在Python中,可以通过input()
函数实现键盘输入、输入的数据默认是字符串、可以通过类型转换函数将输入的数据转换为其他类型。在Python中,键盘输入是通过调用input()
函数实现的。这个函数会暂停程序的执行,直到用户输入数据并按下回车键为止。输入的数据默认以字符串形式返回,因此,如果需要使用其他数据类型,需要进行类型转换。下面将详细介绍如何使用input()
函数进行键盘输入、处理输入数据及一些常见的应用场景。
一、INPUT()函数的基本用法
input()
函数是Python中用于从标准输入设备(通常是键盘)读取一行文本的内置函数。其基本语法如下:
variable = input("Prompt message: ")
- 提示信息:
input()
函数可以接受一个可选参数,即提示信息。当调用input()
函数时,提示信息会显示在控制台上,提醒用户输入数据。 - 返回值:
input()
函数返回用户输入的字符串。无论用户输入什么内容,返回值始终是字符串类型。
例子:
name = input("Please enter your name: ")
print("Hello, " + name + "!")
在这个例子中,程序会提示用户输入名字,然后输出一条问候消息。
二、类型转换
由于input()
函数的返回值始终是字符串类型,因此在需要进行数值计算或其他类型的操作时,必须进行类型转换。Python提供了多种类型转换函数,例如int()
、float()
、str()
等。
- 整数转换
如果希望用户输入整数,可以使用int()
函数进行转换:
age = int(input("Please enter your age: "))
print("You are " + str(age) + " years old.")
- 浮点数转换
同样地,如果需要输入浮点数,可以使用float()
函数:
height = float(input("Please enter your height in meters: "))
print("Your height is " + str(height) + " meters.")
三、处理异常输入
在实际应用中,用户的输入可能并不总是符合预期,例如输入非数字字符导致类型转换失败。为了提高程序的健壮性,可以使用try-except
语句来处理异常输入。
try:
number = int(input("Please enter an integer: "))
print("You entered:", number)
except ValueError:
print("Invalid input. Please enter a valid integer.")
在这个例子中,如果用户输入的不是整数,将捕获ValueError
异常并提示用户重新输入。
四、多种输入场景
- 读取多个输入
通过split()
方法,可以在一行中读取多个输入值:
data = input("Enter two numbers separated by a space: ").split()
num1 = int(data[0])
num2 = int(data[1])
print("The sum is:", num1 + num2)
- 输入列表
用户可以输入一系列值,并通过split()
和列表推导式将其转换为列表:
numbers = input("Enter numbers separated by space: ").split()
numbers = [int(x) for x in numbers]
print("The list of numbers is:", numbers)
- 处理布尔输入
对于需要布尔值输入的场景,可以根据用户输入的字符串进行判断:
response = input("Do you agree? (yes/no): ").strip().lower()
if response == "yes":
print("Agreed.")
elif response == "no":
print("Not agreed.")
else:
print("Invalid response.")
五、优化用户输入体验
- 提供默认值
在某些情况下,您可能希望为输入提供默认值。这可以通过在提示信息中包含默认值,并在用户输入为空时使用默认值来实现:
default_age = 25
age_input = input(f"Enter your age (default {default_age}): ").strip()
age = int(age_input) if age_input else default_age
print("Your age is:", age)
- 限制输入长度
可以使用len()
函数限制输入字符串的长度:
username = input("Enter your username (max 10 characters): ")
if len(username) > 10:
print("Username is too long!")
else:
print("Welcome,", username)
- 输入验证
在某些情况下,需要对用户输入进行更复杂的验证。例如,验证电子邮件地址格式:
import re
email = input("Enter your email address: ")
email_pattern = r"^[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,}$"
if re.match(email_pattern, email):
print("Valid email address.")
else:
print("Invalid email address.")
六、综合示例
以下是一个综合示例,展示如何使用input()
函数实现一个简单的交互式程序:
def get_user_info():
try:
name = input("Enter your name: ")
age = int(input("Enter your age: "))
height = float(input("Enter your height in meters: "))
response = input("Do you agree to the terms? (yes/no): ").strip().lower()
if response not in ("yes", "no"):
raise ValueError("Invalid response.")
user_info = {
"name": name,
"age": age,
"height": height,
"agreed": response == "yes"
}
return user_info
except ValueError as e:
print("Error:", e)
return None
def main():
print("Welcome to the user information program!")
user_info = get_user_info()
if user_info:
print("User information collected successfully:")
print("Name:", user_info["name"])
print("Age:", user_info["age"])
print("Height:", user_info["height"])
print("Agreed to terms:", "Yes" if user_info["agreed"] else "No")
else:
print("Failed to collect user information.")
if __name__ == "__main__":
main()
在这个例子中,程序会提示用户输入名字、年龄、身高,并询问是否同意条款。输入信息会被存储在字典中,并在输入成功后进行显示。
总结来说,Python中的input()
函数提供了一种简单而强大的方式来获取用户输入。通过适当的类型转换和错误处理,可以确保程序能够处理各种输入场景,并提供良好的用户体验。在实际应用中,合理使用提示信息、默认值和输入验证,可以进一步优化用户输入体验,提升程序的可用性和健壮性。
相关问答FAQs:
如何在Python中实现键盘输入的功能?
在Python中,可以使用内置的input()
函数来实现键盘输入。这个函数会暂停程序的运行,等待用户输入数据。当用户输入完成后,程序会继续执行,并返回输入的数据。使用示例如下:
user_input = input("请输入一些内容:")
print("您输入的内容是:", user_input)
Python输入的内容可以是哪些类型的数据?
使用input()
函数获取的所有输入内容默认都是字符串类型。如果需要其他类型,比如整数或浮点数,可以在获取输入后进行类型转换。例如:
age = int(input("请输入您的年龄:"))
print("您的年龄是:", age)
这种方式将用户输入的字符串转换为整数。
如何处理用户在键盘输入时的异常情况?
在进行键盘输入时,用户可能输入不符合预期的内容,导致程序出错。为了提高程序的健壮性,可以使用try...except
语句来捕获和处理异常。示例如下:
try:
number = int(input("请输入一个数字:"))
print("您输入的数字是:", number)
except ValueError:
print("输入无效,请输入一个有效的数字。")
通过这种方式,程序能够友好地提示用户重新输入。
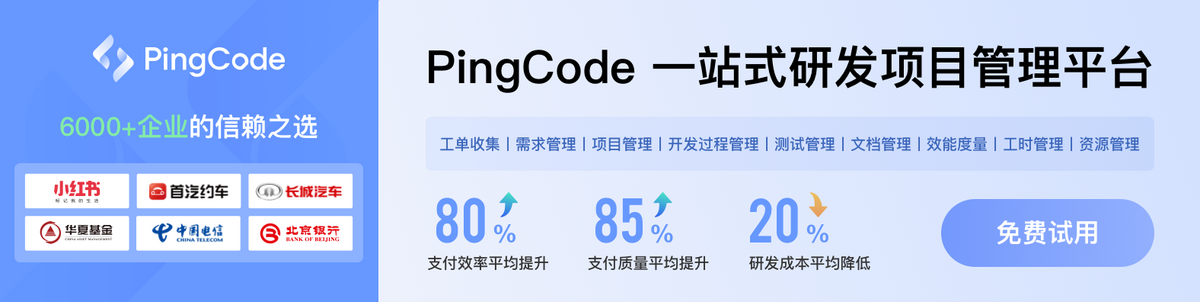