理解Python装饰器的核心在于理解它们的本质、使用场景、实现方法。装饰器本质上是一个函数,它接收另一个函数作为参数,并返回一个新的函数。装饰器的主要作用是为现有函数或方法添加额外的功能,而无需修改其代码。函数嵌套、函数作为参数传递、高阶函数是理解装饰器的关键。以下是对“函数嵌套”的详细描述:
函数嵌套是指在一个函数内部定义另一个函数。在Python中,函数是第一类对象,可以作为参数传递,也可以在另一个函数中定义和使用。这种嵌套定义的函数可以访问其外部函数的变量,这为装饰器提供了强大的灵活性。例如:
def outer_function():
message = 'Hello'
def inner_function():
print(message)
inner_function()
outer_function()
在这个例子中,inner_function
是在outer_function
内部定义的,并且它可以访问outer_function
中的message
变量。这是装饰器能够修改或扩展函数行为的基础。
一、装饰器的基本概念
装饰器是Python中的一种高级特性,可以用来修改或扩展函数、方法或类的行为,而无需直接修改它们的代码。装饰器本质上是一个函数,它接收一个函数作为输入,并返回一个新函数。
1.1 函数即对象
在Python中,函数是一等公民,意味着函数可以像其他对象一样传递、赋值和返回。例如:
def greet(name):
return f"Hello, {name}!"
say_hello = greet
print(say_hello("Alice")) # 输出: Hello, Alice!
这表明函数可以作为变量赋值给其他名称,这为创建装饰器提供了基础。
1.2 高阶函数
高阶函数是指接受一个或多个函数作为参数,或者返回另一个函数作为结果的函数。装饰器就是一种高阶函数。以下是一个简单的高阶函数示例:
def shout(text):
return text.upper()
def whisper(text):
return text.lower()
def greet(func):
greeting = func("Hello, World!")
print(greeting)
greet(shout) # 输出: HELLO, WORLD!
greet(whisper) # 输出: hello, world!
在这个例子中,greet
函数接受另一个函数作为参数,并调用它。
二、创建装饰器
装饰器的主要目的是在不改变原函数代码的情况下,动态地添加一些功能。装饰器通常用于日志记录、性能测试、事务管理、权限验证等场景。
2.1 使用函数创建装饰器
最简单的装饰器是使用函数来创建。以下是一个基本的装饰器示例:
def simple_decorator(func):
def wrapper():
print("Something is happening before the function is called.")
func()
print("Something is happening after the function is called.")
return wrapper
def say_hello():
print("Hello!")
decorated_function = simple_decorator(say_hello)
decorated_function()
输出结果为:
Something is happening before the function is called.
Hello!
Something is happening after the function is called.
在这个例子中,simple_decorator
是一个装饰器,它接收func
函数作为参数,并返回一个新的wrapper
函数。这个wrapper
函数在调用原始函数func
之前和之后打印一些信息。
2.2 使用@
语法糖
Python提供了@
语法糖来简化装饰器的应用,使代码更简洁。以下是使用@
语法糖的示例:
def simple_decorator(func):
def wrapper():
print("Something is happening before the function is called.")
func()
print("Something is happening after the function is called.")
return wrapper
@simple_decorator
def say_hello():
print("Hello!")
say_hello()
输出结果与前一个示例相同,但代码更简洁易读。
三、带参数的装饰器
有时我们需要创建带参数的装饰器,以便更灵活地控制装饰器的行为。带参数的装饰器本质上是一个返回装饰器的函数。
3.1 带参数的装饰器示例
以下是一个带参数的装饰器示例:
def repeat(num_times):
def decorator_repeat(func):
def wrapper(*args, kwargs):
for _ in range(num_times):
result = func(*args, kwargs)
return result
return wrapper
return decorator_repeat
@repeat(num_times=3)
def say_hello(name):
print(f"Hello, {name}!")
say_hello("Alice")
输出结果为:
Hello, Alice!
Hello, Alice!
Hello, Alice!
