使用Python爬取网站标题,可以通过以下几种方法:requests库、BeautifulSoup库、Scrapy框架、Selenium库。 其中,requests库和BeautifulSoup库的组合是最常见的方法。下面我们将详细介绍如何使用这两种库爬取网站标题。
requests库和BeautifulSoup库的组合:
requests库用于发送HTTP请求并获取响应,BeautifulSoup库用于解析HTML文档并提取所需数据。通过这两个库的组合,我们可以轻松实现对网页标题的爬取。
一、安装所需库
在使用requests和BeautifulSoup库之前,需要先安装这两个库。可以使用以下命令进行安装:
pip install requests
pip install beautifulsoup4
二、发送HTTP请求
首先,使用requests库发送HTTP请求,获取网页的响应内容。以下是一个简单的示例,展示了如何发送HTTP GET请求并获取网页内容:
import requests
url = 'http://example.com'
response = requests.get(url)
html_content = response.text
在上面的代码中,我们使用requests.get(url)
方法发送HTTP GET请求,并将响应内容存储在html_content
变量中。
三、解析HTML文档
接下来,使用BeautifulSoup库解析HTML文档,并提取网站的标题。以下是一个示例,展示了如何使用BeautifulSoup库解析HTML文档并提取标题:
from bs4 import BeautifulSoup
soup = BeautifulSoup(html_content, 'html.parser')
title = soup.title.string
print(title)
在上面的代码中,我们使用BeautifulSoup(html_content, 'html.parser')
方法解析HTML文档,并使用soup.title.string
方法提取网页的标题。
四、完整示例代码
下面是一个完整的示例代码,展示了如何使用requests和BeautifulSoup库爬取网站标题:
import requests
from bs4 import BeautifulSoup
def get_website_title(url):
response = requests.get(url)
if response.status_code == 200:
html_content = response.text
soup = BeautifulSoup(html_content, 'html.parser')
title = soup.title.string
return title
else:
return None
url = 'http://example.com'
title = get_website_title(url)
if title:
print(f'Title of the website "{url}" is: {title}')
else:
print(f'Failed to retrieve the title of the website "{url}".')
在上面的代码中,我们定义了一个get_website_title
函数,用于发送HTTP请求并解析HTML文档,最终返回网站的标题。我们还添加了错误处理逻辑,以便在请求失败时返回None
。
五、处理不同的网页结构
在实际应用中,不同的网页结构可能导致我们无法直接使用soup.title.string
方法提取标题。我们可以通过分析网页的HTML结构,使用BeautifulSoup库提供的其他方法来提取标题。例如,有些网页可能将标题存储在特定的标签中,如<h1>
标签。以下是一个示例,展示了如何提取<h1>
标签中的标题:
def get_website_title(url):
response = requests.get(url)
if response.status_code == 200:
html_content = response.text
soup = BeautifulSoup(html_content, 'html.parser')
title_tag = soup.find('h1')
if title_tag:
return title_tag.string
else:
return None
else:
return None
在上面的代码中,我们使用soup.find('h1')
方法查找第一个<h1>
标签,并提取其内容。
六、处理动态网页
对于一些使用JavaScript动态加载内容的网页,requests和BeautifulSoup库可能无法直接获取到所需内容。这时,我们可以使用Selenium库来处理动态网页。
安装Selenium库
首先,需要安装Selenium库和对应的浏览器驱动。以Chrome浏览器为例,可以使用以下命令安装Selenium库和ChromeDriver:
pip install selenium
下载ChromeDriver后,将其路径添加到系统环境变量中。
使用Selenium库
以下是一个使用Selenium库爬取网站标题的示例代码:
from selenium import webdriver
from selenium.webdriver.chrome.service import Service
from selenium.webdriver.common.by import By
from selenium.webdriver.chrome.options import Options
def get_website_title(url):
options = Options()
options.headless = True
service = Service('/path/to/chromedriver')
driver = webdriver.Chrome(service=service, options=options)
driver.get(url)
title = driver.title
driver.quit()
return title
url = 'http://example.com'
title = get_website_title(url)
if title:
print(f'Title of the website "{url}" is: {title}')
else:
print(f'Failed to retrieve the title of the website "{url}".')
在上面的代码中,我们使用Selenium库启动Chrome浏览器,加载指定网址,并提取网页的标题。最后,关闭浏览器并返回标题。
七、总结
通过以上方法,我们可以使用Python爬取网站标题。根据不同的网页结构和需求,可以选择使用requests和BeautifulSoup库的组合,或者使用Selenium库处理动态网页。通过灵活运用这些工具,我们可以有效地完成网页数据爬取任务。
八、进阶技巧
在实际项目中,爬取网站标题可能会遇到一些特殊情况和挑战。下面介绍一些进阶技巧,以应对这些情况。
1. 处理反爬机制
一些网站会使用反爬机制来阻止自动化爬虫访问。常见的反爬机制包括IP封禁、验证码、请求头检测等。为了绕过这些反爬机制,可以尝试以下方法:
- 使用代理IP:通过使用代理IP,可以避免因频繁请求而被封禁IP。可以使用免费代理IP服务或购买付费代理IP。
proxies = {
'http': 'http://your_proxy_ip:port',
'https': 'http://your_proxy_ip:port'
}
response = requests.get(url, proxies=proxies)
- 模拟浏览器请求头:通过设置请求头,将爬虫伪装成正常的浏览器请求。常见的请求头包括User-Agent、Referer、Cookie等。
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/91.0.4472.124 Safari/537.36'
}
response = requests.get(url, headers=headers)
- 处理验证码:对于需要输入验证码的网站,可以使用OCR技术识别验证码,或者通过手动输入验证码。
2. 提取多种标题信息
有些网页可能包含多种标题信息,例如网页标题、文章标题、副标题等。为了提取这些标题信息,可以结合使用BeautifulSoup库的多种方法。
def get_website_titles(url):
response = requests.get(url)
if response.status_code == 200:
html_content = response.text
soup = BeautifulSoup(html_content, 'html.parser')
titles = {
'page_title': soup.title.string if soup.title else None,
'article_title': soup.find('h1').string if soup.find('h1') else None,
'sub_title': soup.find('h2').string if soup.find('h2') else None
}
return titles
else:
return None
url = 'http://example.com'
titles = get_website_titles(url)
if titles:
print(f'Titles of the website "{url}" are: {titles}')
else:
print(f'Failed to retrieve the titles of the website "{url}".')
