Python实现后台操作的方法主要有:多线程、多进程、协程、守护进程。 其中,多线程和多进程适用于需要并发执行的任务,而协程则适用于需要异步执行的任务。守护进程适用于需要在后台长期运行的任务。下面将详细介绍多线程的实现方法。
多线程(Threading) 是一种允许多个线程在同一进程中并发执行的技术。Python 提供了 threading
模块来实现多线程。使用多线程可以提高程序的并发性和响应性,特别适用于 I/O 密集型任务。下面是一个简单的多线程示例:
import threading
import time
def worker():
print("Worker thread is running")
time.sleep(2)
print("Worker thread is done")
threads = []
for i in range(5):
thread = threading.Thread(target=worker)
threads.append(thread)
thread.start()
for thread in threads:
thread.join()
print("All threads are done")
在这个示例中,我们创建了五个线程,每个线程执行 worker
函数。主线程等待所有子线程完成后再退出。
一、多线程
多线程是实现并发编程的一种重要方法。在 Python 中,threading
模块提供了对多线程的支持。通过创建多个线程,可以同时执行多个任务,从而提高程序的性能。下面将详细介绍多线程的概念、使用方法以及相关注意事项。
1、线程的创建与启动
在 Python 中,可以使用 threading.Thread
类来创建和启动线程。每个线程都需要一个目标函数,即线程要执行的任务。可以通过以下步骤创建并启动线程:
import threading
def task():
print("Thread is running")
创建线程
thread = threading.Thread(target=task)
启动线程
thread.start()
等待线程完成
thread.join()
print("Main thread is done")
2、线程同步
多线程编程中,线程之间可能会共享资源。为了避免资源竞争,需要进行线程同步。Python 提供了多种同步机制,如锁(Lock)、条件变量(Condition)、事件(Event)等。下面是使用锁进行同步的示例:
import threading
lock = threading.Lock()
shared_resource = 0
def increment():
global shared_resource
with lock:
for _ in range(1000):
shared_resource += 1
threads = []
for _ in range(10):
thread = threading.Thread(target=increment)
threads.append(thread)
thread.start()
for thread in threads:
thread.join()
print("Shared resource value:", shared_resource)
3、线程池
如果需要创建大量线程,可以使用 concurrent.futures.ThreadPoolExecutor
来管理线程池。线程池可以限制同时运行的线程数量,并简化线程管理。以下是使用线程池的示例:
import concurrent.futures
def task(x):
return x * x
with concurrent.futures.ThreadPoolExecutor(max_workers=5) as executor:
futures = [executor.submit(task, i) for i in range(10)]
results = [future.result() for future in futures]
print("Results:", results)
4、线程之间的通信
线程之间可以通过队列(Queue)进行通信。Python 提供了 queue.Queue
类来实现线程安全的队列。以下是一个线程间通信的示例:
import threading
import queue
def producer(q):
for i in range(5):
q.put(i)
print(f"Produced {i}")
def consumer(q):
while True:
item = q.get()
if item is None:
break
print(f"Consumed {item}")
q = queue.Queue()
producer_thread = threading.Thread(target=producer, args=(q,))
consumer_thread = threading.Thread(target=consumer, args=(q,))
producer_thread.start()
consumer_thread.start()
producer_thread.join()
q.put(None)
consumer_thread.join()
二、多进程
多进程是一种并行编程技术,可以在多个 CPU 核心上同时执行多个任务,从而提高程序的性能。Python 提供了 multiprocessing
模块来实现多进程编程。下面将详细介绍多进程的概念、使用方法以及相关注意事项。
1、进程的创建与启动
在 Python 中,可以使用 multiprocessing.Process
类来创建和启动进程。每个进程都需要一个目标函数,即进程要执行的任务。可以通过以下步骤创建并启动进程:
import multiprocessing
def task():
print("Process is running")
创建进程
process = multiprocessing.Process(target=task)
启动进程
process.start()
等待进程完成
process.join()
print("Main process is done")
2、进程之间的通信
