Python 可以通过多种方式来使用和执行 Shell 脚本,包括使用 subprocess 模块、os 模块、以及通过直接调用 Shell 命令等。 最常用的方法是使用 subprocess 模块,因为它提供了更强大的功能和更细粒度的控制。在本文中,我们将重点介绍如何使用 subprocess 模块来执行 Shell 脚本,并详细解释其中的一种方法。
一、SUBPROCESS 模块
subprocess 模块是 Python 中用于执行外部命令和 Shell 脚本的最常用工具。它提供了多种函数和方法,可以创建子进程、连接它们的输入/输出/错误管道,并获取返回值。以下是如何使用 subprocess 模块的详细介绍。
1、使用 subprocess.run() 方法
subprocess.run() 是一个简单且常用的方法,用于执行 Shell 命令或脚本,并等待命令执行完成。它返回一个 CompletedProcess 实例,包含了命令的执行结果。以下是一个基本示例:
import subprocess
result = subprocess.run(['ls', '-l'], capture_output=True, text=True)
print(result.stdout)
在这个示例中,我们使用 subprocess.run()
来执行 ls -l
命令,并捕获其输出。capture_output=True
参数指示捕获标准输出和错误,text=True
表示将输出作为字符串处理。
2、捕获输出和错误
除了捕获标准输出,我们还可以捕获标准错误。以下是一个示例:
import subprocess
result = subprocess.run(['ls', '-l', '/nonexistent'], capture_output=True, text=True)
print('stdout:', result.stdout)
print('stderr:', result.stderr)
在这个示例中,我们尝试列出一个不存在的目录,因此会生成错误信息。我们使用 result.stderr
来获取标准错误输出。
3、设置超时
有时我们需要设置命令的执行时间,以防止它无限期地运行。我们可以使用 timeout
参数来实现:
import subprocess
try:
result = subprocess.run(['sleep', '10'], timeout=5)
except subprocess.TimeoutExpired:
print('Command timed out')
在这个示例中,sleep 10
命令被设置为 5 秒的超时,因此会引发 subprocess.TimeoutExpired
异常。
二、OS 模块
os 模块是 Python 标准库的一部分,也可以用于执行 Shell 命令和脚本。尽管不如 subprocess 模块强大,但在一些简单的场景中仍然非常有用。
1、使用 os.system() 方法
os.system() 是一个简单的方法,用于执行 Shell 命令。它直接在命令行中运行命令,并返回命令的退出状态:
import os
exit_status = os.system('ls -l')
print('Exit status:', exit_status)
需要注意的是,os.system() 不会捕获命令的输出,因此它更适合用于不需要获取输出的简单命令。
2、使用 os.popen() 方法
os.popen() 方法可以打开一个管道,从中读取命令的输出:
import os
with os.popen('ls -l') as pipe:
output = pipe.read()
print(output)
在这个示例中,我们使用 os.popen() 打开一个管道,并读取 ls -l
命令的输出。
三、直接调用 Shell 命令
除了使用 subprocess 和 os 模块,我们还可以通过直接调用 Shell 命令来执行脚本。例如,使用 Python 的 !
运算符(在 Jupyter Notebook 中):
!ls -l
这个方法非常简单,但仅适用于交互式环境(如 Jupyter Notebook)。
四、综合示例
以下是一个综合示例,展示了如何使用 subprocess 模块来执行一个 Shell 脚本,并处理其输出和错误:
import subprocess
def run_shell_script(script_path):
try:
result = subprocess.run(['bash', script_path], capture_output=True, text=True, timeout=10)
print('stdout:', result.stdout)
print('stderr:', result.stderr)
print('Exit status:', result.returncode)
except subprocess.TimeoutExpired:
print('Command timed out')
except Exception as e:
print(f'An error occurred: {e}')
执行一个示例脚本
run_shell_script('example.sh')
在这个示例中,我们定义了一个函数 run_shell_script()
,用于执行指定路径的 Shell 脚本,并处理其输出、错误和超时情况。
五、总结
通过以上方法,Python 程序员可以灵活地使用 Shell 脚本来完成各种任务。subprocess 模块 提供了最强大的功能和控制选项,是执行 Shell 命令和脚本的首选工具。os 模块 也可以在一些简单的场景中使用。希望这篇文章能帮助你更好地理解如何在 Python 中使用 Shell 脚本,并为你的项目提供有用的参考。
六、扩展阅读
1、使用 subprocess.Popen() 方法
subprocess.Popen() 方法提供了更细粒度的控制,可以异步执行命令并与其交互。以下是一个基本示例:
import subprocess
process = subprocess.Popen(['ls', '-l'], stdout=subprocess.PIPE, stderr=subprocess.PIPE, text=True)
stdout, stderr = process.communicate()
print('stdout:', stdout)
print('stderr:', stderr)
print('Exit status:', process.returncode)
在这个示例中,subprocess.Popen()
创建了一个新的子进程,并使用 communicate()
方法与其交互。stdout
和 stderr
分别捕获标准输出和错误,process.returncode
获取进程的退出状态。
2、传递输入到子进程
有时我们需要将输入传递给子进程,可以使用 stdin
