在开发Python爬虫时,如何获取最大页数是一个常见的问题。获取最大页数的方法有多种,主要包括解析网页中的页码信息、检测是否有“下一页”按钮、使用API请求等。其中,解析网页中的页码信息是一种常见且有效的方法。通过分析网页的源代码,找到包含页码信息的HTML元素,然后提取出最大页数。
例如,假设你正在爬取一个论坛或电商网站的商品列表页,你可以通过查看网页的分页部分,找到包含最大页码的HTML标签,如<div class="pagination">
或 <ul class="pagination">
等,然后使用BeautifulSoup或正则表达式提取出最大页码。
一、解析网页中的页码信息
解析网页中的页码信息是获取最大页数的常见方法之一。通过查看网页源代码,找到包含页码信息的HTML元素,然后提取出最大页数。
1. 使用BeautifulSoup解析页码信息
BeautifulSoup是一个用于解析HTML和XML文档的Python库,可以轻松地提取网页中的特定信息。以下是一个示例代码,演示如何使用BeautifulSoup解析网页中的页码信息:
import requests
from bs4 import BeautifulSoup
def get_max_page(url):
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
# 假设最大页码在class为pagination的div中
pagination = soup.find('div', class_='pagination')
if pagination:
pages = pagination.find_all('a')
max_page = max(int(page.text) for page in pages if page.text.isdigit())
return max_page
return 1
url = 'https://example.com/listing'
max_page = get_max_page(url)
print(f"最大页数为: {max_page}")
在这个示例中,我们首先发送HTTP请求获取网页内容,然后使用BeautifulSoup解析网页。通过查找包含分页信息的<div>
标签,提取出所有页码链接,并找到最大的页码。
2. 使用正则表达式提取页码信息
正则表达式是一种强大的字符串匹配工具,可以用于从网页内容中提取特定信息。以下是一个示例代码,演示如何使用正则表达式提取网页中的最大页码:
import requests
import re
def get_max_page(url):
response = requests.get(url)
content = response.text
# 假设最大页码在pagination的div中
pattern = re.compile(r'<div class="pagination">.*?</div>', re.S)
match = pattern.search(content)
if match:
pagination = match.group(0)
pages = re.findall(r'\d+', pagination)
max_page = max(int(page) for page in pages)
return max_page
return 1
url = 'https://example.com/listing'
max_page = get_max_page(url)
print(f"最大页数为: {max_page}")
在这个示例中,我们首先发送HTTP请求获取网页内容,然后使用正则表达式匹配包含分页信息的<div>
标签。通过提取所有页码,并找到最大的页码。
二、检测是否有“下一页”按钮
另一种获取最大页数的方法是检测是否有“下一页”按钮。通过判断网页中是否存在“下一页”按钮,可以确定是否有更多的页面。
1. 使用BeautifulSoup检测“下一页”按钮
以下是一个示例代码,演示如何使用BeautifulSoup检测网页中是否有“下一页”按钮:
import requests
from bs4 import BeautifulSoup
def get_max_page(url):
current_page = 1
while True:
response = requests.get(url, params={'page': current_page})
soup = BeautifulSoup(response.text, 'html.parser')
next_button = soup.find('a', class_='next')
if next_button:
current_page += 1
else:
break
return current_page
url = 'https://example.com/listing'
max_page = get_max_page(url)
print(f"最大页数为: {max_page}")
在这个示例中,我们通过循环发送HTTP请求,逐页获取网页内容,并使用BeautifulSoup检测是否存在“下一页”按钮。如果存在,则继续下一页;如果不存在,则停止循环,并返回当前页码。
2. 使用正则表达式检测“下一页”按钮
以下是一个示例代码,演示如何使用正则表达式检测网页中是否有“下一页”按钮:
import requests
import re
def get_max_page(url):
current_page = 1
while True:
response = requests.get(url, params={'page': current_page})
content = response.text
next_button = re.search(r'class="next"', content)
if next_button:
current_page += 1
else:
break
return current_page
url = 'https://example.com/listing'
max_page = get_max_page(url)
print(f"最大页数为: {max_page}")
在这个示例中,我们通过循环发送HTTP请求,逐页获取网页内容,并使用正则表达式检测是否存在“下一页”按钮。如果存在,则继续下一页;如果不存在,则停止循环,并返回当前页码。
三、使用API请求获取最大页数
有些网站提供API接口,可以直接通过API请求获取最大页数。这种方法通常更为简单和高效,因为API返回的结果通常是结构化的数据,容易解析。
1. 使用JSON API请求获取最大页数
以下是一个示例代码,演示如何使用JSON API请求获取最大页数:
import requests
def get_max_page(api_url):
response = requests.get(api_url)
data = response.json()
# 假设最大页码在total_pages字段中
max_page = data.get('total_pages', 1)
return max_page
api_url = 'https://api.example.com/listing'
max_page = get_max_page(api_url)
print(f"最大页数为: {max_page}")
在这个示例中,我们通过发送API请求获取JSON数据,并从中提取出最大页码。
