在Python中,设置布尔值非常简单。布尔值只有两个可能的取值:True 和 False。要设置布尔值,只需将变量赋值为这两个值之一即可。布尔值常用于条件判断、逻辑运算、控制程序流程。例如:
is_active = True
is_logged_in = False
布尔值在条件判断中非常常见。例如,我们可以使用布尔值来控制程序的执行流程:
if is_active:
print("The user is active.")
else:
print("The user is not active.")
下面我们将详细介绍Python中如何设置布尔值及其相关应用,包括布尔值的基本用法、在条件判断中的应用、逻辑运算、布尔值在函数中的应用等。
一、布尔值的基本用法
布尔值是Python中的一种基本数据类型,用于表示逻辑上的真或假。布尔值的基本用法如下:
1、直接赋值
直接将布尔值赋值给变量是最简单的方式:
is_valid = True
is_error = False
2、布尔运算
布尔运算是指对布尔值进行逻辑运算,如与(and)、或(or)、非(not)等。以下是一些示例:
a = True
b = False
与运算
result = a and b # result 为 False
或运算
result = a or b # result 为 True
非运算
result = not a # result 为 False
二、条件判断中的应用
布尔值在条件判断中非常常见。我们可以使用布尔值来控制程序的执行流程:
1、if语句
if语句用于根据布尔值的真或假来执行不同的代码块:
is_active = True
if is_active:
print("The user is active.")
else:
print("The user is not active.")
2、if-elif-else语句
if-elif-else语句允许我们在多个条件之间进行选择:
status = "active"
if status == "active":
print("The user is active.")
elif status == "inactive":
print("The user is not active.")
else:
print("Unknown status.")
三、逻辑运算
Python支持多种逻辑运算符,这些运算符可以用于布尔值的计算。
1、与运算符(and)
与运算符用于判断多个条件是否同时为真:
a = True
b = False
if a and b:
print("Both are true.")
else:
print("One or both are false.")
2、或运算符(or)
或运算符用于判断至少一个条件为真:
a = True
b = False
if a or b:
print("At least one is true.")
else:
print("Both are false.")
3、非运算符(not)
非运算符用于取反布尔值:
a = True
if not a:
print("a is false.")
else:
print("a is true.")
四、布尔值在函数中的应用
布尔值在函数中也有广泛应用,常用于返回函数的执行结果或控制函数的执行流程。
1、返回布尔值
函数可以返回布尔值,用于表示某个条件是否满足:
def is_even(number):
return number % 2 == 0
result = is_even(4) # result 为 True
result = is_even(5) # result 为 False
2、作为函数参数
布尔值可以作为函数的参数,用于控制函数的执行行为:
def print_message(is_active):
if is_active:
print("The user is active.")
else:
print("The user is not active.")
print_message(True) # 输出 "The user is active."
print_message(False) # 输出 "The user is not active."
五、布尔值与其他数据类型的关系
在Python中,其他数据类型的值可以转换为布尔值,常用于条件判断。
1、数值类型
在布尔上下文中,数值类型的0被视为False,其他值被视为True:
if 0:
print("This will not be printed.")
if 1:
print("This will be printed.")
2、字符串类型
在布尔上下文中,空字符串被视为False,非空字符串被视为True:
if "":
print("This will not be printed.")
if "hello":
print("This will be printed.")
3、列表类型
在布尔上下文中,空列表被视为False,非空列表被视为True:
if []:
print("This will not be printed.")
if [1, 2, 3]:
print("This will be printed.")
六、布尔值在循环中的应用
布尔值在循环中也有广泛应用,常用于控制循环的执行。
1、while循环
while循环根据布尔值的真或假来决定是否继续执行循环体:
is_running = True
count = 0
while is_running:
print("Running...")
count += 1
if count >= 5:
is_running = False
2、for循环
for循环可以结合布尔值来控制循环的执行流程:
numbers = [1, 2, 3, 4, 5]
found = False
for number in numbers:
if number == 3:
found = True
break
if found:
print("Number 3 was found.")
else:
print("Number 3 was not found.")
七、布尔值在数据结构中的应用
布尔值在数据结构中也有广泛应用,常用于表示某种状态或条件。
1、字典中的布尔值
字典中的布尔值常用于表示某个键的状态:
user_status = {
"is_active": True,
"is_admin": False
}
if user_status["is_active"]:
print("The user is active.")
if not user_status["is_admin"]:
print("The user is not an admin.")
