Python读写配置文件可以通过多个方法实现,如使用configparser模块、json模块、以及YAML模块等。 其中,configparser模块是Python标准库中专门用于读取和写入配置文件的模块,json模块则适用于处理JSON格式的配置文件,YAML模块则更适合处理更复杂的配置文件。接下来将详细介绍configparser模块的使用方法。
使用configparser模块读取和写入配置文件
configparser模块提供了一种方便的方式来处理配置文件,这些文件通常以.ini格式保存。这个模块允许你读取、修改和写入配置文件中的数据。
一、CONFIGPARSER模块
1、读取配置文件
首先,我们需要创建一个.ini格式的配置文件,如下所示:
[DEFAULT]
ServerAliveInterval = 45
Compression = yes
CompressionLevel = 9
[bitbucket.org]
User = hg
[topsecret.server.com]
Port = 50022
ForwardX11 = no
然后,我们可以使用configparser模块来读取这个配置文件:
import configparser
创建一个ConfigParser对象
config = configparser.ConfigParser()
读取配置文件
config.read('example.ini')
访问数据
server_alive_interval = config['DEFAULT']['ServerAliveInterval']
compression = config['DEFAULT']['Compression']
compression_level = config['DEFAULT'].getint('CompressionLevel')
打印读取的数据
print(f"ServerAliveInterval: {server_alive_interval}")
print(f"Compression: {compression}")
print(f"CompressionLevel: {compression_level}")
2、修改配置文件
configparser模块还允许我们修改配置文件中的数据,并将修改后的数据写回到配置文件中。
# 修改配置文件中的数据
config['DEFAULT']['ServerAliveInterval'] = '60'
添加新的数据
config['DEFAULT']['Timeout'] = '30'
将修改后的数据写回到配置文件中
with open('example.ini', 'w') as configfile:
config.write(configfile)
3、创建新的配置文件
我们也可以使用configparser模块创建一个新的配置文件:
config = configparser.ConfigParser()
config['DEFAULT'] = {
'ServerAliveInterval': '45',
'Compression': 'yes',
'CompressionLevel': '9'
}
config['bitbucket.org'] = {'User': 'hg'}
config['topsecret.server.com'] = {'Port': '50022', 'ForwardX11': 'no'}
with open('example.ini', 'w') as configfile:
config.write(configfile)
二、JSON模块
1、读取JSON配置文件
JSON是一种轻量级的数据交换格式,非常适合用来存储配置信息。我们可以使用Python的json模块来读取JSON格式的配置文件。
首先,创建一个JSON格式的配置文件,如下所示:
{
"DEFAULT": {
"ServerAliveInterval": 45,
"Compression": "yes",
"CompressionLevel": 9
},
"bitbucket.org": {
"User": "hg"
},
"topsecret.server.com": {
"Port": 50022,
"ForwardX11": "no"
}
}
然后,我们可以使用json模块来读取这个配置文件:
import json
读取JSON配置文件
with open('config.json', 'r') as f:
config = json.load(f)
访问数据
server_alive_interval = config['DEFAULT']['ServerAliveInterval']
compression = config['DEFAULT']['Compression']
compression_level = config['DEFAULT']['CompressionLevel']
打印读取的数据
print(f"ServerAliveInterval: {server_alive_interval}")
print(f"Compression: {compression}")
print(f"CompressionLevel: {compression_level}")
