使用Python爬取信息需要以下几个步骤:安装必要的库、设置请求头、解析HTML页面、处理数据。首先,安装必要的库,如requests和BeautifulSoup。然后,设置请求头以模拟浏览器行为,避免被网站拒绝访问。接着,使用requests库发送HTTP请求获取网页内容,并使用BeautifulSoup解析HTML页面。最后,处理和保存所需的数据。例如,解析HTML页面是一个关键步骤。在这一过程中,使用BeautifulSoup库将HTML文档转换为一个便于操作的BeautifulSoup对象,可以轻松地搜索和提取所需的数据。
一、安装必要的库
要使用Python进行网页爬取,首先需要安装一些必要的库。常用的库包括requests、BeautifulSoup和lxml。requests库用于发送HTTP请求,BeautifulSoup库用于解析HTML文档,而lxml库则提供了高效的XML和HTML解析功能。
pip install requests
pip install beautifulsoup4
pip install lxml
安装这些库后,就可以开始进行网页爬取了。
二、设置请求头
许多网站会检测来自爬虫的请求,并可能会拒绝这些请求。为了避免这种情况,我们可以设置请求头,以模拟浏览器的行为。请求头中包含了浏览器的信息,如User-Agent、Referer等,这样可以让服务器认为请求是来自真实的用户。
import requests
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/91.0.4472.124 Safari/537.36',
'Referer': 'https://www.example.com'
}
response = requests.get('https://www.example.com', headers=headers)
三、发送HTTP请求
使用requests库发送HTTP请求,获取网页的内容。可以使用get方法发送GET请求,使用post方法发送POST请求。这里以GET请求为例。
url = 'https://www.example.com'
response = requests.get(url, headers=headers)
if response.status_code == 200:
html_content = response.text
print(html_content)
else:
print(f'Failed to retrieve the webpage. Status code: {response.status_code}')
四、解析HTML页面
获取到网页的内容后,需要解析HTML页面,以提取所需的数据。可以使用BeautifulSoup库进行解析。首先,将HTML内容转换为BeautifulSoup对象,然后使用BeautifulSoup提供的方法进行搜索和提取。
from bs4 import BeautifulSoup
soup = BeautifulSoup(html_content, 'html.parser')
title = soup.title.string
print(f'Title: {title}')
提取所有的链接
links = soup.find_all('a')
for link in links:
print(link.get('href'))
五、处理和保存数据
提取到所需的数据后,可以对数据进行处理和保存。可以将数据保存到CSV文件、数据库或其他存储介质中。这里以将数据保存到CSV文件为例。
import csv
data = []
for link in links:
data.append(link.get('href'))
with open('links.csv', 'w', newline='') as file:
writer = csv.writer(file)
writer.writerow(['Link'])
for item in data:
writer.writerow([item])
六、处理动态网页
有些网页是通过JavaScript动态生成内容的,使用requests库无法直接获取到这些内容。此时,可以使用Selenium库模拟浏览器行为,以获取动态生成的内容。
pip install selenium
安装Selenium库后,还需要下载相应的浏览器驱动程序,如ChromeDriver。然后,可以使用Selenium模拟浏览器行为,获取动态生成的内容。
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.chrome.service import Service
from webdriver_manager.chrome import ChromeDriverManager
设置Chrome选项
chrome_options = webdriver.ChromeOptions()
chrome_options.add_argument('--headless') # 无头模式
初始化Chrome驱动
service = Service(ChromeDriverManager().install())
driver = webdriver.Chrome(service=service, options=chrome_options)
打开网页
driver.get('https://www.example.com')
获取页面内容
html_content = driver.page_source
driver.quit()
解析HTML页面
soup = BeautifulSoup(html_content, 'html.parser')
title = soup.title.string
print(f'Title: {title}')
七、处理分页
在爬取信息时,常常会遇到分页的情况。为了爬取所有页面的信息,需要处理分页。可以在循环中发送多个请求,并逐页解析和提取数据。
page_number = 1
while True:
url = f'https://www.example.com/page/{page_number}'
response = requests.get(url, headers=headers)
if response.status_code != 200:
break
soup = BeautifulSoup(response.text, 'html.parser')
