Python中的对象方法是指在类定义中绑定到对象实例的方法。对象方法可以访问实例变量,并且可以通过调用它们来操作这些变量。理解对象方法的关键点包括:对象方法是绑定到实例的、使用self参数访问实例变量、可以通过实例调用。以下将详细描述其中一个关键点,即如何通过实例调用对象方法。
通过实例调用对象方法:在Python中,对象方法是定义在类中的函数,并且通常接收一个特殊的第一个参数self,这个参数是对调用该方法的对象实例的引用。当你创建一个类的实例时,你可以通过该实例调用类中的方法。例如:
class Dog:
def __init__(self, name):
self.name = name
def bark(self):
print(f"{self.name} says woof!")
创建一个Dog类的实例
my_dog = Dog("Buddy")
调用对象方法
my_dog.bark() # 输出:Buddy says woof!
在这个例子中,bark
方法是绑定到Dog
类实例的对象方法,并且可以通过实例my_dog
调用。
一、对象方法的定义和调用
对象方法是定义在类内部的函数,它们以第一个参数self
开始。self
参数在方法中表示实例本身,因此可以通过self
访问实例变量和其他方法。
class Car:
def __init__(self, model, year):
self.model = model
self.year = year
def display_info(self):
print(f"Car model: {self.model}, Year: {self.year}")
创建一个Car类的实例
my_car = Car("Toyota Camry", 2020)
调用对象方法
my_car.display_info() # 输出:Car model: Toyota Camry, Year: 2020
在这个例子中,display_info
方法通过self
参数访问实例变量model
和year
,并在调用时输出它们的值。
二、对象方法与类方法、静态方法的区别
在Python中,除了对象方法,还有类方法和静态方法。它们之间的区别在于:
- 对象方法:使用
self
参数,绑定到实例,可以访问和修改实例变量。 - 类方法:使用
cls
参数,绑定到类本身,通过@classmethod
装饰器定义,只能访问类变量和类方法。 - 静态方法:不使用
self
或cls
参数,通过@staticmethod
装饰器定义,不绑定到实例或类,只是类命名空间中的普通函数。
class Example:
class_variable = "Class Variable"
def __init__(self, instance_variable):
self.instance_variable = instance_variable
def instance_method(self):
print(f"Instance method called with {self.instance_variable}")
@classmethod
def class_method(cls):
print(f"Class method called with {cls.class_variable}")
@staticmethod
def static_method():
print("Static method called")
创建实例
example = Example("Instance Variable")
调用对象方法
example.instance_method() # 输出:Instance method called with Instance Variable
调用类方法
Example.class_method() # 输出:Class method called with Class Variable
调用静态方法
Example.static_method() # 输出:Static method called
三、对象方法的实际应用
对象方法在面向对象编程中非常重要,因为它们允许类的实例执行操作和行为。以下是一些实际应用:
- 操作实例数据:对象方法可以操作和修改实例变量,从而影响实例的状态。
class BankAccount:
def __init__(self, balance=0):
self.balance = balance
def deposit(self, amount):
self.balance += amount
print(f"Deposited {amount}, new balance is {self.balance}")
def withdraw(self, amount):
if amount <= self.balance:
self.balance -= amount
print(f"Withdrew {amount}, new balance is {self.balance}")
else:
print("Insufficient funds")
创建一个BankAccount类的实例
account = BankAccount(100)
调用对象方法
account.deposit(50) # 输出:Deposited 50, new balance is 150
account.withdraw(20) # 输出:Withdrew 20, new balance is 130
- 封装复杂逻辑:对象方法可以封装复杂的逻辑和行为,使代码更具模块化和可维护性。
class ShoppingCart:
def __init__(self):
self.items = []
def add_item(self, item, price):
self.items.append({"item": item, "price": price})
print(f"Added {item} to the cart")
def total_price(self):
total = sum(item["price"] for item in self.items)
print(f"Total price is {total}")
return total
创建一个ShoppingCart类的实例
cart = ShoppingCart()
调用对象方法
cart.add_item("Apple", 1.5) # 输出:Added Apple to the cart
cart.add_item("Banana", 2.0) # 输出:Added Banana to the cart
cart.total_price() # 输出:Total price is 3.5
四、对象方法的高级用法
除了基本的定义和调用,对象方法还有一些高级用法,可以更好地利用Python的特性和功能。
- 私有方法:使用双下划线前缀定义私有方法,使其只能在类内部访问。
class Example:
def __init__(self, data):
self.data = data
def public_method(self):
print("Public method")
self.__private_method()
def __private_method(self):
print("Private method")
创建一个Example类的实例
example = Example("data")
调用公共方法
