Python可以通过多种方式实现页面跳转,如Flask、Django等Web框架、使用重定向函数redirect、利用模板引擎渲染页面等、利用JavaScript进行前端跳转。本文将详细介绍如何使用这些方法实现页面跳转,包括具体的代码示例和详细的解释,帮助读者更好地理解和应用这些技术。
一、使用Flask实现页面跳转
Flask是一个轻量级的Python Web框架,适合快速开发和小型项目。下面是使用Flask实现页面跳转的示例代码。
1、安装Flask
首先,需要安装Flask。可以使用以下命令安装:
pip install Flask
2、创建Flask应用
创建一个名为app.py的文件,并编写以下代码:
from flask import Flask, redirect, url_for
app = Flask(__name__)
@app.route('/')
def home():
return 'Welcome to the Home Page!'
@app.route('/about')
def about():
return 'This is the About Page.'
@app.route('/redirect_to_about')
def redirect_to_about():
return redirect(url_for('about'))
if __name__ == '__main__':
app.run(debug=True)
3、运行Flask应用
在终端中运行以下命令启动Flask应用:
python app.py
然后,在浏览器中访问http://127.0.0.1:5000/redirect_to_about
,你将被重定向到关于页面(About Page)。
二、使用Django实现页面跳转
Django是一个功能强大的Python Web框架,适合开发复杂的Web应用程序。下面是使用Django实现页面跳转的示例代码。
1、安装Django
首先,需要安装Django。可以使用以下命令安装:
pip install Django
2、创建Django项目
在终端中运行以下命令创建一个新的Django项目:
django-admin startproject myproject
cd myproject
django-admin startapp myapp
3、配置Django应用
在myproject/settings.py文件中添加myapp到INSTALLED_APPS列表中:
INSTALLED_APPS = [
...
'myapp',
]
4、编写视图函数
在myapp/views.py文件中编写以下代码:
from django.shortcuts import render, redirect
def home(request):
return render(request, 'home.html')
def about(request):
return render(request, 'about.html')
def redirect_to_about(request):
return redirect('about')
5、配置URL路由
在myapp/urls.py文件中编写以下代码:
from django.urls import path
from . import views
urlpatterns = [
path('', views.home, name='home'),
path('about/', views.about, name='about'),
path('redirect_to_about/', views.redirect_to_about, name='redirect_to_about'),
]
在myproject/urls.py文件中包含myapp的URL配置:
from django.contrib import admin
from django.urls import include, path
urlpatterns = [
path('admin/', admin.site.urls),
path('', include('myapp.urls')),
]
6、创建模板文件
在myapp目录下创建一个templates目录,并在其中创建home.html和about.html文件。home.html文件内容如下:
<!DOCTYPE html>
<html>
<head>
<title>Home Page</title>
</head>
<body>
<h1>Welcome to the Home Page!</h1>
<a href="{% url 'redirect_to_about' %}">Go to About Page</a>
</body>
</html>
about.html文件内容如下:
<!DOCTYPE html>
<html>
<head>
<title>About Page</title>
</head>
<body>
<h1>This is the About Page.</h1>
</body>
</html>
7、运行Django应用
在终端中运行以下命令启动Django开发服务器:
python manage.py runserver
然后,在浏览器中访问http://127.0.0.1:8000/
,点击页面上的链接,你将被重定向到关于页面(About Page)。
三、使用重定向函数redirect实现页面跳转
在Flask和Django中都可以使用重定向函数redirect实现页面跳转。下面是一个通用的示例代码,展示了如何使用redirect函数实现页面跳转。
1、Flask中的redirect函数
在Flask中,可以使用redirect函数实现页面跳转。下面是一个示例代码:
from flask import Flask, redirect, url_for
app = Flask(__name__)
@app.route('/')
def home():
return 'Welcome to the Home Page!'
@app.route('/about')
def about():
return 'This is the About Page.'