进程之间可以通过队列(Queue)和管道(Pipe)进行通信。Python 提供了 multiprocessing.Queue
和 multiprocessing.Pipe
类来实现进程间通信。以下是使用队列进行通信的示例:
import multiprocessing
def producer(q):
for i in range(5):
q.put(i)
print(f"Produced {i}")
def consumer(q):
while True:
item = q.get()
if item is None:
break
print(f"Consumed {item}")
q = multiprocessing.Queue()
producer_process = multiprocessing.Process(target=producer, args=(q,))
consumer_process = multiprocessing.Process(target=consumer, args=(q,))
producer_process.start()
consumer_process.start()
producer_process.join()
q.put(None)
consumer_process.join()
3、进程池
如果需要创建大量进程,可以使用 multiprocessing.Pool
来管理进程池。进程池可以限制同时运行的进程数量,并简化进程管理。以下是使用进程池的示例:
import multiprocessing
def task(x):
return x * x
with multiprocessing.Pool(processes=5) as pool:
results = pool.map(task, range(10))
print("Results:", results)
4、进程同步
多进程编程中,进程之间可能会共享资源。为了避免资源竞争,需要进行进程同步。Python 提供了多种同步机制,如锁(Lock)、条件变量(Condition)、事件(Event)等。下面是使用锁进行同步的示例:
import multiprocessing
lock = multiprocessing.Lock()
shared_resource = multiprocessing.Value('i', 0)
def increment():
with lock:
for _ in range(1000):
shared_resource.value += 1
processes = []
for _ in range(10):
process = multiprocessing.Process(target=increment)
processes.append(process)
process.start()
for process in processes:
process.join()
print("Shared resource value:", shared_resource.value)
三、协程
协程是一种比线程更轻量级的并发编程技术。Python 提供了 asyncio
模块来实现协程编程。协程适用于 I/O 密集型任务,可以在单线程中实现并发执行。下面将详细介绍协程的概念、使用方法以及相关注意事项。
1、协程的定义与运行
在 Python 中,可以使用 async def
关键字定义协程函数,并使用 await
关键字等待异步操作。可以通过以下步骤定义并运行协程:
import asyncio
async def task():
print("Coroutine is running")
await asyncio.sleep(1)
print("Coroutine is done")
创建事件循环
loop = asyncio.get_event_loop()
运行协程
loop.run_until_complete(task())
2、协程的调度与并发
可以使用 asyncio.gather
或 asyncio.create_task
来调度多个协程并发执行。以下是调度多个协程的示例:
import asyncio
async def task(id):
print(f"Task {id} is running")
await asyncio.sleep(1)
print(f"Task {id} is done")
async def main():
tasks = [asyncio.create_task(task(i)) for i in range(5)]
await asyncio.gather(*tasks)
运行主协程
asyncio.run(main())
3、协程间的通信
协程之间可以通过队列(Queue)进行通信。Python 提供了 asyncio.Queue
类来实现协程间通信。以下是一个协程间通信的示例:
import asyncio
async def producer(q):
for i in range(5):
await q.put(i)
print(f"Produced {i}")
async def consumer(q):
while True:
item = await q.get()
if item is None:
break
print(f"Consumed {item}")
async def main():
q = asyncio.Queue()
producer_task = asyncio.create_task(producer(q))
consumer_task = asyncio.create_task(consumer(q))
await producer_task
await q.put(None)
await consumer_task
运行主协程
asyncio.run(main())
4、协程的异常处理
在协程中进行异常处理时,可以使用 try
和 except
语句来捕获和处理异常。以下是一个协程异常处理的示例:
import asyncio
async def task():
try:
print("Coroutine is running")
await asyncio.sleep(1)
raise ValueError("An error occurred")
