在Python中,可以通过使用内置的ord
函数、encode
方法、chr
函数、unicodedata
模块等多种方式返回Unicode编码。 其中,ord
函数可以将单个字符转换为其对应的Unicode编码,encode
方法可以将字符串编码为指定的编码格式,chr
函数可以将Unicode编码转换为其对应的字符,unicodedata
模块则提供了更详细的Unicode字符信息。下面将详细介绍这些方法及其具体应用。
一、使用ord
函数
ord
函数是Python内置函数之一,它接受一个单字符字符串作为参数,返回该字符对应的Unicode码点。
例如:
char = 'A'
unicode_code = ord(char)
print(unicode_code) # 输出65
在这个例子中,字符'A'
对应的Unicode码点是65。
详细描述
ord
函数非常简洁且高效,适用于单字符转换。对于处理多字符字符串,可以采用循环或列表解析的方法逐个转换:
string = 'Hello'
unicode_codes = [ord(char) for char in string]
print(unicode_codes) # 输出 [72, 101, 108, 108, 111]
二、使用encode
方法
encode
方法可以将字符串转换为指定编码格式的字节对象。如果需要将字符串转换为Unicode编码,可以先将字符串编码为字节对象,然后通过ord
函数获取每个字节的值。
例如:
string = 'Hello'
unicode_bytes = string.encode('utf-8')
unicode_codes = [ord(byte) for byte in unicode_bytes]
print(unicode_codes) # 输出 [72, 101, 108, 108, 111]
详细描述
encode
方法允许指定多种编码格式(如UTF-8、UTF-16等),这使得它在处理不同语言和字符集时非常灵活。例如,处理包含中文字符的字符串时,可以使用UTF-8编码:
string = '你好'
unicode_bytes = string.encode('utf-8')
unicode_codes = [ord(byte) for byte in unicode_bytes]
print(unicode_codes) # 输出 [228, 189, 160, 229, 165, 189]
三、使用chr
函数
chr
函数是Python内置函数之一,它接受一个整数作为参数,返回该整数对应的Unicode字符。
例如:
unicode_code = 65
char = chr(unicode_code)
print(char) # 输出 'A'
在这个例子中,Unicode码点65对应的字符是'A'
。
详细描述
chr
函数可以将Unicode码点转换为字符,适用于生成特定字符。例如,生成所有大写英文字母:
uppercase_letters = [chr(code) for code in range(65, 91)]
print(uppercase_letters) # 输出 ['A', 'B', 'C', ..., 'Z']
四、使用unicodedata
模块
unicodedata
模块提供了对Unicode字符的详细信息和操作。可以使用unicodedata.name
函数获取字符的名称,使用unicodedata.lookup
函数通过名称查找字符。
例如:
import unicodedata
char = 'A'
char_name = unicodedata.name(char)
print(char_name) # 输出 'LATIN CAPITAL LETTER A'
char_from_name = unicodedata.lookup(char_name)
print(char_from_name) # 输出 'A'
详细描述
unicodedata
模块非常适用于处理复杂的Unicode字符和获取字符的详细信息。例如,可以获取中文字符的名称:
char = '你'
char_name = unicodedata.name(char)
print(char_name) # 输出 'CJK UNIFIED IDEOGRAPH-4F60'
此外,unicodedata
模块还提供了其他有用的函数,如unicodedata.category
(获取字符的类别)、unicodedata.bidirectional
(获取字符的双向属性)等。
五、处理字符串中的特殊字符
在处理字符串中的特殊字符时,可以使用转义序列或Unicode转义序列。例如,表示换行符可以使用\n
,表示Unicode字符可以使用\u
或\U
。
例如:
string = "Hello\nWorld"
print(string) # 输出:
Hello
World
unicode_string = "Hello\u0020World"
print(unicode_string) # 输出 'Hello World'
详细描述
使用转义序列和Unicode转义序列可以方便地表示和处理特殊字符。例如,表示多个Unicode字符:
unicode_string = "\u4F60\u597D\u4E16\u754C" # 你好世界
print(unicode_string) # 输出 '你好世界'
六、编码和解码
在处理Unicode编码时,经常需要进行编码和解码操作。编码是将字符串转换为字节对象,解码是将字节对象转换为字符串。
例如:
