要生成PDF报告,可以使用Python中的多个库,如ReportLab、FPDF、WeasyPrint、Pandas。这些库各自有不同的特点和适用场景。下面将详细介绍其中一种使用方法,以帮助你更好地理解和应用。
一、使用ReportLab生成PDF报告
ReportLab是一个功能强大且灵活的库,适用于需要生成复杂布局和高质量PDF的场景。
安装ReportLab
首先,确保你已经安装了ReportLab库。你可以使用pip进行安装:
pip install reportlab
基本示例
下面是一个使用ReportLab生成简单PDF的示例:
from reportlab.lib.pagesizes import letter
from reportlab.pdfgen import canvas
def create_pdf(output_filename):
c = canvas.Canvas(output_filename, pagesize=letter)
width, height = letter
# 添加标题
c.setFont("Helvetica-Bold", 24)
c.drawString(100, height - 100, "PDF报告标题")
# 添加正文
c.setFont("Helvetica", 12)
text = "这是PDF报告的正文内容。这是一个示例,用于展示如何使用ReportLab生成PDF文件。"
c.drawString(100, height - 150, text)
# 保存PDF
c.save()
create_pdf("example_report.pdf")
添加图片和表格
可以通过以下代码添加图片和表格到PDF中:
from reportlab.lib import colors
from reportlab.lib.pagesizes import letter
from reportlab.platypus import SimpleDocTemplate, Table, TableStyle, Image
def create_pdf_with_image_and_table(output_filename):
doc = SimpleDocTemplate(output_filename, pagesize=letter)
elements = []
# 添加图片
img = Image("example_image.png")
img.drawHeight = 2 * inch
img.drawWidth = 2 * inch
elements.append(img)
# 添加表格
data = [
['Header 1', 'Header 2', 'Header 3'],
['Row 1 Column 1', 'Row 1 Column 2', 'Row 1 Column 3'],
['Row 2 Column 1', 'Row 2 Column 2', 'Row 2 Column 3']
]
table = Table(data)
table.setStyle(TableStyle([
('BACKGROUND', (0, 0), (-1, 0), colors.gray),
('TEXTCOLOR', (0, 0), (-1, 0), colors.whitesmoke),
('ALIGN', (0, 0), (-1, -1), 'CENTER'),
('FONTNAME', (0, 0), (-1, 0), 'Helvetica-Bold'),
('BOTTOMPADDING', (0, 0), (-1, 0), 12),
('BACKGROUND', (0, 1), (-1, -1), colors.beige),
('GRID', (0, 0), (-1, -1), 1, colors.black)
]))
elements.append(table)
# 构建PDF
doc.build(elements)
create_pdf_with_image_and_table("example_report_with_image_and_table.pdf")
二、使用FPDF生成PDF报告
FPDF是另一个流行的库,适用于需要快速生成简单PDF文件的场景。
安装FPDF
首先,确保你已经安装了FPDF库。你可以使用pip进行安装:
pip install fpdf
基本示例
下面是一个使用FPDF生成简单PDF的示例:
from fpdf import FPDF
class PDF(FPDF):
def header(self):
self.set_font('Arial', 'B', 12)
self.cell(0, 10, 'PDF报告标题', 0, 1, 'C')
def footer(self):
self.set_y(-15)
self.set_font('Arial', 'I', 8)
self.cell(0, 10, f'Page {self.page_no()}', 0, 0, 'C')
def chapter_title(self, title):
self.set_font('Arial', 'B', 12)
self.cell(0, 10, title, 0, 1, 'L')
self.ln(10)
def chapter_body(self, body):
self.set_font('Arial', '', 12)
self.multi_cell(0, 10, body)
self.ln()
pdf = PDF()
pdf.add_page()
pdf.chapter_title('第一章')
pdf.chapter_body('这是PDF报告的正文内容。这是一个示例,用于展示如何使用FPDF生成PDF文件。')
pdf.output('example_report.pdf')
