一、如何使用Python爬虫得到网页内容
使用requests库发送HTTP请求、使用BeautifulSoup解析网页内容、处理和保存数据。首先,使用requests库发送HTTP请求获取网页的HTML内容。然后,使用BeautifulSoup解析HTML内容,并提取所需的数据。最后,处理和保存获取到的数据。接下来将详细描述其中的请求与解析过程。
发送HTTP请求是Python爬虫的第一步。我们可以使用requests库发送GET或POST请求,并获得响应内容。requests库使用简单,只需几行代码即可获取网页内容。例如:
import requests
url = 'http://example.com'
response = requests.get(url)
html_content = response.text
二、发送HTTP请求
1、requests库简介
requests库是一个用于发送HTTP请求的Python库。它使得HTTP请求变得非常简单,并且支持GET、POST、PUT、DELETE等多种请求方式。requests库的使用非常广泛,是构建爬虫程序的基础工具之一。
2、发送GET请求
GET请求是最常用的请求方式,用于从服务器获取数据。使用requests库发送GET请求非常简单,只需调用requests.get()方法并传入URL即可。例如:
import requests
url = 'http://example.com'
response = requests.get(url)
html_content = response.text
在上述代码中,requests.get()方法返回一个响应对象,使用response.text可以获取响应的HTML内容。
3、发送POST请求
POST请求通常用于向服务器提交数据。使用requests库发送POST请求可以通过requests.post()方法实现。例如:
import requests
url = 'http://example.com/login'
data = {'username': 'user', 'password': 'pass'}
response = requests.post(url, data=data)
html_content = response.text
在上述代码中,data参数用于传递POST请求的表单数据。
4、处理响应
requests库的响应对象包含了许多有用的信息,例如状态码、头信息、内容等。常用属性包括:
- response.status_code:响应状态码
- response.headers:响应头信息
- response.text:响应内容(字符串形式)
- response.content:响应内容(二进制形式)
例如:
import requests
url = 'http://example.com'
response = requests.get(url)
if response.status_code == 200:
html_content = response.text
print(html_content)
else:
print(f"Failed to retrieve page, status code: {response.status_code}")
三、解析网页内容
1、BeautifulSoup简介
BeautifulSoup是一个用于解析HTML和XML文档的Python库。它提供了简单的API,使得解析和提取网页内容变得非常容易。BeautifulSoup可以与lxml或html.parser等解析器配合使用。
2、创建BeautifulSoup对象
创建BeautifulSoup对象的第一步是将HTML内容传递给BeautifulSoup类的构造函数,并指定解析器。例如:
from bs4 import BeautifulSoup
html_content = '<html><head><title>Example</title></head><body><p>Hello, world!</p></body></html>'
soup = BeautifulSoup(html_content, 'html.parser')
3、查找元素
BeautifulSoup提供了多种方法用于查找元素,例如find()、find_all()、select()等。常用的查找方法包括:
- find(name, attrs, recursive, text, kwargs):查找第一个符合条件的元素
- find_all(name, attrs, recursive, text, limit, kwargs):查找所有符合条件的元素
- select(selector):使用CSS选择器查找元素
例如:
from bs4 import BeautifulSoup
html_content = '<html><head><title>Example</title></head><body><p>Hello, world!</p></body></html>'
soup = BeautifulSoup(html_content, 'html.parser')
title = soup.find('title').text
paragraph = soup.find('p').text
print(f"Title: {title}")
print(f"Paragraph: {paragraph}")
4、提取属性和文本
BeautifulSoup对象可以轻松提取元素的属性和文本内容。例如:
from bs4 import BeautifulSoup
html_content = '<html><body><a href="http://example.com">Link</a></body></html>'
soup = BeautifulSoup(html_content, 'html.parser')
link = soup.find('a')
href = link['href']
text = link.text
print(f"Href: {href}")
print(f"Text: {text}")
四、处理和保存数据
1、数据处理
在获取到所需的数据后,通常需要对数据进行处理。数据处理包括清洗、转换、格式化等操作。例如,将日期字符串转换为日期对象,去除多余的空格等。
2、保存数据
保存数据的方式有很多种,可以将数据保存到文件、数据库或其他存储介质。例如,将数据保存到CSV文件中:
import csv
data = [
{'name': 'Alice', 'age': 25},
{'name': 'Bob', 'age': 30},
]
with open('data.csv', 'w', newline='') as csvfile:
fieldnames = ['name', 'age']
writer = csv.DictWriter(csvfile, fieldnames=fieldnames)
writer.writeheader()
writer.writerows(data)
3、保存数据到数据库
除了文件存储,还可以选择将数据保存到数据库中。Python提供了多种数据库接口,例如SQLite、MySQL、PostgreSQL等。下面是将数据保存到SQLite数据库的示例:
import sqlite3
连接到SQLite数据库(如果数据库不存在,则会自动创建)
conn = sqlite3.connect('example.db')
cursor = conn.cursor()
创建表
