发送电子邮件、使用twilio
库发送短信、以及通过调用社交媒体平台的API发送消息。接下来我将详细介绍如何使用twilio
库发送短信。
twilio
是一个广泛使用的第三方库,允许开发者通过其API发送短信、进行语音通话等。首先,需要在Twilio网站上注册一个账号,并获取自己的Account SID
和Auth Token
。然后,可以通过安装twilio库并编写简单的Python代码来发送短信。
以下是一个简单的示例代码:
from twilio.rest import Client
Your Account SID from twilio.com/console
account_sid = "your_account_sid"
Your Auth Token from twilio.com/console
auth_token = "your_auth_token"
client = Client(account_sid, auth_token)
message = client.messages.create(
to="+1234567890",
from_="+0987654321",
body="Hello from Python!")
print(message.sid)
在这个示例中,首先导入Client
类,然后使用account_sid
和auth_token
创建一个Client
对象,接着调用messages.create()
方法,传入目标电话号码、发送电话号码和消息内容,即可发送短信。
一、使用smtplib
库发送电子邮件
配置SMTP服务器
Python的smtplib
库提供了一个简单的接口,用于发送电子邮件。首先需要配置SMTP服务器,常见的SMTP服务器包括Gmail、Outlook等。以下是配置Gmail SMTP服务器的示例代码:
import smtplib
from email.mime.text import MIMEText
from email.mime.multipart import MIMEMultipart
smtp_server = "smtp.gmail.com"
smtp_port = 587
sender_email = "your_email@gmail.com"
password = "your_password"
Create the email content
message = MIMEMultipart()
message["From"] = sender_email
message["To"] = "recipient_email@gmail.com"
message["Subject"] = "Test Email"
body = "This is a test email sent from Python."
message.attach(MIMEText(body, "plain"))
Connect to the SMTP server and send email
with smtplib.SMTP(smtp_server, smtp_port) as server:
server.starttls() # Secure the connection
server.login(sender_email, password)
server.sendmail(sender_email, "recipient_email@gmail.com", message.as_string())
发送HTML格式的电子邮件
如果你想发送HTML格式的电子邮件,可以使用MIMEText
类并指定subtype
为html
:
html_body = """
<html>
<body>
<h1>Hello</h1>
<p>This is a <b>test email</b> sent from Python.</p>
</body>
</html>
"""
message.attach(MIMEText(html_body, "html"))
这样,接收方将会看到HTML格式的内容,而不仅仅是纯文本。
二、使用twilio
库发送短信
安装twilio
库
首先需要安装twilio
库,可以使用以下命令通过pip
进行安装:
pip install twilio
获取Twilio账户信息
在Twilio网站注册并登录后,可以在控制台中找到Account SID
和Auth Token
。这些信息将用于验证和授权。
发送短信的示例代码
下面是一个使用twilio
库发送短信的完整示例:
from twilio.rest import Client
Your Account SID from twilio.com/console
account_sid = "your_account_sid"
Your Auth Token from twilio.com/console
auth_token = "your_auth_token"
client = Client(account_sid, auth_token)
message = client.messages.create(
to="+1234567890",
from_="+0987654321",
body="Hello from Python!")
print(message.sid)
处理发送结果和错误
可以在发送短信后处理结果和捕获可能的异常,以确保程序的稳定性:
try:
message = client.messages.create(
to="+1234567890",
from_="+0987654321",
body="Hello from Python!")
print(f"Message sent successfully. SID: {message.sid}")
except Exception as e:
print(f"Failed to send message: {e}")
三、使用requests
库调用API发送消息
调用Slack API发送消息
通过requests
库调用Slack API发送消息也是一种常见的方法。首先需要在Slack中创建一个应用并获取OAuth令牌。以下是一个示例代码:
import requests
import json
url = "https://slack.com/api/chat.postMessage"
token = "your_slack_token"
channel = "#general"
text = "Hello from Python!"