@app.route('/redirect_to_about')
def redirect_to_about():
return redirect(url_for('about'))
if __name__ == '__main__':
app.run(debug=True)
2、Django中的redirect函数
在Django中,可以使用redirect函数实现页面跳转。下面是一个示例代码:
from django.shortcuts import render, redirect
def home(request):
return render(request, 'home.html')
def about(request):
return render(request, 'about.html')
def redirect_to_about(request):
return redirect('about')
四、利用模板引擎渲染页面实现页面跳转
在Flask和Django中都可以使用模板引擎渲染页面,并通过超链接实现页面跳转。下面是一个通用的示例代码,展示了如何使用模板引擎渲染页面并实现页面跳转。
1、Flask中的模板引擎渲染页面
在Flask中,可以使用Jinja2模板引擎渲染页面。下面是一个示例代码:
from flask import Flask, render_template, redirect, url_for
app = Flask(__name__)
@app.route('/')
def home():
return render_template('home.html')
@app.route('/about')
def about():
return render_template('about.html')
@app.route('/redirect_to_about')
def redirect_to_about():
return redirect(url_for('about'))
if __name__ == '__main__':
app.run(debug=True)
在templates目录下创建home.html和about.html文件。home.html文件内容如下:
<!DOCTYPE html>
<html>
<head>
<title>Home Page</title>
</head>
<body>
<h1>Welcome to the Home Page!</h1>
<a href="{{ url_for('redirect_to_about') }}">Go to About Page</a>
</body>
</html>
about.html文件内容如下:
<!DOCTYPE html>
<html>
<head>
<title>About Page</title>
</head>
<body>
<h1>This is the About Page.</h1>
</body>
</html>
2、Django中的模板引擎渲染页面
在Django中,可以使用Django模板引擎渲染页面。下面是一个示例代码:
在myapp/views.py文件中编写以下代码:
from django.shortcuts import render, redirect
def home(request):
return render(request, 'home.html')
def about(request):
return render(request, 'about.html')
def redirect_to_about(request):
return redirect('about')
在myapp目录下创建一个templates目录,并在其中创建home.html和about.html文件。home.html文件内容如下:
<!DOCTYPE html>
<html>
<head>
<title>Home Page</title>
</head>
<body>
<h1>Welcome to the Home Page!</h1>
<a href="{% url 'redirect_to_about' %}">Go to About Page</a>
</body>
</html>
about.html文件内容如下:
<!DOCTYPE html>
<html>
<head>
<title>About Page</title>
</head>
<body>
<h1>This is the About Page.</h1>
</body>
</html>
五、利用JavaScript进行前端跳转
除了使用服务器端的重定向,还可以利用JavaScript在前端实现页面跳转。下面是一个示例代码,展示了如何使用JavaScript进行页面跳转。
1、通过window.location.href跳转
可以使用JavaScript的window.location.href
属性进行页面跳转。下面是一个示例代码:
<!DOCTYPE html>
<html>
<head>