example.public_method() # 输出:Public method followed by Private method
尝试调用私有方法(会报错)
example.__private_method() # AttributeError: 'Example' object has no attribute '__private_method'
- 魔术方法:特殊的对象方法,以双下划线开头和结尾,用于实现特定的行为,如运算符重载、对象表示等。
class Vector:
def __init__(self, x, y):
self.x = x
self.y = y
def __add__(self, other):
return Vector(self.x + other.x, self.y + other.y)
def __repr__(self):
return f"Vector({self.x}, {self.y})"
创建两个Vector类的实例
v1 = Vector(2, 3)
v2 = Vector(4, 5)
使用重载的+运算符
v3 = v1 + v2
输出结果
print(v3) # 输出:Vector(6, 8)
五、对象方法的最佳实践
在实际开发中,遵循一些最佳实践可以使对象方法的定义和使用更加规范和高效。
- 明确方法职责:每个对象方法应有明确的职责,避免方法过于复杂和庞大。
class Order:
def __init__(self, items):
self.items = items
def calculate_total(self):
return sum(item["price"] for item in self.items)
def add_item(self, item, price):
self.items.append({"item": item, "price": price})
def print_receipt(self):
for item in self.items:
print(f"{item['item']}: ${item['price']}")
print(f"Total: ${self.calculate_total()}")
创建一个Order类的实例
order = Order([])
调用对象方法
order.add_item("Laptop", 1200)
order.add_item("Mouse", 25)
order.print_receipt()
- 使用合适的访问控制:根据方法的用途选择适当的访问控制级别,公有方法、私有方法或保护方法(单下划线前缀)。
class Example:
def __init__(self, data):
self.data = data
def public_method(self):
print("Public method")
self._protected_method()
def _protected_method(self):
print("Protected method")
def __private_method(self):
print("Private method")
创建一个Example类的实例
example = Example("data")
调用公共方法
example.public_method() # 输出:Public method followed by Protected method
调用保护方法(可以访问,但不推荐)
example._protected_method() # 输出:Protected method
尝试调用私有方法(会报错)
example.__private_method() # AttributeError: 'Example' object has no attribute '__private_method'
- 保持方法简洁:避免方法中包含过多逻辑,保持方法简洁明了,易于理解和维护。
class User:
def __init__(self, username, password):
self.username = username
self.password = password
def authenticate(self, input_username, input_password):
if self.username == input_username and self.password == input_password:
print("Authentication successful")
else:
print("Authentication failed")
创建一个User类的实例
user = User("admin", "secret")
调用对象方法
user.authenticate("admin", "secret") # 输出:Authentication successful
user.authenticate("admin", "wrong") # 输出:Authentication failed
六、对象方法在设计模式中的应用
在软件设计模式中,对象方法扮演着重要的角色,不同的设计模式利用对象方法来实现特定的设计原则和结构。
- 单例模式:确保一个类只有一个实例,并提供一个全局访问点。
class Singleton:
_instance = None
def __new__(cls, *args, kwargs):
if cls._instance is None:
cls._instance = super(Singleton, cls).__new__(cls, *args, kwargs)
return cls._instance
def __init__(self, value):
self.value = value
创建两个Singleton类的实例
s1 = Singleton("First")
s2 = Singleton("Second")
检查是否是同一个实例
print(s1 is s2) # 输出:True
print(s1.value) # 输出:Second
print(s2.value) # 输出:Second
- 工厂模式:定义一个创建对象的接口,但让子类决定实例化哪个类。
class Animal:
def speak(self):
raise NotImplementedError("Subclasses must implement this method")
class Dog(Animal):
def speak(self):
return "Woof!"
class Cat(Animal):
def speak(self):
return "Meow!"
class AnimalFactory:
def create_animal(self, animal_type):
if animal_type == "dog":
return Dog()
elif animal_type == "cat":
return Cat()
else:
return None
创建AnimalFactory类的实例
factory = AnimalFactory()
使用工厂创建动物实例
dog = factory.create_animal("dog")
cat = factory.create_animal("cat")
调用对象方法
print(dog.speak()) # 输出:Woof!
print(cat.speak()) # 输出:Meow!