headers = {
"Content-Type": "application/json",
"Authorization": f"Bearer {token}"
}
payload = {
"channel": channel,
"text": text
}
response = requests.post(url, headers=headers, data=json.dumps(payload))
if response.status_code == 200:
print("Message sent successfully.")
else:
print(f"Failed to send message: {response.text}")
调用Telegram Bot API发送消息
同样地,可以通过调用Telegram Bot API发送消息。首先需要在Telegram中创建一个Bot并获取Bot Token。以下是一个示例代码:
import requests
bot_token = "your_bot_token"
chat_id = "your_chat_id"
text = "Hello from Python!"
url = f"https://api.telegram.org/bot{bot_token}/sendMessage"
payload = {
"chat_id": chat_id,
"text": text
}
response = requests.post(url, data=payload)
if response.status_code == 200:
print("Message sent successfully.")
else:
print(f"Failed to send message: {response.text}")
四、使用自动化工具
Selenium自动化工具
Selenium是一个强大的自动化工具,可以用于自动化网页操作,如发送消息、填写表单等。以下是一个使用Selenium发送Facebook消息的示例:
from selenium import webdriver
from selenium.webdriver.common.keys import Keys
import time
Set up the webdriver
driver = webdriver.Chrome(executable_path="path_to_chromedriver")
Open Facebook and log in
driver.get("https://www.facebook.com")
email_elem = driver.find_element_by_id("email")
email_elem.send_keys("your_email")
password_elem = driver.find_element_by_id("pass")
password_elem.send_keys("your_password")
password_elem.send_keys(Keys.RETURN)
Wait for the page to load
time.sleep(5)
Open Messenger and send a message
driver.get("https://www.facebook.com/messages/t/recipient_username")
message_box = driver.find_element_by_css_selector("div[contenteditable='true']")
message_box.send_keys("Hello from Python!")
message_box.send_keys(Keys.RETURN)
Close the browser
time.sleep(2)
driver.quit()
使用PyAutoGUI进行桌面自动化
PyAutoGUI是一个用于进行桌面自动化的库,可以模拟鼠标和键盘操作。以下是一个使用PyAutoGUI发送消息的示例:
import pyautogui
import time
Open the messaging application (e.g., WhatsApp Web)
pyautogui.hotkey('ctrl', 't') # Open a new tab in the browser
pyautogui.typewrite('https://web.whatsapp.com')
pyautogui.press('enter')
Wait for the page to load and the user to scan the QR code
time.sleep(15)
Find the chat and send a message
pyautogui.typewrite('recipient_name')
pyautogui.press('enter')
time.sleep(2)
pyautogui.typewrite('Hello from Python!')
pyautogui.press('enter')
五、综合应用与扩展
综合应用示例:发送定时消息
可以结合多种方法,创建一个定时发送消息的程序。例如,使用smtplib
库发送定时电子邮件:
import smtplib
from email.mime.text import MIMEText
from email.mime.multipart import MIMEMultipart
import time
from datetime import datetime
def send_email():
smtp_server = "smtp.gmail.com"
smtp_port = 587
sender_email = "your_email@gmail.com"
password = "your_password"
message = MIMEMultipart()
message["From"] = sender_email
message["To"] = "recipient_email@gmail.com"
message["Subject"] = "Scheduled Email"
body = "This is a scheduled email sent from Python."
