Python读取Word文档中的标题可以使用Python的python-docx
库、使用文档对象模型(DOM)解析文档、利用样式和级别区分标题。下面将详细描述如何使用这些方法来读取Word文档中的标题。
使用python-docx
库
python-docx
是一个用于处理Word文档的Python库。它可以方便地读取、修改和创建Word文档。要读取Word文档中的标题,可以通过检查段落的样式属性来识别标题。
安装python-docx
首先,需要安装python-docx
库。可以使用pip进行安装:
pip install python-docx
读取Word文档中的标题
接下来,我们可以使用python-docx
库来读取Word文档中的标题。下面是一个示例代码:
from docx import Document
def read_word_titles(file_path):
document = Document(file_path)
titles = []
for paragraph in document.paragraphs:
if paragraph.style.name.startswith('Heading'):
titles.append(paragraph.text)
return titles
示例使用
file_path = 'example.docx'
titles = read_word_titles(file_path)
for title in titles:
print(title)
在这个示例中,首先使用Document
类加载Word文档。然后遍历文档中的所有段落,检查每个段落的样式名称是否以“Heading”开头。如果是,则将该段落的文本视为标题并添加到titles
列表中。
使用文档对象模型(DOM)解析文档
另一种读取Word文档中的标题的方法是使用文档对象模型(DOM)来解析文档。这种方法适用于更复杂的需求,例如需要对文档进行更多的自定义处理。
可以使用lxml
库来解析Word文档的XML结构。下面是一个示例代码:
安装lxml
首先,需要安装lxml
库。可以使用pip进行安装:
pip install lxml
解析Word文档中的标题
接下来,我们可以使用lxml
库来解析Word文档中的标题。下面是一个示例代码:
from lxml import etree
import zipfile
def read_word_titles(file_path):
with zipfile.ZipFile(file_path, 'r') as docx:
xml_content = docx.read('word/document.xml')
tree = etree.XML(xml_content)
namespaces = {'w': 'http://schemas.openxmlformats.org/wordprocessingml/2006/main'}
titles = []
for paragraph in tree.xpath('//w:p', namespaces=namespaces):
if any(paragraph.xpath('.//w:pStyle[@w:val="Heading1"]', namespaces=namespaces)):
titles.append(paragraph.xpath('.//w:t', namespaces=namespaces)[0].text)
return titles
示例使用
file_path = 'example.docx'
titles = read_word_titles(file_path)
for title in titles:
print(title)
在这个示例中,首先使用zipfile
库打开Word文档并读取其XML内容。然后使用lxml
库解析XML,并使用XPath查找样式为“Heading1”的段落。找到这些段落后,提取其文本并添加到titles
列表中。
结论
通过以上方法,可以方便地读取Word文档中的标题。使用python-docx
库是最简单的方法,而使用文档对象模型(DOM)解析文档则适用于更复杂的需求。无论选择哪种方法,都可以灵活地处理Word文档并提取所需的标题信息。下面将进一步详细描述每种方法的细节和扩展应用。
一、使用python-docx
库
1、基础使用
加载文档
要使用python-docx
库,首先需要加载Word文档。可以使用Document
类来完成这一操作:
from docx import Document
document = Document('example.docx')
遍历段落
加载文档后,可以遍历文档中的所有段落。每个段落都是一个Paragraph
对象:
for paragraph in document.paragraphs:
print(paragraph.text)
识别标题
可以通过检查段落的样式属性来识别标题。标题通常使用“Heading1”、“Heading2”等样式:
for paragraph in document.paragraphs:
if paragraph.style.name.startswith('Heading'):
print(paragraph.text)
2、扩展应用
读取不同级别的标题
可以进一步区分不同级别的标题,例如“Heading1”、“Heading2”等:
headings = {'Heading1': [], 'Heading2': [], 'Heading3': []}
for paragraph in document.paragraphs:
if paragraph.style.name in headings:
headings[paragraph.style.name].append(paragraph.text)
