在Python中,定时装饰器是一种非常有效的工具,可以用于在特定时间间隔后执行某些函数。要实现定时装饰器,通常可以使用Python的标准库,如time
、threading
或者sched
来实现。其中一种简单而常用的方法是使用threading.Timer
类。通过将函数包装在装饰器内部,并使用计时器在指定时间间隔后调用该函数,可以轻松实现定时功能。
一、装饰器基础
1.1 什么是装饰器
装饰器是Python中的一种高级特性,允许你在不改变函数代码的情况下,添加额外的功能。装饰器本质上是一个返回函数的函数,它能够在函数调用前后执行额外的代码逻辑。
1.2 装饰器的基本形式
装饰器通常是一个函数,它接受另一个函数作为参数,并返回一个新的函数。示例如下:
def my_decorator(func):
def wrapper():
print("Something is happening before the function is called.")
func()
print("Something is happening after the function is called.")
return wrapper
@my_decorator
def say_hello():
print("Hello!")
say_hello()
在这个例子中,my_decorator
装饰了say_hello
函数,使得在say_hello
函数调用之前和之后,分别打印一些额外的内容。
二、使用threading.Timer
实现定时装饰器
2.1 基本实现
可以使用threading.Timer
来创建一个定时装饰器。threading.Timer
类允许你在指定的时间间隔之后执行某个函数。下面是一个基本的定时装饰器实现示例:
import threading
def timer_decorator(interval):
def decorator(func):
def wrapper(*args, kwargs):
def delayed_execution():
func(*args, kwargs)
timer = threading.Timer(interval, delayed_execution)
timer.start()
return wrapper
return decorator
@timer_decorator(5)
def greet():
print("Hello, world!")
greet()
在这个例子中,timer_decorator
是一个装饰器,它接受一个时间间隔(以秒为单位)。当装饰的函数被调用时,它会启动一个计时器,在指定的时间间隔之后执行该函数。
2.2 处理多次调用
上面的实现假设每次调用装饰的函数时,都会启动一个新的计时器。如果需要处理多次调用,可以改进装饰器,使得它能够处理多个计时器实例:
import threading
def timer_decorator(interval):
def decorator(func):
timers = []
def wrapper(*args, kwargs):
def delayed_execution():
func(*args, kwargs)
timers.remove(timer)
timer = threading.Timer(interval, delayed_execution)
timers.append(timer)
timer.start()
return wrapper
return decorator
@timer_decorator(5)
def greet():
print("Hello, world!")
greet()
greet()
在这个改进的版本中,timers
列表用于跟踪所有活动的计时器实例,并在计时器触发后将其从列表中移除。
三、使用sched
模块实现定时装饰器
3.1 基本实现
sched
模块提供了一种更高级的调度机制,允许你安排在特定时间或时间间隔之后执行函数。下面是一个使用sched
模块的定时装饰器示例:
import sched
import time
def scheduler_decorator(interval):
scheduler = sched.scheduler(time.time, time.sleep)
def decorator(func):
def wrapper(*args, kwargs):
def delayed_execution():
func(*args, kwargs)
scheduler.enter(interval, 1, delayed_execution)
scheduler.run(blocking=False)
return wrapper
return decorator
@scheduler_decorator(5)
def greet():
print("Hello, world!")
greet()
在这个例子中,scheduler_decorator
使用sched.scheduler
来安排函数在指定时间间隔之后执行。
3.2 优化并处理多次调用
可以进一步优化装饰器,使其能够处理多次调用并执行所有计划的任务:
import sched
import time
import threading
def scheduler_decorator(interval):
scheduler = sched.scheduler(time.time, time.sleep)
def decorator(func):
def wrapper(*args, kwargs):
def delayed_execution():
func(*args, kwargs)
scheduler.enter(interval, 1, delayed_execution)
threading.Thread(target=scheduler.run, args=(False,)).start()
return wrapper
return decorator
@scheduler_decorator(5)
def greet():
print("Hello, world!")
greet()
greet()
在这个改进的版本中,使用线程来运行调度器,以确保每次调用装饰的函数时,都能够安排并执行所有计划的任务。
四、实际应用
4.1 定时任务调度
定时装饰器在实际应用中非常有用,尤其是在需要定期执行某些任务的场景中。例如,可以用它来定期检查数据库更新,发送定时通知,或者执行周期性的清理任务。
4.2 简化代码
使用定时装饰器可以简化代码,使定时任务的实现变得更加简洁和可读。通过将定时逻辑封装在装饰器中,可以避免重复代码,并提高代码的可维护性。
4.3 异步定时任务
在某些情况下,可能需要执行异步定时任务。可以结合asyncio
模块来实现异步定时装饰器。例如:
import asyncio
def async_timer_decorator(interval):
def decorator(func):
async def wrapper(*args, kwargs):
await asyncio.sleep(interval)
await func(*args, kwargs)
return wrapper
return decorator
@async_timer_decorator(5)
async def greet():
print("Hello, world!")
