Python项目查找HTML页面的方法包括使用BeautifulSoup解析HTML、利用requests库获取页面内容、使用正则表达式进行文本搜索、通过Selenium进行网页自动化等。这些方法各有优劣,具体选择取决于项目需求和复杂程度。
其中,使用BeautifulSoup解析HTML页面是最常用的方法之一。BeautifulSoup是一个Python库,可以从HTML和XML文件中提取数据。它通过Python的标准库中的html.parser解析HTML文档,并提供了一些简单的方法来导航、搜索和修改解析树。以下是详细介绍这一方法的内容:
BeautifulSoup解析HTML页面的详细步骤:
- 安装BeautifulSoup和requests库:
pip install beautifulsoup4 requests
- 使用requests库获取HTML页面内容:
import requests
from bs4 import BeautifulSoup
url = 'http://example.com'
response = requests.get(url)
if response.status_code == 200:
html_content = response.text
else:
print("Failed to retrieve the webpage.")
- 解析HTML内容:
soup = BeautifulSoup(html_content, 'html.parser')
- 查找所需元素:
BeautifulSoup提供了多种方法来查找和搜索HTML元素,例如
find()
,find_all()
,select()
等。title = soup.find('title').text
print(f"Page Title: {title}")
all_links = soup.find_all('a')
for link in all_links:
print(link.get('href'))
一、BEAUTIFULSOUP解析HTML页面
BeautifulSoup是一个非常强大的工具,用于解析和提取HTML或XML文件中的数据。它能够让你轻松地进行HTML文档的导航、搜索和修改。以下是一些关于BeautifulSoup的具体内容:
1. 安装和基础使用
首先,安装BeautifulSoup和requests库。requests库用于获取网页的HTML内容,而BeautifulSoup用于解析这些内容。
pip install beautifulsoup4 requests
然后,使用requests库获取网页的HTML内容:
import requests
from bs4 import BeautifulSoup
url = 'http://example.com'
response = requests.get(url)
html_content = response.text
接着,将HTML内容传递给BeautifulSoup进行解析:
soup = BeautifulSoup(html_content, 'html.parser')
2. 查找和提取数据
BeautifulSoup提供了许多方法来查找和提取数据。常用的方法包括find()
, find_all()
, select()
等。
例如,查找网页的标题:
title = soup.find('title').text
print(f"Page Title: {title}")
查找所有的链接:
all_links = soup.find_all('a')
for link in all_links:
print(link.get('href'))
3. 处理复杂的HTML结构
BeautifulSoup还可以处理复杂的HTML结构。例如,查找特定的class或id的元素:
special_div = soup.find('div', class_='special-class')
print(special_div.text)
specific_id = soup.find(id='specific-id')
print(specific_id.text)
通过CSS选择器查找元素:
elements = soup.select('div.special-class > p')
for element in elements:
print(element.text)
二、使用REQUESTS库获取HTML页面内容
requests库是Python中一个简单而强大的HTTP库,用于发送所有HTTP请求。它是获取网页HTML内容的基础工具。
1. 安装和基础使用
首先,安装requests库:
pip install requests
然后,使用requests库发送一个HTTP GET请求以获取网页内容:
import requests
url = 'http://example.com'
response = requests.get(url)
if response.status_code == 200:
html_content = response.text
else:
print("Failed to retrieve the webpage.")
2. 处理请求和响应
requests库可以处理各种HTTP请求和响应,包括GET、POST、PUT、DELETE等。你可以传递参数、头信息、数据等来定制请求。
例如,发送一个带参数的GET请求:
params = {'key1': 'value1', 'key2': 'value2'}
response = requests.get(url, params=params)
发送一个POST请求:
data = {'key1': 'value1', 'key2': 'value2'}
response = requests.post(url, data=data)
处理响应头信息和内容:
print(response.headers)
print(response.content)
三、使用正则表达式进行文本搜索
正则表达式(Regular Expressions, regex)是一种强大的模式匹配工具,可以用于搜索和操作字符串。它在处理文本数据时非常有用,包括HTML内容。
1. 安装和基础使用
Python内置了re库用于处理正则表达式。你可以直接使用它来进行文本搜索和操作。
import re
2. 基本的正则表达式操作
正则表达式的基本操作包括匹配、搜索、替换等。以下是一些常用的操作:
匹配模式:
pattern = r'\d+'
text = 'There are 123 apples.'
match = re.match(pattern, text)
if match:
print(f"Matched: {match.group()}")
else:
print("No match found.")
搜索模式:
search = re.search(pattern, text)
if search:
print(f"Found: {search.group()}")
else:
print("No match found.")
