Python发送微信信息的方法主要有:使用第三方库如itchat
、利用微信企业号API、通过微信公众号开发接口。 在这些方法中,利用微信企业号API发送微信信息是比较常用和专业的方式。下面详细介绍利用微信企业号API发送微信信息的方法。
一、ITCHAT库
1、安装ITCHAT库
在使用itchat
库之前,需要先安装这个库。可以使用以下命令安装:
pip install itchat
2、登录微信
使用itchat
登录微信,并获取到你的微信账号信息:
import itchat
登录微信
itchat.auto_login(hotReload=True)
获取自己的用户信息
user_info = itchat.search_friends()
print(user_info)
3、发送微信消息
使用itchat
发送微信消息非常简单,示例如下:
# 发送消息给自己
itchat.send('Hello, this is a test message', toUserName='filehelper')
发送消息给好友
friends = itchat.search_friends(name='好友昵称')
if friends:
friend = friends[0]
itchat.send('Hello, this is a test message', toUserName=friend['UserName'])
二、利用微信企业号API
1、注册微信企业号
首先需要注册一个微信企业号,获取到企业号的CorpID
和CorpSecret
,这两个参数在后续的API调用中非常重要。
2、获取ACCESS_TOKEN
使用CorpID
和CorpSecret
获取ACCESS_TOKEN
,这是调用企业号API的凭证。具体代码如下:
import requests
def get_access_token(corpid, corpsecret):
url = f'https://qyapi.weixin.qq.com/cgi-bin/gettoken?corpid={corpid}&corpsecret={corpsecret}'
response = requests.get(url)
data = response.json()
return data['access_token']
corpid = '你的企业号ID'
corpsecret = '你的企业号Secret'
access_token = get_access_token(corpid, corpsecret)
print(access_token)
3、发送微信消息
获取到ACCESS_TOKEN
后,就可以使用企业号的API发送微信消息了。以下是发送文本消息的示例代码:
def send_message(access_token, user, content):
url = f'https://qyapi.weixin.qq.com/cgi-bin/message/send?access_token={access_token}'
message = {
"touser": user,
"msgtype": "text",
"agentid": "你的AgentID",
"text": {
"content": content
},
"safe": 0
}
response = requests.post(url, json=message)
return response.json()
user = '接收消息的用户ID'
content = 'Hello, this is a test message'
response = send_message(access_token, user, content)
print(response)
三、微信公众号开发接口
1、注册微信公众号
首先需要注册一个微信公众号,并在微信公众平台上完成相关设置,获取到AppID
和AppSecret
。
2、获取ACCESS_TOKEN
使用AppID
和AppSecret
获取ACCESS_TOKEN
,这是调用微信公众号API的凭证。具体代码如下:
import requests
def get_access_token(appid, appsecret):
url = f'https://api.weixin.qq.com/cgi-bin/token?grant_type=client_credential&appid={appid}&secret={appsecret}'
response = requests.get(url)
data = response.json()
return data['access_token']
appid = '你的AppID'
appsecret = '你的AppSecret'
access_token = get_access_token(appid, appsecret)
print(access_token)
3、发送微信消息
获取到ACCESS_TOKEN
后,就可以使用微信公众号的API发送微信消息了。以下是发送文本消息的示例代码:
def send_message(access_token, openid, content):
url = f'https://api.weixin.qq.com/cgi-bin/message/custom/send?access_token={access_token}'
message = {
"touser": openid,
"msgtype": "text",
"text": {
"content": content
}
}
response = requests.post(url, json=message)
return response.json()
openid = '接收消息的用户OpenID'
content = 'Hello, this is a test message'
response = send_message(access_token, openid, content)
print(response)
四、总结
使用Python发送微信消息的方法多种多样,其中利用微信企业号API和微信公众号开发接口是比较常用和专业的方式。这些方法各有优劣,开发者可以根据实际需求选择合适的方法。无论哪种方法,都需要先获取到相应的凭证(如ACCESS_TOKEN
),然后调用相应的API接口发送消息。
通过对本文的介绍,相信你已经了解了如何使用Python发送微信消息,希望这些内容能对你有所帮助。
相关问答FAQs:
如何使用Python库发送微信信息?
使用Python发送微信信息通常可以通过一些第三方库来实现,如itchat
。这个库允许你轻松地登录微信并发送消息。安装itchat
非常简单,只需运行pip install itchat
。登录后,可以使用itchat.send()
方法发送消息到指定的好友。确保在发送消息前,先使用itchat.auto_login()
进行登录。
我需要准备哪些东西才能用Python发送微信信息?
在使用Python发送微信信息之前,你需要安装Python及相关库,尤其是itchat
。此外,还需要一个有效的微信账号进行登录。为了保证顺利发送消息,请确保你的网络连接稳定,并且微信客户端没有被限制或封禁。
如果在使用Python发送微信信息时遇到问题,该如何解决?
如果在使用Python发送微信信息时遇到问题,可以先检查网络连接是否正常,确认itchat
库是否已正确安装。常见问题包括登录失败、消息发送失败等。如果问题持续存在,可以参考itchat
的官方文档或社区论坛,查找可能的解决方案或使用案例。
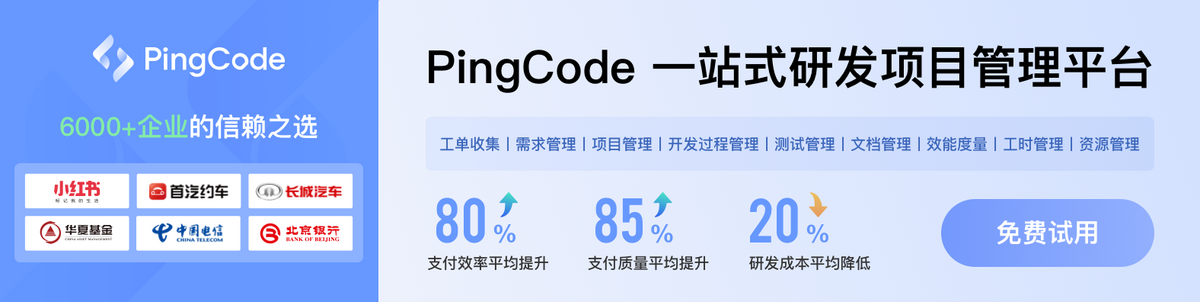