Python 中不需要单独安装 urllib2,因为它是 Python 标准库的一部分。urllib2 是 Python 2.x 中用于处理 URL 请求的库,但在 Python 3.x 中,urllib2 已被拆分并重新命名为 urllib.request 和 urllib.error。 如果你使用的是 Python 3.x,请使用 urllib.request 代替 urllib2。以下是如何在 Python 3 中使用 urllib.request 的详细描述。
一、URLOPEN 用法
在 Python 3 中,urllib.request 模块提供了一个与 urllib2 类似的函数 urlopen 来打开 URL 并读取其内容。我们来看一个简单的示例:
import urllib.request
url = 'http://www.example.com'
response = urllib.request.urlopen(url)
html = response.read()
print(html)
在这个示例中,我们首先导入 urllib.request 模块,然后使用 urlopen 打开指定的 URL,并读取其内容。
二、处理 HTTP 请求
1、GET 请求
GET 请求是最常见的 HTTP 请求方法,用于从服务器检索数据。我们来看一个示例:
import urllib.request
url = 'http://www.example.com'
response = urllib.request.urlopen(url)
html = response.read()
print(html.decode('utf-8'))
在这个示例中,我们使用 urlopen 发送一个 GET 请求,并读取响应内容。
2、POST 请求
POST 请求用于向服务器发送数据。我们可以使用 urllib.request 模块中的 Request 类来构造一个 POST 请求:
import urllib.request
import urllib.parse
url = 'http://www.example.com'
data = urllib.parse.urlencode({'key': 'value'}).encode('utf-8')
req = urllib.request.Request(url, data)
response = urllib.request.urlopen(req)
result = response.read()
print(result.decode('utf-8'))
在这个示例中,我们首先使用 urllib.parse.urlencode 将数据编码为 URL 编码格式,然后将其转换为字节字符串。接着,我们使用 Request 类构造一个 POST 请求,并使用 urlopen 发送请求。
三、处理 HTTP 响应
在处理 HTTP 响应时,我们可能需要检查响应的状态码和头信息:
import urllib.request
url = 'http://www.example.com'
response = urllib.request.urlopen(url)
print('Status:', response.status)
print('Headers:', response.getheaders())
print('Content:', response.read().decode('utf-8'))
在这个示例中,我们使用 response.status 获取响应状态码,使用 response.getheaders 获取响应头信息,并使用 response.read 读取响应内容。
四、错误处理
在处理 HTTP 请求时,我们可能会遇到错误。urllib.error 模块提供了几个异常类来处理这些错误:
import urllib.request
import urllib.error
url = 'http://www.nonexistentwebsite.com'
try:
response = urllib.request.urlopen(url)
except urllib.error.HTTPError as e:
print('HTTPError: ', e.code)
except urllib.error.URLError as e:
print('URLError: ', e.reason)
else:
print('Content:', response.read().decode('utf-8'))
在这个示例中,我们使用 try-except 语句来捕获 HTTPError 和 URLError 异常,并分别处理它们。
五、添加请求头
有时候我们需要在发送请求时添加一些请求头,例如 User-Agent:
import urllib.request
url = 'http://www.example.com'
headers = {'User-Agent': 'Mozilla/5.0'}
req = urllib.request.Request(url, headers=headers)
response = urllib.request.urlopen(req)
html = response.read()
print(html.decode('utf-8'))
在这个示例中,我们通过向 Request 构造函数传递一个字典来添加请求头。
六、处理 Cookies
在处理需要登录的网站时,我们需要管理 Cookies。我们可以使用 http.cookiejar 模块来处理 Cookies:
import urllib.request
import http.cookiejar
url = 'http://www.example.com'
创建一个 CookieJar 对象来保存 Cookies
cj = http.cookiejar.CookieJar()
创建一个带有 CookieJar 对象的 HTTPCookieProcessor
opener = urllib.request.build_opener(urllib.request.HTTPCookieProcessor(cj))
使用 opener 发送请求
response = opener.open(url)
print(response.read().decode('utf-8'))
打印 Cookies
for cookie in cj:
print('Cookie:', cookie)
在这个示例中,我们创建了一个 CookieJar 对象来保存 Cookies,并使用 HTTPCookieProcessor 来处理 Cookies。
七、代理设置
如果需要通过代理服务器访问互联网,可以使用 ProxyHandler 来设置代理:
import urllib.request
url = 'http://www.example.com'
proxy = 'http://proxyserver:port'
