在Python中查找单词的个数可以通过多种方法实现,包括使用字符串方法、使用正则表达式、使用集合计数等。其中,使用字符串方法是一种简单而常见的方法,接下来我们将详细描述这种方法。
使用字符串方法查找单词的个数可以通过以下步骤实现:首先,读取文本内容,然后使用字符串的split()
方法将文本分割成单词列表,最后统计列表中单词的数量。具体步骤如下:
def count_words(text):
words = text.split() # 使用split方法将文本分割成单词列表
return len(words) # 返回单词列表的长度,即单词的数量
示例文本
text = "Python is a powerful programming language."
word_count = count_words(text)
print(f"The number of words in the text is: {word_count}")
上述代码中,我们定义了一个函数count_words
,它接收一个字符串参数text
,并返回该字符串中单词的数量。split()
方法默认会以空格为分隔符,将字符串分割成单词列表。
接下来,我们将进一步探讨其他方法以及更详细的应用场景。
一、字符串方法
1、基本使用
如前所述,使用字符串的split()
方法可以简单地将文本分割成单词列表。split()
方法默认以空格为分隔符,但它也可以接受其他分隔符作为参数。
text = "Python is a powerful programming language."
words = text.split()
word_count = len(words)
print(f"The number of words in the text is: {word_count}")
2、处理标点符号
在实际应用中,文本中可能包含标点符号,这些标点符号会影响单词的统计。可以使用Python的string
模块来处理标点符号。
import string
def count_words(text):
# 去除标点符号
text = text.translate(str.maketrans('', '', string.punctuation))
words = text.split()
return len(words)
text = "Hello, world! Python is amazing."
word_count = count_words(text)
print(f"The number of words in the text is: {word_count}")
二、正则表达式
1、基本使用
正则表达式是一种强大的文本处理工具,可以用来匹配单词,并统计单词的数量。Python的re
模块提供了支持正则表达式的功能。
import re
def count_words(text):
words = re.findall(r'\b\w+\b', text)
return len(words)
text = "Hello, world! Python is amazing."
word_count = count_words(text)
print(f"The number of words in the text is: {word_count}")
2、处理复杂文本
正则表达式可以处理更复杂的文本情况,例如处理带有连字符的单词、处理缩写等。
import re
def count_words(text):
# 匹配单词,包括带连字符的单词和缩写
words = re.findall(r'\b\w[\w-]*\b', text)
return len(words)
text = "Hello, world! Python-based tools are user-friendly. It's amazing."
word_count = count_words(text)
print(f"The number of words in the text is: {word_count}")
三、集合计数
1、使用collections.Counter
collections
模块中的Counter
类提供了一种简单的方法来统计单词的出现次数。
from collections import Counter
def count_words(text):
words = text.split()
word_count = Counter(words)
return word_count
text = "Python is powerful. Python is easy to learn."
word_count = count_words(text)
print(word_count)
2、统计词频
除了统计单词的数量,有时还需要统计每个单词的出现频率。Counter
类可以轻松实现这一点。
from collections import Counter
def count_word_frequencies(text):
words = text.split()
word_frequencies = Counter(words)
return word_frequencies
text = "Python is powerful. Python is easy to learn. Learning Python is fun."
word_frequencies = count_word_frequencies(text)
print(word_frequencies)
四、文件处理
1、读取文件内容
在实际应用中,单词统计通常应用于文件处理,例如统计一个文本文件中的单词数量。可以使用Python的文件操作功能读取文件内容,并对其进行单词统计。
def count_words_in_file(file_path):
with open(file_path, 'r', encoding='utf-8') as file:
text = file.read()
word_count = count_words(text)
return word_count
file_path = 'sample.txt'
word_count = count_words_in_file(file_path)
print(f"The number of words in the file is: {word_count}")
2、处理大文件
对于大文件,可以逐行读取文件内容,以减少内存消耗。每读取一行,统计其中的单词数量,最后累加得到总的单词数量。
def count_words_in_large_file(file_path):
total_word_count = 0
with open(file_path, 'r', encoding='utf-8') as file:
for line in file:
total_word_count += count_words(line)
return total_word_count
file_path = 'large_sample.txt'
total_word_count = count_words_in_large_file(file_path)
print(f"The total number of words in the large file is: {total_word_count}")
五、文本预处理
1、去除停用词
在自然语言处理(NLP)任务中,停用词(如“the”、“is”、“in”等)通常会被去除,以提高处理效率和准确性。可以使用NLTK库来去除停用词。
import nltk
from nltk.corpus import stopwords
nltk.download('stopwords')
def count_words_without_stopwords(text):
stop_words = set(stopwords.words('english'))
words = text.split()
filtered_words = [word for word in words if word.lower() not in stop_words]
return len(filtered_words)
text = "Python is powerful and easy to learn."
word_count = count_words_without_stopwords(text)
print(f"The number of words without stopwords is: {word_count}")
2、词形还原
词形还原(Lemmatization)是将词语还原到其基本形式的过程。在统计单词时,词形还原可以帮助统一词语的不同形式。
import nltk
from nltk.stem import WordNetLemmatizer
nltk.download('wordnet')
def count_lemmatized_words(text):
lemmatizer = WordNetLemmatizer()
words = text.split()
lemmatized_words = [lemmatizer.lemmatize(word) for word in words]
return len(lemmatized_words)
text = "The foxes are running quickly."
