要使用Python打开网址图片,可以使用以下几种方法:urllib、requests、Pillow。下面将详细介绍这三种方法,并对其中一种方法进行详细描述。
使用urllib、使用requests、使用Pillow
详细描述“使用requests”方法:
requests库是Python中一个功能强大的HTTP库,使用它可以非常方便地下载网络资源。首先需要安装requests库,可以通过pip安装:
pip install requests
接下来,通过以下代码下载并打开图片:
import requests
from PIL import Image
from io import BytesIO
url = 'https://example.com/path/to/image.jpg'
response = requests.get(url)
img = Image.open(BytesIO(response.content))
img.show()
这段代码首先从指定URL下载图片,然后使用Pillow库(PIL)打开并显示图片。
一、使用urllib
urllib是Python内置的一个模块,用于处理URL相关的操作。我们可以使用它来下载网络上的图片并进行处理。首先需要导入相关模块:
import urllib.request
from PIL import Image
from io import BytesIO
然后,可以通过以下代码从指定URL下载图片并打开:
url = 'https://example.com/path/to/image.jpg'
with urllib.request.urlopen(url) as response:
img = Image.open(BytesIO(response.read()))
img.show()
在这段代码中,urllib.request.urlopen(url)
用于打开指定的URL并读取数据,BytesIO
用于将读取的字节流转换为内存中的文件对象,最后使用PIL.Image.open()
打开图片并显示。
二、使用requests
requests库是Python中一个功能强大的HTTP库,使用它可以非常方便地下载网络资源。首先需要安装requests库,可以通过pip安装:
pip install requests
接下来,通过以下代码下载并打开图片:
import requests
from PIL import Image
from io import BytesIO
url = 'https://example.com/path/to/image.jpg'
response = requests.get(url)
img = Image.open(BytesIO(response.content))
img.show()
这段代码首先从指定URL下载图片,然后使用Pillow库(PIL)打开并显示图片。
三、使用Pillow
Pillow是Python中一个强大的图像处理库,原名为PIL(Python Imaging Library),我们可以使用它来处理和显示图像。我们可以结合requests库来下载并打开网络图片。首先需要安装Pillow和requests库:
pip install Pillow requests
然后可以使用以下代码来实现:
import requests
from PIL import Image
from io import BytesIO
url = 'https://example.com/path/to/image.jpg'
response = requests.get(url)
img = Image.open(BytesIO(response.content))
img.show()
这段代码与前面介绍的使用requests的方法类似,首先从指定URL下载图片,然后使用Pillow库打开并显示图片。
四、总结
以上介绍了三种使用Python打开网址图片的方法:urllib、requests、Pillow。requests库是一个功能强大的HTTP库,使用它可以非常方便地下载网络资源。结合Pillow库,可以非常方便地处理和显示图像。总的来说,推荐使用requests库来下载图片,再使用Pillow库来处理和显示图片,这样可以更灵活地处理各种网络资源。
相关问答FAQs:
如何使用Python下载网页上的图片?
要下载网页上的图片,可以使用Python的requests库获取网页内容,然后利用BeautifulSoup解析HTML,并找到图片的URL。接下来,可以使用requests库的get方法下载图片并保存到本地。以下是一个简单的代码示例:
import requests
from bs4 import BeautifulSoup
url = '目标网址'
response = requests.get(url)
soup = BeautifulSoup(response.content, 'html.parser')
img_tags = soup.find_all('img')
for img in img_tags:
img_url = img['src']
img_data = requests.get(img_url).content
with open('图片名称.jpg', 'wb') as handler:
handler.write(img_data)
使用哪个库可以更方便地处理图片?
在Python中,Pillow库是处理图片的常用工具。它可以让你轻松地打开、操作和保存图片。结合requests库,用户可以方便地下载并处理图片。例如,下载后可以使用Pillow进行缩放、裁剪或格式转换等操作。
是否可以使用Python在下载时指定图片的保存路径?
当然可以。在使用open()函数时,可以指定完整的文件路径来保存图片。例如:
with open('C:/Users/用户名/Downloads/图片名称.jpg', 'wb') as handler:
handler.write(img_data)
这样就能将图片直接保存到指定的文件夹中,方便管理和查找。
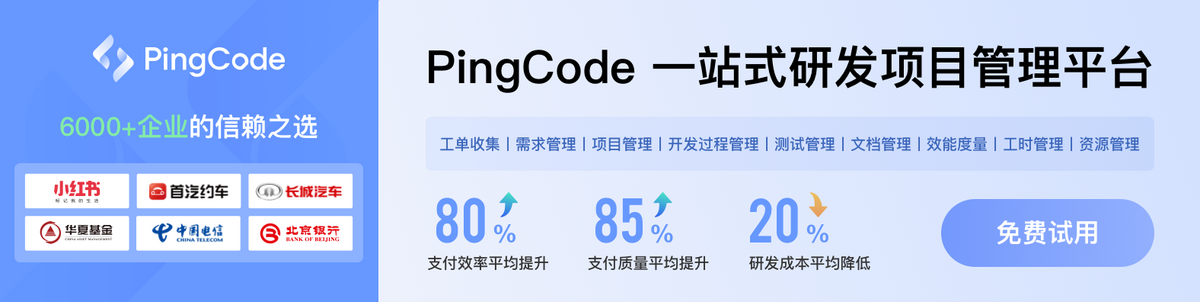