要将文件存储到Python中的方法有很多,主要包括使用内置函数打开文件、写入数据、使用库函数处理文件、处理异常等。以下是详细描述:
一、使用内置函数打开文件
Python提供了一些内置函数来打开文件,如open()
函数,它可以以多种模式打开文件,如读取('r')、写入('w')、追加('a')等。以下是一个简单示例:
# 以写入模式打开文件
file = open('example.txt', 'w')
file.write('Hello, World!')
file.close()
在这个示例中,我们使用了open()
函数以写入模式打开名为example.txt
的文件,并写入了字符串“Hello, World!”。
二、写入数据
1、写入字符串
你可以使用write()
方法将字符串写入到文件中。
with open('example.txt', 'w') as file:
file.write('Hello, World!\n')
file.write('Welcome to Python file handling.\n')
2、写入列表
你也可以将一个包含多个字符串的列表写入到文件中,每个字符串作为文件中的一行。
lines = ['Hello, World!\n', 'Welcome to Python file handling.\n']
with open('example.txt', 'w') as file:
file.writelines(lines)
三、使用库函数处理文件
Python的标准库中有许多模块可以帮助处理文件,如csv
、json
、pickle
等。
1、处理CSV文件
csv
模块提供了用于读写CSV文件的功能。
import csv
data = [
['Name', 'Age', 'City'],
['Alice', 30, 'New York'],
['Bob', 25, 'Los Angeles']
]
with open('example.csv', 'w', newline='') as file:
writer = csv.writer(file)
writer.writerows(data)
2、处理JSON文件
json
模块提供了用于读写JSON文件的功能。
import json
data = {
'name': 'Alice',
'age': 30,
'city': 'New York'
}
with open('example.json', 'w') as file:
json.dump(data, file)
四、处理异常
在处理文件时,可能会遇到各种异常,如文件不存在、权限不足等。可以使用try-except
语句来处理这些异常。
try:
with open('example.txt', 'r') as file:
content = file.read()
except FileNotFoundError:
print('File not found.')
except PermissionError:
print('Permission denied.')
五、总结
通过使用内置函数打开文件、写入数据、使用库函数处理文件、处理异常,你可以轻松地将文件存储到Python中。无论是处理简单的文本文件,还是复杂的CSV或JSON文件,Python提供了丰富的工具和库来满足你的需求。
接下来,我们将深入探讨每一个方面,提供更多的示例和详细解释。
一、使用内置函数打开文件
Python的内置函数open()
是处理文件的基础。我们将详细介绍open()
函数的不同模式和参数。
1、打开文件的模式
open()
函数可以以不同的模式打开文件:
'r'
: 读取模式(默认)'w'
: 写入模式,会覆盖文件内容'a'
: 追加模式,在文件末尾添加内容'b'
: 二进制模式't'
: 文本模式(默认)'x'
: 创建模式,文件存在时会报错
以下是每种模式的示例:
读取模式
with open('example.txt', 'r') as file:
content = file.read()
print(content)
写入模式
with open('example.txt', 'w') as file:
file.write('This is a new line.\n')
追加模式
with open('example.txt', 'a') as file:
file.write('This line will be added to the file.\n')
二进制模式
with open('example.png', 'rb') as file:
content = file.read()
print(content)
创建模式
try:
with open('example.txt', 'x') as file:
file.write('This file is newly created.\n')
except FileExistsError:
print('File already exists.')