word_count = count_lemmatized_words(text)
print(f"The number of lemmatized words is: {word_count}")
六、应用场景
1、文本分析
单词统计是文本分析中的基本任务之一。在文本分析中,我们可以通过统计单词的数量、频率等信息,来了解文本的主题、情感等。
def analyze_text(text):
word_count = count_words(text)
word_frequencies = count_word_frequencies(text)
print(f"Total number of words: {word_count}")
print("Word Frequencies:")
for word, frequency in word_frequencies.items():
print(f"{word}: {frequency}")
text = "Python is powerful. Python is easy to learn. Learning Python is fun."
analyze_text(text)
2、搜索引擎优化(SEO)
在SEO中,统计网页中的关键词出现频率,可以帮助优化网页内容,提高搜索引擎排名。
def analyze_keywords(text, keywords):
word_frequencies = count_word_frequencies(text)
keyword_frequencies = {keyword: word_frequencies[keyword] for keyword in keywords}
return keyword_frequencies
text = "Python is a powerful programming language. Python is popular for web development."
keywords = ["Python", "programming", "web"]
keyword_frequencies = analyze_keywords(text, keywords)
print("Keyword Frequencies:")
for keyword, frequency in keyword_frequencies.items():
print(f"{keyword}: {frequency}")
3、教育评估
在教育领域,单词统计可以用于评估学生的作文、阅读材料等。例如,可以统计学生作文中的单词数量、词汇丰富度等指标,以评估学生的语言能力。
def evaluate_essay(essay):
word_count = count_words(essay)
unique_words = len(set(essay.split()))
print(f"Total number of words: {word_count}")
print(f"Number of unique words: {unique_words}")
essay = "Learning Python is fun. Python is a powerful programming language."
evaluate_essay(essay)
七、扩展应用
1、词云生成
词云(Word Cloud)是一种可视化技术,用于显示文本数据中词语的重要性。词频越高的词在词云中显示得越大。可以使用wordcloud
库生成词云。
from wordcloud import WordCloud
import matplotlib.pyplot as plt
def generate_wordcloud(text):
wordcloud = WordCloud(width=800, height=400, background_color='white').generate(text)
plt.figure(figsize=(10, 5))
plt.imshow(wordcloud, interpolation='bilinear')
plt.axis('off')
plt.show()
text = "Python is powerful. Python is easy to learn. Learning Python is fun."
generate_wordcloud(text)
2、情感分析
在情感分析中,单词统计可以帮助识别文本中的情感倾向。可以使用TextBlob
库进行情感分析。
from textblob import TextBlob
def analyze_sentiment(text):
blob = TextBlob(text)
sentiment = blob.sentiment
print(f"Polarity: {sentiment.polarity}, Subjectivity: {sentiment.subjectivity}")
text = "Python is powerful. Python is easy to learn. I love learning Python."
analyze_sentiment(text)
八、总结
在Python中查找单词的个数有多种方法,包括使用字符串方法、正则表达式、集合计数等。每种方法都有其适用场景和优缺点。在实际应用中,选择合适的方法可以提高效率和准确性。此外,单词统计还可以应用于文本分析、SEO、教育评估等多个领域,具有广泛的应用前景。通过结合词云生成、情感分析等技术,可以进一步扩展单词统计的应用范围,为数据分析和自然语言处理提供有力支持。
相关问答FAQs:
如何使用Python读取文本文件并统计单词个数?
要在Python中读取文本文件并统计单词个数,可以使用内置的文件操作和字符串处理功能。可以通过以下步骤实现:打开文件,读取内容,使用字符串的split()方法将文本分割成单词,最后使用len()函数统计单词的数量。以下是一个简单的示例代码:
with open('yourfile.txt', 'r') as file:
text = file.read()
words = text.split()
word_count = len(words)
print(f'The number of words is: {word_count}')
在Python中如何统计字符串中某个特定单词的出现次数?
如果需要计算某个特定单词在字符串中的出现次数,可以使用字符串的count()方法。这一方法非常简单且高效。以下是一个示例:
text = "Python is great. Python is easy to learn."
word_to_count = "Python"
count = text.count(word_to_count)
print(f'The word "{word_to_count}" appears {count} times.')
有哪些第三方库可以帮助更高效地统计单词个数?
在Python中,除了基本的字符串操作,还可以利用一些第三方库来更方便地统计单词个数。例如,使用collections模块中的Counter类可以快速计算字符串中各个单词的出现次数。以下是如何使用Counter的示例:
from collections import Counter
text = "Python is great. Python is easy to learn."
words = text.split()
word_count = Counter(words)
print(word_count)
通过这些方法,用户可以轻松实现单词计数的需求。
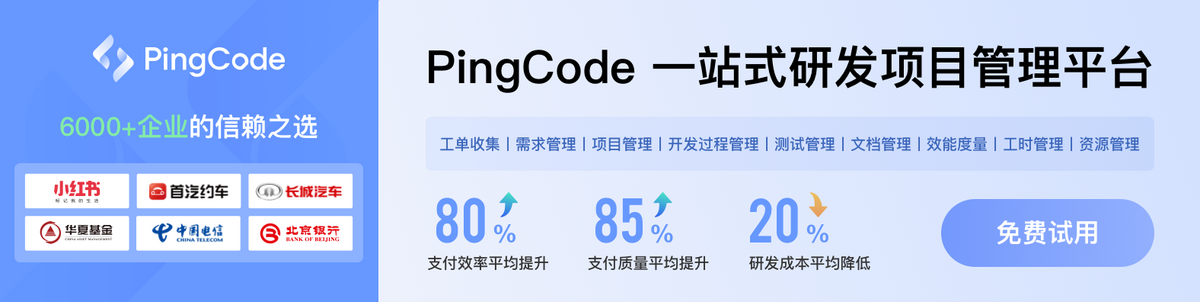