创建一个 ProxyHandler 对象
proxy_handler = urllib.request.ProxyHandler({'http': proxy, 'https': proxy})
创建一个自定义的 opener
opener = urllib.request.build_opener(proxy_handler)
使用 opener 发送请求
response = opener.open(url)
print(response.read().decode('utf-8'))
在这个示例中,我们创建了一个 ProxyHandler 对象来设置代理,并使用这个对象创建了一个自定义的 opener。
八、超时设置
在发送 HTTP 请求时,我们可以设置超时时间来避免长时间等待:
import urllib.request
url = 'http://www.example.com'
try:
response = urllib.request.urlopen(url, timeout=10)
print(response.read().decode('utf-8'))
except urllib.error.URLError as e:
print('URLError:', e.reason)
在这个示例中,我们在调用 urlopen 时传递了一个 timeout 参数来设置超时时间。
九、使用 SSL
如果需要处理 HTTPS 请求,可以使用 ssl 模块来设置 SSL 上下文:
import urllib.request
import ssl
url = 'https://www.example.com'
创建一个不验证证书的 SSL 上下文
context = ssl._create_unverified_context()
response = urllib.request.urlopen(url, context=context)
print(response.read().decode('utf-8'))
在这个示例中,我们创建了一个不验证证书的 SSL 上下文,并在调用 urlopen 时传递了这个上下文。
十、下载文件
使用 urllib.request 模块也可以方便地下载文件:
import urllib.request
url = 'http://www.example.com/sample.txt'
filename = 'sample.txt'
urllib.request.urlretrieve(url, filename)
print('File downloaded:', filename)
在这个示例中,我们使用 urlretrieve 函数将文件下载到本地。
十一、处理 JSON 数据
在处理 RESTful API 时,我们经常会遇到 JSON 数据。我们可以使用 json 模块来解析 JSON 数据:
import urllib.request
import json
url = 'http://api.example.com/data'
response = urllib.request.urlopen(url)
data = json.loads(response.read().decode('utf-8'))
print(data)
在这个示例中,我们使用 json.loads 将 JSON 字符串解析为 Python 字典。
十二、处理表单数据
我们还可以使用 urllib.parse 模块来处理表单数据:
import urllib.request
import urllib.parse
url = 'http://www.example.com/form'
data = {'name': 'John', 'age': '30'}
encoded_data = urllib.parse.urlencode(data).encode('utf-8')
response = urllib.request.urlopen(url, data=encoded_data)
print(response.read().decode('utf-8'))
在这个示例中,我们使用 urlencode 将表单数据编码为 URL 编码格式,并将其转换为字节字符串,然后发送 POST 请求。
十三、使用代理认证
如果代理服务器需要认证,可以在设置代理时包含认证信息:
import urllib.request
url = 'http://www.example.com'
proxy = 'http://user:password@proxyserver:port'
创建一个 ProxyHandler 对象
proxy_handler = urllib.request.ProxyHandler({'http': proxy, 'https': proxy})
创建一个自定义的 opener
opener = urllib.request.build_opener(proxy_handler)
使用 opener 发送请求
response = opener.open(url)
print(response.read().decode('utf-8'))
在这个示例中,我们在代理地址中包含了用户名和密码。
十四、处理重定向
urllib.request 模块会自动处理 HTTP 重定向,但我们也可以自定义处理重定向:
import urllib.request
class RedirectHandler(urllib.request.HTTPRedirectHandler):
def http_error_301(self, req, fp, code, msg, headers):
print('Redirected to:', headers['Location'])
return super().http_error_301(req, fp, code, msg, headers)
url = 'http://www.example.com'
opener = urllib.request.build_opener(RedirectHandler)
response = opener.open(url)
print(response.read().decode('utf-8'))
在这个示例中,我们自定义了一个 HTTPRedirectHandler 来处理重定向,并打印重定向的目标 URL。
十五、模拟浏览器行为
有时候我们需要模拟浏览器行为来访问某些网站,可以通过设置请求头来实现:
import urllib.request
url = 'http://www.example.com'
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3'
}
req = urllib.request.Request(url, headers=headers)
response = urllib.request.urlopen(req)
print(response.read().decode('utf-8'))
