要在Python页面中实现便签功能,可以使用Flask框架、JavaScript交互、SQLite数据库。其中,Flask框架是Python的一个轻量级Web框架,适合构建简单的Web应用。JavaScript交互用于实现页面动态效果,提升用户体验。SQLite数据库则负责存储便签数据,确保数据的持久化。在下面的详细描述中,我将重点解释如何使用Flask框架来构建Web应用的后端部分。
一、搭建Flask环境
1、安装Flask
首先,确保你的计算机上安装了Python。然后,通过pip安装Flask:
pip install Flask
2、创建项目结构
创建一个新的文件夹作为你的项目目录。目录结构如下:
my_notebook_app/
|-- app.py
|-- templates/
| |-- index.html
|-- static/
| |-- style.css
| |-- script.js
|-- database.db
二、编写Flask后端
1、初始化Flask应用
在app.py
文件中编写如下代码:
from flask import Flask, render_template, request, redirect, url_for, jsonify
import sqlite3
app = Flask(__name__)
数据库初始化
def init_db():
conn = sqlite3.connect('database.db')
c = conn.cursor()
c.execute('''CREATE TABLE IF NOT EXISTS notes
(id INTEGER PRIMARY KEY, content TEXT)''')
conn.commit()
conn.close()
init_db()
2、路由与视图函数
为便签应用创建视图函数:
@app.route('/')
def index():
conn = sqlite3.connect('database.db')
c = conn.cursor()
c.execute('SELECT * FROM notes')
notes = c.fetchall()
conn.close()
return render_template('index.html', notes=notes)
@app.route('/add_note', methods=['POST'])
def add_note():
content = request.form['content']
conn = sqlite3.connect('database.db')
c = conn.cursor()
c.execute('INSERT INTO notes (content) VALUES (?)', (content,))
conn.commit()
conn.close()
return redirect(url_for('index'))
@app.route('/delete_note/<int:note_id>', methods=['POST'])
def delete_note(note_id):
conn = sqlite3.connect('database.db')
c = conn.cursor()
c.execute('DELETE FROM notes WHERE id=?', (note_id,))
conn.commit()
conn.close()
return redirect(url_for('index'))
if __name__ == '__main__':
app.run(debug=True)
三、编写前端页面
1、创建模板文件
在templates
文件夹中创建index.html
:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>便签应用</title>
<link rel="stylesheet" href="{{ url_for('static', filename='style.css') }}">
</head>
<body>
<h1>我的便签</h1>
<form action="{{ url_for('add_note') }}" method="POST">
<textarea name="content" rows="4" cols="50"></textarea>
<button type="submit">添加便签</button>
</form>
<ul>
{% for note in notes %}
<li>
{{ note[1] }}
<form action="{{ url_for('delete_note', note_id=note[0]) }}" method="POST" style="display:inline;">
<button type="submit">删除</button>
</form>
</li>
{% endfor %}
</ul>
<script src="{{ url_for('static', filename='script.js') }}"></script>
</body>
</html>
2、添加样式
在static
文件夹中创建style.css
,添加一些基本样式:
body {
font-family: Arial, sans-serif;
}
h1 {
text-align: center;
}
form {
margin: 20px;
text-align: center;
}
ul {
list-style-type: none;
}
li {
margin: 10px 0;
text-align: center;
}
3、添加JavaScript(可选)
如果需要一些动态效果,可以在static
文件夹中创建script.js
,并编写相关JavaScript代码:
// 示例:简单的客户端交互
document.addEventListener('DOMContentLoaded', function() {
console.log('页面加载完成');
});
四、运行应用
在项目目录下运行Flask应用:
python app.py
打开浏览器,访问http://127.0.0.1:5000/
,你应该能看到一个简单的便签应用,能够添加和删除便签。
五、扩展功能
1、编辑便签
添加编辑便签的功能:
@app.route('/edit_note/<int:note_id>', methods=['GET', 'POST'])
def edit_note(note_id):
if request.method == 'POST':
new_content = request.form['content']
conn = sqlite3.connect('database.db')
c = conn.cursor()
c.execute('UPDATE notes SET content=? WHERE id=?', (new_content, note_id))
conn.commit()
conn.close()
return redirect(url_for('index'))
else:
conn = sqlite3.connect('database.db')
c = conn.cursor()
c.execute('SELECT content FROM notes WHERE id=?', (note_id,))
note = c.fetchone()
conn.close()
return render_template('edit.html', note=note, note_id=note_id)
创建edit.html
模板:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>编辑便签</title>