2. 使用XML API请求获取最大页数
以下是一个示例代码,演示如何使用XML API请求获取最大页数:
import requests
from xml.etree import ElementTree
def get_max_page(api_url):
response = requests.get(api_url)
tree = ElementTree.fromstring(response.content)
# 假设最大页码在total_pages标签中
max_page = int(tree.find('total_pages').text)
return max_page
api_url = 'https://api.example.com/listing'
max_page = get_max_page(api_url)
print(f"最大页数为: {max_page}")
在这个示例中,我们通过发送API请求获取XML数据,并使用ElementTree解析XML,提取出最大页码。
四、结合多种方法获取最大页数
在实际项目中,为了提高爬虫的健壮性和适应性,通常会结合多种方法来获取最大页数。例如,先尝试使用API请求获取最大页数,如果API不可用或返回错误,则退而求其次,解析网页中的页码信息或检测“下一页”按钮。
1. 示例代码:结合多种方法获取最大页数
以下是一个示例代码,演示如何结合多种方法来获取最大页数:
import requests
from bs4 import BeautifulSoup
import re
def get_max_page(api_url=None, web_url=None):
if api_url:
try:
response = requests.get(api_url)
data = response.json()
max_page = data.get('total_pages', 1)
return max_page
except Exception as e:
print(f"API请求失败: {e}")
if web_url:
try:
response = requests.get(web_url)
soup = BeautifulSoup(response.text, 'html.parser')
pagination = soup.find('div', class_='pagination')
if pagination:
pages = pagination.find_all('a')
max_page = max(int(page.text) for page in pages if page.text.isdigit())
return max_page
except Exception as e:
print(f"解析网页失败: {e}")
return 1
api_url = 'https://api.example.com/listing'
web_url = 'https://example.com/listing'
max_page = get_max_page(api_url=api_url, web_url=web_url)
print(f"最大页数为: {max_page}")
在这个示例中,我们首先尝试使用API请求获取最大页数,如果API请求失败,则退而求其次,解析网页中的页码信息,最终返回最大页数。
五、处理特殊情况
在实际爬虫开发中,可能会遇到一些特殊情况,如网页内容动态加载、分页信息隐藏在JavaScript中等。针对这些情况,可以使用以下方法处理:
1. 处理动态加载网页
对于动态加载的网页,可以使用Selenium等浏览器自动化工具,模拟浏览器行为,获取动态加载后的网页内容。
from selenium import webdriver
def get_max_page(url):
driver = webdriver.Chrome()
driver.get(url)
# 等待页面加载完成
driver.implicitly_wait(10)
# 获取最大页码
pagination = driver.find_element_by_class_name('pagination')
pages = pagination.find_elements_by_tag_name('a')
max_page = max(int(page.text) for page in pages if page.text.isdigit())
driver.quit()
return max_page
url = 'https://example.com/listing'
max_page = get_max_page(url)
print(f"最大页数为: {max_page}")
在这个示例中,我们使用Selenium模拟浏览器行为,访问动态加载的网页,并提取最大页码。
2. 处理分页信息隐藏在JavaScript中的情况
对于分页信息隐藏在JavaScript中的网页,可以使用正则表达式从JavaScript代码中提取分页信息。
import requests
import re
def get_max_page(url):
response = requests.get(url)
content = response.text
# 假设最大页码在某个JavaScript变量中
pattern = re.compile(r'var totalPages = (\d+);')
match = pattern.search(content)
if match:
max_page = int(match.group(1))
return max_page
return 1
url = 'https://example.com/listing'
max_page = get_max_page(url)
print(f"最大页数为: {max_page}")
在这个示例中,我们通过正则表达式从JavaScript代码中提取最大页码。
六、总结
在开发Python爬虫时,获取最大页数是一个常见的问题,可以通过多种方法解决。解析网页中的页码信息、检测是否有“下一页”按钮、使用API请求是常见且有效的方法。结合多种方法可以提高爬虫的健壮性和适应性。此外,对于动态加载网页或分页信息隐藏在JavaScript中的情况,可以使用Selenium或正则表达式进行处理。
通过上述方法,可以有效地获取网页的最大页数,为后续的数据爬取提供必要的信息。希望这些方法和示例代码能够帮助你更好地开发Python爬虫,解决获取最大页数的问题。
相关问答FAQs:
如何在Python爬虫中获取最大页数?
要获取最大页数,通常需要分析网页的结构。很多网站在分页时会在最后一页的链接中提供页数信息,可以通过解析该链接来获取最大页数。此外,使用BeautifulSoup等库提取页面内容,查找相关的分页元素也是一种常见方法。
在获取最大页数时需要注意哪些问题?
在获取最大页数时,可能会遇到反爬虫机制。许多网站会限制频繁请求,甚至会返回错误页面。因此,使用适当的请求间隔和代理可以帮助绕过这些限制。同时,确保遵循网站的robots.txt文件,尊重网站的爬虫政策。
如何处理动态加载的页面以获取最大页数?
对于动态加载的页面,使用Selenium等工具可以模拟浏览器操作,从而获取加载后的完整页面内容。通过分析AJAX请求,直接调用API接口也是一种高效的获取最大页数的方法。确保对请求的数据进行解析,提取出页数信息。
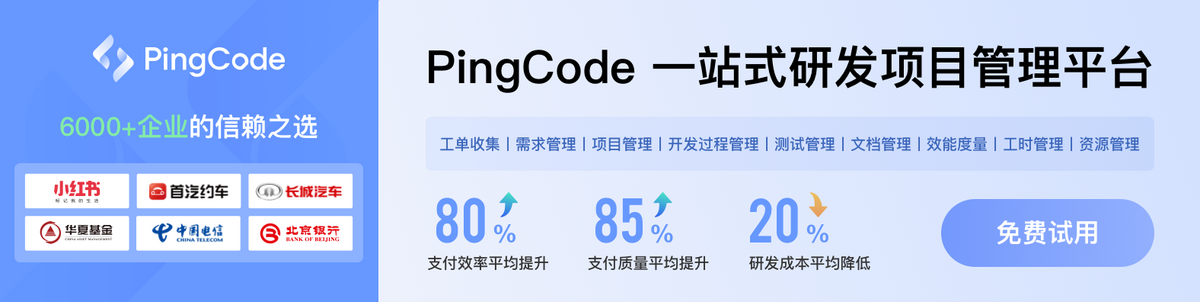