Python多线程运行代码的方法包括使用threading模块、使用concurrent.futures模块、使用multiprocessing.dummy模块。 其中,threading模块是最常用的多线程工具,它提供了对线程的管理和控制,使开发者可以方便地创建和管理线程。concurrent.futures模块提供了更高层次的接口,可以更简洁地实现多线程。multiprocessing.dummy模块使用与multiprocessing相同的接口,但实际使用的是线程而不是进程。下面将详细介绍如何使用threading模块来实现多线程运行代码。
使用threading模块进行多线程编程:
threading模块是Python中进行多线程编程的标准库。它提供了Thread类和其他一些工具,方便地创建和管理线程。使用threading模块时,可以通过继承Thread类或者直接创建Thread对象并传递目标函数来实现多线程。
一、threading模块
1、创建线程的方法
在Python中,使用threading模块创建线程主要有两种方式:通过继承Thread类和直接创建Thread对象。
通过继承Thread类
通过继承Thread类,可以创建一个新的线程类,并重写其run方法。run方法包含了线程执行的代码逻辑。
import threading
class MyThread(threading.Thread):
def __init__(self, name):
threading.Thread.__init__(self)
self.name = name
def run(self):
print(f"Thread {self.name} is running")
创建线程
thread1 = MyThread("Thread-1")
thread2 = MyThread("Thread-2")
启动线程
thread1.start()
thread2.start()
等待所有线程执行完毕
thread1.join()
thread2.join()
print("All threads have finished execution")
直接创建Thread对象
直接创建Thread对象,并将目标函数和参数传递给Thread类的构造函数。
import threading
def print_message(message):
print(f"Message: {message}")
创建线程
thread1 = threading.Thread(target=print_message, args=("Hello from Thread-1",))
thread2 = threading.Thread(target=print_message, args=("Hello from Thread-2",))
启动线程
thread1.start()
thread2.start()
等待所有线程执行完毕
thread1.join()
thread2.join()
print("All threads have finished execution")
2、线程同步与锁机制
多线程编程中,线程同步和锁机制是非常重要的概念。Python提供了多种同步原语,如Lock、RLock、Condition、Semaphore等,来确保线程之间的同步和共享数据的一致性。
使用Lock进行线程同步
Lock是最基本的同步原语,提供了简单的锁机制。
import threading
class Counter:
def __init__(self):
self.value = 0
self.lock = threading.Lock()
def increment(self):
with self.lock:
self.value += 1
counter = Counter()
def worker():
for _ in range(100000):
counter.increment()
创建并启动线程
threads = []
for _ in range(10):
thread = threading.Thread(target=worker)
threads.append(thread)
thread.start()
等待所有线程执行完毕
for thread in threads:
thread.join()
print(f"Final counter value: {counter.value}")
使用RLock进行递归锁同步
RLock(递归锁)允许同一个线程多次获取锁,而不会造成死锁。
import threading
class RecursiveCounter:
def __init__(self):
self.value = 0
self.lock = threading.RLock()
def increment(self):
with self.lock:
self.value += 1
self._increment_helper()
def _increment_helper(self):
with self.lock:
self.value += 1
counter = RecursiveCounter()
def worker():
for _ in range(100000):
counter.increment()
创建并启动线程
threads = []
for _ in range(10):
thread = threading.Thread(target=worker)
threads.append(thread)
thread.start()
等待所有线程执行完毕
for thread in threads:
thread.join()
print(f"Final recursive counter value: {counter.value}")
3、线程通信与共享数据
在多线程编程中,线程之间的通信和共享数据是常见需求。Python提供了多种方式实现线程通信和数据共享,如使用Queue、Condition等。
使用Queue进行线程通信
Queue是线程安全的队列,适用于线程之间的通信。
import threading
import queue
def producer(q):
for i in range(10):
q.put(i)
print(f"Produced {i}")
def consumer(q):
while True:
item = q.get()
if item is None:
break
print(f"Consumed {item}")
q = queue.Queue()
创建并启动生产者线程
producer_thread = threading.Thread(target=producer, args=(q,))
producer_thread.start()
创建并启动消费者线程
consumer_thread = threading.Thread(target=consumer, args=(q,))
consumer_thread.start()
等待生产者线程执行完毕
producer_thread.join()
向队列中添加None表示结束
q.put(None)
等待消费者线程执行完毕
consumer_thread.join()
print("All threads have finished execution")
使用Condition进行线程通信
Condition是更高级的同步原语,允许线程在特定条件下等待和通知。
import threading
class SharedData:
def __init__(self):
self.value = 0
self.condition = threading.Condition()
def produce(self):
with self.condition:
self.value += 1
print(f"Produced {self.value}")
self.condition.notify_all()
