自动投票是一种利用Python脚本模拟用户在网站上进行投票的技术。自动投票需要使用Python的HTTP请求库、模拟用户行为的库、处理动态内容的库。其中一个关键点是使用HTTP请求库来发送请求。以下是具体步骤:
一、使用requests库发送HTTP请求
Python中的requests库是一个简单的HTTP库,可以发送各种HTTP请求。我们可以使用requests库来模拟用户在网站上进行投票。
import requests
url = 'https://example.com/vote'
data = {
'option': 'A', # 投票选项
'user_id': '12345' # 用户ID
}
response = requests.post(url, data=data)
print(response.text)
详细描述:在发送请求之前,我们需要了解目标网站的API接口文档,以确保我们发送的请求符合网站的要求。我们可以使用浏览器的开发者工具查看网站的网络请求,找到投票请求的URL和请求参数。
二、模拟用户行为
有些网站会检查用户行为,以防止自动投票。我们可以使用Python的Selenium库来模拟用户行为,例如点击按钮、输入文本等。
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.common.keys import Keys
driver = webdriver.Chrome()
driver.get('https://example.com/vote')
option = driver.find_element(By.ID, 'option_A')
option.click()
submit_button = driver.find_element(By.ID, 'submit')
submit_button.click()
driver.quit()
三、处理动态内容
有些网站会使用JavaScript动态加载内容,这时候仅仅发送HTTP请求或模拟用户点击是无法实现自动投票的。我们需要使用Selenium或其他库来处理动态内容。
from selenium import webdriver
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
from selenium.webdriver.common.by import By
driver = webdriver.Chrome()
driver.get('https://example.com/vote')
等待投票选项加载完成
option = WebDriverWait(driver, 10).until(
EC.presence_of_element_located((By.ID, 'option_A'))
)
option.click()
submit_button = driver.find_element(By.ID, 'submit')
submit_button.click()
driver.quit()
四、使用代理和用户代理
为了防止被网站封禁IP,我们可以使用代理服务器和修改用户代理。
import requests
url = 'https://example.com/vote'
data = {
'option': 'A',
'user_id': '12345'
}
proxies = {
'http': 'http://10.10.1.10:3128',
'https': 'http://10.10.1.10:1080',
}
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3'
}
response = requests.post(url, data=data, proxies=proxies, headers=headers)
print(response.text)
五、使用多线程或异步编程
为了提高投票效率,我们可以使用多线程或异步编程来同时发送多个投票请求。
import requests
import threading
def vote():
url = 'https://example.com/vote'
data = {
'option': 'A',
'user_id': '12345'
}
response = requests.post(url, data=data)
print(response.text)
threads = []
for _ in range(10):
t = threading.Thread(target=vote)
t.start()
threads.append(t)
for t in threads:
t.join()
六、应对验证码
一些网站会使用验证码来防止自动投票。应对验证码是一个复杂的问题,可以使用OCR技术或第三方验证码识别服务。
import requests
from PIL import Image
import pytesseract
下载验证码图片
url = 'https://example.com/captcha'
response = requests.get(url)
with open('captcha.png', 'wb') as f:
f.write(response.content)
识别验证码
image = Image.open('captcha.png')
captcha_text = pytesseract.image_to_string(image)
发送投票请求
vote_url = 'https://example.com/vote'
data = {
'option': 'A',
'user_id': '12345',
'captcha': captcha_text
}
response = requests.post(vote_url, data=data)
print(response.text)
七、保持会话状态
有些网站需要用户登录才能投票,我们需要保持会话状态。
import requests
session = requests.Session()
登录
login_url = 'https://example.com/login'
login_data = {
'username': 'user',
'password': 'pass'
}
session.post(login_url, data=login_data)
投票
vote_url = 'https://example.com/vote'
vote_data = {
'option': 'A',
'user_id': '12345'
}
response = session.post(vote_url, data=vote_data)
print(response.text)
八、处理异常情况
在进行自动投票时,我们需要处理各种可能的异常情况,如网络错误、服务器错误等。
import requests
url = 'https://example.com/vote'
data = {
'option': 'A',
'user_id': '12345'
}
try:
response = requests.post(url, data=data)
response.raise_for_status() # 检查HTTP状态码
print(response.text)
except requests.exceptions.RequestException as e:
print(f'Error: {e}')
九、日志记录
为了便于调试和跟踪,我们可以在脚本中添加日志记录。
import logging
logging.basicConfig(level=logging.INFO, format='%(asctime)s - %(levelname)s - %(message)s')
def vote():
url = 'https://example.com/vote'
data = {
'option': 'A',
'user_id': '12345'
}
try:
response = requests.post(url, data=data)
response.raise_for_status()
logging.info('Vote successful')
except requests.exceptions.RequestException as e:
logging.error(f'Error: {e}')
vote()
十、遵守法律和道德规范
在进行自动投票时,我们需要遵守法律和道德规范。自动投票可能违反网站的使用条款和政策,甚至违法。我们应该确保我们的行为合法合规,并尊重他人的权益。
总结:以上是关于如何使用Python进行自动投票的详细步骤和注意事项。通过使用requests库、Selenium库、代理服务器、用户代理、多线程或异步编程、OCR技术、会话状态管理、异常处理和日志记录等技术,我们可以实现自动投票。但我们需要注意,自动投票可能违反网站的使用条款和政策,甚至违法。我们应该确保我们的行为合法合规,并尊重他人的权益。
相关问答FAQs:
如何使用Python进行自动投票?
要实现自动投票功能,您需要了解如何使用Python编写脚本来模拟用户行为。这通常涉及到使用库如requests
或selenium
来处理HTTP请求或控制浏览器。确保您遵循相关网站的使用条款和法律规定,避免违反任何规则。
Python自动投票的实现步骤有哪些?
在实现自动投票时,您可以按照以下步骤进行:首先,选择一个合适的库,比如requests
用于发送HTTP请求或selenium
用于浏览器自动化。接着,了解目标网站的投票流程,通过网络抓包工具获取所需的请求参数。最后,编写脚本来模拟提交投票的过程,并进行测试以确保其有效性。
使用Python自动投票是否存在风险?
自动投票可能会带来一定的风险,尤其是如果目标网站对投票行为有严格的监控或限制。可能会面临账号封禁、IP封禁等后果。此外,某些网站的使用条款明确禁止自动化操作,因此在进行自动投票之前,请务必仔细阅读并遵循相关政策,以避免不必要的法律问题。
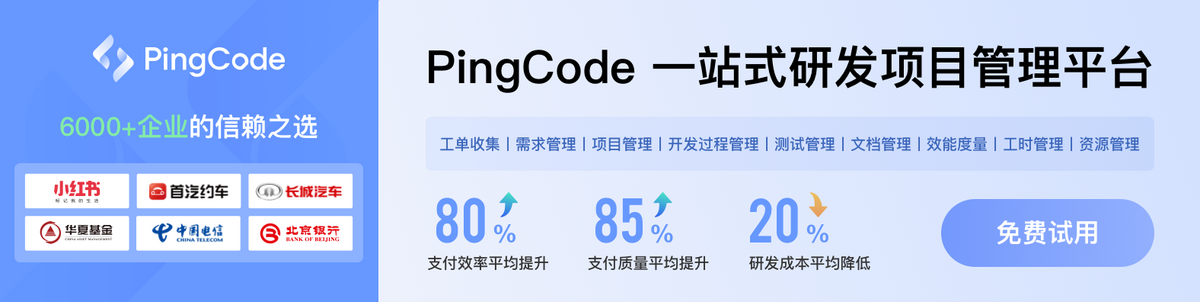