for heading, texts in headings.items():
print(f"{heading}:")
for text in texts:
print(f" {text}")
读取标题和内容
可以在读取标题的同时,读取每个标题下的内容:
current_heading = None
document_structure = {}
for paragraph in document.paragraphs:
if paragraph.style.name.startswith('Heading'):
current_heading = paragraph.text
document_structure[current_heading] = []
elif current_heading:
document_structure[current_heading].append(paragraph.text)
for heading, content in document_structure.items():
print(f"{heading}:")
for text in content:
print(f" {text}")
这种方法可以帮助我们构建文档结构,方便后续处理。
二、使用文档对象模型(DOM)解析文档
1、基础使用
读取XML内容
首先使用zipfile
库打开Word文档并读取其XML内容:
import zipfile
with zipfile.ZipFile('example.docx', 'r') as docx:
xml_content = docx.read('word/document.xml')
解析XML
使用lxml
库解析XML内容:
from lxml import etree
tree = etree.XML(xml_content)
namespaces = {'w': 'http://schemas.openxmlformats.org/wordprocessingml/2006/main'}
2、XPath查询
使用XPath查询可以方便地查找特定元素,例如标题段落:
titles = []
for paragraph in tree.xpath('//w:p', namespaces=namespaces):
if any(paragraph.xpath('.//w:pStyle[@w:val="Heading1"]', namespaces=namespaces)):
titles.append(paragraph.xpath('.//w:t', namespaces=namespaces)[0].text)
for title in titles:
print(title)
3、扩展应用
读取不同级别的标题
可以通过修改XPath查询,读取不同级别的标题:
headings = {'Heading1': [], 'Heading2': [], 'Heading3': []}
for level in headings:
for paragraph in tree.xpath(f'//w:p[w:pStyle/@w:val="{level}"]', namespaces=namespaces):
headings[level].append(paragraph.xpath('.//w:t', namespaces=namespaces)[0].text)
for heading, texts in headings.items():
print(f"{heading}:")
for text in texts:
print(f" {text}")
读取标题和内容
可以在读取标题的同时,读取每个标题下的内容:
current_heading = None
document_structure = {}
for paragraph in tree.xpath('//w:p', namespaces=namespaces):
if any(paragraph.xpath('.//w:pStyle[@w:val="Heading1"]', namespaces=namespaces)):
current_heading = paragraph.xpath('.//w:t', namespaces=namespaces)[0].text
document_structure[current_heading] = []
elif current_heading:
texts = [node.text for node in paragraph.xpath('.//w:t', namespaces=namespaces)]
if texts:
document_structure[current_heading].append(''.join(texts))
for heading, content in document_structure.items():
print(f"{heading}:")
for text in content:
print(f" {text}")
这种方法可以帮助我们构建文档结构,方便后续处理。
三、使用其他库
除了python-docx
和lxml
,还有其他一些库可以用于读取Word文档中的标题,例如pywin32
和comtypes
。
1、使用pywin32
pywin32
库可以通过COM接口与Word进行交互。这种方法适用于Windows操作系统,需要安装Microsoft Word。
安装pywin32
首先,需要安装pywin32
库。可以使用pip进行安装:
pip install pywin32
读取标题
下面是一个示例代码,使用pywin32
读取Word文档中的标题:
import win32com.client
def read_word_titles(file_path):
word = win32com.client.Dispatch("Word.Application")
doc = word.Documents.Open(file_path)
titles = []
for paragraph in doc.Paragraphs:
if paragraph.Style.NameLocal.startswith('Heading'):
titles.append(paragraph.Range.Text.strip())
doc.Close()
word.Quit()
return titles
示例使用
file_path = 'example.docx'
titles = read_word_titles(file_path)
for title in titles:
print(title)
在这个示例中,首先使用win32com.client.Dispatch
打开Word应用程序并加载文档。然后遍历文档中的所有段落,检查每个段落的样式名称是否以“Heading”开头。如果是,则将该段落的文本视为标题并添加到titles
列表中。
2、使用comtypes
comtypes
库也是一种与Word进行交互的方式,与pywin32
类似。
安装comtypes
首先,需要安装comtypes
库。可以使用pip进行安装:
pip install comtypes
读取标题
下面是一个示例代码,使用comtypes
读取Word文档中的标题:
import comtypes.client
def read_word_titles(file_path):
word = comtypes.client.CreateObject('Word.Application')
doc = word.Documents.Open(file_path)
titles = []
for paragraph in doc.Paragraphs:
if paragraph.Style.NameLocal.startswith('Heading'):
titles.append(paragraph.Range.Text.strip())
doc.Close()
word.Quit()
return titles
示例使用
file_path = 'example.docx'
titles = read_word_titles(file_path)
for title in titles:
print(title)
在这个示例中,首先使用comtypes.client.CreateObject
打开Word应用程序并加载文档。然后遍历文档中的所有段落,检查每个段落的样式名称是否以“Heading”开头。如果是,则将该段落的文本视为标题并添加到titles
列表中。
四、总结
通过以上几种方法,可以方便地读取Word文档中的标题。具体选择哪种方法可以根据实际需求和环境进行判断。
1、python-docx
库
优点: 简单易用,适合大多数常见需求。
缺点: 处理复杂文档结构时可能需要更多的自定义代码。
2、文档对象模型(DOM)解析
优点: 灵活性高,适合处理复杂文档结构。
缺点: 需要了解XML和XPath,代码相对复杂。
3、pywin32
和comtypes
优点: 可以通过COM接口直接与Word进行交互,功能强大。
缺点: 仅适用于Windows系统,需要安装Microsoft Word。
4、其他库
根据需求,还可以选择其他库,例如docx2txt
、textract
等。这些库也可以用于读取Word文档中的内容,但其功能和使用方法可能有所不同。
无论选择哪种方法,都可以灵活地处理Word文档并提取所需的标题信息。希望本文能帮助您更好地理解和应用这些方法。
相关问答FAQs:
如何使用Python读取Word文档中的所有标题?
要读取Word文档中的所有标题,可以使用python-docx
库。通过遍历文档中的段落,检查每个段落的样式是否为标题样式(如标题1、标题2等),即可提取出所有标题。具体代码示例如下:
from docx import Document
def extract_headings(file_path):
doc = Document(file_path)
headings = []
for para in doc.paragraphs:
if para.style.name.startswith('Heading'):
headings.append(para.text)
return headings
file_path = 'your_document.docx'
headings = extract_headings(file_path)
print(headings)
Python读取Word标题时可以自定义哪些样式?
在使用python-docx
库时,除了读取默认的标题样式(如标题1、标题2等),用户可以自定义样式,通过指定段落的样式名称来提取特定的标题。只需在代码中调整条件判断,添加用户定义的样式名称即可。
我该如何处理读取Word标题时遇到的错误?
在读取Word文档时,可能会遇到文件不存在、格式不支持或权限问题等错误。确保文件路径正确且文件格式为.docx
。此外,使用异常处理(try-except语句)可以有效捕捉和处理这些错误,确保程序的稳定性。例如:
try:
doc = Document(file_path)
except Exception as e:
print(f"Error reading the document: {e}")
这样可以提高代码的健壮性并方便调试。
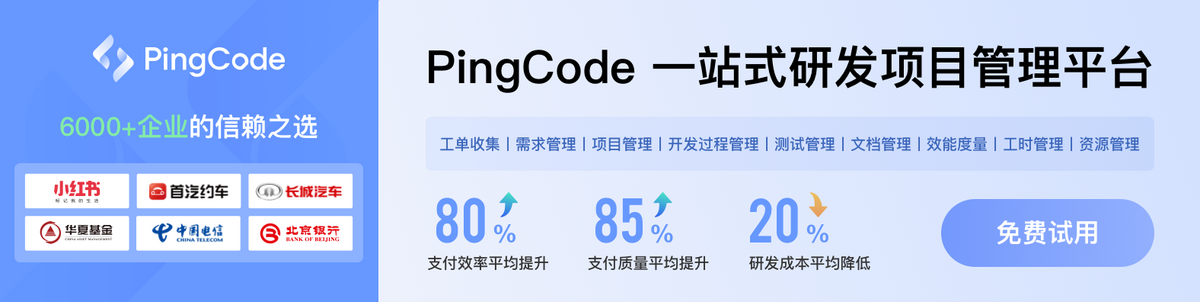