在这个例子中,repeat
是一个带参数的装饰器,它接收一个参数num_times
,并返回一个decorator_repeat
装饰器。decorator_repeat
装饰器接收原始函数func
,并返回一个新的wrapper
函数,该函数调用原始函数num_times
次。
四、装饰器的应用场景
装饰器在Python中有广泛的应用,以下是一些常见的应用场景。
4.1 日志记录
装饰器可以用来自动记录函数的调用情况,这对于调试和监控非常有用。
def log_decorator(func):
def wrapper(*args, kwargs):
print(f"Calling function {func.__name__} with arguments {args} and {kwargs}")
result = func(*args, kwargs)
print(f"Function {func.__name__} returned {result}")
return result
return wrapper
@log_decorator
def add(a, b):
return a + b
add(2, 3)
输出结果为:
Calling function add with arguments (2, 3) and {}
Function add returned 5
4.2 权限验证
装饰器可以用来验证用户是否有权访问某个函数,这是实现权限控制的常见方式。
def require_permission(permission):
def decorator(func):
def wrapper(user, *args, kwargs):
if user.has_permission(permission):
return func(user, *args, kwargs)
else:
print(f"User {user.name} does not have permission {permission}")
return wrapper
return decorator
class User:
def __init__(self, name, permissions):
self.name = name
self.permissions = permissions
def has_permission(self, permission):
return permission in self.permissions
@require_permission('admin')
def delete_user(user, user_to_delete):
print(f"User {user_to_delete.name} deleted by {user.name}")
admin = User('Admin', ['admin'])
guest = User('Guest', [])
delete_user(admin, guest)
delete_user(guest, admin)
输出结果为:
User Guest deleted by Admin
User Guest does not have permission admin
五、装饰器的注意事项
虽然装饰器非常强大,但在使用时需要注意一些问题,以避免常见的陷阱。
5.1 保持函数签名
装饰器会改变原始函数的签名,使得原始函数的元信息(如名称和文档字符串)丢失。为了保持原始函数的签名,可以使用functools.wraps
装饰器。
import functools
def simple_decorator(func):
@functools.wraps(func)
def wrapper(*args, kwargs):
print("Something is happening before the function is called.")
result = func(*args, kwargs)
print("Something is happening after the function is called.")
return result
return wrapper
@simple_decorator
def say_hello(name):
"""This function greets the person with the given name."""
print(f"Hello, {name}!")
print(say_hello.__name__)
print(say_hello.__doc__)
输出结果为:
say_hello
This function greets the person with the given name.
functools.wraps
装饰器会将原始函数的元信息(如名称和文档字符串)复制到wrapper
函数中。
5.2 调试困难
装饰器会增加代码的复杂性,使得调试变得更加困难。在使用装饰器时,应该保持代码的可读性,并在必要时添加适当的日志记录和注释。
5.3 链式装饰器
多个装饰器可以应用于同一个函数,这被称为链式装饰器。链式装饰器的执行顺序是从内向外。
def decorator1(func):
def wrapper(*args, kwargs):
print("Decorator 1")
return func(*args, kwargs)
return wrapper
def decorator2(func):
def wrapper(*args, kwargs):
print("Decorator 2")
return func(*args, kwargs)
return wrapper
@decorator1
@decorator2
def say_hello(name):
print(f"Hello, {name}!")
say_hello("Alice")
输出结果为:
Decorator 1
Decorator 2
Hello, Alice!
在这个例子中,say_hello
函数首先被decorator2
装饰,然后被decorator1
装饰。执行顺序是从内向外。
六、类装饰器
除了函数装饰器,Python还支持类装饰器。类装饰器与函数装饰器类似,但它们是使用类来实现的。
6.1 基本类装饰器
以下是一个基本的类装饰器示例:
class SimpleDecorator:
def __init__(self, func):
self.func = func
def __call__(self, *args, kwargs):
print("Something is happening before the function is called.")
result = self.func(*args, kwargs)
print("Something is happening after the function is called.")
return result
@SimpleDecorator
def say_hello(name):
print(f"Hello, {name}!")
say_hello("Alice")
输出结果为:
Something is happening before the function is called.
Hello, Alice!
Something is happening after the function is called.
