使用Python爬虫翻页可以通过多种方式实现,包括模拟浏览器请求、解析HTML内容等。主要方法有:requests库结合BeautifulSoup解析、Selenium模拟浏览器操作。 其中,requests库结合BeautifulSoup解析是一种高效且简单的方式,适用于静态网页;Selenium模拟浏览器操作适用于动态加载内容的网页。
具体来说,requests库结合BeautifulSoup解析的方法主要通过发送HTTP请求获取网页内容,然后使用BeautifulSoup进行解析和数据提取。对于需要翻页的场景,可以通过分析网页的翻页机制,找到页码参数并通过循环实现翻页爬取。
一、使用requests库和BeautifulSoup解析静态网页
1. 安装依赖库
首先,我们需要安装requests和BeautifulSoup库。可以通过以下命令安装:
pip install requests beautifulsoup4
2. 爬取单页内容
以爬取某个网站的商品列表为例,首先爬取第一页内容:
import requests
from bs4 import BeautifulSoup
url = 'https://example.com/products?page=1'
headers = {'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3'}
response = requests.get(url, headers=headers)
soup = BeautifulSoup(response.content, 'html.parser')
products = soup.find_all('div', class_='product-item')
for product in products:
title = product.find('h2').text
price = product.find('span', class_='price').text
print(f'Title: {title}, Price: {price}')
3. 分析翻页机制
通常网页的翻页是通过URL的参数变化实现的,比如page=1
、page=2
等。我们可以通过循环来构造这些URL并进行请求。
4. 实现翻页爬取
在上面的基础上,我们实现一个循环来爬取多个页面:
for page in range(1, 6): # 爬取前5页
url = f'https://example.com/products?page={page}'
response = requests.get(url, headers=headers)
soup = BeautifulSoup(response.content, 'html.parser')
products = soup.find_all('div', class_='product-item')
for product in products:
title = product.find('h2').text
price = product.find('span', class_='price').text
print(f'Title: {title}, Price: {price}')
二、使用Selenium模拟浏览器操作爬取动态网页
1. 安装依赖库
需要安装Selenium库和浏览器驱动,例如ChromeDriver。可以通过以下命令安装Selenium:
pip install selenium
2. 配置浏览器驱动
下载ChromeDriver并将其路径添加到系统环境变量中,或者在代码中指定路径。
3. 爬取单页内容
以爬取动态加载的商品列表为例,首先爬取第一页内容:
from selenium import webdriver
from selenium.webdriver.common.by import By
driver = webdriver.Chrome() # 如果未将ChromeDriver添加到系统路径中,需要指定executable_path参数
driver.get('https://example.com/products')
products = driver.find_elements(By.CLASS_NAME, 'product-item')
for product in products:
title = product.find_element(By.TAG_NAME, 'h2').text
price = product.find_element(By.CLASS_NAME, 'price').text
print(f'Title: {title}, Price: {price}')
4. 分析翻页机制
通常网页的翻页按钮会触发JavaScript事件,通过观察可以找到翻页按钮的定位方式。
5. 实现翻页爬取
在上面的基础上,我们实现翻页操作:
from selenium.webdriver.common.by import By
from selenium.webdriver.common.keys import Keys
driver = webdriver.Chrome()
driver.get('https://example.com/products')
for page in range(1, 6): # 爬取前5页
products = driver.find_elements(By.CLASS_NAME, 'product-item')
for product in products:
title = product.find_element(By.TAG_NAME, 'h2').text
price = product.find_element(By.CLASS_NAME, 'price').text
print(f'Title: {title}, Price: {price}')
next_button = driver.find_element(By.LINK_TEXT, 'Next')
next_button.click()
driver.implicitly_wait(3) # 等待页面加载
三、处理反爬机制
在实际操作中,有些网站会有反爬机制,例如通过检测频繁请求、使用验证码等来阻止爬虫。以下是一些常见的应对方法:
1. 设置请求头
通过设置User-Agent等请求头,可以模拟正常用户的请求:
headers = {'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3'}
response = requests.get(url, headers=headers)
2. 使用代理IP
使用代理IP可以隐藏真实IP地址,避免被封禁:
proxies = {
'http': 'http://10.10.1.10:3128',
'https': 'http://10.10.1.10:1080',
}
response = requests.get(url, headers=headers, proxies=proxies)
3. 添加延迟
通过在请求之间添加随机延迟,可以降低被检测到的风险:
import time
import random
for page in range(1, 6):
url = f'https://example.com/products?page={page}'
response = requests.get(url, headers=headers)
soup = BeautifulSoup(response.content, 'html.parser')
products = soup.find_all('div', class_='product-item')
for product in products:
title = product.find('h2').text