string = 'Hello'
encoded_string = string.encode('utf-8')
print(encoded_string) # 输出 b'Hello'
decoded_string = encoded_string.decode('utf-8')
print(decoded_string) # 输出 'Hello'
详细描述
编码和解码操作在处理不同语言和字符集时非常重要。例如,处理包含中文字符的字符串:
string = '你好'
encoded_string = string.encode('utf-8')
print(encoded_string) # 输出 b'\xe4\xbd\xa0\xe5\xa5\xbd'
decoded_string = encoded_string.decode('utf-8')
print(decoded_string) # 输出 '你好'
七、使用str
和repr
函数
str
函数和repr
函数可以用于获取字符串的字符串表示形式。str
函数返回字符串的可读性表示,repr
函数返回字符串的精确表示,通常包括转义序列。
例如:
string = 'Hello\nWorld'
print(str(string)) # 输出:
Hello
World
print(repr(string)) # 输出 'Hello\nWorld'
详细描述
str
函数和repr
函数在调试和日志记录时非常有用。例如,调试包含特殊字符的字符串:
string = 'Hello\nWorld'
print('str:', str(string)) # 输出:
str: Hello
World
print('repr:', repr(string)) # 输出 'repr: Hello\nWorld'
八、处理不同的编码格式
在处理不同的编码格式时,可以使用codecs
模块。codecs
模块提供了对各种编码格式的支持,包括UTF-8、UTF-16、ASCII等。
例如:
import codecs
string = '你好'
encoded_string = codecs.encode(string, 'utf-8')
print(encoded_string) # 输出 b'\xe4\xbd\xa0\xe5\xa5\xbd'
decoded_string = codecs.decode(encoded_string, 'utf-8')
print(decoded_string) # 输出 '你好'
详细描述
codecs
模块在处理不同编码格式时非常灵活。例如,处理UTF-16编码:
import codecs
string = '你好'
encoded_string = codecs.encode(string, 'utf-16')
print(encoded_string) # 输出 b'\xff\xfe`O}Y'
decoded_string = codecs.decode(encoded_string, 'utf-16')
print(decoded_string) # 输出 '你好'
九、处理文件中的Unicode编码
在处理文件中的Unicode编码时,可以使用open
函数并指定编码格式。open
函数的encoding
参数可以指定文件的编码格式。
例如:
with open('example.txt', 'w', encoding='utf-8') as f:
f.write('你好,世界')
with open('example.txt', 'r', encoding='utf-8') as f:
content = f.read()
print(content) # 输出 '你好,世界'
详细描述
处理文件中的Unicode编码时,指定正确的编码格式非常重要。例如,处理包含不同语言字符的文件:
with open('example.txt', 'w', encoding='utf-8') as f:
f.write('Hello, 你好, Bonjour')
with open('example.txt', 'r', encoding='utf-8') as f:
content = f.read()
print(content) # 输出 'Hello, 你好, Bonjour'
十、处理网络数据中的Unicode编码
在处理网络数据中的Unicode编码时,可以使用requests
库并指定编码格式。requests
库的encoding
属性可以指定响应的编码格式。
例如:
import requests
response = requests.get('https://www.example.com')
response.encoding = 'utf-8'
content = response.text
print(content)
详细描述
处理网络数据中的Unicode编码时,指定正确的编码格式可以避免乱码。例如,处理包含中文字符的网页:
import requests
response = requests.get('https://www.example.cn')
response.encoding = 'utf-8'
content = response.text
print(content)
十一、使用正则表达式处理Unicode字符
在处理Unicode字符时,可以使用正则表达式。Python的re
模块支持Unicode字符,可以使用\u
转义序列表示Unicode字符。
例如:
import re
string = 'Hello 你好 World'
