Python3 读取文件的方法包括使用内置的 open() 函数、使用 with 语句管理文件上下文、读取整个文件内容、逐行读取文件内容、读取指定字节数等。推荐使用 with 语句管理文件上下文,因为它可以确保文件在使用完毕后被正确关闭,避免资源泄漏。
一、使用 open() 函数
在 Python 中,读取文件最常用的方式是使用内置的 open() 函数。open() 函数有两个主要的参数:文件路径和模式。文件路径指定要读取的文件的路径,模式指定文件的打开方式(例如只读、写入、追加等)。
1.1、基本用法
file = open('example.txt', 'r') # 以只读模式打开文件
content = file.read() # 读取整个文件内容
print(content)
file.close() # 关闭文件
这种方法需要手动调用 close() 方法来关闭文件,以释放资源。
1.2、使用 with 语句
使用 with 语句可以自动管理文件资源,确保文件在使用完毕后被正确关闭。
with open('example.txt', 'r') as file:
content = file.read() # 读取整个文件内容
print(content)
使用 with 语句时,即使发生异常,也能确保文件被正确关闭。
二、逐行读取文件
2.1、使用 readline() 方法
readline() 方法每次读取文件的一行内容。
with open('example.txt', 'r') as file:
line = file.readline()
while line:
print(line, end='') # 打印每行内容
line = file.readline()
2.2、使用 for 循环
使用 for 循环可以更简洁地逐行读取文件。
with open('example.txt', 'r') as file:
for line in file:
print(line, end='') # 打印每行内容
三、读取指定字节数
3.1、使用 read() 方法
read() 方法可以读取指定字节数的内容。
with open('example.txt', 'r') as file:
content = file.read(10) # 读取前10个字节
print(content)
3.2、使用 readlines() 方法
readlines() 方法读取文件的所有行,并返回一个包含每行内容的列表。
with open('example.txt', 'r') as file:
lines = file.readlines()
for line in lines:
print(line, end='') # 打印每行内容
四、处理文件路径
在读取文件时,处理文件路径是很重要的一部分。Python 提供了 os 模块来帮助管理文件路径。
4.1、使用 os.path.join()
os.path.join() 方法可以根据操作系统自动选择合适的路径分隔符。
import os
file_path = os.path.join('folder', 'example.txt')
with open(file_path, 'r') as file:
content = file.read()
print(content)
4.2、使用绝对路径
使用绝对路径可以避免路径问题。
file_path = '/absolute/path/to/example.txt'
with open(file_path, 'r') as file:
content = file.read()
print(content)
五、文件编码
读取文件时,指定文件编码是非常重要的,特别是处理非ASCII字符时。可以使用 encoding 参数来指定文件编码。
5.1、指定文件编码
with open('example.txt', 'r', encoding='utf-8') as file:
content = file.read()
print(content)
5.2、处理不同编码的文件
读取不同编码的文件时,可以根据具体情况指定合适的编码。
with open('example.txt', 'r', encoding='latin-1') as file:
content = file.read()
print(content)
六、异常处理
读取文件时,可能会遇到文件不存在、权限不足等问题。可以使用 try-except 语句进行异常处理。
6.1、基本异常处理
try:
with open('example.txt', 'r') as file:
content = file.read()
print(content)
except FileNotFoundError:
print("文件未找到")
except PermissionError:
print("权限不足")
6.2、捕获所有异常
捕获所有异常并打印错误信息。
try:
with open('example.txt', 'r') as file:
content = file.read()
print(content)
except Exception as e:
print(f"读取文件时发生错误: {e}")
七、二进制文件读取
读取二进制文件时,需要使用 'rb' 模式。
7.1、读取二进制文件
with open('example.bin', 'rb') as file:
content = file.read()
print(content)