参数和 communicate()
方法:
import subprocess
process = subprocess.Popen(['grep', 'hello'], stdin=subprocess.PIPE, stdout=subprocess.PIPE, text=True)
stdout, stderr = process.communicate(input='hello world\nhello python\n')
print('stdout:', stdout)
print('stderr:', stderr)
print('Exit status:', process.returncode)
在这个示例中,我们使用 stdin=subprocess.PIPE
参数创建一个管道,并通过 communicate()
方法将输入传递给 grep
命令。
3、处理长时间运行的命令
对于长时间运行的命令,可以使用 subprocess.Popen()
方法并手动处理其输出。以下是一个示例:
import subprocess
process = subprocess.Popen(['ping', 'google.com'], stdout=subprocess.PIPE, stderr=subprocess.PIPE, text=True)
try:
while True:
output = process.stdout.readline()
if output == '' and process.poll() is not None:
break
if output:
print(output.strip())
except KeyboardInterrupt:
process.terminate()
print('Process terminated')
在这个示例中,我们使用 while True
循环读取 ping
命令的输出,并在用户中断时终止进程。
七、应用场景
1、自动化运维
在自动化运维中,Python 脚本可以用来执行各种 Shell 命令,如文件操作、系统监控和服务管理。例如,可以编写一个脚本来定期备份数据库:
import subprocess
import datetime
def backup_database():
timestamp = datetime.datetime.now().strftime('%Y%m%d%H%M%S')
backup_file = f'database_backup_{timestamp}.sql'
try:
result = subprocess.run(['pg_dump', 'mydatabase', '-f', backup_file], capture_output=True, text=True)
if result.returncode == 0:
print(f'Backup successful: {backup_file}')
else:
print(f'Backup failed: {result.stderr}')
except Exception as e:
print(f'An error occurred: {e}')
backup_database()
2、数据处理
在数据处理中,Python 脚本可以调用 Shell 命令来处理大规模数据。例如,可以使用 grep
和 awk
命令过滤和处理日志文件:
import subprocess
def process_log_file(log_file):
try:
grep_process = subprocess.Popen(['grep', 'ERROR', log_file], stdout=subprocess.PIPE, text=True)
awk_process = subprocess.Popen(['awk', '{print $1, $2, $3}'], stdin=grep_process.stdout, stdout=subprocess.PIPE, text=True)
grep_process.stdout.close()
output, _ = awk_process.communicate()
print(output)
except Exception as e:
print(f'An error occurred: {e}')
process_log_file('system.log')
3、集成测试
在集成测试中,Python 脚本可以调用 Shell 命令来启动和管理测试环境。例如,可以使用 Docker 命令启动测试容器:
import subprocess
def start_test_container(image_name):
try:
result = subprocess.run(['docker', 'run', '-d', '--name', 'test_container', image_name], capture_output=True, text=True)
if result.returncode == 0:
print(f'Container started: {result.stdout.strip()}')
else:
print(f'Failed to start container: {result.stderr}')
except Exception as e:
print(f'An error occurred: {e}')
start_test_container('mytestimage')
通过这些示例和应用场景,可以看到 Python 与 Shell 脚本的结合在各种任务中具有很大的灵活性和实用性。希望这篇文章能为你提供更多的灵感和帮助,让你在实际项目中更好地使用 Python 和 Shell 脚本。
相关问答FAQs:
在Python中如何调用Shell脚本?
您可以使用Python的subprocess
模块来调用Shell脚本。通过subprocess.run()
或者subprocess.Popen()
函数,您能够执行Shell命令或脚本,并获取其输出。比如,subprocess.run(['sh', 'your_script.sh'])
可以执行名为your_script.sh
的Shell脚本。
Python与Shell脚本结合的最佳实践有哪些?
结合Python与Shell脚本时,保持代码清晰和模块化是非常重要的。尽量将复杂的逻辑放在Python中处理,而将简单的系统任务留给Shell脚本。此外,确保在Shell脚本中添加适当的错误处理,以便在Python中能捕获到问题并进行相应处理。
Python脚本和Shell脚本的性能对比如何?
性能方面,Shell脚本通常在处理系统命令时更为高效,因为它们是直接在Shell环境中运行的。而Python在处理复杂的数据结构和逻辑时表现更佳。根据具体任务的需求,您可以选择将两者结合使用,以获得最优的性能和可维护性。
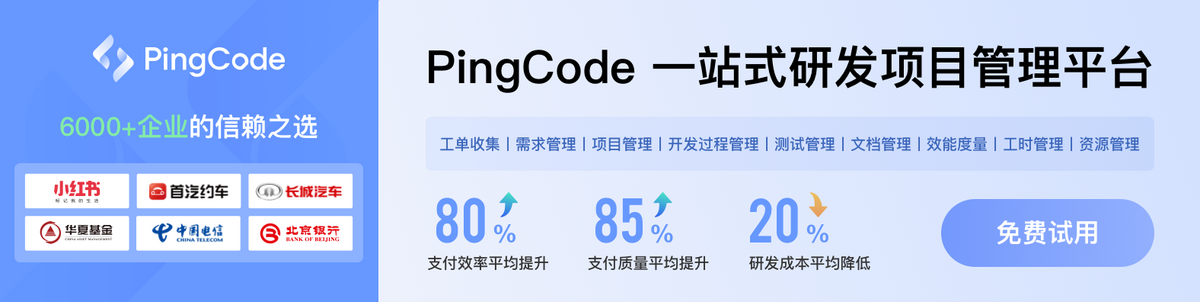