在这个示例中,我们设置了一个常见的浏览器 User-Agent 来模拟浏览器行为。
十六、处理多部分表单数据
在上传文件时,我们需要处理多部分表单数据:
import urllib.request
import mimetypes
url = 'http://www.example.com/upload'
file_path = 'path/to/file.txt'
boundary = '----WebKitFormBoundary7MA4YWxkTrZu0gW'
创建多部分表单数据
data = []
data.append('--' + boundary)
data.append('Content-Disposition: form-data; name="file"; filename="file.txt"')
data.append('Content-Type: ' + mimetypes.guess_type(file_path)[0])
data.append('')
with open(file_path, 'rb') as f:
data.append(f.read())
data.append('--' + boundary + '--')
data.append('')
body = b'\r\n'.join([d.encode('utf-8') if isinstance(d, str) else d for d in data])
headers = {
'Content-Type': 'multipart/form-data; boundary=' + boundary,
'Content-Length': str(len(body))
}
发送请求
req = urllib.request.Request(url, data=body, headers=headers)
response = urllib.request.urlopen(req)
print(response.read().decode('utf-8'))
在这个示例中,我们手动构建了一个多部分表单数据,并设置了适当的请求头。
十七、处理自定义 HTTP 方法
如果需要使用自定义的 HTTP 方法,可以在构造 Request 对象时指定方法:
import urllib.request
url = 'http://www.example.com/resource'
req = urllib.request.Request(url, method='DELETE')
response = urllib.request.urlopen(req)
print(response.read().decode('utf-8'))
在这个示例中,我们使用 DELETE 方法发送了一个请求。
十八、处理压缩内容
有些服务器会返回压缩内容,我们需要解压缩内容:
import urllib.request
import zlib
url = 'http://www.example.com'
headers = {'Accept-Encoding': 'gzip'}
req = urllib.request.Request(url, headers=headers)
response = urllib.request.urlopen(req)
if response.info().get('Content-Encoding') == 'gzip':
data = zlib.decompress(response.read(), 16+zlib.MAX_WBITS)
else:
data = response.read()
print(data.decode('utf-8'))
在这个示例中,我们检查响应头中的 Content-Encoding,如果内容是 gzip 压缩的,则解压缩内容。
十九、使用 HTTPS 证书验证
如果需要使用自签名的 HTTPS 证书,可以使用 ssl 模块来设置 SSL 上下文:
import urllib.request
import ssl
url = 'https://www.example.com'
context = ssl.create_default_context(cafile='path/to/certfile')
response = urllib.request.urlopen(url, context=context)
print(response.read().decode('utf-8'))
在这个示例中,我们创建了一个包含自签名证书的 SSL 上下文。
二十、总结
通过以上示例,我们详细介绍了如何在 Python 3 中使用 urllib.request 模块来处理各种 HTTP 请求,包括 GET、POST 请求,处理响应,错误处理,添加请求头,处理 Cookies,代理设置,超时设置,使用 SSL,下载文件,处理 JSON 数据,处理表单数据,处理多部分表单数据,处理自定义 HTTP 方法,处理压缩内容,使用 HTTPS 证书验证等。希望这些示例能够帮助你更好地理解和使用 urllib.request 模块。
相关问答FAQs:
如何在Python中使用urllib2?
urllib2是Python 2.x中的一个库,主要用于处理URL请求。如果你正在使用Python 2.x,urllib2是默认包含的,无需单独安装。可以通过以下方式导入并使用它:
import urllib2
response = urllib2.urlopen('http://www.example.com')
html = response.read()
print(html)
如果你使用的是Python 3.x,urllib2已被拆分并集成到urllib模块中。可以使用以下方式导入:
import urllib.request
response = urllib.request.urlopen('http://www.example.com')
html = response.read()
print(html)
为什么选择urllib2而不是其他库?
urllib2在处理HTTP请求时提供了丰富的功能,比如支持基本的HTTP认证、处理Cookie、支持HTTP代理等。虽然现今有很多其他库(如requests)可以选择,但urllib2在Python 2.x环境下仍然是一个强大的工具。如果你在使用Python 3.x,可以考虑使用requests库,它更加直观和易于使用。
我该如何解决urllib2安装过程中遇到的问题?
如果在使用urllib2时遇到问题,首先要确认你使用的是Python 2.x,因为在Python 3.x中该模块并不存在。如果你遇到ImportError,可能是因为你的Python环境设置不正确。确保你的Python版本和环境变量配置正确。可以尝试使用virtualenv创建一个隔离的Python环境,确保库的使用不会受到其他项目的影响。
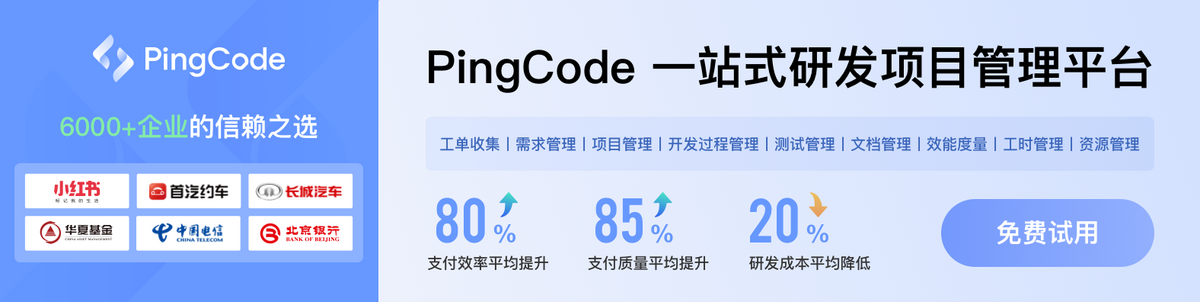