2、列表中的布尔值
列表中的布尔值常用于表示多个元素的状态:
tasks_status = [True, False, True, False]
for index, status in enumerate(tasks_status):
if status:
print(f"Task {index + 1} is complete.")
else:
print(f"Task {index + 1} is not complete.")
八、布尔值在面向对象编程中的应用
布尔值在面向对象编程中也有广泛应用,常用于表示对象的属性或方法的返回值。
1、对象的布尔属性
对象的布尔属性常用于表示对象的某种状态:
class User:
def __init__(self, is_active, is_admin):
self.is_active = is_active
self.is_admin = is_admin
user = User(True, False)
if user.is_active:
print("The user is active.")
if not user.is_admin:
print("The user is not an admin.")
2、方法的布尔返回值
对象的方法可以返回布尔值,用于表示某个条件是否满足:
class Number:
def __init__(self, value):
self.value = value
def is_even(self):
return self.value % 2 == 0
number = Number(4)
if number.is_even():
print("The number is even.")
else:
print("The number is odd.")
九、布尔值在异常处理中的应用
布尔值在异常处理中也有广泛应用,常用于表示是否捕获到异常或某个操作是否成功。
1、布尔值表示操作成功
我们可以使用布尔值来表示某个操作是否成功:
def divide(a, b):
if b == 0:
return False, "Division by zero"
else:
return True, a / b
success, result = divide(4, 2)
if success:
print(f"Result: {result}")
else:
print(f"Error: {result}")
2、布尔值表示异常捕获
我们可以使用布尔值来表示是否捕获到异常:
def safe_divide(a, b):
try:
result = a / b
return True, result
except ZeroDivisionError:
return False, "Division by zero"
success, result = safe_divide(4, 0)
if success:
print(f"Result: {result}")
else:
print(f"Error: {result}")
十、布尔值在单元测试中的应用
布尔值在单元测试中也有广泛应用,常用于表示测试用例是否通过。
1、布尔值表示测试结果
我们可以使用布尔值来表示测试用例是否通过:
def test_is_even():
assert is_even(4) == True
assert is_even(5) == False
test_is_even()
print("All tests passed.")
2、使用单元测试框架
单元测试框架如unittest提供了丰富的断言方法,这些方法通常返回布尔值来表示测试结果:
import unittest
class TestMathFunctions(unittest.TestCase):
def test_is_even(self):
self.assertTrue(is_even(4))
self.assertFalse(is_even(5))
if __name__ == "__main__":
unittest.main()
十一、布尔值在数据分析中的应用
布尔值在数据分析中也有广泛应用,常用于数据筛选、条件判断等。
1、数据筛选
我们可以使用布尔值来筛选数据,如筛选出满足某个条件的行:
import pandas as pd
data = pd.DataFrame({
"name": ["Alice", "Bob", "Charlie"],
"age": [25, 30, 35]
})
筛选出年龄大于30的行
filtered_data = data[data["age"] > 30]
print(filtered_data)
2、条件判断
我们可以使用布尔值来进行条件判断,如判断某列是否满足某个条件:
data["is_adult"] = data["age"] >= 18
print(data)
十二、布尔值在网络编程中的应用
布尔值在网络编程中也有广泛应用,常用于表示网络连接状态、请求结果等。
1、表示网络连接状态
我们可以使用布尔值来表示网络连接状态:
import socket
def check_connection(host, port):
try:
socket.create_connection((host, port))
return True
except OSError:
return False
is_connected = check_connection("www.google.com", 80)
if is_connected:
print("Connected to the internet.")
else:
print("Not connected to the internet.")
2、表示请求结果
我们可以使用布尔值来表示HTTP请求是否成功:
import requests
def fetch_url(url):
try:
response = requests.get(url)
response.raise_for_status()
return True, response.text
except requests.RequestException as e:
return False, str(e)
success, content = fetch_url("https://www.example.com")
if success:
print("Request successful.")
else:
print(f"Request failed: {content}")
十三、布尔值在游戏开发中的应用
布尔值在游戏开发中也有广泛应用,常用于表示游戏状态、控制游戏逻辑等。
1、表示游戏状态
我们可以使用布尔值来表示游戏是否在进行中:
class Game:
def __init__(self):
self.is_running = True
def start(self):
while self.is_running:
# 游戏逻辑
print("Game is running...")
# 假设某个条件满足,游戏结束
self.is_running = False
game = Game()
game.start()
2、控制游戏逻辑
我们可以使用布尔值来控制游戏中的特定逻辑,如玩家是否获胜:
class Player:
def __init__(self):
self.has_won = False
def win(self):
self.has_won = True
player = Player()
假设某个条件满足,玩家获胜
player.win()
if player.has_won:
print("Player has won the game.")