替换模式:
replaced_text = re.sub(pattern, '456', text)
print(replaced_text)
3. 在HTML内容中使用正则表达式
你可以使用正则表达式在HTML内容中查找特定的模式。例如,查找所有的链接:
html_content = '''
<html>
<body>
<a href="http://example.com">Example</a>
<a href="http://example.org">Example</a>
</body>
</html>
'''
pattern = r'href="(http[s]?://.*?)"'
links = re.findall(pattern, html_content)
for link in links:
print(link)
四、通过SELENIUM进行网页自动化
Selenium是一个强大的工具,用于自动化网页浏览。它可以模拟用户与网页的互动,并提取动态加载的内容。
1. 安装和基础使用
首先,安装Selenium库和浏览器驱动。例如,使用Chrome浏览器:
pip install selenium
下载并安装ChromeDriver:
# 下载相应的ChromeDriver版本,并将其路径添加到系统环境变量中
2. 启动浏览器并访问网页
使用Selenium启动浏览器并访问网页:
from selenium import webdriver
driver = webdriver.Chrome()
driver.get('http://example.com')
3. 查找和提取数据
Selenium提供了多种方法来查找和提取网页中的数据,例如find_element_by_id()
, find_elements_by_class_name()
, find_element_by_xpath()
等。
例如,查找网页标题:
title = driver.title
print(f"Page Title: {title}")
查找所有的链接:
all_links = driver.find_elements_by_tag_name('a')
for link in all_links:
print(link.get_attribute('href'))
4. 处理动态内容和表单
Selenium还可以处理动态加载的内容和表单。例如,点击按钮、输入文本、提交表单等。
点击按钮:
button = driver.find_element_by_id('submit-button')
button.click()
输入文本并提交表单:
input_field = driver.find_element_by_name('username')
input_field.send_keys('testuser')
form = driver.find_element_by_id('login-form')
form.submit()
五、总结
在Python项目中查找HTML页面的方法多种多样,使用BeautifulSoup解析HTML、利用requests库获取页面内容、使用正则表达式进行文本搜索、通过Selenium进行网页自动化等方法各有优劣。具体选择取决于项目需求和复杂程度。BeautifulSoup适合处理静态HTML内容,requests库用于发送HTTP请求,正则表达式用于模式匹配和文本操作,Selenium适合处理动态加载的内容和模拟用户操作。根据具体需求选择合适的方法,可以提高工作效率并获得更好的结果。
相关问答FAQs:
如何在Python项目中查找特定的HTML页面?
在Python项目中,可以使用不同的方法来查找特定的HTML页面。常见的方式包括利用文件系统模块,如os
和glob
,通过遍历项目目录来定位特定的HTML文件。此外,使用框架如Flask或Django时,可以通过路由和视图函数来定义和查找HTML页面。
有哪些工具可以帮助我在Python项目中搜索HTML文件?
有多种工具可以帮助搜索HTML文件,例如使用文本编辑器或IDE的全局搜索功能。Visual Studio Code、PyCharm等IDE允许用户快速找到项目中的HTML文件。此外,命令行工具如grep
也可以用于在文件中搜索特定的关键词,从而找到相关的HTML页面。
如何在Python爬虫中提取HTML页面的内容?
在Python爬虫中,提取HTML页面内容通常使用requests
库获取网页内容,并结合BeautifulSoup
库解析HTML结构。通过查找特定标签、类名或ID,可以轻松提取所需的信息。爬虫还可以处理不同的网页结构和动态内容,确保获取到最新的数据。
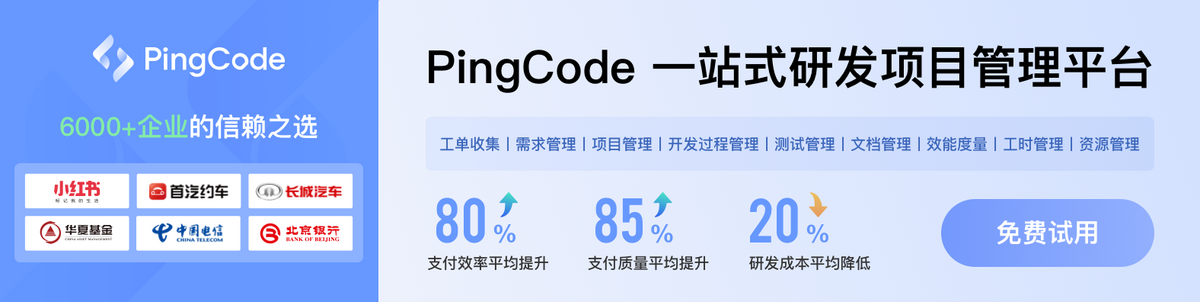