在Python中查找列表中的数值有多种方法。你可以使用in
运算符、index()
方法、count()
方法、列表推导式、filter()
函数、以及自定义的函数来查找特定数值。其中,使用in
运算符是最简单和直观的方式,而index()
方法可以精确查找到某个值的第一个出现位置。接下来,我们将详细介绍每一种方法,并给出相应的示例代码。
一、使用in
运算符
in
运算符用于检查一个元素是否存在于列表中。它返回一个布尔值,表示元素是否存在。
my_list = [1, 2, 3, 4, 5]
value_to_find = 3
if value_to_find in my_list:
print(f"{value_to_find} exists in the list.")
else:
print(f"{value_to_find} does not exist in the list.")
二、使用index()
方法
index()
方法用于查找某个元素在列表中的第一个出现位置。如果元素不存在,会抛出ValueError
异常。
my_list = [1, 2, 3, 4, 5]
value_to_find = 3
try:
index = my_list.index(value_to_find)
print(f"{value_to_find} found at index {index}.")
except ValueError:
print(f"{value_to_find} does not exist in the list.")
三、使用count()
方法
count()
方法用于统计某个元素在列表中出现的次数。可以用来判断元素是否存在以及出现的频率。
my_list = [1, 2, 3, 3, 4, 5]
value_to_find = 3
count = my_list.count(value_to_find)
print(f"{value_to_find} appears {count} times in the list.")
四、使用列表推导式
列表推导式是一种简洁的方式来筛选出列表中所有符合条件的元素。
my_list = [1, 2, 3, 3, 4, 5]
value_to_find = 3
matching_indices = [index for index, value in enumerate(my_list) if value == value_to_find]
print(f"Indices of {value_to_find}: {matching_indices}")
五、使用filter()
函数
filter()
函数用于过滤出符合条件的元素,可以与lambda
函数结合使用。
my_list = [1, 2, 3, 3, 4, 5]
value_to_find = 3
filtered_list = list(filter(lambda x: x == value_to_find, my_list))
print(f"Filtered list: {filtered_list}")
六、自定义函数
你还可以编写自定义函数来查找列表中的特定数值,例如查找所有出现位置或返回布尔值。
def find_indices(lst, value):
return [index for index, val in enumerate(lst) if val == value]
def value_exists(lst, value):
return value in lst
my_list = [1, 2, 3, 3, 4, 5]
value_to_find = 3
indices = find_indices(my_list, value_to_find)
exists = value_exists(my_list, value_to_find)
print(f"Indices of {value_to_find}: {indices}")
print(f"Does {value_to_find} exist in the list? {exists}")
七、查找多个数值
有时候你可能需要在列表中查找多个数值,可以使用集合或字典来实现这一点。
my_list = [1, 2, 3, 3, 4, 5]
values_to_find = [2, 3, 6]
使用集合
found_values = set(my_list).intersection(values_to_find)
print(f"Found values: {found_values}")
使用字典
found_indices = {value: [index for index, val in enumerate(my_list) if val == value] for value in values_to_find}
print(f"Found indices: {found_indices}")
八、处理嵌套列表
如果列表是嵌套的,即其中的元素本身也是列表,那么查找数值就需要递归方法。
def find_in_nested_list(nested_list, value):
indices = []
for index, element in enumerate(nested_list):
if isinstance(element, list):
nested_indices = find_in_nested_list(element, value)
for nested_index in nested_indices:
indices.append([index] + nested_index)
elif element == value:
indices.append([index])
return indices
nested_list = [1, [2, 3], [4, [3, 5]], 6]
value_to_find = 3
indices = find_in_nested_list(nested_list, value_to_find)
print(f"Indices of {value_to_find} in nested list: {indices}")
九、性能优化
在处理大列表时,性能可能会成为一个问题。你可以使用诸如set
、dict
等数据结构来加快查找速度。
import time
large_list = list(range(1000000))
value_to_find = 999999
start_time = time.time()
exists = value_to_find in large_list
end_time = time.time()
print(f"Using 'in' operator: {exists}, Time taken: {end_time - start_time} seconds")
使用 set 来优化
large_set = set(large_list)
start_time = time.time()
exists = value_to_find in large_set
end_time = time.time()
print(f"Using 'set': {exists}, Time taken: {end_time - start_time} seconds")
十、查找并操作
有时候在查找元素的同时,你可能需要对其进行某些操作。可以使用列表推导式或循环来实现。
my_list = [1, 2, 3, 3, 4, 5]
value_to_find = 3
new_value = 99
使用列表推导式
my_list = [new_value if x == value_to_find else x for x in my_list]
print(f"Updated list: {my_list}")
使用循环
for i in range(len(my_list)):
if my_list[i] == value_to_find:
my_list[i] = new_value
print(f"Updated list: {my_list}")
十一、总结
在Python中查找列表中的数值有多种方法,每种方法都有其适用的场景。使用in
运算符可以快速判断元素是否存在,使用index()
方法可以找到元素的第一个出现位置,使用count()
方法可以统计元素的出现次数,使用列表推导式和filter()
函数可以灵活地筛选元素,自定义函数可以满足更复杂的需求。对于处理大列表或嵌套列表,可以采用优化策略或递归方法。根据具体需求选择合适的方法,可以提高代码的效率和可读性。
相关问答FAQs:
如何在Python中查找列表中是否存在特定的数值?
在Python中,可以使用in
关键字来检查列表中是否存在某个特定的数值。例如,如果有一个列表my_list = [1, 2, 3, 4, 5]
,可以通过if 3 in my_list:
来判断3是否在列表中。这个方法简单直观,非常适合进行快速查找。
使用Python查找列表中的数值有何高效的方法?
对于较大的列表,可以考虑使用list.index()
方法来查找特定数值的索引位置。如果数值在列表中存在,my_list.index(3)
将返回数值3的索引;如果不存在,将引发ValueError
。为避免错误,可以先使用in
检查数值是否存在。
如何查找列表中所有符合条件的数值?
若希望查找列表中所有符合特定条件的数值,可以使用列表推导式。例如,若要查找所有大于2的数值,可以使用result = [x for x in my_list if x > 2]
。这种方法不仅高效,而且代码简洁,易于理解。
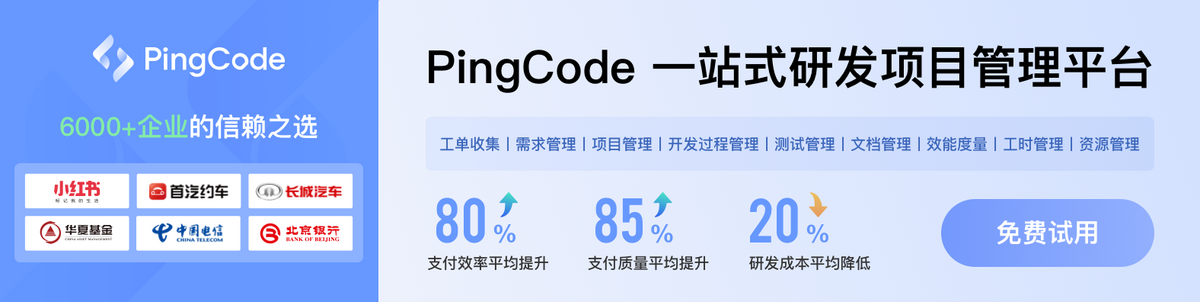