2、修改JSON配置文件
我们可以直接修改读取到的数据,并将修改后的数据写回到配置文件中:
# 修改配置文件中的数据
config['DEFAULT']['ServerAliveInterval'] = 60
添加新的数据
config['DEFAULT']['Timeout'] = 30
将修改后的数据写回到配置文件中
with open('config.json', 'w') as f:
json.dump(config, f, indent=4)
3、创建新的JSON配置文件
我们也可以使用json模块创建一个新的JSON格式的配置文件:
config = {
"DEFAULT": {
"ServerAliveInterval": 45,
"Compression": "yes",
"CompressionLevel": 9
},
"bitbucket.org": {
"User": "hg"
},
"topsecret.server.com": {
"Port": 50022,
"ForwardX11": "no"
}
}
with open('config.json', 'w') as f:
json.dump(config, f, indent=4)
三、YAML模块
1、读取YAML配置文件
YAML是一种专门用于配置文件的格式,比JSON更简洁,更易读。我们可以使用PyYAML库来读取YAML格式的配置文件。
首先,创建一个YAML格式的配置文件,如下所示:
DEFAULT:
ServerAliveInterval: 45
Compression: yes
CompressionLevel: 9
bitbucket.org:
User: hg
topsecret.server.com:
Port: 50022
ForwardX11: no
然后,我们可以使用PyYAML库来读取这个配置文件:
import yaml
读取YAML配置文件
with open('config.yaml', 'r') as f:
config = yaml.safe_load(f)
访问数据
server_alive_interval = config['DEFAULT']['ServerAliveInterval']
compression = config['DEFAULT']['Compression']
compression_level = config['DEFAULT']['CompressionLevel']
打印读取的数据
print(f"ServerAliveInterval: {server_alive_interval}")
print(f"Compression: {compression}")
print(f"CompressionLevel: {compression_level}")
2、修改YAML配置文件
我们可以直接修改读取到的数据,并将修改后的数据写回到配置文件中:
# 修改配置文件中的数据
config['DEFAULT']['ServerAliveInterval'] = 60
添加新的数据
config['DEFAULT']['Timeout'] = 30
将修改后的数据写回到配置文件中
with open('config.yaml', 'w') as f:
yaml.safe_dump(config, f)
3、创建新的YAML配置文件
我们也可以使用PyYAML库创建一个新的YAML格式的配置文件:
config = {
'DEFAULT': {
'ServerAliveInterval': 45,
'Compression': 'yes',
'CompressionLevel': 9
},
'bitbucket.org': {
'User': 'hg'
},
'topsecret.server.com': {
'Port': 50022,
'ForwardX11': 'no'
}
}
with open('config.yaml', 'w') as f:
yaml.safe_dump(config, f)
四、XML模块
1、读取XML配置文件
XML是一种标记语言,适用于存储结构化数据。我们可以使用Python的xml.etree.ElementTree模块来读取XML格式的配置文件。
首先,创建一个XML格式的配置文件,如下所示:
<config>
<DEFAULT>
<ServerAliveInterval>45</ServerAliveInterval>
<Compression>yes</Compression>
<CompressionLevel>9</CompressionLevel>
</DEFAULT>
<bitbucket.org>
<User>hg</User>
</bitbucket.org>
<topsecret.server.com>
<Port>50022</Port>
<ForwardX11>no</ForwardX11>
</topsecret.server.com>
</config>
然后,我们可以使用xml.etree.ElementTree模块来读取这个配置文件:
import xml.etree.ElementTree as ET
读取XML配置文件