message.attach(MIMEText(body, "plain"))
with smtplib.SMTP(smtp_server, smtp_port) as server:
server.starttls()
server.login(sender_email, password)
server.sendmail(sender_email, "recipient_email@gmail.com", message.as_string())
def schedule_email(send_time):
while True:
current_time = datetime.now().strftime("%H:%M:%S")
if current_time == send_time:
send_email()
break
time.sleep(1)
Schedule the email to be sent at 14:30:00
schedule_email("14:30:00")
扩展:结合数据库和消息队列
对于更复杂的应用,可以结合数据库和消息队列来管理和发送大量消息。例如,使用MySQL数据库存储待发送的消息,并使用RabbitMQ消息队列来处理消息的发送。
import mysql.connector
import pika
import json
def send_message(message):
# Implement message sending logic (e.g., using smtplib or twilio)
pass
def callback(ch, method, properties, body):
message = json.loads(body)
send_message(message)
ch.basic_ack(delivery_tag=method.delivery_tag)
def main():
# Connect to the database
db = mysql.connector.connect(
host="localhost",
user="user",
password="password",
database="messages_db"
)
cursor = db.cursor(dictionary=True)
cursor.execute("SELECT * FROM messages WHERE status='pending'")
messages = cursor.fetchall()
# Connect to RabbitMQ
connection = pika.BlockingConnection(pika.ConnectionParameters('localhost'))
channel = connection.channel()
channel.queue_declare(queue='message_queue')
for message in messages:
channel.basic_publish(exchange='', routing_key='message_queue', body=json.dumps(message))
cursor.execute("UPDATE messages SET status='queued' WHERE id=%s", (message['id'],))
db.commit()
channel.basic_consume(queue='message_queue', on_message_callback=callback)
channel.start_consuming()
if __name__ == "__main__":
main()
安全性和隐私保护
在处理消息发送时,安全性和隐私保护是非常重要的。以下是一些建议:
- 保护敏感信息:避免在代码中硬编码敏感信息,如密码、API密钥等。可以使用环境变量或配置文件存储这些信息。
- 加密:在发送消息时,使用加密技术保护数据的传输。例如,使用TLS/SSL加密电子邮件的发送。
- 认证和授权:确保只有授权用户能够发送消息,避免滥用消息发送功能。
- 日志记录和监控:记录消息发送的日志,并进行监控,及时发现和处理异常情况。
通过以上方法和建议,可以使用Python实现自动发送消息,并确保消息发送的安全性和可靠性。
相关问答FAQs:
如何使用Python发送自动化消息?
Python提供了多种库和工具来实现自动发送消息的功能。最常见的方法是使用smtplib
库来通过电子邮件发送消息,或者使用像requests
和json
这样的库与API接口进行交互。例如,您可以通过调用Telegram、Slack或WhatsApp的API来发送消息。具体步骤包括安装相应库、设置API密钥、构建消息体和发送请求。
在Python中,发送消息需要哪些权限或设置?
发送消息的权限和设置通常取决于您选择的通信平台。对于电子邮件,您需要确保SMTP服务器的设置正确,并可能需要启用"低安全性应用程序"访问权限。对于社交媒体平台,您需要获取API密钥和相关权限,以确保可以通过编程方式发送消息。建议查看相关平台的开发者文档,以获得详细的权限要求。
如何调试Python自动发送消息的代码?
调试代码时,您可以使用Python内置的logging
模块来记录发送请求的详细信息。捕获异常并打印错误信息也是一个有效的方法。使用print()
语句可以帮助您跟踪每一步的执行情况。此外,使用测试账号或开发环境进行测试,确保在实际发送之前验证所有功能是否正常工作。
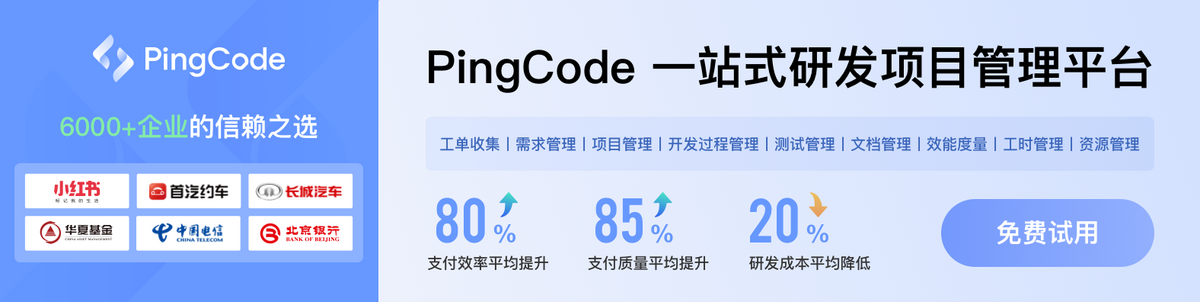