7.2、逐块读取二进制文件
逐块读取二进制文件可以处理大文件。
with open('example.bin', 'rb') as file:
while chunk := file.read(1024):
print(chunk)
八、读取文件到内存
将文件内容读取到内存中进行处理。
8.1、读取小文件
对于小文件,可以一次性读取到内存中。
with open('example.txt', 'r') as file:
content = file.read()
lines = content.splitlines()
for line in lines:
print(line)
8.2、处理大文件
对于大文件,可以逐行读取并处理,避免占用过多内存。
with open('example.txt', 'r') as file:
for line in file:
process(line)
九、读取特定格式的文件
读取特定格式的文件(如 CSV、JSON、XML 等)需要使用相应的库。
9.1、读取 CSV 文件
使用 csv 模块读取 CSV 文件。
import csv
with open('example.csv', 'r') as file:
reader = csv.reader(file)
for row in reader:
print(row)
9.2、读取 JSON 文件
使用 json 模块读取 JSON 文件。
import json
with open('example.json', 'r') as file:
data = json.load(file)
print(data)
9.3、读取 XML 文件
使用 xml.etree.ElementTree 模块读取 XML 文件。
import xml.etree.ElementTree as ET
tree = ET.parse('example.xml')
root = tree.getroot()
for child in root:
print(child.tag, child.attrib)
十、读取压缩文件
读取压缩文件(如 ZIP、GZIP 等)需要使用相应的库。
10.1、读取 ZIP 文件
使用 zipfile 模块读取 ZIP 文件。
import zipfile
with zipfile.ZipFile('example.zip', 'r') as zip_file:
with zip_file.open('example.txt') as file:
content = file.read()
print(content.decode('utf-8'))
10.2、读取 GZIP 文件
使用 gzip 模块读取 GZIP 文件。
import gzip
with gzip.open('example.gz', 'rb') as file:
content = file.read()
print(content.decode('utf-8'))
十一、读取网络文件
读取网络文件需要使用相应的库(如 requests)。
11.1、使用 requests 库
使用 requests 库读取网络文件。
import requests
url = 'https://example.com/example.txt'
response = requests.get(url)
if response.status_code == 200:
content = response.text
print(content)
十二、总结
Python3 提供了丰富的文件读取方法,使用 open() 函数、使用 with 语句管理文件上下文、逐行读取文件内容、读取指定字节数、处理文件路径、指定文件编码、异常处理、读取二进制文件、读取特定格式的文件、读取压缩文件、读取网络文件 等都是常用的技巧。根据具体需求选择合适的方法,可以提高代码的可读性和效率。确保文件在使用完毕后被正确关闭,避免资源泄漏,是文件读取过程中需要特别注意的点。
相关问答FAQs:
如何在Python3中读取文本文件的内容?
在Python3中,可以使用内置的open()
函数来读取文本文件。可以通过'r'
模式打开文件,例如:
with open('filename.txt', 'r') as file:
content = file.read()
print(content)
这种方式会将整个文件的内容读入内存,并存储在变量content
中。使用with
语句可以确保文件在读取后自动关闭。
读取文件时如何处理编码问题?
在读取文本文件时,编码可能会影响文件内容的正确显示。可以在open()
函数中指定编码,例如:
with open('filename.txt', 'r', encoding='utf-8') as file:
content = file.read()
使用encoding='utf-8'
可以确保正确处理包含非ASCII字符的文件。
如何逐行读取文件而不是一次性读取全部内容?
若需要逐行读取文件,可以使用readline()
或readlines()
方法,或者通过循环直接遍历文件对象。例如:
with open('filename.txt', 'r') as file:
for line in file:
print(line.strip())
这种方式在处理大型文件时更加高效,因为它不会一次性将整个文件加载到内存中。使用strip()
方法可以去掉每行末尾的换行符。
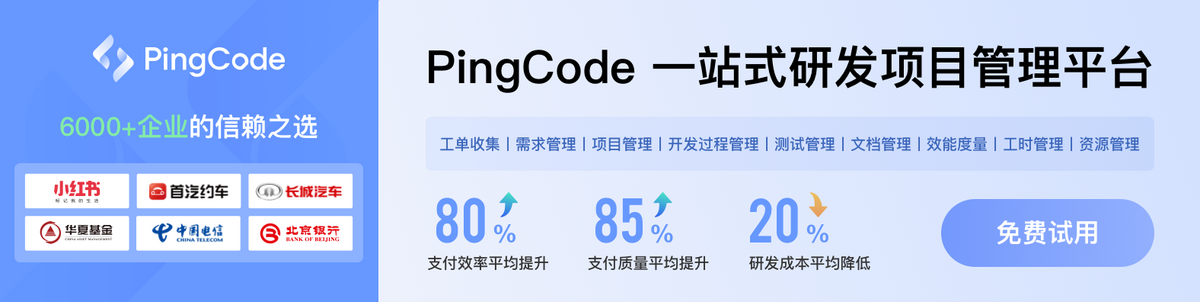