- 观察者模式:定义对象间的一对多依赖关系,当一个对象改变状态时,其所有依赖者都会收到通知并自动更新。
class Subject:
def __init__(self):
self._observers = []
def attach(self, observer):
self._observers.append(observer)
def detach(self, observer):
self._observers.remove(observer)
def notify(self, message):
for observer in self._observers:
observer.update(message)
class Observer:
def update(self, message):
raise NotImplementedError("Subclasses must implement this method")
class ConcreteObserver(Observer):
def update(self, message):
print(f"Received message: {message}")
创建Subject类的实例
subject = Subject()
创建ConcreteObserver类的实例
observer1 = ConcreteObserver()
observer2 = ConcreteObserver()
订阅观察者
subject.attach(observer1)
subject.attach(observer2)
通知观察者
subject.notify("Hello, Observers!")
七、对象方法的测试与调试
在开发过程中,测试和调试对象方法是确保代码质量的重要环节。Python提供了丰富的工具和框架来帮助开发者测试和调试对象方法。
- 单元测试:使用unittest模块编写和运行测试用例,确保对象方法按预期工作。
import unittest
class TestBankAccount(unittest.TestCase):
def test_deposit(self):
account = BankAccount(100)
account.deposit(50)
self.assertEqual(account.balance, 150)
def test_withdraw(self):
account = BankAccount(100)
account.withdraw(20)
self.assertEqual(account.balance, 80)
def test_insufficient_funds(self):
account = BankAccount(100)
account.withdraw(200)
self.assertEqual(account.balance, 100)
if __name__ == "__main__":
unittest.main()
- 调试:使用print语句或调试器(如pdb)来检查对象方法的执行流程和变量状态。
import pdb
class Calculator:
def add(self, a, b):
pdb.set_trace() # 设置断点
return a + b
创建一个Calculator类的实例
calc = Calculator()
调用对象方法并进入调试模式
result = calc.add(2, 3)
print(result)
通过遵循这些最佳实践和利用工具,可以更好地理解、定义和使用Python中的对象方法,提高代码的可读性和可维护性。
相关问答FAQs:
什么是Python中的对象方法?
对象方法是与类的实例(对象)相关联的函数。在Python中,这些方法可以访问和操作对象的属性。对象方法通常以self
作为第一个参数,表示调用该方法的对象自身。通过对象方法,您可以实现更复杂的逻辑,并使代码更具可重用性和组织性。
如何定义和调用对象方法?
在Python中,可以通过在类中定义一个函数来创建对象方法。定义方法时,使用def
关键字,然后在类中调用该方法时,只需使用对象名加上方法名即可。例如:
class MyClass:
def my_method(self):
print("这是一个对象方法")
obj = MyClass()
obj.my_method() # 输出: 这是一个对象方法
这样,您就成功定义并调用了一个对象方法。
对象方法与类方法有什么区别?
对象方法和类方法的主要区别在于它们的调用方式和访问范围。对象方法通过实例调用,能够访问实例的属性和其他实例方法。而类方法则是通过类调用,使用@classmethod
装饰器定义,并以cls
作为第一个参数,通常用于处理与类相关的逻辑,而不是特定的实例。了解这两者的区别可以帮助您更好地设计类的结构和功能。
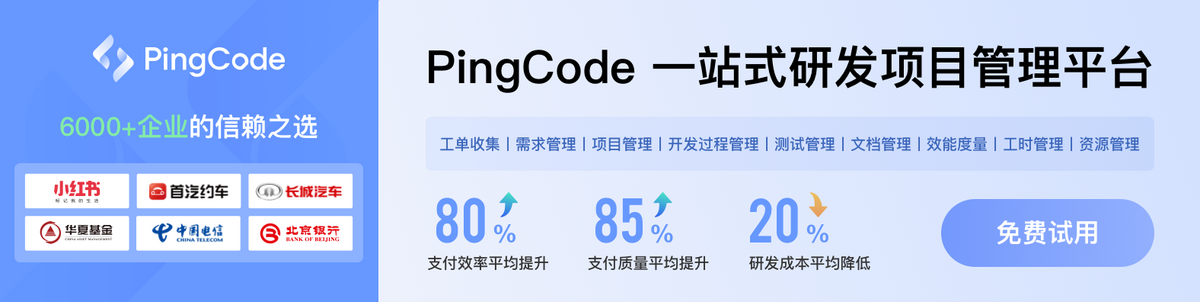