Python读取PCI卡的方法有:使用现有的驱动库、利用C扩展模块、通过系统命令读取信息。其中,使用现有的驱动库是一种较为简单和高效的方法。
使用现有的驱动库详细描述
现有的驱动库如pyPCI
, pyPCIe
等提供了方便的接口来读取和操作PCI卡。这些库封装好了底层的系统调用,使得用户可以通过Python代码直接访问PCI卡的数据和功能。
例如,使用pyPCI
库可以快速读取PCI卡的配置信息:
import pyPCI
初始化PCI
pci = pyPCI.PCI()
获取所有PCI设备信息
devices = pci.get_devices()
输出设备信息
for device in devices:
print(f"Device: {device['name']}, Vendor: {device['vendor']}, Device ID: {device['device_id']}")
以上代码展示了如何使用pyPCI
库来获取并打印所有PCI设备的信息。通过这种方式,用户可以很方便地对PCI卡进行检测和读取。
一、使用现有的驱动库
pyPCI库
pyPCI
库是一个用于访问和操作PCI卡的Python库。它提供了一组API,可以方便地读取和写入PCI卡的配置信息。这个库的优点是易于使用,适合快速开发和测试。
安装pyPCI
库:
pip install pyPCI
使用pyPCI
库读取PCI卡信息的示例代码:
import pyPCI
初始化PCI
pci = pyPCI.PCI()
获取所有PCI设备信息
devices = pci.get_devices()
输出设备信息
for device in devices:
print(f"Device: {device['name']}, Vendor: {device['vendor']}, Device ID: {device['device_id']}")
以上代码展示了如何使用pyPCI
库来获取并打印所有PCI设备的信息。通过这种方式,用户可以很方便地对PCI卡进行检测和读取。
pyPCIe库
pyPCIe
库是另一个用于访问和操作PCIe卡的Python库。它提供了更丰富的功能,适合复杂的PCIe卡操作。
安装pyPCIe
库:
pip install pyPCIe
使用pyPCIe
库读取PCIe卡信息的示例代码:
import pyPCIe
初始化PCIe
pcie = pyPCIe.PCIe()
获取所有PCIe设备信息
devices = pcie.get_devices()
输出设备信息
for device in devices:
print(f"Device: {device['name']}, Vendor: {device['vendor']}, Device ID: {device['device_id']}")
以上代码展示了如何使用pyPCIe
库来获取并打印所有PCIe设备的信息。通过这种方式,用户可以对PCIe卡进行更加复杂的操作。
二、利用C扩展模块
编写C扩展模块
当现有的驱动库不能满足需求时,可以考虑编写C扩展模块来访问PCI卡。通过这种方式,可以直接调用底层的系统API,从而实现对PCI卡的精细控制。
编写一个简单的C扩展模块来读取PCI卡信息:
#include <Python.h>
#include <pci/pci.h>
static PyObject* get_pci_devices(PyObject* self, PyObject* args) {
struct pci_access *pacc;
struct pci_dev *dev;
char namebuf[1024], *name;
pacc = pci_alloc();
pci_init(pacc);
pci_scan_bus(pacc);
PyObject* device_list = PyList_New(0);
for (dev = pacc->devices; dev; dev = dev->next) {
pci_fill_info(dev, PCI_FILL_IDENT | PCI_FILL_BASES | PCI_FILL_CLASS);
name = pci_lookup_name(pacc, namebuf, sizeof(namebuf), PCI_LOOKUP_DEVICE, dev->vendor_id, dev->device_id);
PyObject* device_info = Py_BuildValue("{s:s, s:s, s:i}",
"name", name,
"vendor", pci_lookup_name(pacc, namebuf, sizeof(namebuf), PCI_LOOKUP_VENDOR, dev->vendor_id),
"device_id", dev->device_id);
PyList_Append(device_list, device_info);
}
pci_cleanup(pacc);
return device_list;
}
static PyMethodDef PciMethods[] = {
{"get_pci_devices", get_pci_devices, METH_VARARGS, "Get PCI devices information."},
{NULL, NULL, 0, NULL}
};
static struct PyModuleDef pcimodule = {
PyModuleDef_HEAD_INIT,
"pci",
NULL,
-1,
PciMethods
};
PyMODINIT_FUNC PyInit_pci(void) {
return PyModule_Create(&pcimodule);
}
以上代码展示了如何编写一个简单的C扩展模块来获取PCI卡的信息。通过这种方式,可以直接调用底层的系统API,从而实现对PCI卡的精细控制。
编译和使用C扩展模块
将上述C代码保存为pci.c
文件,然后编写setup.py
文件来编译这个C扩展模块:
from setuptools import setup, Extension
module = Extension('pci', sources=['pci.c'], libraries=['pci'])
setup(name='pci',
version='1.0',