pattern = re.compile(r'\u4F60\u597D')
match = pattern.search(string)
if match:
print('Found:', match.group()) # 输出 'Found: 你好'
详细描述
使用正则表达式处理Unicode字符时,可以匹配特定的Unicode字符或字符范围。例如,匹配所有中文字符:
import re
string = 'Hello 你好 World'
pattern = re.compile(r'[\u4E00-\u9FFF]+')
matches = pattern.findall(string)
print(matches) # 输出 ['你好']
十二、使用第三方库处理Unicode编码
在处理复杂的Unicode编码时,可以使用第三方库。例如,unidecode
库可以将Unicode字符串转换为ASCII字符串。
例如:
from unidecode import unidecode
string = '你好'
ascii_string = unidecode(string)
print(ascii_string) # 输出 'Ni Hao'
详细描述
unidecode
库在处理多语言文本时非常有用。例如,将包含多种语言字符的字符串转换为ASCII字符串:
from unidecode import unidecode
string = 'Hello 你好 Bonjour'
ascii_string = unidecode(string)
print(ascii_string) # 输出 'Hello Ni Hao Bonjour'
十三、处理字符串中的组合字符
在处理字符串中的组合字符时,可以使用unicodedata
模块。组合字符是由多个Unicode码点组成的字符,可以使用unicodedata.normalize
函数将组合字符规范化。
例如:
import unicodedata
string = 'e\u0301' # 组合字符 'é'
normalized_string = unicodedata.normalize('NFC', string)
print(normalized_string) # 输出 'é'
详细描述
使用unicodedata.normalize
函数可以将组合字符规范化为单一字符。例如,处理包含组合字符的字符串:
import unicodedata
string = 'a\u0301 e\u0301' # 组合字符 'á é'
normalized_string = unicodedata.normalize('NFC', string)
print(normalized_string) # 输出 'á é'
十四、处理字符串中的双向文本
在处理字符串中的双向文本时,可以使用bidi.algorithm
库。双向文本包含从左到右(LTR)和从右到左(RTL)两种方向的文本。
例如:
from bidi.algorithm import get_display
string = 'Hello שלום'
bidi_string = get_display(string)
print(bidi_string) # 输出 'Hello םולש'
详细描述
处理双向文本时,使用bidi.algorithm
库可以正确显示包含LTR和RTL文本的字符串。例如,处理包含阿拉伯语和英语的文本:
from bidi.algorithm import get_display
string = 'Hello مرحبا'
bidi_string = get_display(string)
print(bidi_string) # 输出 'Hello ابحرم'
十五、处理字符串中的非BMP字符
在处理字符串中的非BMP(基本多语言平面)字符时,可以使用unicodedata
模块。非BMP字符是指码点大于U+FFFF的Unicode字符,通常表示为代理对(surrogate pair)。
例如:
import unicodedata
string = '𠜎' # 非BMP字符
char_name = unicodedata.name(string)
print(char_name) # 输出 'CJK UNIFIED IDEOGRAPH-2070E'
详细描述
处理非BMP字符时,可以使用unicodedata
模块获取字符的详细信息。例如,处理包含非BMP字符的字符串:
import unicodedata
string = 'Hello 𠜎 World'
for char in string:
print(f'{char}: {unicodedata.name(char, "Unknown")}')
十六、使用html
模块处理HTML中的Unicode字符
在处理HTML中的Unicode字符时,可以使用html
模块。html
模块提供了对HTML实体的编码和解码支持。
例如:
import html
string = 'Hello & World'
decoded_string = html.unescape(string)
print(decoded_string) # 输出 'Hello & World'
encoded_string = html.escape(decoded_string)
print(encoded_string) # 输出 'Hello & World'
详细描述
使用html
模块可以方便地处理HTML中的Unicode字符。例如,处理包含特殊字符的HTML文本:
