要在Python中运行命令,可以通过以下几种方法:使用os.system()、使用subprocess模块、使用os.popen()。其中,subprocess模块是最常用且推荐的,因为它功能更强大,更加灵活,且能更好地处理输入输出。下面详细介绍如何使用subprocess模块运行命令。
一、使用subprocess模块
subprocess模块是Python中用于运行外部命令和程序的模块。它提供了更高的灵活性和更好的控制,比os.system()更加推荐使用。
1. 基本使用
subprocess.run()是subprocess模块中最常用的函数,用于运行命令并等待其完成。它返回一个CompletedProcess实例,包含了命令的返回码、输出等信息。
import subprocess
result = subprocess.run(['ls', '-l'], capture_output=True, text=True)
print(result.stdout)
在上述代码中,subprocess.run
函数接收一个列表作为参数,其中列表的第一个元素是命令,后续元素是命令的参数。capture_output=True
表示捕获命令的输出,text=True
表示将输出作为字符串处理。
2. 捕获错误输出
有时,我们需要捕获命令执行过程中的错误输出,可以通过stderr
参数实现。
import subprocess
result = subprocess.run(['ls', '-l', '/nonexistent'], capture_output=True, text=True)
print('stdout:', result.stdout)
print('stderr:', result.stderr)
在上述代码中,如果目录不存在,错误信息将被捕获并输出到stderr
。
3. 使用Popen
对于需要更复杂的交互,subprocess.Popen提供了更灵活的接口。它允许我们创建一个子进程,并与之进行通信。
import subprocess
process = subprocess.Popen(['ls', '-l'], stdout=subprocess.PIPE, stderr=subprocess.PIPE, text=True)
stdout, stderr = process.communicate()
print('stdout:', stdout)
print('stderr:', stderr)
在上述代码中,Popen
用于启动子进程,communicate
方法用于与子进程进行通信并获取输出。
二、使用os.system()
os.system()是Python中另一种运行命令的方式,但它功能较为有限,只能返回命令的退出状态,无法捕获命令的输出。
import os
status = os.system('ls -l')
print('Status:', status)
在上述代码中,os.system
函数运行命令并返回其退出状态。虽然简单,但不推荐使用,因为它缺乏对输入输出的控制。
三、使用os.popen()
os.popen()允许我们运行命令并获取其输出,但它已经被弃用,不推荐使用。
import os
output = os.popen('ls -l').read()
print(output)
在上述代码中,os.popen
函数运行命令并返回一个可读的文件对象,通过调用read
方法获取输出。
四、总结
在Python中运行命令有多种方式,但推荐使用subprocess模块,因为它功能强大、灵活性高,能够满足大多数需求。具体使用时,可以根据需求选择subprocess.run
或subprocess.Popen
。
SUBPROCESS模块的高级用法
1. 运行带有输入的命令
有时候我们需要向命令提供输入,例如使用管道符号时,可以通过input
参数实现。
import subprocess
result = subprocess.run(['python3', '-c', 'print(input())'], input='Hello, World!', capture_output=True, text=True)
print(result.stdout)
上述代码运行了一个Python命令,向其提供输入并捕获输出。
2. 设置环境变量
我们可以通过env
参数为子进程设置环境变量。
import subprocess
import os
my_env = os.environ.copy()
my_env['MY_VARIABLE'] = 'value'
result = subprocess.run(['printenv', 'MY_VARIABLE'], capture_output=True, text=True, env=my_env)
print(result.stdout)
上述代码通过env
参数设置了一个环境变量,并在子进程中使用printenv
命令打印该变量。
3. 超时控制
我们可以通过timeout
参数为命令设置超时,防止命令运行时间过长。
import subprocess
try:
result = subprocess.run(['sleep', '10'], timeout=5)
except subprocess.TimeoutExpired:
print('Command timed out')
上述代码设置了一个5秒的超时,如果命令超过5秒未完成,将引发TimeoutExpired
异常。
4. 管道通信
有时候我们需要通过管道将一个命令的输出作为另一个命令的输入,可以使用Popen
和PIPE
实现。
import subprocess
p1 = subprocess.Popen(['echo', 'Hello, World!'], stdout=subprocess.PIPE)
p2 = subprocess.Popen(['grep', 'World'], stdin=p1.stdout, stdout=subprocess.PIPE)
output, _ = p2.communicate()
print(output.decode())
上述代码将echo
命令的输出通过管道传递给grep
命令,并捕获最终输出。
SUBPROCESS模块的错误处理
在使用subprocess模块时,处理错误是非常重要的。我们可以通过捕获异常来处理常见的错误。
1. 捕获CalledProcessError
当命令返回非零退出状态时,将引发subprocess.CalledProcessError
异常。
import subprocess
try:
result = subprocess.run(['ls', '/nonexistent'], check=True)
except subprocess.CalledProcessError as e:
print('Error:', e)
上述代码在命令返回非零退出状态时捕获异常并输出错误信息。
2. 捕获FileNotFoundError
当命令不存在时,将引发FileNotFoundError
异常。
import subprocess
try:
result = subprocess.run(['nonexistent_command'], check=True)
except FileNotFoundError as e:
print('Error:', e)
上述代码在命令不存在时捕获异常并输出错误信息。
SUBPROCESS模块的性能优化
在某些情况下,运行命令可能会影响性能,特别是当需要处理大量数据时。以下是一些优化建议:
1. 使用异步IO
对于长时间运行的命令,可以考虑使用异步IO来提高性能。Python的asyncio
模块提供了对异步IO的支持。
import asyncio
import subprocess
async def run_command(cmd):
process = await asyncio.create_subprocess_shell(
cmd, stdout=subprocess.PIPE, stderr=subprocess.PIPE)
stdout, stderr = await process.communicate()
print('stdout:', stdout.decode())
print('stderr:', stderr.decode())
asyncio.run(run_command('ls -l'))
上述代码使用asyncio
模块异步运行命令,提高了性能。
2. 并行运行命令
对于可以并行运行的命令,可以使用多线程或多进程来提高性能。
import subprocess
from concurrent.futures import ThreadPoolExecutor
def run_command(cmd):
result = subprocess.run(cmd, capture_output=True, text=True)
print(result.stdout)
commands = [['ls', '-l'], ['echo', 'Hello, World!']]