cursor.execute('''
CREATE TABLE IF NOT EXISTS users (
id INTEGER PRIMARY KEY,
name TEXT,
age INTEGER
)
''')
插入数据
data = [
('Alice', 25),
('Bob', 30),
]
cursor.executemany('INSERT INTO users (name, age) VALUES (?, ?)', data)
提交事务
conn.commit()
查询数据
cursor.execute('SELECT * FROM users')
rows = cursor.fetchall()
for row in rows:
print(row)
关闭连接
conn.close()
五、处理动态网页
1、使用Selenium
有些网页是通过JavaScript动态加载内容的,requests库无法直接获取这些内容。这时候可以使用Selenium库,它是一个自动化测试工具,可以驱动浏览器执行操作,并获取动态加载的内容。
2、安装Selenium
首先,需要安装Selenium库和浏览器驱动。例如,使用pip安装Selenium:
pip install selenium
根据所使用的浏览器下载相应的驱动程序(例如ChromeDriver、GeckoDriver),并确保驱动程序在系统路径中。
3、使用Selenium获取动态内容
下面是一个使用Selenium获取动态网页内容的示例:
from selenium import webdriver
from selenium.webdriver.chrome.service import Service
from selenium.webdriver.common.by import By
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
创建浏览器对象
service = Service('path/to/chromedriver')
driver = webdriver.Chrome(service=service)
打开网页
url = 'http://example.com'
driver.get(url)
等待元素加载完成
wait = WebDriverWait(driver, 10)
element = wait.until(EC.presence_of_element_located((By.ID, 'dynamic-content')))
获取动态内容
html_content = driver.page_source
解析和提取数据
from bs4 import BeautifulSoup
soup = BeautifulSoup(html_content, 'html.parser')
dynamic_content = soup.find(id='dynamic-content').text
print(dynamic_content)
关闭浏览器
driver.quit()
4、模拟用户操作
Selenium还可以模拟用户操作,例如点击按钮、填写表单等。下面是一个模拟用户登录的示例:
from selenium import webdriver
from selenium.webdriver.chrome.service import Service
from selenium.webdriver.common.by import By
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
创建浏览器对象
service = Service('path/to/chromedriver')
driver = webdriver.Chrome(service=service)
打开登录页面
url = 'http://example.com/login'
driver.get(url)
填写表单
username = driver.find_element(By.NAME, 'username')
password = driver.find_element(By.NAME, 'password')
login_button = driver.find_element(By.NAME, 'login')
username.send_keys('user')
password.send_keys('pass')
login_button.click()
等待登录完成
wait = WebDriverWait(driver, 10)
wait.until(EC.presence_of_element_located((By.ID, 'welcome-message')))
获取登录后的内容
html_content = driver.page_source
解析和提取数据
from bs4 import BeautifulSoup
soup = BeautifulSoup(html_content, 'html.parser')
welcome_message = soup.find(id='welcome-message').text
print(welcome_message)
关闭浏览器
driver.quit()
六、处理反爬虫措施
1、设置请求头
一些网站会通过检查请求头来判断是否为爬虫程序。可以通过设置请求头来模拟浏览器请求。例如:
import requests
url = 'http://example.com'
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.36',
}
response = requests.get(url, headers=headers)
html_content = response.text
2、使用代理
有些网站会通过IP地址限制访问频率,可以使用代理来避免被封禁。例如:
import requests
url = 'http://example.com'
proxies = {
'http': 'http://10.10.1.10:3128',
'https': 'http://10.10.1.10:1080',
}
response = requests.get(url, proxies=proxies)
html_content = response.text
3、处理Cookies
有些网站会使用Cookies来跟踪用户,可以使用requests库的Session对象来自动处理Cookies。例如:
import requests
url = 'http://example.com'
session = requests.Session()
response = session.get(url)
html_content = response.text
七、并发爬取
1、使用多线程
为了提高爬取效率,可以使用多线程同时爬取多个网页。可以使用threading库来实现多线程爬取。例如:
import threading
import requests
def fetch_url(url):
response = requests.get(url)
html_content = response.text
print(f"Fetched {url}")
urls = ['http://example.com/page1', 'http://example.com/page2', 'http://example.com/page3']
threads = []