在这个例子中,SimpleDecorator
类实现了__call__
方法,使得它可以像函数一样调用。类装饰器的优点是它们可以在类中维护状态和数据。
6.2 带参数的类装饰器
类装饰器也可以接受参数,以便更灵活地控制装饰器的行为。
class Repeat:
def __init__(self, num_times):
self.num_times = num_times
def __call__(self, func):
def wrapper(*args, kwargs):
for _ in range(self.num_times):
result = func(*args, kwargs)
return result
return wrapper
@Repeat(num_times=3)
def say_hello(name):
print(f"Hello, {name}!")
say_hello("Alice")
输出结果为:
Hello, Alice!
Hello, Alice!
Hello, Alice!
在这个例子中,Repeat
类接收一个参数num_times
,并在__call__
方法中使用该参数来控制函数的调用次数。
七、装饰器的最佳实践
在使用装饰器时,遵循一些最佳实践可以提高代码的可读性和可维护性。
7.1 保持简单
装饰器的实现应该尽量保持简单,以便代码易于理解和维护。复杂的逻辑可以拆分为多个装饰器,分别处理不同的功能。
7.2 避免副作用
装饰器应该尽量避免引入副作用,以免影响原始函数的行为。副作用可能会导致意外的错误和难以调试的问题。
7.3 使用现有库
在实现常见功能(如日志记录、缓存、权限验证等)时,可以考虑使用现有的第三方库,这些库通常经过了广泛的测试和优化,可以减少重复劳动和潜在的错误。
八、总结
装饰器是Python中的一种强大特性,可以用来动态地修改或扩展函数、方法或类的行为。理解装饰器的本质、使用场景和实现方法,对于编写高效、可维护的Python代码至关重要。装饰器的主要应用场景包括日志记录、性能测试、事务管理、权限验证等。在使用装饰器时,应该遵循保持简单、避免副作用、使用现有库等最佳实践,以提高代码的可读性和可维护性。通过掌握装饰器的使用方法,开发者可以编写出更加灵活和强大的Python程序。
相关问答FAQs:
什么是Python装饰器,它们的主要用途是什么?
Python装饰器是一种特殊的函数,用于在不修改函数代码的情况下,增强或改变其他函数的功能。它们主要用于记录日志、执行权限检查、缓存结果、检测函数执行时间等。通过装饰器,可以提高代码的可重用性和可维护性,使得程序结构更加清晰。
如何创建一个简单的装饰器?
创建一个简单的装饰器涉及定义一个函数,该函数接收另一个函数作为参数,并返回一个新的函数。这个新的函数可以在调用原函数之前或之后添加额外的功能。示例代码如下:
def my_decorator(func):
def wrapper():
print("Something is happening before the function is called.")
func()
print("Something is happening after the function is called.")
return wrapper
通过这种方式,您可以在函数调用的前后执行自定义逻辑。
装饰器可以接受参数吗?
装饰器不仅可以修饰没有参数的函数,还可以处理带参数的函数。要实现这一点,可以在装饰器内再嵌套一个函数,以接受参数。示例代码如下:
def decorator_with_args(arg):
def my_decorator(func):
def wrapper(*args, **kwargs):
print(f"Decorator argument: {arg}")
return func(*args, **kwargs)
return wrapper
return my_decorator
这种灵活性使得装饰器在Python编程中非常强大和实用。
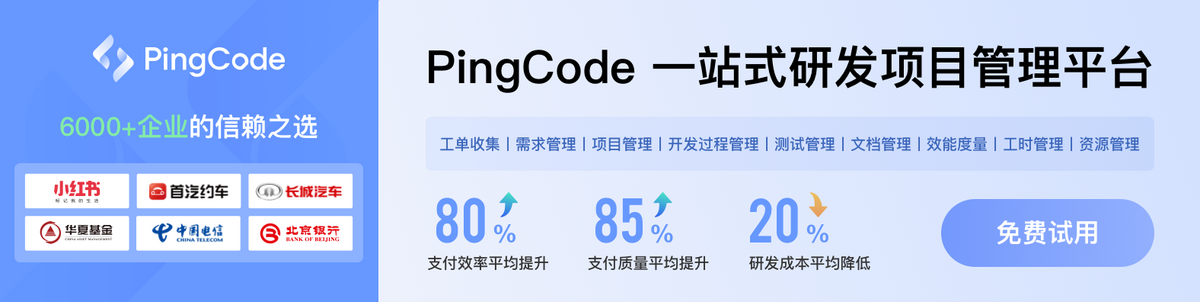