<title>Home Page</title>
</head>
<body>
<h1>Welcome to the Home Page!</h1>
<button onclick="redirectToAbout()">Go to About Page</button>
<script>
function redirectToAbout() {
window.location.href = '/about';
}
</script>
</body>
</html>
2、通过window.location.replace跳转
还可以使用JavaScript的window.location.replace
方法进行页面跳转。下面是一个示例代码:
<!DOCTYPE html>
<html>
<head>
<title>Home Page</title>
</head>
<body>
<h1>Welcome to the Home Page!</h1>
<button onclick="redirectToAbout()">Go to About Page</button>
<script>
function redirectToAbout() {
window.location.replace('/about');
}
</script>
</body>
</html>
六、总结
通过本文的介绍,我们了解了如何使用Python实现页面跳转,包括使用Flask和Django框架、使用重定向函数redirect、利用模板引擎渲染页面以及使用JavaScript进行前端跳转。每种方法都有其独特的优势和适用场景,读者可以根据实际需求选择合适的方法来实现页面跳转。
1、使用Flask实现页面跳转
Flask是一个轻量级的Python Web框架,适合快速开发和小型项目。通过Flask的路由和重定向功能,可以轻松实现页面跳转。
2、使用Django实现页面跳转
Django是一个功能强大的Python Web框架,适合开发复杂的Web应用程序。通过Django的视图和URL路由功能,可以方便地实现页面跳转。
3、使用重定向函数redirect实现页面跳转
在Flask和Django中都可以使用重定向函数redirect实现页面跳转。通过redirect函数,可以将用户重定向到指定的URL。
4、利用模板引擎渲染页面实现页面跳转
在Flask和Django中都可以使用模板引擎渲染页面,并通过超链接实现页面跳转。通过模板引擎,可以动态生成HTML页面,并实现页面跳转。
5、利用JavaScript进行前端跳转
除了使用服务器端的重定向,还可以利用JavaScript在前端实现页面跳转。通过JavaScript的window.location.href
和window.location.replace
方法,可以实现页面跳转。
希望通过本文的介绍,读者能够更好地理解和应用这些技术,实现Python Web应用中的页面跳转。
相关问答FAQs:
Python中有哪些常用的页面跳转方法?
在Python中,页面跳转通常依赖于Web框架,例如Flask或Django。在Flask中,可以使用redirect
函数结合url_for
来实现跳转。例如,return redirect(url_for('target_function'))
。而在Django中,使用HttpResponseRedirect
或redirect
函数也能实现类似的功能。这些方法允许你根据不同的路由或视图进行页面跳转。
如何在Flask应用中实现条件跳转?
在Flask中,可以通过判断条件来决定跳转的目标。例如,在处理表单提交时,可以根据用户输入的值进行不同的跳转。通过if-else
语句,检查条件并使用redirect
结合相应的视图函数实现。例如:
if form.validate_on_submit():
if form.username.data == 'admin':
return redirect(url_for('admin_dashboard'))
else:
return redirect(url_for('user_dashboard'))
这种方式能够灵活地根据不同的情况进行页面跳转。
在Django中如何处理跳转后的数据传递?
在Django中,可以使用HttpResponseRedirect
进行页面跳转,同时通过request.GET
或request.POST
传递数据。如果需要在跳转后保持某些数据,可以考虑使用会话(session)或在URL中添加查询参数。例如:
from django.shortcuts import redirect
def my_view(request):
request.session['my_data'] = 'value'
return redirect('target_view')
在目标视图中,可以通过request.session.get('my_data')
来获取传递的数据。这种方法可以帮助在不同页面间保持状态和数据。
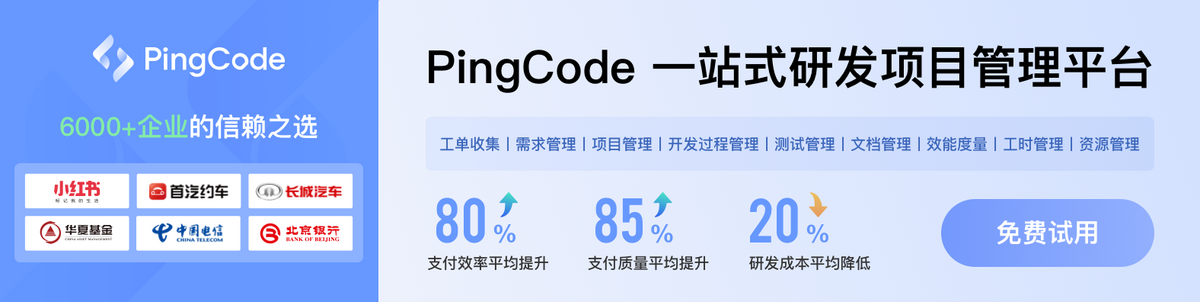