def consume(self):
with self.condition:
while self.value == 0:
self.condition.wait()
print(f"Consumed {self.value}")
self.value -= 1
shared_data = SharedData()
def producer():
for _ in range(10):
shared_data.produce()
def consumer():
for _ in range(10):
shared_data.consume()
创建并启动生产者线程
producer_thread = threading.Thread(target=producer)
producer_thread.start()
创建并启动消费者线程
consumer_thread = threading.Thread(target=consumer)
consumer_thread.start()
等待所有线程执行完毕
producer_thread.join()
consumer_thread.join()
print("All threads have finished execution")
二、concurrent.futures模块
concurrent.futures模块提供了ThreadPoolExecutor类,可以更简洁地实现多线程编程。它提供了线程池的概念,可以方便地管理多个线程。
1、使用ThreadPoolExecutor
ThreadPoolExecutor类提供了submit和map方法,用于提交线程任务和批量执行任务。
from concurrent.futures import ThreadPoolExecutor
def task(message):
print(f"Task: {message}")
with ThreadPoolExecutor(max_workers=2) as executor:
futures = [executor.submit(task, f"Hello from Task-{i}") for i in range(5)]
# 等待所有任务完成
for future in futures:
future.result()
print("All tasks have finished execution")
2、使用map方法批量执行任务
map方法可以方便地批量执行任务,并返回结果。
from concurrent.futures import ThreadPoolExecutor
def task(number):
return number * 2
with ThreadPoolExecutor(max_workers=2) as executor:
results = executor.map(task, range(5))
for result in results:
print(f"Result: {result}")
print("All tasks have finished execution")
三、multiprocessing.dummy模块
multiprocessing.dummy模块使用与multiprocessing相同的接口,但实际使用的是线程而不是进程。它提供了与multiprocessing相同的API,使得代码可以在多进程和多线程之间无缝切换。
1、使用Pool类
multiprocessing.dummy模块的Pool类提供了线程池的概念,可以方便地管理多个线程。
from multiprocessing.dummy import Pool as ThreadPool
def task(number):
return number * 2
创建线程池
pool = ThreadPool(4)
批量执行任务
results = pool.map(task, range(10))
关闭线程池
pool.close()
pool.join()
for result in results:
print(f"Result: {result}")
print("All tasks have finished execution")
2、使用apply_async方法
apply_async方法可以异步执行任务,并返回结果。
from multiprocessing.dummy import Pool as ThreadPool
def task(message):
print(f"Task: {message}")
创建线程池
pool = ThreadPool(4)
异步执行任务
results = [pool.apply_async(task, args=(f"Hello from Task-{i}",)) for i in range(10)]
等待所有任务完成
for result in results:
result.wait()
关闭线程池
pool.close()
pool.join()
print("All tasks have finished execution")
四、线程和进程的区别
在多线程编程中,理解线程和进程的区别是非常重要的。线程和进程是操作系统中并发执行的基本单位,它们之间有一些重要的区别。
1、进程
进程是操作系统中资源分配的基本单位,每个进程都有自己的内存空间和资源。进程之间相互独立,进程中的线程共享相同的内存空间。
2、线程
线程是进程中执行的基本单位,同一个进程中的多个线程共享相同的内存空间和资源。线程之间可以直接通信,但需要进行同步以避免数据竞争。
五、多线程编程的注意事项
在进行多线程编程时,需要注意以下几个方面:
1、线程安全
在多线程编程中,多个线程可能会同时访问共享数据,导致数据竞争和不一致。需要使用锁机制(如Lock、RLock等)来确保线程安全。
2、死锁
死锁是指两个或多个线程相互等待对方释放资源,导致线程无法继续执行。需要避免死锁,可以使用递归锁(RLock)或者避免嵌套锁。
3、性能
多线程编程可以提高程序的并发性和响应速度,但也会带来线程切换和同步的开销。在某些情况下,多线程编程可能会降低程序性能。
4、GIL(全局解释器锁)
Python的GIL限制了同一时刻只有一个线程在执行Python字节码,这在一定程度上限制了多线程编程的性能提升。在需要充分利用多核CPU的情况下,可以考虑使用多进程编程。
六、总结
多线程编程是Python中实现并发编程的重要手段,threading模块、concurrent.futures模块和multiprocessing.dummy模块是常用的多线程工具。通过合理使用线程和同步机制,可以有效提高程序的并发性和响应速度。在进行多线程编程时,需要注意线程安全、死锁和性能等问题,同时需要理解线程和进程的区别,以便在不同场景下选择合适的并发编程方式。
相关问答FAQs:
如何在Python中实现多线程?
在Python中实现多线程可以使用threading
模块。首先,需要导入该模块,然后创建一个Thread
对象,传入目标函数和参数。最后,调用start()
方法来启动线程,使用join()
方法确保主线程等待子线程完成。这样可以有效地利用多核处理器,提高程序的执行效率。
多线程在Python中有什么优势和劣势?
多线程的优势在于可以同时执行多个任务,特别适合IO密集型操作,如网络请求或文件读取。然而,由于Python的全局解释器锁(GIL),多线程在CPU密集型任务中并不总是有效。因此,在处理CPU密集型任务时,考虑使用多进程(如multiprocessing
模块)可能更为合适。
如何调试Python中的多线程代码?
调试多线程代码可以使用一些特定的工具和技术。Python的logging
模块可以帮助记录线程的执行情况和状态变化,便于追踪问题。此外,使用调试器(如pdb
)时,注意查看线程的状态和上下文切换情况,以确保对并发执行的任务有清晰的理解。通过适当的日志和调试工具,可以有效定位多线程代码中的问题。
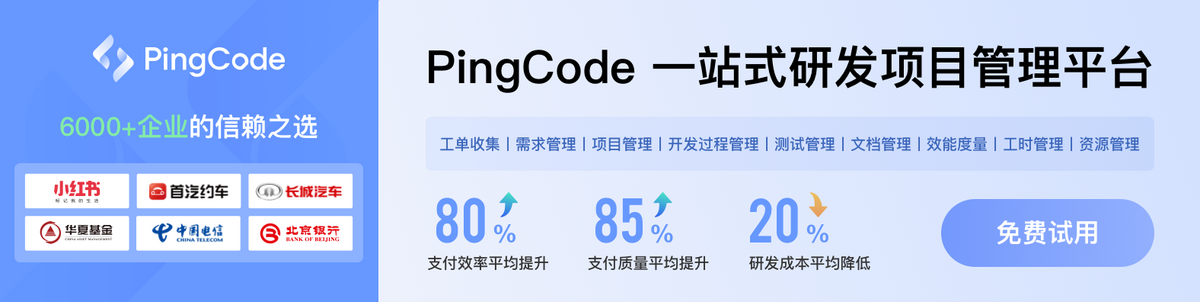