一、如何使用Python查找物理地址(MAC地址)
要使用Python查找物理地址(MAC地址),可以使用系统命令、第三方库如uuid
、psutil
和socket
等。 其中,使用uuid
库是一种非常简单且直接的方法。通过调用uuid
库中的getnode()
方法,我们可以获取到本机的MAC地址。下面将详细讲解如何使用这些方法来查找物理地址。
使用 uuid
库
uuid
库是Python标准库的一部分,它提供了很多与UUID(Universally Unique Identifier,通用唯一识别码)相关的功能。uuid
库中的 getnode()
方法可以用来获取机器的物理地址(MAC地址)。
import uuid
mac_address = uuid.getnode()
mac_address = ':'.join(("%012X" % mac_address)[i:i+2] for i in range(0, 12, 2))
print(f"MAC地址: {mac_address}")
在上面的代码中,我们首先使用 uuid.getnode()
方法获取到机器的物理地址。然后,我们将其格式化为常见的MAC地址格式,通过每两个字符之间添加一个冒号。
使用 psutil
库
psutil
是一个跨平台库,用于检索系统和进程的运行信息。它提供了对系统网络接口的访问,可以用来获取物理地址。
import psutil
for interface, addrs in psutil.net_if_addrs().items():
for addr in addrs:
if addr.family == psutil.AF_LINK:
print(f"{interface} 的MAC地址: {addr.address}")
在这段代码中,我们使用 psutil.net_if_addrs()
方法来获取所有网络接口及其地址信息。然后,我们遍历每个接口,查找地址族为 psutil.AF_LINK
的地址,这些地址即为MAC地址。
使用 socket
库和系统命令
在某些情况下,我们可能需要使用系统命令来获取MAC地址,并通过 socket
库来执行这些命令。
在Windows系统上
import subprocess
def get_mac_address():
result = subprocess.run(["getmac"], capture_output=True, text=True)
return result.stdout
print(f"MAC地址: {get_mac_address()}")
在Linux系统上
import subprocess
def get_mac_address():
result = subprocess.run(["ifconfig"], capture_output=True, text=True)
return result.stdout
print(f"MAC地址: {get_mac_address()}")
通过 subprocess.run
方法,我们可以执行系统命令并捕获其输出。getmac
命令在Windows上使用,而 ifconfig
命令在Linux上使用。
使用 netifaces
库
netifaces
是一个第三方库,用于获取网络接口信息。它可以跨平台使用,并且相对简单易用。
import netifaces
def get_mac_address():
interfaces = netifaces.interfaces()
mac_addresses = []
for interface in interfaces:
addrs = netifaces.ifaddresses(interface)
if netifaces.AF_LINK in addrs:
mac = addrs[netifaces.AF_LINK][0]['addr']
mac_addresses.append((interface, mac))
return mac_addresses
for interface, mac in get_mac_address():
print(f"{interface} 的MAC地址: {mac}")
在这段代码中,我们使用 netifaces.interfaces()
方法获取所有网络接口,然后遍历每个接口,查找地址族为 netifaces.AF_LINK
的地址,从而获取MAC地址。
二、获取网络接口信息
使用 socket
库
socket
库是Python标准库的一部分,它提供了用于网络通信的低级接口。尽管它不能直接获取MAC地址,但可以用来获取一些网络接口信息。
import socket
def get_ip_address():
hostname = socket.gethostname()
ip_address = socket.gethostbyname(hostname)
return ip_address
print(f"IP地址: {get_ip_address()}")
在这段代码中,我们使用 socket.gethostname()
方法获取主机名,然后使用 socket.gethostbyname()
方法获取与该主机名关联的IP地址。
使用 psutil
库
psutil
库除了可以获取MAC地址之外,还可以获取更多的网络接口信息。
import psutil
def get_network_info():
network_info = {}
for interface, addrs in psutil.net_if_addrs().items():
network_info[interface] = {}
for addr in addrs:
if addr.family == psutil.AF_INET:
network_info[interface]['IPv4'] = addr.address
elif addr.family == psutil.AF_INET6:
network_info[interface]['IPv6'] = addr.address
elif addr.family == psutil.AF_LINK:
network_info[interface]['MAC'] = addr.address
return network_info
for interface, info in get_network_info().items():
print(f"{interface} 信息: {info}")
在这段代码中,我们使用 psutil.net_if_addrs()
方法获取所有网络接口及其地址信息。然后,我们根据地址族来分别获取IPv4、IPv6和MAC地址,并将其存储在一个字典中。