asyncio.run(greet())
在这个例子中,使用了asyncio.sleep
来实现异步定时功能,使得装饰的函数在指定时间间隔之后异步执行。
五、错误处理和日志记录
5.1 错误处理
在实际应用中,处理可能出现的错误是非常重要的。在装饰器中添加错误处理逻辑,可以确保在发生错误时,能够记录错误信息并继续执行其他任务。例如:
import threading
import logging
logging.basicConfig(level=logging.ERROR)
def timer_decorator(interval):
def decorator(func):
def wrapper(*args, kwargs):
def delayed_execution():
try:
func(*args, kwargs)
except Exception as e:
logging.error(f"Error occurred: {e}")
timer = threading.Timer(interval, delayed_execution)
timer.start()
return wrapper
return decorator
@timer_decorator(5)
def greet():
raise ValueError("An error occurred in greet function")
greet()
在这个例子中,如果greet
函数抛出异常,错误信息将被记录到日志中。
5.2 日志记录
除了错误处理外,日志记录也是非常重要的,特别是在调试和监控定时任务时。可以在装饰器中添加日志记录,记录函数的调用和执行时间。例如:
import threading
import logging
logging.basicConfig(level=logging.INFO)
def timer_decorator(interval):
def decorator(func):
def wrapper(*args, kwargs):
def delayed_execution():
logging.info(f"Executing {func.__name__} after {interval} seconds")
start_time = time.time()
func(*args, kwargs)
end_time = time.time()
logging.info(f"Execution of {func.__name__} took {end_time - start_time} seconds")
timer = threading.Timer(interval, delayed_execution)
timer.start()
return wrapper
return decorator
@timer_decorator(5)
def greet():
print("Hello, world!")
greet()
在这个例子中,日志记录了函数的调用和执行时间,便于监控和调试。
六、高级应用
6.1 可配置的定时装饰器
在某些情况下,可能需要一个更加灵活和可配置的定时装饰器。例如,可以允许用户指定不同的时间间隔和调度策略:
import threading
import time
def configurable_timer_decorator(interval, repeat=False):
def decorator(func):
def wrapper(*args, kwargs):
def delayed_execution():
func(*args, kwargs)
if repeat:
timer = threading.Timer(interval, delayed_execution)
timer.start()
timer = threading.Timer(interval, delayed_execution)
timer.start()
return wrapper
return decorator
@configurable_timer_decorator(5, repeat=True)
def greet():
print("Hello, world!")
greet()
在这个例子中,configurable_timer_decorator
允许用户指定是否重复执行装饰的函数。
6.2 结合其他装饰器使用
定时装饰器可以与其他装饰器结合使用,以实现更复杂的功能。例如,可以将定时装饰器与缓存装饰器结合,定期清理缓存:
import threading
import time
from functools import lru_cache
def timer_decorator(interval):
def decorator(func):
def wrapper(*args, kwargs):
def delayed_execution():
func(*args, kwargs)
timer = threading.Timer(interval, delayed_execution)
timer.start()
return wrapper
return decorator
@lru_cache(maxsize=128)
def expensive_operation(x):
time.sleep(2)
return x * x
@timer_decorator(10)
def clear_cache():
expensive_operation.cache_clear()
print(expensive_operation(4))
print(expensive_operation(4))
clear_cache()
在这个例子中,clear_cache
函数会每隔10秒清理一次缓存。
七、总结
定时装饰器在Python编程中是一个非常有用的工具,可以用于实现定时任务调度、简化代码、处理异步任务等。通过使用threading.Timer
、sched
模块或asyncio
,可以灵活地创建各种定时装饰器。结合错误处理和日志记录,可以进一步提高定时任务的可靠性和可维护性。高级应用还包括可配置的定时装饰器和与其他装饰器的结合使用,使得定时装饰器在实际应用中具有广泛的应用场景。
相关问答FAQs:
如何在Python中使用装饰器实现定时功能?
在Python中,可以通过结合装饰器和时间模块来实现定时功能。首先,您需要定义一个装饰器,该装饰器将接受一个函数作为参数,并在调用该函数之前或之后进行时间计算。可以使用time
模块中的time()
函数来获取当前时间,计算函数执行的持续时间,并在需要时进行延迟。
装饰器定时执行的常见应用场景有哪些?
装饰器定时执行可以广泛应用于多种场景,例如定时任务、性能监控、日志记录等。例如,您可以使用装饰器来定期调用某个函数以收集数据或执行清理操作。此类装饰器能帮助开发者减少代码重复,提高代码的可维护性。
如何控制装饰器的执行频率?
控制装饰器的执行频率可以通过增加参数来实现。您可以在装饰器中添加一个时间间隔参数,指定每次调用函数之间的最小间隔。例如,通过使用time.sleep()
函数来暂停执行,确保函数在指定的时间间隔后再次执行。这样的设计可以确保您的函数不会频繁执行,避免潜在的性能问题。
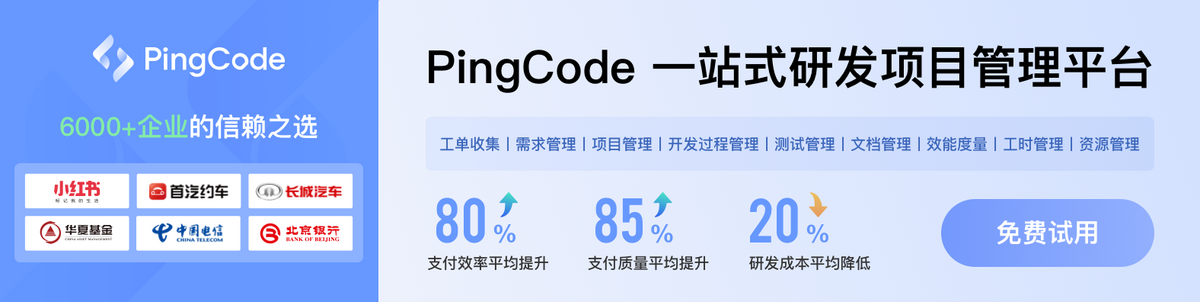