description='Python interface to read PCI cards',
ext_modules=[module])
使用以下命令编译并安装C扩展模块:
python setup.py build
python setup.py install
安装完成后,就可以在Python中使用这个C扩展模块来读取PCI卡信息:
import pci
devices = pci.get_pci_devices()
for device in devices:
print(f"Device: {device['name']}, Vendor: {device['vendor']}, Device ID: {device['device_id']}")
以上代码展示了如何使用编写的C扩展模块来获取并打印所有PCI设备的信息。通过这种方式,可以直接调用底层的系统API,从而实现对PCI卡的精细控制。
三、通过系统命令读取信息
lspci命令
在Linux系统中,可以通过lspci
命令来读取PCI卡的信息。lspci
命令是一个常用的Linux命令,用于显示所有PCI设备的信息。
使用subprocess
模块在Python中调用lspci
命令:
import subprocess
def get_pci_devices():
result = subprocess.run(['lspci'], stdout=subprocess.PIPE)
return result.stdout.decode('utf-8')
devices = get_pci_devices()
print(devices)
以上代码展示了如何在Python中调用lspci
命令来获取并打印所有PCI设备的信息。通过这种方式,可以非常方便地获取到PCI卡的详细信息。
解析lspci输出
可以进一步解析lspci
命令的输出,以便更好地处理这些信息:
import subprocess
def get_pci_devices():
result = subprocess.run(['lspci', '-vmm'], stdout=subprocess.PIPE)
lines = result.stdout.decode('utf-8').split('\n')
devices = []
device = {}
for line in lines:
if line.strip() == '':
if device:
devices.append(device)
device = {}
else:
key, value = line.split('\t', 1)
device[key] = value
return devices
devices = get_pci_devices()
for device in devices:
print(f"Device: {device['Slot']}, Vendor: {device['Vendor']}, Device: {device['Device']}, Class: {device['Class']}")
以上代码展示了如何进一步解析lspci
命令的输出,以便更好地处理这些信息。通过这种方式,可以非常方便地获取到PCI卡的详细信息。
四、总结
使用Python读取PCI卡的信息有多种方法,包括使用现有的驱动库、利用C扩展模块、通过系统命令读取信息等。每种方法都有其优点和适用场景,用户可以根据自己的需求选择合适的方法。
使用现有的驱动库(如pyPCI
, pyPCIe
)提供了方便的接口,适合快速开发和测试;利用C扩展模块可以直接调用底层的系统API,适合需要精细控制的场景;通过系统命令读取信息(如lspci
命令)是一种简单而有效的方法,适合在Linux系统中使用。
无论采用哪种方法,最终的目的是能够准确、快捷地读取PCI卡的信息,从而为进一步的开发和调试提供支持。
相关问答FAQs:
如何在Python中识别和列出所有PCI设备?
要在Python中识别和列出所有PCI设备,可以使用lspci
命令结合Python的subprocess
模块。通过执行lspci
命令,您可以获取系统中所有PCI设备的信息,并在Python中处理这些信息。以下是一个示例代码:
import subprocess
def list_pci_devices():
result = subprocess.run(['lspci'], stdout=subprocess.PIPE)
devices = result.stdout.decode('utf-8').strip().split('\n')
for device in devices:
print(device)
list_pci_devices()
执行此代码将打印出所有PCI设备的列表。
如何在Python中访问特定的PCI设备信息?
要访问特定的PCI设备信息,可以通过lspci -v
命令获取更详细的信息,并结合Python进行解析。您可以通过设备ID或其他标识符来筛选所需的设备。例如:
def get_pci_device_info(device_id):
result = subprocess.run(['lspci', '-v', '-s', device_id], stdout=subprocess.PIPE)
info = result.stdout.decode('utf-8').strip()
return info
device_id = '00:1f.2' # 替换为所需设备的ID
print(get_pci_device_info(device_id))
这种方法可以帮助您获取有关特定PCI设备的详细信息。
Python如何与PCI设备进行通信?
与PCI设备进行通信通常需要使用特定的库或API,具体取决于设备的类型和功能。比如,如果您在处理网络PCI卡,可能需要使用pyroute2
库来发送和接收网络数据包。对于其他类型的PCI设备,您可能需要查找相应的Python库或驱动程序。例如:
from pyroute2 import IPRoute
ip = IPRoute()
# 示例:列出所有网络接口
interfaces = ip.get_links()
for interface in interfaces:
print(interface)
在使用这些库之前,请确保您已经正确安装了相关依赖,并了解设备的通信协议。
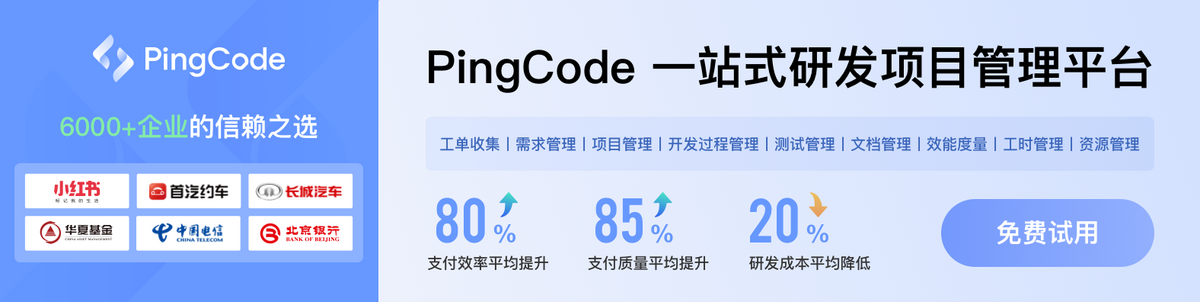