添加图片和表格
可以通过以下代码添加图片和表格到PDF中:
class PDF(FPDF):
def header(self):
self.set_font('Arial', 'B', 12)
self.cell(0, 10, 'PDF报告标题', 0, 1, 'C')
def footer(self):
self.set_y(-15)
self.set_font('Arial', 'I', 8)
self.cell(0, 10, f'Page {self.page_no()}', 0, 0, 'C')
def chapter_title(self, title):
self.set_font('Arial', 'B', 12)
self.cell(0, 10, title, 0, 1, 'L')
self.ln(10)
def chapter_body(self, body):
self.set_font('Arial', '', 12)
self.multi_cell(0, 10, body)
self.ln()
def add_image(self, image_path, x, y, w, h):
self.image(image_path, x=x, y=y, w=w, h=h)
def add_table(self, data):
self.set_font('Arial', 'B', 12)
for row in data:
for item in row:
self.cell(40, 10, item, 1)
self.ln()
pdf = PDF()
pdf.add_page()
添加章节
pdf.chapter_title('第一章')
pdf.chapter_body('这是PDF报告的正文内容。这是一个示例,用于展示如何使用FPDF生成PDF文件。')
添加图片
pdf.add_image('example_image.png', 10, 100, 50, 50)
添加表格
data = [
['Header 1', 'Header 2', 'Header 3'],
['Row 1 Column 1', 'Row 1 Column 2', 'Row 1 Column 3'],
['Row 2 Column 1', 'Row 2 Column 2', 'Row 2 Column 3']
]
pdf.add_page()
pdf.add_table(data)
pdf.output('example_report_with_image_and_table.pdf')
三、使用WeasyPrint生成PDF报告
WeasyPrint是一个现代化的工具,可以将HTML和CSS转换为PDF。适用于需要生成复杂布局和支持CSS样式的PDF文件。
安装WeasyPrint
首先,确保你已经安装了WeasyPrint库。你可以使用pip进行安装:
pip install WeasyPrint
基本示例
下面是一个使用WeasyPrint生成简单PDF的示例:
from weasyprint import HTML
html_content = """
<!DOCTYPE html>
<html>
<head>
<title>PDF报告</title>
<style>
body { font-family: Arial, sans-serif; }
h1 { text-align: center; }
p { text-indent: 2em; }
</style>
</head>
<body>
<h1>PDF报告标题</h1>
<p>这是PDF报告的正文内容。这是一个示例,用于展示如何使用WeasyPrint生成PDF文件。</p>
</body>
</html>
"""
HTML(string=html_content).write_pdf("example_report.pdf")
添加图片和表格
可以通过以下代码添加图片和表格到PDF中:
html_content = """
<!DOCTYPE html>
<html>
<head>
<title>PDF报告</title>
<style>
body { font-family: Arial, sans-serif; }
h1 { text-align: center; }
p { text-indent: 2em; }
table { width: 100%; border-collapse: collapse; }
th, td { border: 1px solid black; padding: 8px; text-align: center; }
th { background-color: #f2f2f2; }
</style>
</head>
<body>
<h1>PDF报告标题</h1>
<p>这是PDF报告的正文内容。这是一个示例,用于展示如何使用WeasyPrint生成PDF文件。</p>
<img src="example_image.png" alt="示例图片" width="200" height="200">
<table>
<tr>
<th>Header 1</th>
<th>Header 2</th>
<th>Header 3</th>
</tr>
<tr>
<td>Row 1 Column 1</td>
<td>Row 1 Column 2</td>
<td>Row 1 Column 3</td>
</tr>
<tr>
<td>Row 2 Column 1</td>
<td>Row 2 Column 2</td>
<td>Row 2 Column 3</td>
</tr>
</table>
</body>
</html>
"""
HTML(string=html_content).write_pdf("example_report_with_image_and_table.pdf")