3. 处理多页数据
在某些情况下,我们需要爬取多个网页的数据,例如分页的文章列表。可以通过循环遍历分页URL,逐页爬取数据。
def get_titles_from_multiple_pages(base_url, page_count):
titles = []
for page in range(1, page_count + 1):
url = f'{base_url}?page={page}'
page_titles = get_website_titles(url)
if page_titles:
titles.append(page_titles)
return titles
base_url = 'http://example.com/articles'
titles = get_titles_from_multiple_pages(base_url, 5)
for i, title in enumerate(titles, 1):
print(f'Page {i} titles: {title}')
九、使用Scrapy框架
Scrapy是一个功能强大的Python爬虫框架,适用于大型爬虫项目。通过使用Scrapy框架,可以更加高效地管理爬虫任务和数据提取。
安装Scrapy
首先,需要安装Scrapy框架,可以使用以下命令进行安装:
pip install scrapy
创建Scrapy项目
创建一个新的Scrapy项目,可以使用以下命令:
scrapy startproject myproject
定义爬虫
在Scrapy项目中,定义一个爬虫,用于爬取网站标题。以下是一个示例代码,展示了如何定义一个爬虫并提取网站标题:
import scrapy
class TitleSpider(scrapy.Spider):
name = 'title_spider'
start_urls = ['http://example.com']
def parse(self, response):
title = response.xpath('//title/text()').get()
yield {'title': title}
在上面的代码中,我们定义了一个名为TitleSpider
的爬虫,并指定了起始URL。使用response.xpath('//title/text()')
方法提取网页标题,并通过yield
语句返回结果。
运行爬虫
可以使用以下命令运行爬虫:
scrapy crawl title_spider -o titles.json
在上面的命令中,我们使用-o
选项指定输出文件,将爬取到的结果保存为JSON格式。
十、总结
通过本文的介绍,我们详细讲解了使用Python爬取网站标题的方法,包括requests库、BeautifulSoup库、Selenium库和Scrapy框架。同时,我们还介绍了一些进阶技巧,以应对实际项目中的特殊情况和挑战。
无论是处理静态网页还是动态网页,Python都提供了丰富的库和工具,帮助我们高效地完成网页数据爬取任务。通过灵活运用这些工具和方法,可以满足各种爬虫需求,提高数据提取的效率和准确性。
相关问答FAQs:
如何使用Python获取网页的标题?
获取网页标题可以通过多种Python库来实现,最常用的包括requests
和BeautifulSoup
。首先,使用requests
库发送HTTP请求获取网页内容,然后利用BeautifulSoup
解析HTML,提取<title>
标签中的文本。代码示例如下:
import requests
from bs4 import BeautifulSoup
url = 'http://example.com'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
title = soup.title.string
print(title)
在爬取网站时,如何处理反爬虫机制?
许多网站会实施反爬虫策略,可能会阻止你的请求或返回错误信息。可以通过设置请求头(如User-Agent)来伪装成浏览器,使用代理IP以避免IP被封禁,或是添加延时,减少请求频率来降低被检测的风险。
Python爬虫如何避免被网站封禁?
为了避免封禁,建议遵循网站的robots.txt
文件中的爬虫规则,限制请求的频率,并使用随机时间间隔发送请求。此外,定期更换IP地址和User-Agent,可以有效降低被封禁的可能性。使用爬虫框架如Scrapy时,能够更方便地实现这些防护措施。
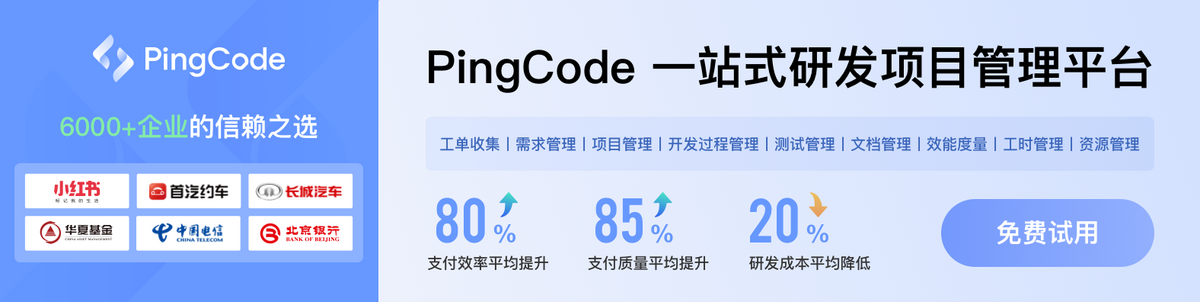