使用 netifaces
库
netifaces
库除了可以获取MAC地址之外,还可以获取其他类型的网络接口信息。
import netifaces
def get_network_info():
interfaces = netifaces.interfaces()
network_info = {}
for interface in interfaces:
addrs = netifaces.ifaddresses(interface)
network_info[interface] = {}
if netifaces.AF_INET in addrs:
network_info[interface]['IPv4'] = addrs[netifaces.AF_INET][0]['addr']
if netifaces.AF_INET6 in addrs:
network_info[interface]['IPv6'] = addrs[netifaces.AF_INET6][0]['addr']
if netifaces.AF_LINK in addrs:
network_info[interface]['MAC'] = addrs[netifaces.AF_LINK][0]['addr']
return network_info
for interface, info in get_network_info().items():
print(f"{interface} 信息: {info}")
在这段代码中,我们使用 netifaces.ifaddresses(interface)
方法获取指定接口的地址信息。然后,我们根据地址族来分别获取IPv4、IPv6和MAC地址,并将其存储在一个字典中。
三、跨平台获取MAC地址
使用 platform
库
platform
库是Python标准库的一部分,它提供了一些关于操作系统的信息。我们可以使用它来确定当前的操作系统,并选择合适的方法来获取MAC地址。
import platform
import uuid
import psutil
import subprocess
import netifaces
def get_mac_address():
system = platform.system()
if system == "Windows":
mac = uuid.getnode()
mac = ':'.join(("%012X" % mac)[i:i+2] for i in range(0, 12, 2))
elif system == "Linux":
result = subprocess.run(["ifconfig"], capture_output=True, text=True)
mac = result.stdout
elif system == "Darwin":
interfaces = netifaces.interfaces()
mac_addresses = []
for interface in interfaces:
addrs = netifaces.ifaddresses(interface)
if netifaces.AF_LINK in addrs:
mac = addrs[netifaces.AF_LINK][0]['addr']
mac_addresses.append((interface, mac))
mac = mac_addresses
else:
raise NotImplementedError("Unsupported system")
return mac
print(f"MAC地址: {get_mac_address()}")
在这段代码中,我们首先使用 platform.system()
方法来确定当前的操作系统。然后,根据操作系统的不同,选择合适的方法来获取MAC地址。
使用 ifconfig
和 ip
命令
在Linux系统上,可以使用 ifconfig
和 ip
命令来获取网络接口信息,包括MAC地址。
import subprocess
def get_mac_address():
try:
result = subprocess.run(["ifconfig"], capture_output=True, text=True)
return result.stdout
except FileNotFoundError:
result = subprocess.run(["ip", "link"], capture_output=True, text=True)
return result.stdout
print(f"MAC地址: {get_mac_address()}")
在这段代码中,我们首先尝试使用 ifconfig
命令来获取网络接口信息。如果 ifconfig
命令不可用,则使用 ip link
命令来获取相同的信息。
四、总结
获取物理地址(MAC地址)的方法有很多,具体选择哪种方法取决于实际需求和操作系统的环境。使用uuid
库是最简单的方法,但在某些情况下,使用psutil
、netifaces
等库可以提供更丰富的信息。此外,对于跨平台的需求,可以结合使用platform
库来确定操作系统,并选择合适的方法来获取MAC地址。无论使用哪种方法,了解网络接口和地址信息对于网络编程和系统管理都是非常重要的技能。
相关问答FAQs:
如何在Python中获取物理地址?
在Python中,获取物理地址并不是一个直接的过程,因为Python的标准库并不提供直接访问物理内存地址的功能。通常,需要使用系统命令或特定的库(如psutil
或ctypes
)来实现。你可以通过调用系统的命令行工具,或使用os
模块来执行相应的命令,来获得网络接口的物理地址(MAC地址)等。
获取MAC地址的常用方法有哪些?
可以通过多种方式获取MAC地址,例如使用uuid
模块中的getnode()
方法,它能返回本机的MAC地址。还可以使用subprocess
模块执行系统命令,如ifconfig
或ip addr
(在Linux系统上)来提取MAC地址信息。Windows系统下,可以使用getmac
命令。每种方法都有其适用场景,选择合适的方式能提高效率。
Python中使用psutil
库有什么优势?psutil
库是一个跨平台的库,用于获取系统信息,包括进程和系统使用情况。使用psutil
获取网络接口信息时,可以方便地获取每个接口的MAC地址及相关状态,且代码简洁。它的优势在于不仅可以获取MAC地址,还能获取CPU、内存、磁盘、网络等各种系统监控信息,适合需要全面系统监控的场景。
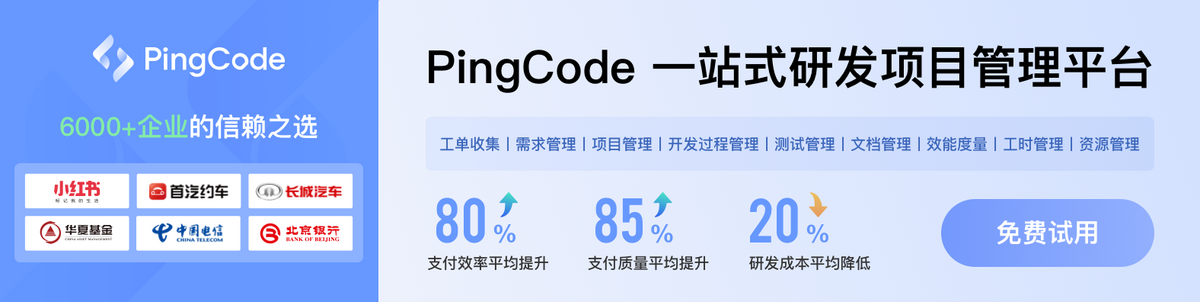