Python中线程的内存分配主要通过设置线程的栈大小、使用线程本地存储(Thread-Local Storage)、优化全局解释器锁(GIL)的使用来实现。下面将详细描述如何通过设置线程的栈大小来实现内存分配。
设置线程的栈大小可以通过threading
模块中的threading.stack_size(size)
函数来完成。这个函数允许你为每个线程设置不同的栈大小,这样就可以根据线程的需要来分配合适的内存大小。默认情况下,Python会为每个线程分配一个系统默认的栈大小,但有些应用可能需要更大或更小的栈。这时,使用threading.stack_size(size)
可以帮助你优化内存使用。
例如,假设你有一个需要大量递归调用的线程,你可以通过增加该线程的栈大小来防止出现RecursionError
:
import threading
def recursive_function(n):
if n == 0:
return
recursive_function(n-1)
设置栈大小为2MB
threading.stack_size(2 * 1024 * 1024)
thread = threading.Thread(target=recursive_function, args=(1000,))
thread.start()
thread.join()
通过这种方式,可以为线程分配合适的内存,以确保其正常运行。
一、线程的栈大小
栈是线程在执行过程中用来存储局部变量和函数调用信息的数据结构。不同的线程可能需要不同大小的栈,特别是在进行深度递归或处理大型数据时。合理设置线程的栈大小可以避免因栈溢出导致的程序崩溃。
1.1 默认栈大小
默认情况下,Python为每个线程分配一个系统默认的栈大小,这个值可能因操作系统不同而异。可以通过threading.stack_size()
函数获取默认栈大小:
import threading
default_stack_size = threading.stack_size()
print(f'Default stack size: {default_stack_size}')
1.2 设置栈大小
可以使用threading.stack_size(size)
函数来设置线程的栈大小。需要注意的是,栈大小应该是系统页大小的倍数(通常是4KB的整数倍)。
import threading
设置栈大小为1MB
threading.stack_size(1024 * 1024)
def task():
# 执行一些任务
pass
thread = threading.Thread(target=task)
thread.start()
thread.join()
通过设置适当的栈大小,可以有效地防止栈溢出错误,确保线程能够正常执行其任务。
二、线程本地存储(Thread-Local Storage)
线程本地存储(Thread-Local Storage,TLS)是一种机制,允许在同一个进程中的多个线程拥有各自独立的变量副本。这样可以避免不同线程之间的变量冲突,提高程序的并发性和稳定性。
2.1 使用threading.local()
Python的threading
模块提供了threading.local()
函数,用于创建线程本地存储对象。每个线程在访问该对象时,都会获取一个独立的副本。
import threading
创建线程本地存储对象
local_data = threading.local()
def process_data():
local_data.value = threading.current_thread().name
print(f'Thread {local_data.value} is processing data')
thread1 = threading.Thread(target=process_data)
thread2 = threading.Thread(target=process_data)
thread1.start()
thread2.start()
thread1.join()
thread2.join()
在上述示例中,local_data.value
在每个线程中都是独立的,不会相互干扰。
2.2 线程本地存储的应用
线程本地存储在多线程环境中非常有用,尤其是在需要处理线程特定的数据时。例如,数据库连接、配置参数等可以存储在线程本地存储中,以避免不同线程之间的数据冲突。
import threading
local_data = threading.local()
def init_db_connection():
local_data.connection = f'DB connection for {threading.current_thread().name}'
def use_db_connection():
print(f'Using {local_data.connection}')
def thread_task():
init_db_connection()
use_db_connection()
thread1 = threading.Thread(target=thread_task)
thread2 = threading.Thread(target=thread_task)
thread1.start()
thread2.start()
thread1.join()
thread2.join()
在这个示例中,每个线程都有自己的数据库连接,避免了线程之间的数据冲突。
三、全局解释器锁(GIL)