<link rel="stylesheet" href="{{ url_for('static', filename='style.css') }}">
</head>
<body>
<h1>编辑便签</h1>
<form action="{{ url_for('edit_note', note_id=note_id) }}" method="POST">
<textarea name="content" rows="4" cols="50">{{ note[0] }}</textarea>
<button type="submit">保存更改</button>
</form>
</body>
</html>
在index.html
中为每个便签添加编辑按钮:
<li>
{{ note[1] }}
<form action="{{ url_for('delete_note', note_id=note[0]) }}" method="POST" style="display:inline;">
<button type="submit">删除</button>
</form>
<a href="{{ url_for('edit_note', note_id=note[0]) }}">编辑</a>
</li>
2、增加用户身份验证
为了让便签应用支持用户身份验证,可以使用Flask-Login扩展。在app.py
中进行如下修改:
from flask import Flask, render_template, request, redirect, url_for, jsonify, session
from flask_login import LoginManager, UserMixin, login_user, login_required, logout_user, current_user
import sqlite3
app = Flask(__name__)
app.secret_key = 'your_secret_key'
login_manager = LoginManager()
login_manager.init_app(app)
login_manager.login_view = 'login'
class User(UserMixin):
def __init__(self, id):
self.id = id
@login_manager.user_loader
def load_user(user_id):
return User(user_id)
@app.route('/login', methods=['GET', 'POST'])
def login():
if request.method == 'POST':
username = request.form['username']
password = request.form['password']
# 验证用户名和密码
if username == 'admin' and password == 'password':
user = User(id=1)
login_user(user)
return redirect(url_for('index'))
return render_template('login.html')
@app.route('/logout')
@login_required
def logout():
logout_user()
return redirect(url_for('login'))
@app.route('/')
@login_required
def index():
conn = sqlite3.connect('database.db')
c = conn.cursor()
c.execute('SELECT * FROM notes')
notes = c.fetchall()
conn.close()
return render_template('index.html', notes=notes)
其他路由保持不变
if __name__ == '__main__':
app.run(debug=True)
创建login.html
模板:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>登录</title>
<link rel="stylesheet" href="{{ url_for('static', filename='style.css') }}">
</head>
<body>
<h1>登录</h1>
<form action="{{ url_for('login') }}" method="POST">
<label for="username">用户名:</label>
<input type="text" id="username" name="username"><br>
<label for="password">密码:</label>
<input type="password" id="password" name="password"><br>
<button type="submit">登录</button>
</form>
</body>
</html>
3、添加搜索功能
在app.py
中添加搜索功能:
@app.route('/search', methods=['GET'])
@login_required
def search():
query = request.args.get('query')
conn = sqlite3.connect('database.db')
c = conn.cursor()
c.execute("SELECT * FROM notes WHERE content LIKE ?", ('%' + query + '%',))
notes = c.fetchall()
conn.close()
return render_template('index.html', notes=notes)
在index.html
中添加搜索表单:
<form action="{{ url_for('search') }}" method="GET">
<input type="text" name="query" placeholder="搜索便签内容">
<button type="submit">搜索</button>
</form>
六、总结
通过以上步骤,我们使用Flask框架创建了一个简单的便签应用,并实现了添加、删除、编辑便签的功能。同时,集成了用户身份验证和搜索功能。Flask框架的灵活性和简洁性使得它非常适合快速构建Web应用。对于更复杂的功能,可以进一步使用Flask的扩展或结合其他前端框架,如Vue.js或React,来提升应用的用户体验和交互性。
在实际开发中,可能还需要考虑一些其他因素,例如表单验证、安全性、性能优化等。这些都可以通过进一步学习和实践来逐步掌握。希望这篇文章对你如何在Python页面中实现便签功能有所帮助。
相关问答FAQs:
如何在Python页面中创建便签应用?
要在Python页面中创建便签应用,可以使用Tkinter库,这是Python的标准GUI库。您可以创建一个简单的窗口,添加文本框来输入便签内容,并利用按钮保存和删除便签。使用SQLite数据库存储便签数据也可以让您的便签应用更具实用性。
便签应用支持哪些功能?
理想的便签应用应支持多种功能,包括添加、编辑和删除便签、查看历史便签、设置提醒、分类便签以及搜索功能。您也可以考虑实现便签的颜色标记功能,以便更好地组织和区分不同主题的便签。
我可以在哪里找到Python便签应用的示例代码?
网络上有很多资源可以找到Python便签应用的示例代码,包括GitHub、Stack Overflow和Python官方文档。搜索相关的开源项目不仅可以帮助您了解不同的实现方式,还能为您提供灵感和代码片段,帮助您快速搭建自己的便签应用。
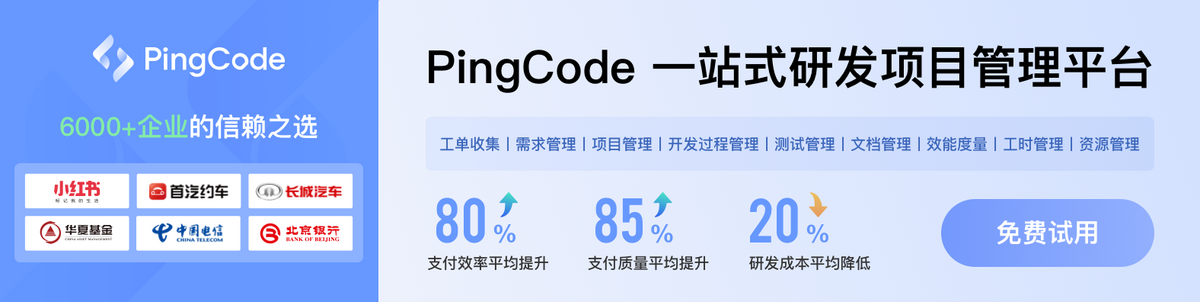