price = product.find('span', class_='price').text
print(f'Title: {title}, Price: {price}')
time.sleep(random.uniform(1, 3)) # 随机延迟1到3秒
四、处理动态内容和JavaScript渲染
有些网页的内容是通过JavaScript动态加载的,requests库无法直接获取到这些内容。这时可以使用Selenium或Pyppeteer等工具。
1. 使用Selenium
Selenium可以模拟用户操作,执行JavaScript并获取渲染后的网页内容:
from selenium import webdriver
from selenium.webdriver.common.by import By
driver = webdriver.Chrome()
driver.get('https://example.com/products')
for page in range(1, 6):
products = driver.find_elements(By.CLASS_NAME, 'product-item')
for product in products:
title = product.find_element(By.TAG_NAME, 'h2').text
price = product.find_element(By.CLASS_NAME, 'price').text
print(f'Title: {title}, Price: {price}')
next_button = driver.find_element(By.LINK_TEXT, 'Next')
next_button.click()
driver.implicitly_wait(3)
2. 使用Pyppeteer
Pyppeteer是Puppeteer的Python版本,同样可以用于处理JavaScript渲染的网页:
import asyncio
from pyppeteer import launch
async def main():
browser = await launch()
page = await browser.newPage()
await page.goto('https://example.com/products')
for page_num in range(1, 6):
products = await page.querySelectorAll('.product-item')
for product in products:
title = await page.evaluate('(element) => element.querySelector("h2").textContent', product)
price = await page.evaluate('(element) => element.querySelector(".price").textContent', product)
print(f'Title: {title}, Price: {price}')
next_button = await page.querySelector('a[rel="next"]')
if next_button:
await next_button.click()
await page.waitForNavigation()
await browser.close()
asyncio.get_event_loop().run_until_complete(main())
五、处理复杂翻页逻辑
有些网站的翻页逻辑比较复杂,可能需要通过POST请求提交表单,或者通过Ajax请求获取数据。
1. 通过POST请求翻页
有些网站的翻页是通过POST请求实现的,可以通过分析请求参数来实现翻页爬取:
import requests
from bs4 import BeautifulSoup
url = 'https://example.com/products'
headers = {'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3'}
for page in range(1, 6):
data = {'page': page}
response = requests.post(url, headers=headers, data=data)
soup = BeautifulSoup(response.content, 'html.parser')
products = soup.find_all('div', class_='product-item')
for product in products:
title = product.find('h2').text
price = product.find('span', class_='price').text
print(f'Title: {title}, Price: {price}')
2. 通过Ajax请求翻页
有些网站的翻页是通过Ajax请求实现的,可以通过分析Ajax请求的URL和参数来实现翻页爬取:
import requests
from bs4 import BeautifulSoup
url = 'https://example.com/ajax/products'
headers = {'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3'}
for page in range(1, 6):
params = {'page': page}
response = requests.get(url, headers=headers, params=params)
data = response.json()
for product in data['products']:
title = product['title']
price = product['price']
print(f'Title: {title}, Price: {price}')
六、总结
使用Python爬虫翻页可以通过requests库结合BeautifulSoup解析静态网页,或使用Selenium、Pyppeteer等工具处理动态网页。通过分析网页的翻页机制,可以构造循环实现多页爬取。同时,需要注意处理反爬机制,如设置请求头、使用代理IP、添加延迟等。对于复杂的翻页逻辑,可以通过POST请求或Ajax请求实现。希望以上内容能帮助你更好地理解和实现Python爬虫翻页。
相关问答FAQs:
如何在Python爬虫中实现翻页功能?
在Python爬虫中,实现翻页功能通常涉及到分析网页的结构和URL参数。很多网站使用查询字符串或URL路径来表示不同的页面。通过修改请求的URL,可以获取到下一页的数据。例如,如果一个网站的分页链接为example.com/page=1
,你可以通过将page
参数的值增加来访问后续页面,如page=2
、page=3
等。
需要注意哪些反爬虫措施,以确保翻页爬虫的有效性?
在进行翻页爬虫时,网站可能会采用多种反爬虫措施,例如限制请求频率、使用验证码或IP封禁。为了应对这些措施,可以在代码中加入随机的请求间隔、使用代理IP、以及模拟人类用户的行为(如随机浏览器User-Agent)。这些策略可以有效减少被封禁的风险。
如何处理爬取到的数据,确保翻页爬虫的结果完整?
在翻页过程中,确保数据完整性是至关重要的。可以通过将每一页的数据存储在一个统一的列表中,或者直接写入数据库。处理数据时,注意去重,以防同一条数据在不同页重复爬取。此外,确保在每次请求时检查返回状态,以处理可能的错误或异常情况,这样可以确保数据的准确性与完整性。
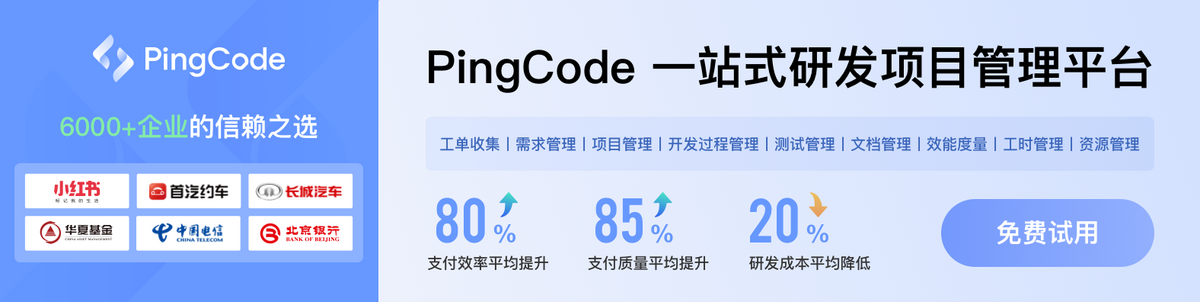