使用Python发送HTTP请求的步骤如下:导入合适的库、构建请求、发送请求、处理响应。其中,导入合适的库是最为重要的步骤之一,Python中最常用的库是requests
库。以下是详细描述如何使用requests
库发送HTTP请求的步骤。
导入合适的库
Python中发送HTTP请求最常用的库是requests
。它是一个简单易用且功能强大的HTTP库。在开始使用之前,首先需要确保已经安装了requests
库。可以使用以下命令来安装它:
pip install requests
安装完成后,可以在脚本中导入requests
库:
import requests
这样就可以使用requests
库来发送HTTP请求了。
一、构建请求
构建HTTP请求是发送请求的第一步。HTTP请求的构建包括指定请求方法、URL和请求头等信息。
1、指定请求方法和URL
在requests
库中,可以使用不同的方法来发送不同类型的HTTP请求,例如GET、POST、PUT、DELETE等。以下是一些示例:
- 发送GET请求:
response = requests.get('https://api.example.com/data')
- 发送POST请求:
response = requests.post('https://api.example.com/data', data={'key': 'value'})
2、设置请求头
有时候需要在HTTP请求中设置一些请求头信息,例如用户代理、内容类型等。可以使用headers
参数来设置请求头:
headers = {
'User-Agent': 'my-app/0.0.1',
'Content-Type': 'application/json'
}
response = requests.get('https://api.example.com/data', headers=headers)
3、发送请求数据
对于POST请求,可以使用data
参数来发送表单数据,或者使用json
参数来发送JSON数据:
# 发送表单数据
response = requests.post('https://api.example.com/data', data={'key': 'value'})
发送JSON数据
response = requests.post('https://api.example.com/data', json={'key': 'value'})
二、发送请求
构建好HTTP请求后,就可以发送请求了。requests
库中提供了多种方法来发送不同类型的请求,包括GET、POST、PUT、DELETE等。以下是一些示例:
1、发送GET请求
GET请求用于从服务器获取数据。例如:
response = requests.get('https://api.example.com/data')
2、发送POST请求
POST请求用于向服务器发送数据。例如:
response = requests.post('https://api.example.com/data', data={'key': 'value'})
3、发送PUT请求
PUT请求用于更新服务器上的数据。例如:
response = requests.put('https://api.example.com/data/1', data={'key': 'new_value'})
4、发送DELETE请求
DELETE请求用于删除服务器上的数据。例如:
response = requests.delete('https://api.example.com/data/1')
三、处理响应
发送HTTP请求后,服务器会返回一个响应。需要对响应进行处理,以获取所需的数据或信息。
1、检查响应状态码
响应状态码用于表示请求是否成功。可以使用response.status_code
来获取响应状态码。例如:
if response.status_code == 200:
print('请求成功')
else:
print('请求失败')
2、获取响应内容
可以使用response.text
或response.json()
来获取响应内容。例如:
# 获取响应文本内容
print(response.text)
获取响应JSON内容
data = response.json()
print(data)
3、处理响应头
可以使用response.headers
来获取响应头信息。例如:
print(response.headers)
四、处理常见问题
在发送HTTP请求时,可能会遇到一些常见问题,例如请求超时、连接错误等。需要对这些问题进行处理,以确保程序的稳定性和可靠性。
1、处理请求超时
可以使用timeout
参数来设置请求的超时时间。例如:
try:
response = requests.get('https://api.example.com/data', timeout=5)
print(response.text)
except requests.Timeout:
print('请求超时')
2、处理连接错误
可以使用requests.ConnectionError
来捕获连接错误。例如:
try:
