在Python中找到特定字符的方法有很多,使用字符串方法(如find()和index())、使用正则表达式(如re模块)、遍历字符串。这些方法各有优缺点,适用于不同场景。下面将详细介绍其中一种方法:使用字符串方法。
使用字符串方法是最简单和直接的方法之一。Python提供了find()和index()两个方法来查找特定字符或子字符串。find()方法返回特定字符或子字符串首次出现的索引,如果未找到则返回-1;而index()方法则在未找到时抛出ValueError异常。示例如下:
text = "Hello, World!"
char = 'o'
使用find()方法
position = text.find(char)
if position != -1:
print(f"字符'{char}'在索引位置:{position}")
else:
print(f"字符'{char}'未找到")
使用index()方法
try:
position = text.index(char)
print(f"字符'{char}'在索引位置:{position}")
except ValueError:
print(f"字符'{char}'未找到")
接下来,我们将详细介绍在Python中找到特定字符的不同方法和技巧。
一、使用字符串方法
1、find()方法
find()方法是Python字符串中最常用的查找方法之一。它返回字符串中第一次出现指定字符或子字符串的索引位置,如果未找到则返回-1。该方法的基本语法如下:
str.find(sub[, start[, end]])
其中,sub
是要查找的子字符串,start
和end
是可选参数,分别表示查找的开始和结束位置。
示例代码:
text = "Hello, World!"
char = 'o'
查找字符'o'的首次出现位置
position = text.find(char)
print(f"字符'{char}'在索引位置:{position}")
查找子字符串"World"的首次出现位置
substring = "World"
position = text.find(substring)
print(f"子字符串'{substring}'在索引位置:{position}")
指定查找范围
position = text.find(char, 5, 10)
print(f"字符'{char}'在索引位置:{position},查找范围为5到10")
2、index()方法
index()方法与find()方法类似,但它在未找到指定字符或子字符串时会抛出ValueError异常。该方法的基本语法如下:
str.index(sub[, start[, end]])
示例代码:
text = "Hello, World!"
char = 'o'
try:
# 查找字符'o'的首次出现位置
position = text.index(char)
print(f"字符'{char}'在索引位置:{position}")
# 查找子字符串"World"的首次出现位置
substring = "World"
position = text.index(substring)
print(f"子字符串'{substring}'在索引位置:{position}")
# 指定查找范围
position = text.index(char, 5, 10)
print(f"字符'{char}'在索引位置:{position},查找范围为5到10")
except ValueError:
print(f"字符或子字符串未找到")
二、使用正则表达式(re模块)
正则表达式是一个强大的工具,用于复杂的字符串匹配和查找。在Python中,re模块提供了支持正则表达式的功能。使用正则表达式可以更灵活地查找特定字符或模式。
1、search()方法
search()方法用于在字符串中查找第一个匹配的模式,并返回一个匹配对象,如果未找到则返回None。基本语法如下:
re.search(pattern, string, flags=0)
示例代码:
import re
text = "Hello, World!"
pattern = 'o'
使用search()方法查找第一个匹配的模式
match = re.search(pattern, text)
if match:
print(f"匹配的字符'{pattern}'在索引位置:{match.start()}")
else:
print(f"匹配的字符'{pattern}'未找到")
2、findall()方法
findall()方法用于查找字符串中所有匹配的模式,并返回一个列表。基本语法如下:
re.findall(pattern, string, flags=0)
示例代码:
import re
text = "Hello, World! Hello, Python!"
pattern = 'o'
使用findall()方法查找所有匹配的模式
matches = re.findall(pattern, text)
print(f"匹配的字符'{pattern}'出现次数:{len(matches)}")
print(f"匹配的字符'{pattern}'位置:{[m.start() for m in re.finditer(pattern, text)]}")
三、遍历字符串
遍历字符串是一种基本但有效的方法,适用于简单的字符查找。通过遍历字符串中的每个字符,可以找到特定字符或子字符串的所有出现位置。
1、遍历字符串查找字符
示例代码:
text = "Hello, World!"
char = 'o'
positions = []
for i, c in enumerate(text):
if c == char:
positions.append(i)
print(f"字符'{char}'出现位置:{positions}")
2、遍历字符串查找子字符串
示例代码:
text = "Hello, World! Hello, Python!"
substring = "Hello"
positions = []
for i in range(len(text) - len(substring) + 1):
if text[i:i+len(substring)] == substring:
positions.append(i)
print(f"子字符串'{substring}'出现位置:{positions}")
四、使用字符串内置方法和高级技巧
除了上述基本方法,Python还提供了一些高级的字符串内置方法和技巧,可以更方便地处理字符串查找。
1、rfind()方法
rfind()方法类似于find()方法,但它从字符串的右边开始查找。该方法的基本语法如下:
str.rfind(sub[, start[, end]])
示例代码:
text = "Hello, World!"
char = 'o'
使用rfind()方法从右边开始查找字符'o'
position = text.rfind(char)
print(f"字符'{char}'从右边开始查找的索引位置:{position}")
2、rindex()方法
rindex()方法类似于index()方法,但它从字符串的右边开始查找。该方法的基本语法如下:
str.rindex(sub[, start[, end]])
示例代码:
text = "Hello, World!"
char = 'o'
try:
# 使用rindex()方法从右边开始查找字符'o'
position = text.rindex(char)
print(f"字符'{char}'从右边开始查找的索引位置:{position}")
except ValueError:
print(f"字符'{char}'未找到")
3、count()方法
count()方法用于统计字符串中指定字符或子字符串的出现次数。该方法的基本语法如下:
str.count(sub[, start[, end]])
示例代码:
text = "Hello, World! Hello, Python!"