Python的全局解释器锁(Global Interpreter Lock,GIL)是一个限制多线程并发执行的机制。GIL确保在任何时候只有一个线程可以执行Python字节码,这样可以避免多线程环境下的数据竞争问题,但也限制了Python的多线程性能。
3.1 GIL的影响
由于GIL的存在,Python多线程程序在CPU密集型任务中无法真正实现并行执行。这意味着,即使在多核处理器上,Python多线程程序也只能利用一个CPU核心。
import threading
def cpu_intensive_task():
count = 0
for _ in range(107):
count += 1
thread1 = threading.Thread(target=cpu_intensive_task)
thread2 = threading.Thread(target=cpu_intensive_task)
thread1.start()
thread2.start()
thread1.join()
thread2.join()
在上述示例中,两个线程无法真正并行执行,导致性能未能显著提升。
3.2 解决GIL限制的方法
虽然GIL限制了Python多线程程序的性能,但可以通过以下方法来优化:
- 使用多进程代替多线程:通过
multiprocessing
模块,可以创建多个进程,每个进程都有独立的GIL,这样可以充分利用多核处理器。
import multiprocessing
def cpu_intensive_task():
count = 0
for _ in range(107):
count += 1
process1 = multiprocessing.Process(target=cpu_intensive_task)
process2 = multiprocessing.Process(target=cpu_intensive_task)
process1.start()
process2.start()
process1.join()
process2.join()
-
使用C扩展模块:通过编写C扩展模块,可以绕过GIL,实现在C代码中进行并行计算。
-
使用第三方库:一些第三方库(如NumPy、SciPy)通过在内部使用多线程或多进程来优化性能,从而绕过GIL的限制。
四、线程池
线程池是一种预先创建一组线程的机制,用于执行任务。线程池可以重用线程,避免频繁创建和销毁线程带来的开销,从而提高程序的性能。
4.1 使用concurrent.futures.ThreadPoolExecutor
Python的concurrent.futures
模块提供了ThreadPoolExecutor
类,用于创建和管理线程池。
from concurrent.futures import ThreadPoolExecutor
def task(n):
return n * n
with ThreadPoolExecutor(max_workers=4) as executor:
futures = [executor.submit(task, i) for i in range(10)]
results = [future.result() for future in futures]
print(results)
在上述示例中,使用ThreadPoolExecutor
创建了一个包含4个线程的线程池,并提交了10个任务进行执行。线程池会自动管理线程的创建和销毁,简化了多线程编程的复杂性。
4.2 线程池的优势
线程池具有以下优势:
- 提高性能:通过重用线程,减少了线程的创建和销毁开销,提升了程序的性能。
- 简化编程:线程池自动管理线程,简化了多线程编程的复杂性。
- 控制并发数量:通过设置线程池的线程数量,可以有效控制并发线程的数量,避免过多线程导致系统资源耗尽。
五、线程安全
在多线程编程中,线程安全是一个重要的问题。线程安全指的是多个线程在访问共享资源时,不会造成数据竞争或数据不一致的问题。Python提供了多种机制来实现线程安全。
5.1 使用锁(Lock)
锁(Lock)是最基本的同步原语,用于保护共享资源。通过锁,多个线程在访问共享资源时,可以确保只有一个线程可以访问资源,避免数据竞争。
import threading
lock = threading.Lock()
shared_resource = 0
def increment():
global shared_resource
with lock:
local_copy = shared_resource
local_copy += 1
shared_resource = local_copy
threads = [threading.Thread(target=increment) for _ in range(100)]
for thread in threads:
thread.start()
for thread in threads:
thread.join()
print(shared_resource)
在上述示例中,通过锁保护了共享资源shared_resource
,确保多个线程在访问该资源时不会发生数据竞争。
5.2 使用条件变量(Condition)
条件变量(Condition)是另一种同步原语,用于线程间的通信。通过条件变量,线程可以等待某个条件发生,然后继续执行。
import threading
condition = threading.Condition()
shared_resource = 0
def producer():
global shared_resource
with condition:
shared_resource += 1