except ValueError as e:
print(f"Exception caught: {e}")
运行协程
asyncio.run(task())
四、守护进程
守护进程是一种在后台运行的长期任务,通常用于执行后台服务或定时任务。在 Python 中,可以使用 threading
或 multiprocessing
模块创建守护进程。下面将详细介绍守护进程的概念、使用方法以及相关注意事项。
1、线程守护进程
可以将线程设置为守护线程,这样当主线程退出时,守护线程也会自动退出。可以通过设置线程的 daemon
属性来实现。以下是一个线程守护进程的示例:
import threading
import time
def task():
while True:
print("Daemon thread is running")
time.sleep(1)
创建守护线程
thread = threading.Thread(target=task)
thread.setDaemon(True)
启动守护线程
thread.start()
主线程休眠
time.sleep(5)
print("Main thread is done")
2、进程守护进程
可以将进程设置为守护进程,这样当主进程退出时,守护进程也会自动退出。可以通过设置进程的 daemon
属性来实现。以下是一个进程守护进程的示例:
import multiprocessing
import time
def task():
while True:
print("Daemon process is running")
time.sleep(1)
创建守护进程
process = multiprocessing.Process(target=task)
process.daemon = True
启动守护进程
process.start()
主进程休眠
time.sleep(5)
print("Main process is done")
3、守护进程的管理
在实际应用中,可能需要管理多个守护进程。可以使用 multiprocessing
模块中的 Manager
类来管理共享资源,并通过进程池来管理多个守护进程。以下是一个示例:
import multiprocessing
import time
def task(shared_resource):
while True:
with shared_resource.get_lock():
shared_resource.value += 1
print(f"Daemon process incremented value to {shared_resource.value}")
time.sleep(1)
def main():
manager = multiprocessing.Manager()
shared_resource = manager.Value('i', 0)
process_pool = []
for _ in range(5):
process = multiprocessing.Process(target=task, args=(shared_resource,))
process.daemon = True
process_pool.append(process)
process.start()
time.sleep(5)
print("Main process is done")
if __name__ == "__main__":
main()
4、守护进程的使用场景
守护进程通常用于后台服务、定时任务和监控任务等场景。例如,可以使用守护进程实现日志监控、定时数据备份和系统状态监控。以下是一个使用守护进程实现定时任务的示例:
import threading
import time
def task():
while True:
print("Performing scheduled task")
time.sleep(10)
def main():
thread = threading.Thread(target=task)
thread.setDaemon(True)
thread.start()
while True:
print("Main thread is running")
time.sleep(1)
if __name__ == "__main__":
main()
五、总结
在 Python 中,实现后台操作的方法主要包括多线程、多进程、协程和守护进程。每种方法都有其适用的场景和优缺点。多线程适用于 I/O 密集型任务,可以提高程序的并发性和响应性;多进程适用于 CPU 密集型任务,可以在多个 CPU 核心上并行执行任务;协程适用于需要异步执行的任务,可以在单线程中实现并发执行;守护进程适用于需要在后台长期运行的任务,可以通过设置守护属性来实现。
在选择使用哪种方法时,需要根据具体的应用场景和任务类型来决定。同时,需要注意线程和进程之间的通信和同步,避免资源竞争和死锁问题。在实际应用中,可以结合使用多线程、多进程和协程,以提高程序的性能和稳定性。
相关问答FAQs:
如何使用Python进行后台任务调度?
Python提供了多种库来实现后台任务调度,例如Celery和APScheduler。Celery是一个强大的异步任务队列,可以轻松地处理分布式任务。而APScheduler则适合用于定时任务调度。用户可以根据需求选择合适的库,并通过设置任务的执行时间和频率,实现有效的后台操作。
在Python中如何处理后台进程?
在Python中,使用multiprocessing
模块可以创建后台进程。用户可以通过创建Process
对象来启动新的进程,并通过start()
方法运行它。这样可以在后台执行长时间运行的任务,而不会阻塞主线程。还可以使用队列来实现进程间的通信,确保数据的安全传递。
如何确保Python后台操作的异常处理?
在进行后台操作时,异常处理是非常重要的。用户可以使用try-except
语句来捕捉可能发生的异常。同时,可以结合日志库(如logging
)记录错误信息,以便后续分析和调试。此外,使用守护进程或重试机制可以增加后台任务的稳定性,确保在出现错误时能够自动恢复。
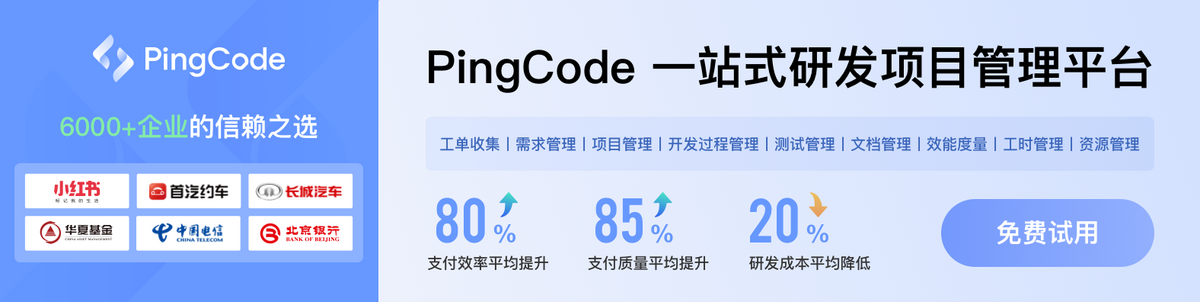