2、文件对象的方法
打开文件后,file
对象提供了多种方法来操作文件:
read(size=-1)
: 读取文件内容readline(size=-1)
: 读取一行readlines(hint=-1)
: 读取所有行write(string)
: 写入字符串writelines(lines)
: 写入多个字符串close()
: 关闭文件
以下是这些方法的示例:
读取文件内容
with open('example.txt', 'r') as file:
content = file.read()
print(content)
读取一行
with open('example.txt', 'r') as file:
line = file.readline()
print(line)
读取所有行
with open('example.txt', 'r') as file:
lines = file.readlines()
for line in lines:
print(line, end='')
写入字符串
with open('example.txt', 'w') as file:
file.write('This is a new line.\n')
写入多个字符串
lines = ['First line.\n', 'Second line.\n']
with open('example.txt', 'w') as file:
file.writelines(lines)
3、文件位置和缓冲区
文件位置
file
对象提供了一些方法来操作文件位置:
tell()
: 返回文件当前位置seek(offset, whence=0)
: 移动到文件的指定位置
以下是这些方法的示例:
with open('example.txt', 'r') as file:
print(file.tell()) # 输出当前位置
file.read(10)
print(file.tell()) # 输出当前位置
file.seek(0)
print(file.tell()) # 输出当前位置
缓冲区
open()
函数的buffering
参数控制文件的缓冲行为:
0
: 不缓冲1
: 行缓冲(文本模式)>1
: 固定大小的缓冲区
以下是设置缓冲区的示例:
with open('example.txt', 'w', buffering=1) as file:
file.write('This is a line with line buffering.\n')
二、写入数据
1、写入字符串
在处理文件时,写入字符串是最常见的操作之一。除了write()
方法外,还可以使用格式化字符串来写入数据。
基本写入
with open('example.txt', 'w') as file:
file.write('Hello, World!\n')
格式化字符串
name = 'Alice'
age = 30
with open('example.txt', 'w') as file:
file.write(f'Name: {name}, Age: {age}\n')
2、写入列表
写入列表时,可以使用writelines()
方法,将列表中的每个字符串写入文件。
基本写入
lines = ['Hello, World!\n', 'Welcome to Python file handling.\n']
with open('example.txt', 'w') as file:
file.writelines(lines)
带换行符的写入
lines = ['First line\n', 'Second line\n', 'Third line\n']
with open('example.txt', 'w') as file:
file.writelines(lines)
3、写入字典
写入字典时,可以将字典序列化为字符串或使用特定的格式写入文件。
序列化为字符串
data = {'name': 'Alice', 'age': 30, 'city': 'New York'}
with open('example.txt', 'w') as file:
file.write(str(data))
使用格式写入
data = {'name': 'Alice', 'age': 30, 'city': 'New York'}
with open('example.txt', 'w') as file:
for key, value in data.items():
file.write(f'{key}: {value}\n')
三、使用库函数处理文件
Python提供了许多库来处理不同类型的文件,如CSV、JSON和二进制文件。我们将详细介绍如何使用这些库。
1、处理CSV文件
csv
模块提供了用于读写CSV文件的功能。
写入CSV文件
import csv
data = [
['Name', 'Age', 'City'],
['Alice', 30, 'New York'],
['Bob', 25, 'Los Angeles']
]
with open('example.csv', 'w', newline='') as file:
writer = csv.writer(file)
writer.writerows(data)
读取CSV文件
import csv
with open('example.csv', 'r') as file:
reader = csv.reader(file)
for row in reader:
print(row)
2、处理JSON文件
json
模块提供了用于读写JSON文件的功能。
写入JSON文件
import json
data = {
'name': 'Alice',
'age': 30,
'city': 'New York'
}
with open('example.json', 'w') as file:
json.dump(data, file)
读取JSON文件
import json
with open('example.json', 'r') as file:
data = json.load(file)
print(data)
3、处理二进制文件
处理二进制文件时,可以使用struct
模块来处理固定格式的二进制数据。
写入二进制文件
import struct
data = 1, b'abc', 2.7
with open('example.bin', 'wb') as file:
file.write(struct.pack('I3sf', *data))
读取二进制文件
import struct
with open('example.bin', 'rb') as file:
data = struct.unpack('I3sf', file.read())
print(data)
四、处理异常
在处理文件时,可能会遇到各种异常,如文件不存在、权限不足等。可以使用try-except
语句来处理这些异常,确保程序不会因为异常而崩溃。
1、捕获常见异常
以下是捕获常见异常的示例:
捕获文件不存在异常
try:
with open('example.txt', 'r') as file:
content = file.read()
except FileNotFoundError:
print('File not found.')