# 提取数据
# ...
page_number += 1
八、处理反爬虫机制
许多网站都有反爬虫机制,如验证码、IP封禁等。为了应对这些反爬虫机制,可以采取以下措施:
- 使用代理IP:通过代理IP发送请求,避免被封禁。
- 设置请求间隔:避免频繁发送请求,以减少被检测到的可能性。
- 模拟用户行为:如随机点击页面上的链接、滚动页面等,以模拟真实用户的行为。
import time
import random
proxies = {
'http': 'http://proxy_ip:proxy_port',
'https': 'http://proxy_ip:proxy_port'
}
for i in range(1, 101):
url = f'https://www.example.com/page/{i}'
response = requests.get(url, headers=headers, proxies=proxies)
if response.status_code != 200:
break
soup = BeautifulSoup(response.text, 'html.parser')
# 提取数据
# ...
time.sleep(random.uniform(1, 5)) # 随机间隔1到5秒
九、处理数据清洗和存储
在爬取数据后,通常需要对数据进行清洗和存储。数据清洗包括去重、处理缺失值等。数据存储可以选择将数据保存到CSV文件、数据库或其他存储介质中。
import pandas as pd
数据清洗
data = pd.DataFrame(data)
data.drop_duplicates(inplace=True)
data.dropna(inplace=True)
保存到CSV文件
data.to_csv('data.csv', index=False)
保存到数据库
from sqlalchemy import create_engine
engine = create_engine('sqlite:///data.db')
data.to_sql('table_name', engine, index=False, if_exists='replace')
十、总结
使用Python爬取信息是一个复杂的过程,需要处理各种问题,如请求头设置、HTML解析、数据处理等。通过合理的库和方法,可以高效地完成网页爬取任务。在实际应用中,还需要根据具体情况灵活调整爬取策略,以应对不同网站的反爬虫机制。希望通过本文的介绍,能够帮助你更好地理解和掌握使用Python进行网页爬取的技术。
相关问答FAQs:
如何选择适合的Python库进行信息爬取?
在进行信息爬取时,选择合适的库非常重要。常用的库包括BeautifulSoup
、Scrapy
和Requests
。Requests
可以帮助你轻松发送HTTP请求,而BeautifulSoup
则用于解析网页内容,适合处理HTML和XML文档。Scrapy
是一个强大的框架,适合进行大规模爬取项目,支持多线程和异步处理。
在进行爬取时,如何避免被网站封禁?
为了防止被网站封禁,可以采取一些措施。首先,合理设置请求频率,避免短时间内发送大量请求。其次,使用随机的用户代理(User-Agent),模拟不同的浏览器访问。此外,可以考虑使用代理服务器来隐藏真实IP地址,增加爬取的安全性。
如何处理爬取后获取的数据?
获取数据后,可以使用pandas
库进行数据处理和分析。将爬取的数据保存为CSV、Excel或数据库形式,方便后续使用。数据清洗也是一个重要步骤,例如去除重复项和处理缺失值。可视化工具如Matplotlib
或Seaborn
可帮助更好地理解数据趋势和分布。
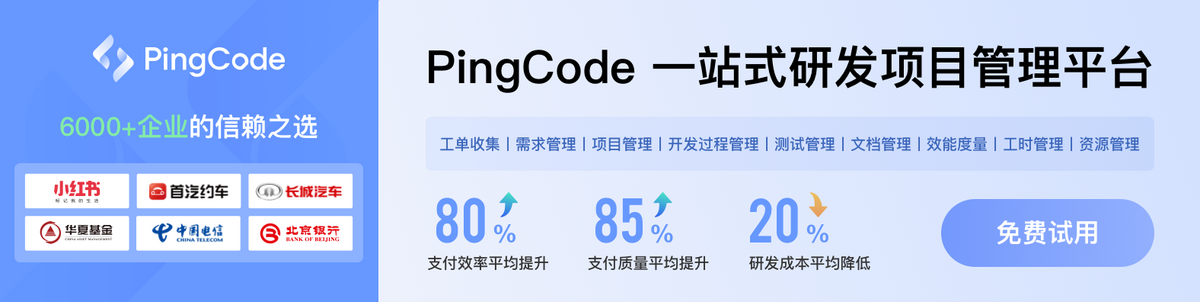