substring = "Hello"
使用count()方法统计子字符串"Hello"的出现次数
count = text.count(substring)
print(f"子字符串'{substring}'出现次数:{count}")
4、字符串切片
字符串切片是一种强大的字符串处理技巧,可以用于更复杂的字符查找和处理。通过字符串切片,可以灵活地获取字符串的子字符串并进行查找。
示例代码:
text = "Hello, World! Hello, Python!"
substring = "Hello"
positions = []
start = 0
while True:
start = text.find(substring, start)
if start == -1:
break
positions.append(start)
start += len(substring)
print(f"子字符串'{substring}'出现位置:{positions}")
五、结合使用多种方法
在实际应用中,结合使用多种方法可以提高字符查找的效率和灵活性。例如,可以先使用正则表达式查找所有匹配的位置,然后结合字符串切片进行处理。
示例代码:
import re
text = "Hello, World! Hello, Python!"
pattern = 'Hello'
使用正则表达式查找所有匹配的位置
matches = [m.start() for m in re.finditer(pattern, text)]
结合字符串切片进行进一步处理
positions = []
for match in matches:
positions.append(match)
print(f"子字符串'{pattern}'出现位置:{positions}")
六、优化字符查找的性能
在处理大规模数据时,字符查找的性能可能会成为瓶颈。为了优化性能,可以采用以下几种方法:
1、使用高效的数据结构
在一些情况下,可以使用高效的数据结构(如字典、集合)来提高查找效率。例如,可以将字符串中的字符存储在字典中,并记录每个字符的出现位置。
示例代码:
text = "Hello, World!"
char_positions = {}
使用字典存储字符的出现位置
for i, char in enumerate(text):
if char in char_positions:
char_positions[char].append(i)
else:
char_positions[char] = [i]
查找字符'o'的出现位置
char = 'o'
positions = char_positions.get(char, [])
print(f"字符'{char}'出现位置:{positions}")
2、使用内置函数和库
Python内置的字符串方法和库函数通常经过高度优化,性能优于自定义实现。在可能的情况下,尽量使用内置函数和库来进行字符查找。
示例代码:
import re
text = "Hello, World! Hello, Python!"
pattern = 'Hello'
使用正则表达式查找所有匹配的位置
matches = [m.start() for m in re.finditer(pattern, text)]
print(f"子字符串'{pattern}'出现位置:{matches}")
3、分而治之
在处理非常大的字符串时,可以将字符串拆分成较小的部分,分别进行查找,然后合并结果。这样可以减少每次查找的范围,提高查找效率。
示例代码:
text = "Hello, World! Hello, Python!"
substring = "Hello"
将字符串拆分成较小的部分
parts = [text[i:i+10] for i in range(0, len(text), 10)]
positions = []
for part_index, part in enumerate(parts):
start = 0
while True:
start = part.find(substring, start)
if start == -1:
break
positions.append(part_index * 10 + start)
start += len(substring)
print(f"子字符串'{substring}'出现位置:{positions}")
七、总结
在Python中找到特定字符的方法多种多样,包括使用字符串方法(如find()和index())、使用正则表达式(如re模块)、遍历字符串。每种方法都有其适用的场景和优缺点。在实际应用中,选择合适的方法可以提高字符查找的效率和灵活性。
通过上述详尽的介绍和示例代码,相信读者已经掌握了在Python中查找特定字符的多种方法和技巧。希望这些内容能够帮助您在实际编程中更高效地处理字符串查找任务。
相关问答FAQs:
如何在Python字符串中查找特定字符?
在Python中,可以使用多种方法查找特定字符。最常用的方法包括使用find()
、index()
和in
关键字。find()
方法返回字符首次出现的索引,如果字符不存在,则返回-1;而index()
方法在字符不存在时会抛出异常。使用in
关键字则可以直接判断字符是否存在于字符串中。
Python中如何查找字符出现的所有位置?
如果需要找到字符在字符串中出现的所有位置,可以使用循环结合enumerate()
函数。通过遍历字符串,并记录下每次出现该字符时的索引,可以得到所有位置的列表。示例代码如下:
text = "hello world"
char = "o"
positions = [i for i, c in enumerate(text) if c == char]
如何处理查找时的大小写问题?
在查找字符时,大小写可能会影响结果。如果希望忽略大小写,可以将字符串和字符都转换为同一大小写形式。使用lower()
或upper()
方法可以轻松实现。例如:
text = "Hello World"
char = "h"
if char.lower() in text.lower():
print(f"'{char}' exists in the string.")
这种方式确保了查找过程不受大小写的限制,从而提高了搜索的灵活性。
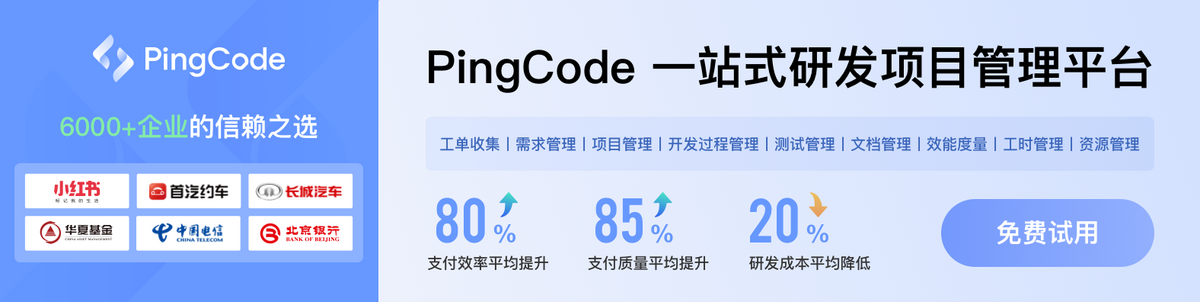