捕获权限异常
try:
with open('example.txt', 'r') as file:
content = file.read()
except PermissionError:
print('Permission denied.')
2、捕获多种异常
可以使用多个except
块来捕获不同类型的异常:
try:
with open('example.txt', 'r') as file:
content = file.read()
except FileNotFoundError:
print('File not found.')
except PermissionError:
print('Permission denied.')
except Exception as e:
print(f'An error occurred: {e}')
3、使用finally
块
finally
块用于在异常发生后执行清理操作,例如关闭文件:
try:
file = open('example.txt', 'r')
content = file.read()
except FileNotFoundError:
print('File not found.')
finally:
file.close()
五、总结
通过上述详细介绍和示例,我们可以看到Python提供了丰富的工具和库来处理文件。无论是简单的文本文件,还是复杂的CSV、JSON或二进制文件,Python都能高效地处理。以下是总结:
- 使用内置函数打开文件:
open()
函数提供了多种模式和参数,可以根据需要灵活使用。 - 写入数据:可以写入字符串、列表和字典,满足不同的数据存储需求。
- 使用库函数处理文件:
csv
、json
和struct
等模块提供了强大的文件处理功能。 - 处理异常:使用
try-except
语句捕获异常,确保程序的健壮性。
通过掌握这些技巧和方法,你可以轻松地将文件存储到Python中,并高效地进行文件操作。无论是进行数据存储、数据分析还是其他应用,Python的文件处理能力都能为你提供强大的支持。
相关问答FAQs:
如何在Python中创建一个新文件?
在Python中,可以使用内置的open()
函数来创建一个新文件。只需指定文件名和模式,例如'w'
(写入模式)或'a'
(追加模式)。例如,使用with open('example.txt', 'w') as file:
可以创建一个名为example.txt
的新文件并准备写入数据。
Python支持哪些文件格式的存储?
Python可以处理多种文件格式,包括文本文件(如.txt)、CSV文件、JSON文件、Excel文件等。每种格式都有对应的库,比如csv
用于CSV文件,json
用于JSON文件,pandas
库则可以处理Excel文件。根据需要选择合适的库和文件格式。
如何将数据写入Python文件?
可以使用write()
方法将数据写入文件。打开文件后,调用file.write('要写入的内容')
即可写入字符串。如果需要写入多行数据,可以使用writelines()
方法,或通过循环写入每一行。注意在写入之前确保文件是以写入模式打开的。
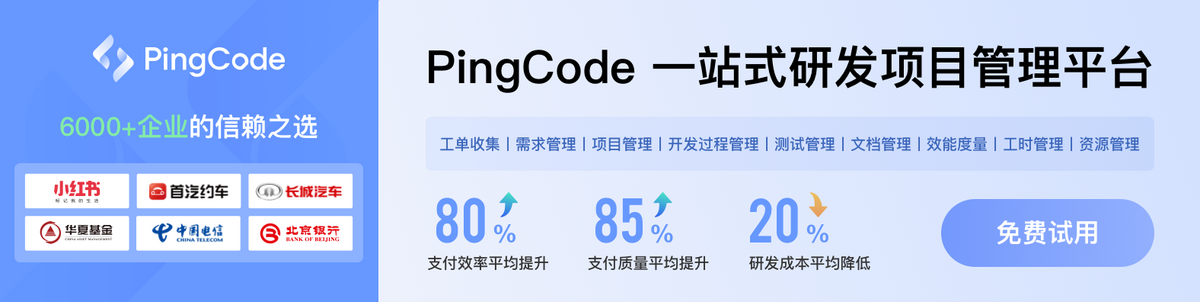