tree = ET.parse('config.xml')
root = tree.getroot()
访问数据
server_alive_interval = root.find('DEFAULT/ServerAliveInterval').text
compression = root.find('DEFAULT/Compression').text
compression_level = root.find('DEFAULT/CompressionLevel').text
打印读取的数据
print(f"ServerAliveInterval: {server_alive_interval}")
print(f"Compression: {compression}")
print(f"CompressionLevel: {compression_level}")
2、修改XML配置文件
我们可以直接修改读取到的数据,并将修改后的数据写回到配置文件中:
# 修改配置文件中的数据
root.find('DEFAULT/ServerAliveInterval').text = '60'
添加新的数据
ET.SubElement(root.find('DEFAULT'), 'Timeout').text = '30'
将修改后的数据写回到配置文件中
tree.write('config.xml')
3、创建新的XML配置文件
我们也可以使用xml.etree.ElementTree模块创建一个新的XML格式的配置文件:
config = ET.Element('config')
default = ET.SubElement(config, 'DEFAULT')
ET.SubElement(default, 'ServerAliveInterval').text = '45'
ET.SubElement(default, 'Compression').text = 'yes'
ET.SubElement(default, 'CompressionLevel').text = '9'
bitbucket = ET.SubElement(config, 'bitbucket.org')
ET.SubElement(bitbucket, 'User').text = 'hg'
topsecret = ET.SubElement(config, 'topsecret.server.com')
ET.SubElement(topsecret, 'Port').text = '50022'
ET.SubElement(topsecret, 'ForwardX11').text = 'no'
tree = ET.ElementTree(config)
tree.write('config.xml')
五、总结
Python提供了多种读取和写入配置文件的方法,其中包括configparser模块、json模块、YAML模块和XML模块。configparser模块适用于处理.ini格式的配置文件,json模块适用于处理JSON格式的配置文件,YAML模块适用于处理YAML格式的配置文件,XML模块则适用于处理XML格式的配置文件。 根据具体的需求选择合适的模块,可以大大提高工作的效率。
不同的配置文件格式各有优缺点,.ini文件结构简单易读,适合存储简单的配置信息;JSON格式适合存储层次化的数据,并且易于与其他编程语言互操作;YAML格式更简洁,更易读,适合存储复杂的配置信息;XML格式虽然冗长,但适合存储结构化数据。 在实际应用中,可以根据具体的需求选择合适的配置文件格式及相应的处理模块。
相关问答FAQs:
如何在Python中读取配置文件的最佳实践是什么?
在Python中,读取配置文件时,通常使用configparser
模块。该模块支持INI文件格式,方便地管理配置项。首先需要导入模块,然后创建一个配置对象,使用read
方法加载文件,最后可以通过get
方法获取相应的配置值。为了提高代码的可读性,可以将配置项分组,确保逻辑清晰。
Python支持哪些格式的配置文件?
Python支持多种配置文件格式,包括INI、JSON、YAML和XML等。configparser
适用于INI格式,json
模块可以处理JSON格式,而PyYAML
库则用于YAML文件。这些格式各有优缺点,INI文件简洁易读,JSON格式则更适合数据交互,YAML格式提供更强的可读性和复杂结构支持。
如何在Python中写入配置文件?
在Python中写入配置文件同样可以使用configparser
模块。创建一个新的配置对象后,可以使用add_section
方法添加新的部分,并通过set
方法定义每个配置项的键值对。最后,使用write
方法将配置写入文件。确保在写入之前,文件路径是正确的,以避免任何I/O错误。
如何处理Python配置文件中的缺失值?
在处理配置文件时,缺失值可能导致程序异常。为了避免这种情况,可以使用get
方法的fallback
参数提供默认值。此外,在读取配置文件之前,可以使用has_section
和has_option
方法检查特定部分或选项是否存在,确保代码的健壮性。这样可以优雅地处理意外情况,确保程序平稳运行。
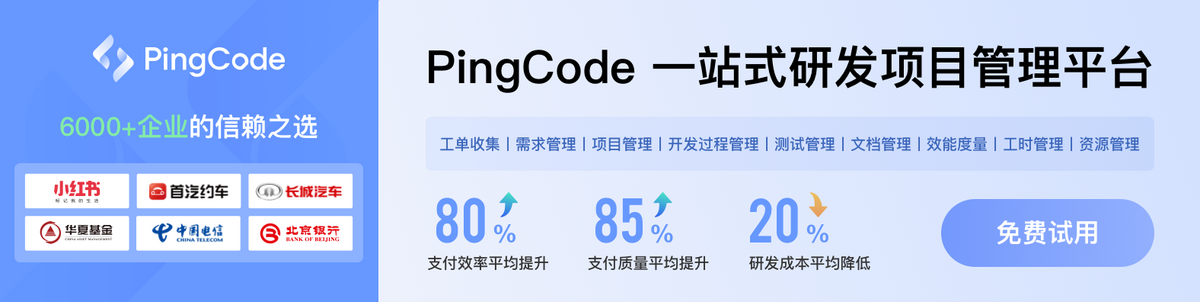