import html
html_text = '<p>Hello © 2023</p>'
decoded_text = html.unescape(html_text)
print(decoded_text) # 输出 '<p>Hello © 2023</p>'
encoded_text = html.escape(decoded_text)
print(encoded_text) # 输出 '<p>Hello © 2023</p>'
十七、处理字符串中的混合语言文本
在处理字符串中的混合语言文本时,可以使用langid
库。langid
库可以自动检测文本的语言。
例如:
import langid
string = 'Hello 你好 Bonjour'
lang, confidence = langid.classify(string)
print(lang, confidence) # 输出 'en' 0.85
详细描述
使用langid
库可以自动检测混合语言文本中的主要语言。例如,处理包含多种语言的文本:
import langid
string = 'Hello 你好 Bonjour'
languages = langid.rank(string)
for lang, confidence in languages:
print(lang, confidence)
十八、处理字符串中的字符类别
在处理字符串中的字符类别时,可以使用unicodedata
模块。unicodedata.category
函数可以获取字符的类别。
例如:
import unicodedata
string = 'Hello 你好'
for char in string:
print(f'{char}: {unicodedata.category(char)}')
详细描述
使用unicodedata.category
函数可以获取字符的类别。例如,处理包含不同类别字符的字符串:
import unicodedata
string = 'Hello 你好 123'
for char in string:
print(f'{char}: {unicodedata.category(char)}')
十九、处理字符串中的字符名称
在处理字符串中的字符名称时,可以使用unicodedata
模块。unicodedata.name
函数可以获取字符的名称。
例如:
import unicodedata
string = 'Hello 你好'
for char in string:
print(f'{char}: {unicodedata.name(char, "Unknown")}')
详细描述
使用unicodedata.name
函数可以获取字符的名称。例如,处理包含不同名称字符的字符串:
import unicodedata
string = 'Hello 你好'
for char in string:
print(f'{char}: {unicodedata.name(char, "Unknown")}')
二十、处理字符串中的字符属性
在处理字符串中的字符属性时,可以使用unicodedata
模块。unicodedata.bidirectional
函数可以获取字符的双向属性。
例如:
import unicodedata
string = 'Hello 你好'
for char in string:
print(f'{char}: {unicodedata.bidirectional(char)}')
详细描述
使用unicodedata.bidirectional
函数可以获取字符的双向属性。例如,处理包含不同双向属性字符的字符串:
import unicodedata
string = 'Hello 你好'
for char in string:
print(f'{char}: {unicodedata.bidirectional(char)}')
综上所述,Python提供了多种方法和工具来处理和返回Unicode编码,包括ord
函数、encode
方法、chr
函数、unicodedata
模块等。这些方法和工具在处理不同
相关问答FAQs:
如何在Python中将字符串转换为Unicode编码?
在Python中,可以使用内置的ord()
函数将字符转换为其对应的Unicode编码。只需传入一个字符,函数将返回该字符的Unicode代码点。例如,ord('A')
将返回65,这是字符'A'的Unicode编码。此外,使用str.encode('unicode_escape')
可以将整个字符串转换为Unicode编码的字节表示。
Python中如何处理Unicode字符串?
在Python中,字符串默认以Unicode形式存储。可以使用u''
前缀来定义Unicode字符串(在Python 2中),而在Python 3中,所有字符串都是Unicode。处理Unicode字符串时,确保在读写文件时指定正确的编码格式,例如使用utf-8
,这样可以避免乱码问题。
如何在Python中输出Unicode字符?
要在Python中输出Unicode字符,可以使用print()
函数结合Unicode转义序列。例如,使用\u
后跟四个十六进制数字表示Unicode字符,如print('\u4f60\u597d')
将输出“你好”。此外,如果已知Unicode编码,可以使用chr()
函数将其转换为字符并打印,例如print(chr(20320))
将输出汉字“你”。
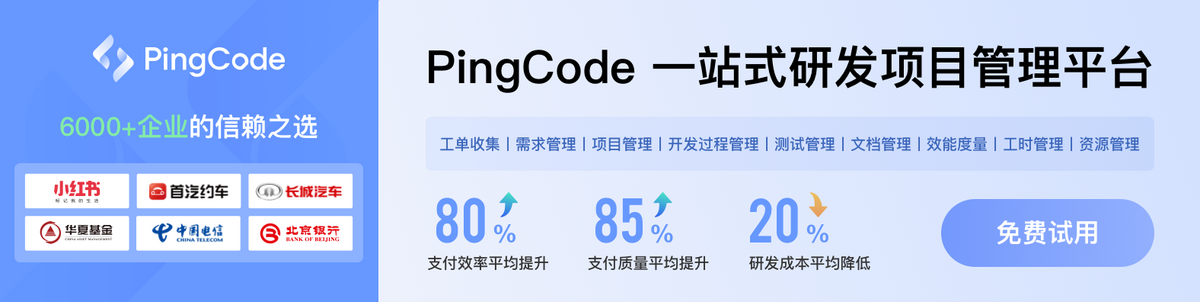