condition.notify()
def consumer():
with condition:
condition.wait()
print(f'Consumed: {shared_resource}')
producer_thread = threading.Thread(target=producer)
consumer_thread = threading.Thread(target=consumer)
consumer_thread.start()
producer_thread.start()
producer_thread.join()
consumer_thread.join()
在上述示例中,生产者线程通过条件变量通知消费者线程,消费者线程在条件满足时继续执行。
六、线程调度
线程调度是指操作系统决定哪个线程在什么时候运行的过程。虽然Python的线程调度是由操作系统管理的,但了解线程调度的基本原理对编写高效的多线程程序非常重要。
6.1 线程的生命周期
线程的生命周期包括以下几个状态:
- 新建(New):线程对象被创建,但尚未启动。
- 就绪(Runnable):线程已经启动,等待操作系统分配CPU时间。
- 运行(Running):线程正在执行。
- 阻塞(Blocked):线程在等待某个事件(如I/O操作完成)时进入阻塞状态。
- 终止(Terminated):线程执行完毕或被中止。
6.2 线程优先级
虽然Python的threading
模块不提供直接设置线程优先级的功能,但可以通过操作系统提供的线程优先级接口来设置线程的优先级。例如,在Windows系统上,可以使用pywin32
库来设置线程优先级。
import threading
import win32api
import win32con
def task():
print(f'Thread {threading.current_thread().name} is running')
thread = threading.Thread(target=task)
thread.start()
handle = win32api.OpenThread(win32con.THREAD_ALL_ACCESS, False, thread.ident)
win32api.SetThreadPriority(handle, win32con.THREAD_PRIORITY_HIGHEST)
thread.join()
在上述示例中,通过pywin32
库设置了线程的优先级。
七、线程池中的内存管理
在线程池中,合理的内存管理对于提高程序的性能和稳定性至关重要。以下是一些优化线程池内存管理的方法。
7.1 任务队列
线程池中的任务通常通过任务队列进行管理。任务队列的大小和管理方式会直接影响线程池的内存使用。
from concurrent.futures import ThreadPoolExecutor
import queue
task_queue = queue.Queue(maxsize=100)
def producer():
for i in range(1000):
task_queue.put(i)
def consumer():
while not task_queue.empty():
task = task_queue.get()
print(f'Processed task: {task}')
task_queue.task_done()
with ThreadPoolExecutor(max_workers=4) as executor:
executor.submit(producer)
for _ in range(4):
executor.submit(consumer)
在上述示例中,通过设置任务队列的最大大小,可以控制线程池的内存使用,避免任务过多导致内存耗尽。
7.2 任务重用
通过任务重用,可以减少线程池中的内存分配和释放,提高内存使用效率。例如,可以使用对象池来管理任务对象的分配和释放。
from concurrent.futures import ThreadPoolExecutor
import queue
class Task:
def __init__(self, data):
self.data = data
task_pool = queue.Queue()
def get_task(data):
try:
task = task_pool.get_nowait()
except queue.Empty:
task = Task(data)
else:
task.data = data
return task
def release_task(task):
task_pool.put(task)
def worker(task):
print(f'Processing task with data: {task.data}')
release_task(task)
with ThreadPoolExecutor(max_workers=4) as executor:
for i in range(100):
task = get_task(i)
executor.submit(worker, task)
在上述示例中,通过对象池管理任务对象的分配和释放,减少了内存分配和释放的开销。
八、线程的性能优化
在实际应用中,合理的性能优化可以显著提高多线程程序的执行效率。以下是一些常见的性能优化方法。
8.1 减少锁的使用
虽然锁可以确保线程安全,但频繁使用锁会导致线程竞争,降低程序的性能。因此,应尽量减少锁的使用,可以通过减少共享资源的访问、使用无锁数据结构等方式来优化性能。