四、使用Pandas生成PDF报告
Pandas是一个强大的数据处理库,通常与其他库结合使用,如ReportLab或FPDF,来生成包含数据表的PDF报告。
安装Pandas
首先,确保你已经安装了Pandas库。你可以使用pip进行安装:
pip install pandas
基本示例
下面是一个使用Pandas和ReportLab结合生成PDF的示例:
import pandas as pd
from reportlab.lib.pagesizes import letter
from reportlab.platypus import SimpleDocTemplate, Table, TableStyle
from reportlab.lib import colors
创建示例数据
data = {
'列1': [1, 2, 3, 4],
'列2': [5, 6, 7, 8],
'列3': [9, 10, 11, 12]
}
df = pd.DataFrame(data)
将DataFrame转换为列表
data_list = [df.columns.to_list()] + df.values.tolist()
创建PDF
doc = SimpleDocTemplate("example_report.pdf", pagesize=letter)
elements = []
创建表格
table = Table(data_list)
table.setStyle(TableStyle([
('BACKGROUND', (0, 0), (-1, 0), colors.gray),
('TEXTCOLOR', (0, 0), (-1, 0), colors.whitesmoke),
('ALIGN', (0, 0), (-1, -1), 'CENTER'),
('FONTNAME', (0, 0), (-1, 0), 'Helvetica-Bold'),
('BOTTOMPADDING', (0, 0), (-1, 0), 12),
('BACKGROUND', (0, 1), (-1, -1), colors.beige),
('GRID', (0, 0), (-1, -1), 1, colors.black)
]))
elements.append(table)
构建PDF
doc.build(elements)
结合Pandas和FPDF
下面是一个使用Pandas和FPDF结合生成PDF的示例:
import pandas as pd
from fpdf import FPDF
创建示例数据
data = {
'列1': [1, 2, 3, 4],
'列2': [5, 6, 7, 8],
'列3': [9, 10, 11, 12]
}
df = pd.DataFrame(data)
class PDF(FPDF):
def header(self):
self.set_font('Arial', 'B', 12)
self.cell(0, 10, 'PDF报告标题', 0, 1, 'C')
def footer(self):
self.set_y(-15)
self.set_font('Arial', 'I', 8)
self.cell(0, 10, f'Page {self.page_no()}', 0, 0, 'C')
def add_table_from_df(self, df):
self.set_font('Arial', 'B', 12)
cols = df.columns
for col in cols:
self.cell(40, 10, col, 1)
self.ln()
self.set_font('Arial', '', 12)
for index, row in df.iterrows():
for item in row:
self.cell(40, 10, str(item), 1)
self.ln()
pdf = PDF()
pdf.add_page()
pdf.add_table_from_df(df)
pdf.output('example_report.pdf')
五、总结
在Python中生成PDF报告的方法很多,每种方法都有其独特的优势和适用场景。ReportLab适用于生成复杂布局和高质量PDF,FPDF适用于快速生成简单PDF,WeasyPrint适用于生成支持CSS样式的复杂布局PDF,Pandas与其他库结合使用则适用于生成包含数据表的PDF报告。根据具体需求选择合适的工具,可以更高效地生成所需的PDF报告。
相关问答FAQs:
如何使用Python库生成PDF报告?
在Python中,有几个流行的库可以帮助用户生成PDF报告。最常用的库包括ReportLab、FPDF和PyPDF2。ReportLab是功能强大的库,能够创建复杂的PDF文档,而FPDF则提供了更简单的接口,适合快速生成基本的PDF文件。用户可以根据需要选择合适的库,并通过编写Python脚本来定义PDF的内容、格式和样式。
生成PDF报告时有哪些常见的格式和样式选择?
在生成PDF报告时,用户可以自定义页面布局、字体、颜色、边距等多种样式。常见的格式包括表格、图表、文本块和图片等。通过设置不同的样式,用户可以制作出更加专业和美观的报告。此外,用户还可以添加页眉、页脚和水印,以提高报告的可读性和美观度。
如何在Python中处理PDF报告的动态数据?
处理动态数据生成PDF报告时,用户可以通过读取数据库、CSV文件或API获取数据。在生成PDF之前,用户可以使用Pandas等库进行数据处理和分析。将处理后的数据整合到PDF报告中,确保报告能够实时反映数据的变化,从而提供更具价值的信息给读者。
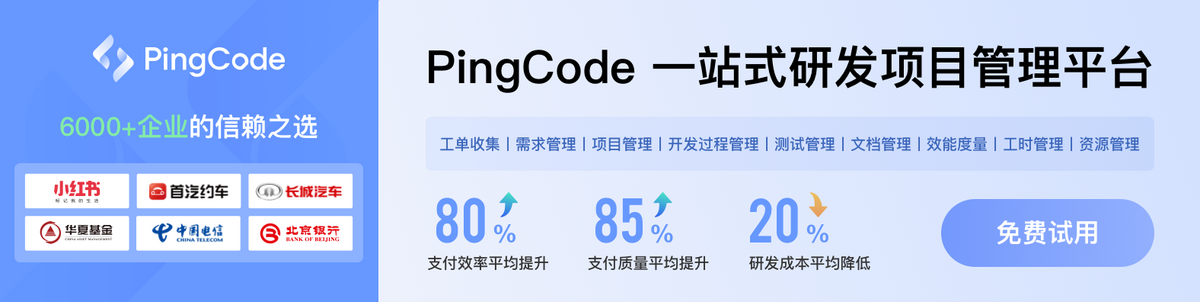