十四、布尔值在配置管理中的应用
布尔值在配置管理中也有广泛应用,常用于表示某个配置项是否启用、某个功能是否激活等。
1、表示配置项是否启用
我们可以使用布尔值来表示某个配置项是否启用:
config = {
"enable_feature_x": True,
"enable_feature_y": False
}
if config["enable_feature_x"]:
print("Feature X is enabled.")
if not config["enable_feature_y"]:
print("Feature Y is disabled.")
2、表示功能是否激活
我们可以使用布尔值来表示某个功能是否激活:
class Feature:
def __init__(self):
self.is_active = False
def activate(self):
self.is_active = True
feature = Feature()
激活功能
feature.activate()
if feature.is_active:
print("Feature is active.")
十五、布尔值在安全领域中的应用
布尔值在安全领域中也有广泛应用,常用于表示认证状态、权限检查等。
1、表示认证状态
我们可以使用布尔值来表示用户是否已认证:
class User:
def __init__(self):
self.is_authenticated = False
def authenticate(self):
self.is_authenticated = True
user = User()
用户认证
user.authenticate()
if user.is_authenticated:
print("User is authenticated.")
else:
print("User is not authenticated.")
2、权限检查
我们可以使用布尔值来进行权限检查,判断用户是否有权限进行某个操作:
class User:
def __init__(self, is_admin):
self.is_admin = is_admin
def check_permission(user):
if user.is_admin:
print("User has admin privileges.")
else:
print("User does not have admin privileges.")
user = User(True)
check_permission(user)
user = User(False)
check_permission(user)
总结
通过以上内容,我们详细介绍了Python中如何设置布尔值及其相关应用。布尔值是Python中的一种基本数据类型,广泛应用于条件判断、逻辑运算、控制程序流程等方面。在实际开发中,我们可以灵活运用布尔值来实现各种功能,提高代码的可读性和维护性。无论是在简单的条件判断还是复杂的逻辑控制中,布尔值都是一个非常重要的工具。希望通过本文的介绍,您能够更好地理解和使用Python中的布尔值。
相关问答FAQs:
如何在Python中定义布尔变量?
在Python中,布尔值通常用True
和False
表示。您可以直接将这些值赋给变量,例如:
is_active = True
is_completed = False
这样您就成功创建了布尔变量,可以在后续的逻辑判断中使用。
布尔值与条件语句的关系是什么?
布尔值在条件语句中起着至关重要的作用。Python中的if
语句会根据布尔表达式的值来决定执行哪个代码块。例如:
if is_active:
print("活动已启用")
else:
print("活动未启用")
在这个例子中,is_active
的值决定了输出的内容。
如何将其他数据类型转换为布尔值?
Python提供了bool()
函数,可以将其他数据类型转换为布尔值。非零数字、非空字符串或非空容器(如列表、元组等)会被转换为True
,而零、空字符串和空容器会被转换为False
。示例代码如下:
print(bool(0)) # 输出: False
print(bool(5)) # 输出: True
print(bool("")) # 输出: False
print(bool("Hello")) # 输出: True
这种类型转换在编写条件判断时非常有用。
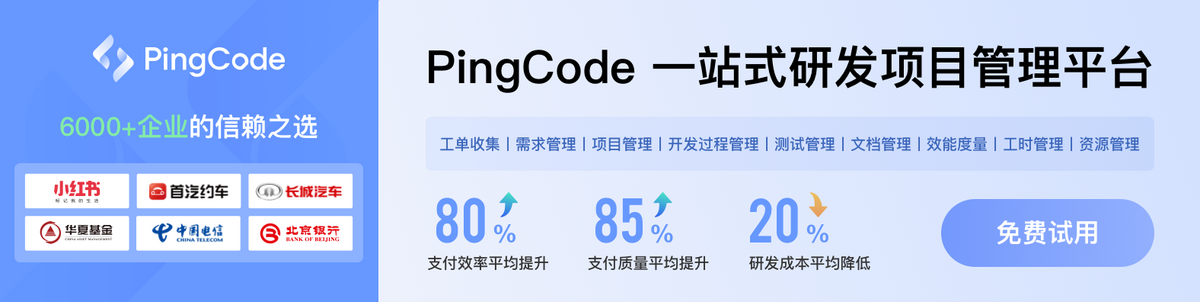