import threading
shared_resource = 0
lock = threading.Lock()
def increment_with_lock():
global shared_resource
with lock:
shared_resource += 1
def increment_without_lock():
global shared_resource
shared_resource += 1
threads = [threading.Thread(target=increment_without_lock) for _ in range(100)]
for thread in threads:
thread.start()
for thread in threads:
thread.join()
print(shared_resource)
在上述示例中,通过减少锁的使用,优化了程序的性能。
8.2 使用无锁数据结构
无锁数据结构是一种不需要显式锁来保护共享资源的数据结构,可以在多线程环境中实现高效的并发访问。例如,Python的queue
模块提供了线程安全的队列,可以用于实现无锁数据结构。
import queue
import threading
task_queue = queue.Queue()
def producer():
for i in range(100):
task_queue.put(i)
def consumer():
while not task_queue.empty():
task = task_queue.get()
print(f'Processed task: {task}')
task_queue.task_done()
threads = [threading.Thread(target=consumer) for _ in range(4)]
for thread in threads:
thread.start()
for thread in threads:
thread.join()
在上述示例中,通过使用queue.Queue
实现了线程安全的任务队列,避免了显式锁的使用,提高了程序的性能。
九、线程的调试和测试
调试和测试是确保多线程程序正确性的重要环节。以下是一些常见的调试和测试方法。
9.1 使用调试器
调试器是定位和修复多线程程序中错误的重要工具。通过调试器,可以逐步执行程序,查看线程的状态和变量的值。例如,可以使用pdb
调试器来调试多线程程序。
import threading
import pdb
def task():
pdb.set_trace()
print(f'Thread {threading.current_thread().name} is running')
thread = threading.Thread(target=task)
thread.start()
thread.join()
在上述示例中,通过pdb.set_trace()
设置断点,可以在调试器中查看线程的状态和变量的值。
9.2 使用单元测试
单元测试是验证多线程程序正确性的重要方法。通过编写单元测试,可以自动化验证程序的行为,确保程序在不同情况下的正确性。例如,可以使用unittest
模块编写多线程程序的单元测试。
import unittest
import threading
class TestMultiThreading(unittest.TestCase):
def test_thread(self):
result = []
def task():
result.append(threading.current_thread().name)
threads = [threading.Thread(target=task) for _ in range(4)]
for thread in threads:
thread.start()
for thread in threads:
thread.join()
self.assertEqual(len(result), 4)
if __name__ == '__main__':
unittest.main()
在上述示例中,通过unittest
模块编写了多线程程序的单元测试,验证了线程的正确性。
十、总结
通过合理设置线程的栈大小、使用线程本地存储、优化全局解释器
相关问答FAQs:
如何在Python中管理线程的内存使用?
在Python中,线程的内存管理主要依赖于Python的内存管理机制。虽然Python的threading
模块允许你创建和管理线程,但内存分配是由Python的解释器处理的。你可以使用threading.local()
来创建一个线程局部存储,确保每个线程都有自己独立的内存空间,避免不同线程间的内存冲突。
Python的线程是否共享内存?
是的,Python的线程是共享内存的。这意味着多个线程可以访问相同的全局变量和数据结构。这种特性虽然可以提高效率,但也增加了数据竞争的风险。因此,开发者需要使用锁(如threading.Lock
)来确保线程安全,避免同时访问同一数据导致的错误。
如何优化Python线程的内存使用以提高性能?
为了优化Python线程的内存使用,可以考虑以下方法:减少线程的数量,确保每个线程只执行必要的任务;使用线程池(如concurrent.futures.ThreadPoolExecutor
)来管理线程的生命周期;避免在多个线程中重复创建大型对象,尽量重用对象,以减少内存开销。同时,监控内存使用情况,及时发现和解决内存泄漏问题。
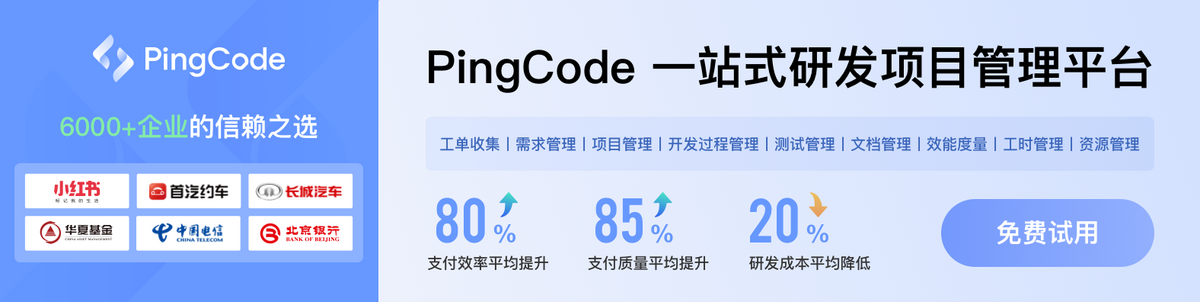