response = requests.get('https://api.example.com/data')
print(response.text)
except requests.ConnectionError:
print('连接错误')
3、处理HTTP错误
可以使用response.raise_for_status()
来捕获HTTP错误。例如:
try:
response = requests.get('https://api.example.com/data')
response.raise_for_status()
print(response.text)
except requests.HTTPError as err:
print(f'HTTP错误: {err}')
五、实际应用示例
以下是一个实际应用示例,展示如何使用requests
库发送HTTP请求并处理响应。
import requests
构建请求
url = 'https://api.example.com/data'
headers = {
'User-Agent': 'my-app/0.0.1',
'Content-Type': 'application/json'
}
data = {'key': 'value'}
try:
# 发送POST请求
response = requests.post(url, headers=headers, json=data, timeout=10)
# 检查响应状态码
response.raise_for_status()
# 获取响应内容
response_data = response.json()
print('请求成功:', response_data)
except requests.HTTPError as err:
print(f'HTTP错误: {err}')
except requests.ConnectionError:
print('连接错误')
except requests.Timeout:
print('请求超时')
except requests.RequestException as err:
print(f'请求异常: {err}')
在这个示例中,我们使用requests.post
方法发送一个POST请求,并设置请求头和请求数据。然后,我们检查响应状态码,并获取响应的JSON内容。如果出现错误,我们会捕获并处理各种类型的异常。
六、总结
通过使用Python的requests
库,可以轻松地发送各种类型的HTTP请求,包括GET、POST、PUT、DELETE等。我们可以设置请求头、发送请求数据、处理响应以及处理常见问题。通过这些步骤,可以构建一个稳定可靠的HTTP请求程序,满足各种应用需求。
相关问答FAQs:
如何在Python中发送GET请求?
要在Python中发送GET请求,可以使用requests
库。首先,确保安装了该库,可以通过pip install requests
进行安装。接着,使用以下代码发送GET请求:
import requests
response = requests.get('https://api.example.com/data')
print(response.status_code)
print(response.json())
这段代码会获取指定URL的数据,并打印响应状态码和JSON格式的返回内容。
Python中如何发送POST请求,并附带数据?
发送POST请求同样可以使用requests
库。使用requests.post()
方法,可以轻松发送包含数据的POST请求。例如:
import requests
data = {'key1': 'value1', 'key2': 'value2'}
response = requests.post('https://api.example.com/submit', data=data)
print(response.status_code)
print(response.json())
这段代码将数据以表单格式发送到指定的URL,并打印响应状态码和返回的JSON数据。
如何处理HTTP请求中的异常和错误?
在发送HTTP请求时,处理异常和错误是非常重要的。可以使用try-except
块捕获可能发生的异常,例如网络错误或请求超时。以下是一个示例:
import requests
try:
response = requests.get('https://api.example.com/data', timeout=5)
response.raise_for_status() # 如果响应状态码不是200,会抛出HTTPError
print(response.json())
except requests.exceptions.HTTPError as err:
print(f'HTTP error occurred: {err}')
except requests.exceptions.RequestException as e:
print(f'Error occurred: {e}')
通过这种方式,可以有效地处理请求中可能遇到的各种问题,确保程序的稳定性。
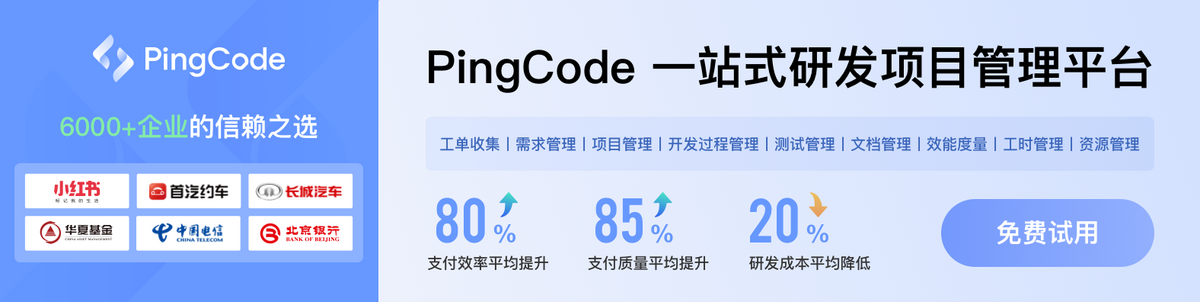