for url in urls:
thread = threading.Thread(target=fetch_url, args=(url,))
threads.append(thread)
thread.start()
for thread in threads:
thread.join()
2、使用多进程
多进程可以更好地利用多核CPU资源,提高爬取效率。可以使用multiprocessing库来实现多进程爬取。例如:
import multiprocessing
import requests
def fetch_url(url):
response = requests.get(url)
html_content = response.text
print(f"Fetched {url}")
urls = ['http://example.com/page1', 'http://example.com/page2', 'http://example.com/page3']
processes = []
for url in urls:
process = multiprocessing.Process(target=fetch_url, args=(url,))
processes.append(process)
process.start()
for process in processes:
process.join()
3、使用协程
协程是一种轻量级的并发方式,可以在单线程中实现高并发。可以使用asyncio库和aiohttp库来实现协程爬取。例如:
import asyncio
import aiohttp
async def fetch_url(session, url):
async with session.get(url) as response:
html_content = await response.text()
print(f"Fetched {url}")
async def main():
urls = ['http://example.com/page1', 'http://example.com/page2', 'http://example.com/page3']
async with aiohttp.ClientSession() as session:
tasks = [fetch_url(session, url) for url in urls]
await asyncio.gather(*tasks)
asyncio.run(main())
八、调试和优化
1、调试技巧
在开发爬虫程序时,调试是非常重要的一环。可以使用以下技巧进行调试:
- 打印调试信息:在关键位置添加print语句,输出变量值和状态信息。
- 使用断点:使用IDE或调试工具在代码中设置断点,逐步执行代码并观察变量值。
- 捕获异常:使用try-except语句捕获异常,输出错误信息,便于定位问题。
例如:
import requests
try:
url = 'http://example.com'
response = requests.get(url)
response.raise_for_status()
html_content = response.text
print(html_content)
except requests.RequestException as e:
print(f"Error: {e}")
2、优化性能
为了提高爬虫程序的性能,可以进行以下优化:
- 减少请求次数:避免重复请求相同的网页,使用缓存或数据库存储已爬取的数据。
- 控制请求频率:设置合理的请求间隔,避免频繁请求导致被封禁。
- 使用高效的解析器:选择合适的HTML解析器(如lxml)提高解析速度。
- 合理使用并发:根据系统资源和目标网站的承受能力,合理设置并发数量。
3、使用日志记录
在爬虫程序中使用日志记录,可以方便地跟踪程序运行状态和调试问题。可以使用logging库进行日志记录。例如:
import logging
import requests
logging.basicConfig(level=logging.INFO, format='%(asctime)s - %(levelname)s - %(message)s')
try:
url = 'http://example.com'
response = requests.get(url)
response.raise_for_status()
html_content = response.text
logging.info(f"Fetched {url}")
except requests.RequestException as e:
logging.error(f"Error: {e}")
总结
通过以上步骤,我们可以使用Python爬虫获取网页内容。首先,使用requests库发送HTTP请求获取网页的HTML内容。然后,使用BeautifulSoup解析HTML内容,并提取所需的数据。对于动态网页,可以使用Selenium库获取动态加载的内容。最后,对数据进行处理和保存。同时,可以通过设置请求头、使用代理、处理Cookies等方式应对反爬虫措施。为了提高爬取效率,可以使用多线程、多进程或协程进行并发爬取。在开发过程中,注意调试和优化,使用日志记录跟踪程序运行状态。通过这些方法,可以构建高效、稳定的Python爬虫程序。
相关问答FAQs:
如何使用Python爬虫获取网页的HTML内容?
使用Python爬虫获取网页内容的常见方法是利用requests
库发送HTTP请求。通过安装requests
库(例如使用pip install requests
),可以轻松获取网页的响应内容。代码示例如下:
import requests
url = 'https://example.com'
response = requests.get(url)
html_content = response.text
print(html_content)
这段代码向指定的URL发送GET请求,并将返回的网页内容存储在html_content
变量中。
在爬取网页内容时如何处理反爬虫机制?
许多网站会实施反爬虫策略以保护其内容。为了绕过这些机制,可以采取一些措施。例如,可以在请求头中添加用户代理(User-Agent)以模拟浏览器请求,或者使用延时请求以减少对服务器的压力。以下是一个示例:
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/91.0.4472.124 Safari/537.36'
}
response = requests.get(url, headers=headers)
通过这种方式,您可以提高获取网页内容的成功率。
如何解析和提取网页中的特定信息?
获取网页内容后,可以使用BeautifulSoup
库对HTML进行解析,从中提取特定信息。首先需要安装该库(例如使用pip install beautifulsoup4
),然后可以通过以下示例代码提取标题标签内容:
from bs4 import BeautifulSoup
soup = BeautifulSoup(html_content, 'html.parser')
title = soup.title.string
print(title)
这段代码会解析HTML内容并提取网页的标题。如果您需要获取其他元素,如特定的类或ID,可以使用find
或find_all
方法进行更精确的选择。
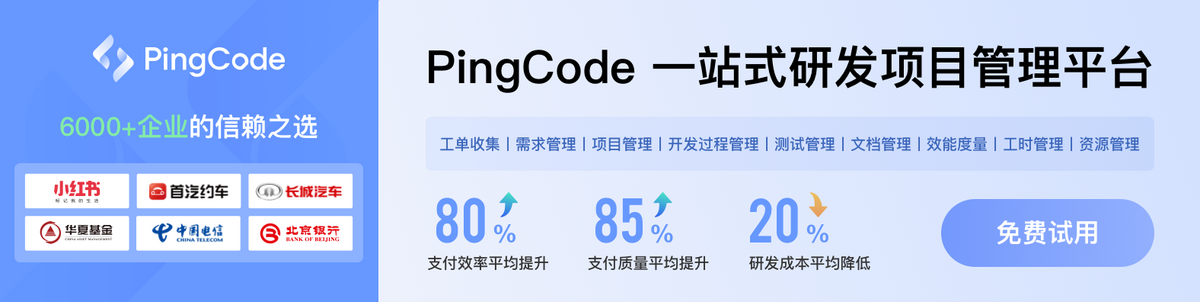