with ThreadPoolExecutor() as executor:
executor.map(run_command, commands)
上述代码使用ThreadPoolExecutor
并行运行多个命令,提高了性能。
SUBPROCESS模块的跨平台兼容性
在使用subprocess模块时,需要注意跨平台兼容性。不同操作系统的命令和路径格式可能不同,因此需要根据操作系统进行适配。
1. 检测操作系统
可以通过os.name
和platform.system()
检测操作系统,并根据操作系统选择不同的命令。
import os
import platform
if os.name == 'nt':
cmd = ['dir']
else:
cmd = ['ls', '-l']
result = subprocess.run(cmd, capture_output=True, text=True)
print(result.stdout)
上述代码根据操作系统选择不同的命令,确保跨平台兼容。
2. 使用绝对路径
为了确保命令在不同操作系统上运行正常,最好使用绝对路径。
import subprocess
result = subprocess.run(['/usr/bin/ls', '-l'], capture_output=True, text=True)
print(result.stdout)
上述代码使用绝对路径运行命令,确保跨平台兼容。
结论
通过以上介绍,我们可以看到,Python提供了多种方式来运行命令,其中subprocess模块是最推荐的,因为它功能强大、灵活性高,能够满足大多数需求。在实际使用中,可以根据具体需求选择合适的方法,并注意处理错误、优化性能和确保跨平台兼容性。通过合理使用这些工具,我们可以在Python中高效地运行和管理命令,提高开发效率和程序性能。
相关问答FAQs:
如何在命令行中检查我的Python版本?
在命令行中,您可以通过输入python --version
或python3 --version
来检查您安装的Python版本。这将显示您当前系统中Python的版本信息,确保您使用的是正确的版本。
在命令行中运行Python脚本需要注意哪些事项?
运行Python脚本时,确保您在命令行中导航到脚本所在的目录。使用cd
命令切换目录后,可以通过输入python script_name.py
或python3 script_name.py
来运行脚本。确保脚本文件具有正确的扩展名(.py),并且代码没有语法错误。
如何在命令行中安装Python库?
您可以使用pip
命令来安装Python库。在命令行中输入pip install library_name
(将library_name
替换为您要安装的库的名称),即可下载并安装所需的库。如果您想确保安装的是特定版本,可以使用pip install library_name==version_number
的格式。
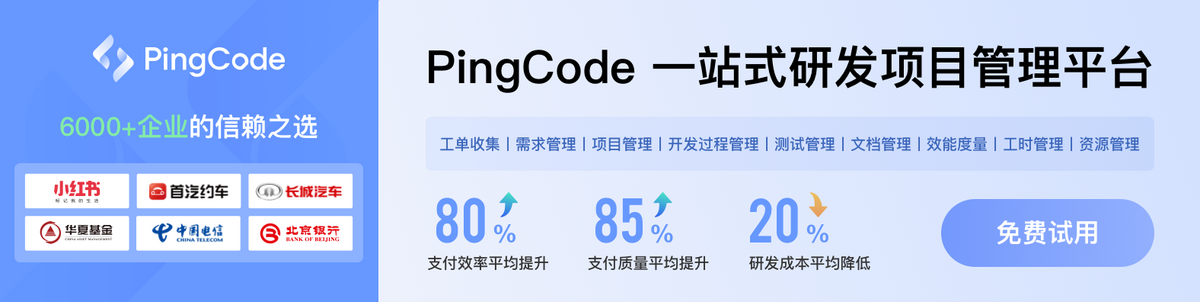